CSE561 Project #3
out: Friday November 22nd, 2013
due: Tuesday December 10th, 2013 by 5:00pm.
[
summary |
overview |
NAT logic |
turn-in |
grading ]
In this project you will be writing a simple NAT that can handle TCP. It will implement a subset of the functionality specified by RFC5382. You can refer to the RFC for what is expected of NATs.
Before beginning this lab, it is crucial that you:
- Understand how NATs work. Consider looking at the NAT slides from the lecture.
- Understand TCP handshake and teardown packet sequences. Consider working through the TCP state diagram.
We will create a NAT that sits in Mininet between the app servers
and the client. The internal interface of the NAT faces the client,
while the external interfaces are connected to app servers. The app
servers are "outside" the NAT, while the client is "inside."
The topology of NAT is as follows, where the NAT's internal
interface (eth1) faces the client and its external interface (eth2)
has two application servers connected with a switch:
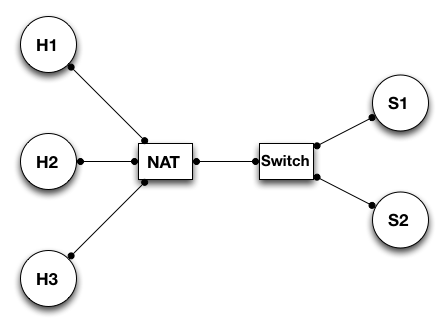
You first need to set up the NAT environment in mininet. Download the tarball in the mininet virtual machine under your home directory:
wget http://courses.cs.washington.edu/courses/csep561/13au/projects/setup.tgz
tar xzvf setup.tgz
To start the mininet, run:
sudo ./run_mininet.py --arp --mac --cip=<Your controller IP addr>
Option description:
- --arp: let the mininet initialize the static ARP entries in each host. For extra credit, you can disable the --arp option and have your NAT handle ARP reply/request.
- --mac: set the MAC address of each host to a simple value, such as 00:00:00:00:00:01. (In this case, the MAC address of each host will be a fixed value, and won't vary at each runtime.)
- --cip: set the remote controller IP address. Default is localhost (127.0.0.1).
Feel free to look inside the script and customize it in whatever way suits your implementation. Some tips/notes about the environment:
- Each server runs a simple HTTP server, serving at port 8000.
- NAT internal and external IP addresses are 10.0.1.1 and 172.64.3.1 respectively. The IP configuration refers to file IP_CONFIG in the tarball.
- The switch in the figure only needs to act as a learning switch. You can directly use your code from Project 1.
- Server1 and server2 operate in folder http_server1 and http_server2 respectively. You can put any files in those folders for clients to download. Clients also operate in different folders.
- If the mininet setting has some problem on your virtual machine, you can try 'sudo mn -c' to clear the previous setting.
These are the major parts of the assignment:
- translating TCP packets
- cleaning up defunct mappings between internal addresses and the external address.
Note that your NAT is not required to handle UDP. It is entirely up to you whether you drop or forward UDP traffic.
A correct implementation should support the following operations
from the emulated client host:
- Clients should be able to download files under directory http_server1 or http_server2 using HTTP from the app servers. Client specifies the server to download files.
- All packets to external hosts (app servers) should appear to come from eth2's address (e.g., 172.64.3.1 above).
When an internal host opens a TCP connection to an external host, your NAT must rewrite the packet so that it appears as if it is coming from the NAT's external address. This requires allocating a globally unique port, under a set of restrictions as detailed below. The requirements for your NAT are a subset of those in specified in RFC5382; in some cases they are more restrictive. Refer to the RFC for details on the terms used. Your NAT has the following requirements:
- Your NAT MUST have an "Endpoint-Independent Mapping" behavior for
TCP. You can refer to the RFC for this requirement, but here is a
quick informal summary of it: The NAT reuses the port binding for
subsequent sessions initiated from the same internal IP address and
port to any external IP address and port.
- Your NAT MUST support all valid sequences of TCP packets (defined
in RFC0793) for
connections initiated both internally as well as externally when the
connection is permitted by the NAT. In particular, in addition to
handling the TCP 3-way handshake mode of connection initiation, A NAT
MUST handle the
TCP simultaneous-open
mode of connection initiation.
- Your NAT MUST have an "Endpoint-Independent Filtering" behavior
for TCP. Again refer to the RFC for this requirement.
- Your NAT MUST NOT have a "Port assignment" behavior of "Port
overloading" for TCP.
- When assigning a port to a mapping, you are free to choose a port
any way you choose. The only requirement is that you do not use the
well-known ports (0-1023).
- Be sure that your NAT's mapping data structure uses locks if
there is going to be concurrency in the controller, otherwise nasty
concurrency bugs will be sure to crop up.
As noted above, mappings should be Endpoint Independent. Once a
mapping is made between an internal host's (ip, port) pair to an
external port in the NAT, any traffic from that host's (ip, port)
directed to any external host, and any traffic from any external host
to the mapped external port will be rewritten and forwarded
accordingly.
Cleaning up defunct mappings
Your NAT must clean up defunct mappings. Your NAT must periodically
timeout idle TCP connections. Once all connections using a particular
mapping are closed or timed out, the mapping should be cleared. Once
cleared, a mapping can be reused in new connections.
The periodic function that handles timeouts should use the following timeout
intervals:
- TCP Established Idle Timeout in seconds (default to 7440)
- TCP Transitory Idle Timeout in seconds (default to 300)
TCP Established Idle Timeout applies to TCP connections in the
established (data transfer) state. TCP Transitory Idle Timeout applies
to connections in other states (e.g. LISTEN). Refer to the TCP state
diagram.
Tracking Connections
You do not need to keep lots of state per connection. For example, there is no need to track seqnos or window values or ensure TCP packets are in proper order to the end hosts. Keep only the information that is useful to the NAT for establishing or clearing mappings.
Each time the controller receives a new TCP flow, it should install a new rule to the NAT which modifies a few fields in data link, network and transport headers. The OpenFlow switch will automatically update the IP and TCP checksums.
Optional
A few optional functions of the NAT for extra credit:
- Handle ARP requests/replies.
- Implement Firewall in the NAT, such as clients are allowed to access http server1 but not http server2
- Implement DHCP server in the NAT
Tips for Floodlight Users
A few hints for flooding users:
- The following actions will allow you to change fields in the packets:
OFActionDataLayerDestination
-- changes the destination
MAC
OFActionDataLayerSource
-- changes the source
MAC
OFActionNetworkLayerDestination
-- changes the destination
IP
OFActionNetworkLayerSource
-- changes the source
IP
Please turn in a tarball or a zip file at https://catalyst.uw.edu/collectit/dropbox/summary/arvindk/29361.
The archive should have following files:
- your source code with comments;
- a readme file including your name, email address, and the platform you use;
- transcript of the commands that you executed to test the code and the output from the commands;
- a report that contains what you did, results and discussion, and experience you learnt from.
We will be basing your grade on several elements:
- Whether your code works! It should be correct, compile
without warnings, and not leak memory, processes, descriptors, etc.
- How well structured your code is: you should have clean
module interfaces, a nice decomposition, good comments, and so on.