CSE466 Lab 1: “One-Minute Timer”
Objectives
Create a device that will count from 00 to 59 on two 7-segment led displays
at a rate of 1Hz. When the end of the count is reached, it should wrap around and
start counting from the beginning. There will be an example setup in the lab
that you can use as a reference.
In this lab you will learn the following:
- AVR
assembly
- Basics
of the ATmega16 (Memory, registers, etc)
- Binary
to decimal 7-segment conversion
- Become
familiar with AVRStudio 4 (which has a simulator)
- Programming
and debugging with JTAG
Reading
Helpful Hints
- Instruction
set summary is on page 336-338 of the ATmega16 datasheet.
- Detailed
instruction descriptions available at Atmel's website.
Please don't print out (150 pages) as the rest of your programming will be
in C.
- General
purpose registers are r0-r31, watch for instructions that only operate on
some of them (ldi, load immediate, only works on r16-31)
- Include
[.include "m16def.inc"] to get logical names [PORTA] for
registers (as opposed to having to use addresses [$3B])
Resources
Part 1: Wiring up the breadboard
- Read all of the directions for Part 1 before doing
ANYTHING. Often the next step reveals why the current one is important.
- Wire the circuit in the schematic
on your breadboard. Make sure the power is disconnected when wiring up
your breadboard.
- The 7-segment LEDs displays are not connected to the ATmega16
in the schematic. You and your partner will need to decide how to connect
the 8 wires from the ATmega16 to the 7-segment LED displays. The wiring
pattern used should be exactly the same for both of the 7-segment displays
allowing the same constant to be used when displaying numbers. Make sure
you connect the decimal point of both 7-segment LEDs. Also note that the
7-segment LED displays combine to make the number (one will represent the
tens digit and the other will represent the ones digit). To make the
actual value of the counter easy to read it would be nice if the 7-segment
LEDs were either next to each other or aligned so the bottom of the
7-segment LEDs were parallel.
- The resistors values have been purposefully left off
the schematic. You will need to consult the datasheet to find the chip’s
electrical characteristics to determine the appropriate values of the
reset pull-up resistor and the current limiting resistors for the LEDs.
For decent brightness the 7-segment LEDs should have around 5mA of
current.
Question 1: There are 3 parts to this question to help you find a
reasonable range for your pull-up resistor (HINT: A reasonable range is
probably the same as the internal pull-up resistor). Even though the ATmega16
contains an internal pull-up resistor the goal of the question is to review how
to calculate a reasonable range for chips that do NOT have an internal pull-up
resistor. Keep in mind that you want to have large safety margins when choosing
the pull-up resistor to use in your reset circuit.
a. Calculate
the range of resistance values for the reset pull-up resistor. Refer to the
schematic to visualize the circuit in question.
The minimum
resistor value must be calculated for the case when the reset switch is
closed. When this switch is closed, Vcc
and ground are connected directly through the pull-up resistor. The maximum
current draw is limited by the 1/4 W resistors we use in this class. Calculate
the min resistor value given this information. Remember V = I * R and Power = V
* I.
The maximum
resistor value must be calculated for the case when the reset switch is open.
The minimum voltage for which the reset pin is considered high can be found on
page 291. For the reset circuit to function properly, the pull-up resistor must
allow at least a minimum amount of current to flow to the chip. A good
indication of what this minimum current should be is the maximum input leakage
current for I/O pins listed on page 291. Using the value of Vcc, minimum reset
high voltage and minimum input current values, calculate the max resistor
value.
What is the
theoretically largest possible range from the values you calculated? (List the
min/max values) In practice a more reliable system will narrow the range
significantly to minimize the risk of failure due to changing conditions.
b. The
ATMega16 includes an internal reset pull-up resistor, what is the range of this
resistor as specified in the datasheet (see page 291)?
c. Choose a pull-up resistor that is within the
acceptable range for the internal pull-up resistor. What resistance value did
you choose?
Question 2: Calculate the theoretical amount of current
flowing through a single segment of the 7-segment LEDs when it is
illuminated. In your calculation use only values from datasheets or
labels. Do not use any measured values in your calculation.
- Before powering on the breadboard, verify with a
multimeter that there are no shorts between power and ground, and that
power and ground are actually hooked up to the chips' pins.
- Turn on the JTAG interface and power up your
breadboard.
- To verify that your hardware is working properly,
program your wired up breadboard with flash.hex.
(This program should cause your 7-sement LEDs to flash “88”). The ATmega16
can be programmed directly with the development environment called AVR
Studio 4 that we’ll be using for half of the quarter. To do so:
- Open AVR Studio
4. If you’re presented with a dialog box like the one pictured below,
click the cancel button, since you are not creating your own program yet.

- Click the Con button on the toolbar, highlighted
below:
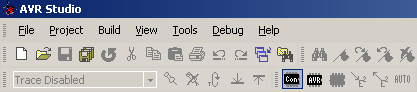
- Select JTAGICE mkII as the platform, and Auto as
the port:
(if you are using the AVRISP programmer, select AVRISP mkII, and set speed to 1.0 MHz)

- Select the ATmega16 as the device, and make sure
that JTAG is selected as the programming mode. If “Verify Device After
Programming” is checked, “Erase Device Before Programming” must also be
checked!

- Under the Flash section, press the program button,
navigate to where you saved the flash.hex file, and click OK.
- Assuming all
goes well, your board should begin flashing “88” in a few seconds.
Part 2: Create a simple program in Assembly
- Read all of the directions for Part 2 before doing
ANYTHING. Often the next step reveals why the current one is
important.
- Select
New Project from the Project menu.
- The
Project Type is “AVR Assembler”. Give
the project a name and change the location to a lab partner’s network
share. Then, hit next.

- Select
“JTAGICE mkII” as the Debug Platform and “ATmega16” as the Device then hit
finished.

- Copy
the following code to the assembly file. (The code should display an “8”
on one of the 7-segment LEDs)
.include "C:\Program Files\Atmel\AVR
Tools\AvrAssembler\Appnotes\m16def.inc"
.cseg
ldi r16, 0xff
out DDRB, r16
ldi r16, 0x00
out PORTB, r16
loop:
jmp loop
-
From the Project menu choose "Assembler options". Change Version 2 to Version 1. You will get a build error otherwise.
- Build
and run the project by selecting “Build and Run” from the Project menu, or
hit Ctrl-F7. This will cause the program to stop at the first instruction.
To run the program, select “Run” from the Debug menu, or press F5.
- Modify
the code to determine the constants needed to generate the other 9 numbers
on the 7-segment LED displays.
Part 3: Create a BCD Counter in Assembly
Read all of the directions for Part 3 before doing
ANYTHING.Write a program in assembly that will count from 00 to 59 on
two 7-segment led displays (one will be the tens digit and the other will be
the ones digit). Implement a lookup table in program memory to convert a 4-bit
nibble (in binary) to a decimal number. Use this skeleton program as a starting point. If you want
to use AVR Studio 4’s debugger, read this tutorial.
Requirements:
- Use a lookup table in program memory to store the constants needed
to generate the 10 digits (‘0’-‘9’) on a 7-sement LED. You will need to
use the LPM instruction to load the constant from program memory. NOTE:
The ATmega16 uses 16-bit instructions even though it is an 8-bit
processor; therefore, the 256th instruction is actually located
at memory address 512 and 513. Keep this in mind when declaring your
constants in program memory. The code/instructions are two bytes wide (16
bits) but when the program accesses the program memory it can only access
one byte (8 bits) at a time causing the number of addresses to be doubled.
- The decimal point segment on the ones-digit 7-segment LED display
should toggle on every change of counter value. The decimal point on the
tens-digit display may be set arbitrarily. If you’re clever, you can take
advantage of this fact to make your code smaller.
- Counting should happen at 1 Hz.
Question 3: Prove that you are counting at a rate of 1Hz through calculations
by using the number of cycles each instruction takes. In the case of branches
where a variable number of cycles may result, you may assume that the most
frequent branch is taken. Please show your work and list any assumptions you
made.
Deliverables
E-mail a single .zip file containing all required documents to cse466-tas@cs.washington.edu
Demonstrate your
working system to a TA. You can either do this during this lab, or during the
first
1/2 hour of the next lab. Counting should happen at 1 Hz.
Turn in the
answers to the three lab questions and a soft copy of your commented
assembly code.