A variable is a name or label that can stand for different things at different times (like the label "professor" can stand for one individual when you're in one class, and a different individual when you're in another class). Since the "thing" the name stands for can vary, it is called a "variable".
In our case, the "things" that can vary are the name the user enters and the concert s/he chooses.
To create, or declare, a variable, you write the word "var" which is short for "variable" and then follow it with the name you made up, like this:
var chosenConcert;
In our case, we want the name "chosenConcert" to refer to whichever concert the user has chosen. We already know how to retrieve that choice (using document.getElementById().value). So we can go ahead and assign it to the variable by changing the line to look like this:
var chosenConcert = document.getElementById("concertChoice").value;
1. Type this line (the one above this) after your list of alert statements (but before the ending curly bracket).
2. Now type a similar line (starting with "var..." to declare a variable called "nameEntered" which will be the name the user entered (the id for that element is "yourName").
3. Now you'll use those variables to write a new alert statement that strings them together (put this line after the two var statements. If you put it before those statements, it wouldn't work because the variables don't exist yet):
alert(chosenConcert + nameEntered);
We no longer need the other alert statements any more, but you may not want to delete them (to be on the cautious side). To stop the browser from displaying them, you can just add two slashes in front of them: // and they will be ignored.

4. Save your file, then choose a concert from the list, enter a name and click the button. The alert box should show which concert you chose and what name you entered:
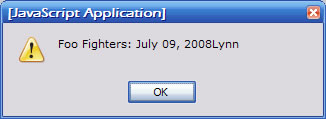
4. This alert statement doesn't make much sense by itself, so modify it to add some text that explains what the values are and add some space between words:
alert("MAIN PERFORMER: " + chosenConcert + " ATTENDEE: " + nameEntered);
Notice that the variable names are not in quotes. If they were in quotes, the browser would just think they were some more text to paste into the pop-up box. Since they are not in quotes, the browser knows that they are variable names and replaces them with whatever is currently in the variable.
5. Now save your file and open it in your browser. Choose a concert from the list, enter and name in the text box, and click on the button. You should see a pop-up-box with the phrase you created:
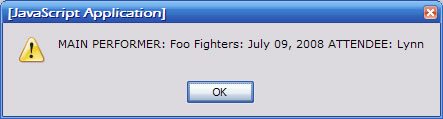
If it doesn't appear, check to make sure
- spelling is correct
- quotes are in the correct places
- plus symbols are in the correct places
- the alert statement ends with a semicolon
- the alert statement is between the curly brackets
When it does appear...congratulations!
6. Go to the Catalyst quick and answer question #8.
The next step is to print the ticket