First, we need to allow the user to specify how many tickets will be printed. We'll have them select the number from a drop-down list.
1. Write the basic tags to create a drop-down list that consists of the numbers 1-4:
Reminders:
2. Give that drop-down list an id value of "ticketNumber" (i.e. add an id attribute to the <select> tag)
3. Save the page and view it in a browser. It should look like this:
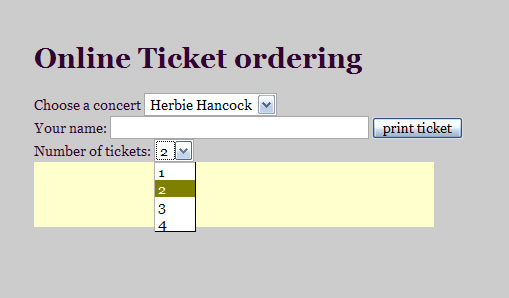
Now, we need to make more table cells where our tickets will be printed.
4. The existing cell looks like this:
<tr>
<td id="result" class="ticket"></td>
</tr>
copy this and paste it in 3 more times so you have 4 rows total (be sure they're all inside the <table> tags)
5. Now you have four cells that all have an id value of "result". That's actually illegal, each id must be unique. So change them to be "result0", "result1", "result2" and "result3"
Next, we'll modify our printTicket() function.
We need to find out which number the user selected. We'll put that number into a variable called "numberTickets". We could write it like this:
var numberTickets = document.getElementById("ticketNumber").value;
But there's one problem with this line. In order to understand this problem you need to understand the difference between text and numbers. JavaScript can interpret '5' as the number 5 or as a text character. What's the difference? If it sees it as the number '5', then if you ask it to do this: 5 + 5, it will give back "10", but if it sees it as text '5', then if you ask it to do this: 5 + 5, it will give back "55".
We need to be sure it sees this as a number, so we'll use the Number function like this:
var numberTickets = Number(document.getElementById("ticketNumber").value);
6. Go ahead and write the line above in your function (which is inside the <script> tags which are inside the <head> section of your page). Put it just below your other "var" statements.
7. Now let's be sure this is correct by adding the following alert statement right after the var statement:
alert("You have chosen to print " + numberTickets + " tickets");
8. Save your page. Open it in a browser. Choose a concert from the list, type in a name and choose a number of tickets. Then click on the button and see if the alert message appears.
If not..
- Did you write your drop-down list correctly? One way to check is to compare it with your other drop down list
- Did you put your statement and your alert statements in the correct location (in the function)?
9. Go to the Catalyst quiz and answer question #10