CSE 557: Computer Graphics
Spring Quarter 1999
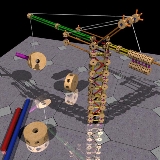 |
Project 2: Ray
Date Assigned: 20 April 1999
Send Email: 28 April 1999
Date Due: 4 May 1999
|
Project Description
You will build a program called Ray
that will generate
ray-traced images of complex scenes. You will trace rays
recursively using the Whitted illumination model.
Getting Started
To install the starting point source code unzip the file,
here. The sample solution can load
scenes and save images. It generates extremely simple "ray-traced"
images, though. They are white if the ray from the camera
hit anything, black otherwise.
Required Functionality
We'll describe these requirements in more detail afterwards:
-
Implement Whitted's illumination model (FvDFH, eq. 16.55), which
includes ambient, diffuse, and specular terms for each light source as
well as reflection and refraction terms. You only need to handle
directional and point light sources, i.e. no area lights, but you
should be able to handle multiple lights.
-
Implement Phong interpolation of normals.
-
Implement anti-aliasing. Regular super-sampling is acceptable, more
advanced anti-aliasing will be considered an extension.
-
Implement data structures that speed the intersection query in large
scenes. On the 28th of April please mail your TA a brief (but
detailed) description of what optimizations you plan to implement.
Notes on Whitted's illumination model
The first three terms in Whitted's model will require you to trace
rays towards each light, and the last two will require you to
recursively trace reflected and refracted rays. When tracing rays
toward lights, you should look for intersections with objects, thereby
rendering shadows. If you intersect a semi-transparent object, you
should attenuate the light, thereby rendering partial shadows, but you
may ignore refraction as explained in class. See the page on creating scenes for some information about how
to map the properties of our material class to the elements of Whitted's
equation.
The skeleton code doesn't implement Phong interpolation of normals.
You need add code for this (only for meshes with per-vertex
normals.)
Anti-aliasing
Once you've implemented the shading model and can generate images, you
will notice that the images you generated are filled with "jaggies".
You must implement an anti-aliasing technique to smooth these rough
edges. In particular, you are required to perform super-sampling and
averaging down. You should provide a slider to control the number of
samples per pixel (1 - 16 samples). You need only implement a box
filter for the averaging down step. More sophisticated anti-aliasing
methods are left as bells and whistles below.
Acclerated ray-surface intersection
The goal of this portion of the assignment is to speed up the
ray-surface intersection module in your ray tracer. In particular, we
want you to improve the running time of the program when ray tracing
complex scenes containing large numbers of triangles. There are two
basic approaches to doing this:
-
Specialize and optimize the ray-triangle intersection test to run as
fast as possible.
-
Add data structures that speed the intersection query when there are
many triangles.
Most of your effort should be spent on approach 2, i.e. reducing the
number of ray-triangle intersections. You are free to experiment with
any of the acceleration schemes described in Chapter 6, ``A Survey of
Ray Tracing Acceleration Techniques,'' of Glassner. Of course, you
are also free to invent new acceleration methods.
Make sure that you design your acceleration module so that it is able
to handle the current set of geometric primitives - that is, triangles
spheres, squares, and cones.
The samples include three complex test
scenes: test1, test2, and test3. You will notice that among these
test1 has per-vertex normals and materials, and test2 has per-vertex
materials but not normals. Per-vertex normals and materials imply
interpolation of these quantities at the current ray-triangle
intersection point (using barycentric coordinates).
Acceleration grading criteria
The new test scenes each contain up to thousands of triangles. A
portion of your grade for this assignment will be based on the speed
of your ray tracer running on these scenes. The faster you can render
a picture, the higher your grade. The remainder of your grade on this
portion of the project will be based on the cleverness of your
acceleration scheme.
All images should be traced at the default size of the sample
solution, with one ray traced per pixel, and rays should be traced
with 5 levels of intersections, i.e. each ray should bounce 5 times.
If during these bounces you strike surfaces with a zero specular
reflectance, stop there. At each bounce, rays should be traced to all
light sources, including shadow testing. None of the test scenes will
include transparent surfaces.
You are welcome to precompute scene-specific (but not
viewpoint-specific) acceleration data structures and make other
time-memory tradeoffs, but your precomputation time and memory use
should be reasonable. Don't try to customize your ray tracer for the
test scenes; we may use other scenes during grading. If you have any
questions about what constitutes a fair acceleration technique, ask
us. Coding your inner loops in machine language is unfair. Using
multiple processors is unfair. Compiling with optimization enabled is
fair. In general, don't go overboard tuning aspects of your system
that aren't related to tracing rays.
Measuring memory use
You should also keep track of the approximate amount of memory you use
rendering each scene. To record memory use it is suffient to look at
what the Windows task manager says. Your ray tracer's memory use will
probably be fairly constant during a run.
Bells and Whistles
This assignment is large, and, furthermore, the optimization element
is completely open-ended, so you can profitably work on that until the
project is due. Therefore we don't neccessarily expect a bunch of
bells and whistles. We can't stop you, though. Here are some
interesting extensions to the anti-aliasing portion of this project.
Approved Bells and Whistles
Implement two more averaging down filters, e.g. Gaussian and cubic.
Implement adaptive supersampling as described in Glassner, Chapter 1,
Section 4.5 and Figure 19.
Implement stochastic (jittered) supersampling. See Glassner, Chapter
5, Section 4.1 - 4.2 and the first 4 pages of Section 7.
Implement adaptive, stochastic supersampling.