CSE466 Lab 6: “Introduction to TinyOS”
Objectives
The goal of this lab is to use tutorials to introduce the structure of TinyOS.
In this lab you will learn the following:
-
The major concepts used in TinyOS programming
-
Basic mote communication
-
How to use motes to obtain sensor readings
-
Become familar with the the TinyOS tools
Important Warnings
-
DO NOT power the mote from the AC adapter and batteries at the same
time. ALWAYS
turn off the motes battery power before placing it in the programming
board. Only one mote should be plugged into the
MIB510 programmer.
-
Be careful when placing your mica2dot into your MIB510
programmer and sound board- do NOT bend the pins.
-
Some instructors of CSE378 use software that changes
your windows profile to point to a network copy of cygwin, not the
local copy of cygwin. To complete the labs in CSE466 you will need
to make sure to use the local copy of cygwin. If for some reason TinyOS
is not working please inform a TA or lab staff member and we will
attempt to undo the profile change. In a few extreme cases,
students' profiles have had to be wiped clean by support staff.
-
TUTORIALS ARE VERY OUT OF DATE, THEY CAN ONLY BE MODERATELY TRUSTED TO BE
ACCURATE!!!!!
Important Notes
-
The type of motes you will be using in lab are the "mica2" and
"mica2dot". Make sure to compile your program for the correct piece of
hardware. (e.g. 'make mica2' NOT 'make mica') For mote programming you will be
using the MIB510 serial programming board.
-
Use the number on your sound board's colored sticker as your group number in
hex. Please make sure to type in your group number as a hex number in the
MakeRules file in the apps directory
.
-
A few of these tutorials may be out of date so please ask questions if you are
confused.
-
The mica2 and mica2dot are built around the
ATmega128 microcontroller not the ATmega16.
-
Use the default radio fequency and programming board. If you don't specify the
radio frequency or programming board it will automatically choose a correct
value.
-
Make sure your MOTECOM variable is properly set when trying to communicate to
the devices over serial. The mica2 communicate at 57600 baud while the
mica2dots communicates at 19200.
-
Only the mica2 motes (not
the mica2dots) have three LEDs on board, therefore the modules that set these
LEDs will only be completely functional (meaning will light all three
LEDs) on the mica2
-
The mica2dot has a light-sensor (photo-resistor) on it, but the mica2s do
not.
-
Some of the tutorials make reference to a "sensor board". This is a modular
package that can be connected to the motes. We don't have enough available for
every group (so you can skip these sections of the tutorials), but if you are
interested in playing around you can talk to the TAs.
Reading
-
"System Architecture Directions for Networked Sensors" in coursepak
-
"A Network-Centric Approach to Embedded Software for Tiny Devices" in coursepak
-
"The nesC Language: A Holistic Approach to Networked Embedded Systems" in
coursepak
-
TinyOS Tutorials
- especially the ones you will be completing
Resources
TinyOS Tutorials
ATmega128 data sheet
avr-gcc manual
Application
notes section for the AVR 8-bit RISC family
Suggested Steps
PART 1: Tutorials
-
Unzip
apps.zip to your Z drive,
creating Z:\apps\.
-
CAREFULLY plug your mica2dot mote into your
sound board. Then plug the sound board into the MIB510 programmer and connect
the power and serial cables to the programmer. Battery power must be OFF!
-
Launch Cygwin and use the alias "cdapps" to navigate to the
apps folder on your z: drive. Type 'alias' in cygwin to obtain a list of
available aliases. The aliases are provided to make navigation around TinyOS
easier.
-
Question 1: What is the flow of
control through the modules of the Blink application? Start with main.nc
and list the files in the order of their execution (this should include only
xxx.nc and xxxM.nc files, make sure to include Leds.nc and Timer.nc) for the
first 2 seconds of operation.
-
Make sure BOTH members of your group attempt to compile
and install an application onto a mote using his/her login to
clear up any Windows profile issues. Make sure that you have set up your group
number (in hex) as per the Important notes section above on BOTH member's
profiles.
-
Using the photoresistor located on the sound board, complete the tutorial
called
Lesson
2: Event-Driven Sensor Acquisition. Refer to the sound board datasheet
for the pin mapping. You do NOT need to complete the exercise from this lesson.
NOTE: Lesson 2 states "Notice the use of the function
rcombine()
in the implementation of
StdControl.init()." However
the recently updated version of SenseM does NOT use rcombine( ). It
is confusing that the Lesson refers to rcombine() even though
the function is no longer demonstrated in SenseM. The function
rcombine()
is a special nesC combining function which returns the logical-and of two
commands who result type is result_t.
NOTE: you don't have a
sensor board for the mica2's, but you do have a light sensor on the mica2dot -
see Lesson 4 for ideas as to how to take info sensed on the mica2dot, and
display it on the mica2 LEDs.
Question 2: What are the events generated during the
execution of the Sense application? For each basic event coming from the
hardware, describe the fountain of execution that is triggered (list each of
the events and commands that are issued from each hardware event and the
interfaces they move across).
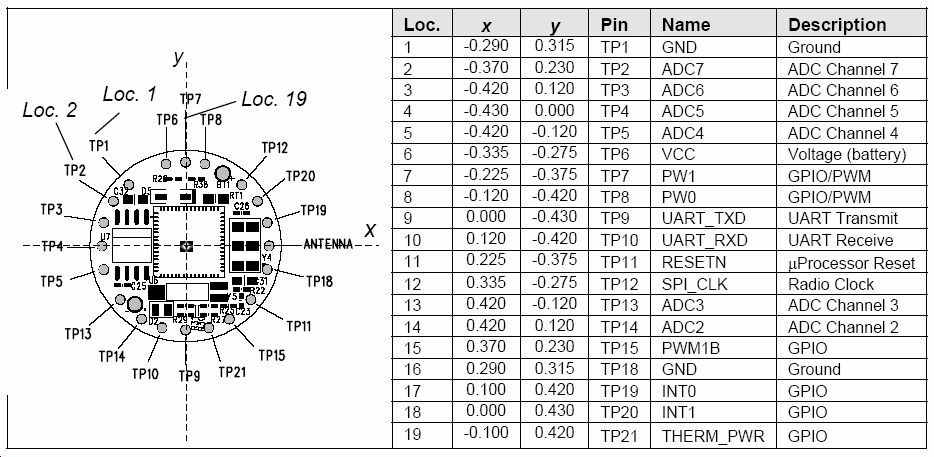
-
Complete the tutorial called
Lesson 4: Component Composition and Radio Communication including the
exercises. You will need to hand in your solution to the exercises for Lesson 4.
-
Complete the tutorial called
Lesson 3: Introducing Tasks for Application Data Processing including
the exercises. You will need to hand in your solution to the exercises for
Lesson 3.
-
Complete the tutorial called
Lesson 6: Displaying Data on PC
including the exercises. The "extra credit" section is optional and will not be
worth any extra credit in this class. You will need to team up with another
group to get a third mote to attempt the "extra credit".
-
You and your partner might want to complete the first part of Lesson
5: Simulating TinyOS Applications in TOSSIM to learn how to debug
with TOSSIM. TinyViz is not working as described in this tutorial and problems
have been reported on the help list. Therefore we will not be using it in this
class to avoid unnessary complications. Feel free to attempt to
experiment with TinyViz but the course staff will NOT support
TinyViz.
PART 2:
In Part 2 of the lab you will control the tri-color led
over a wireless network. The motes will act as a wireless communication conduit
and you will control the color of the LED with the accelerometer on your
breadboard. You will need to add the MoteConn class to SPI/USB control to send
mote packets to the SerialForwarder provided in TinyOS.
Goal: Use the accelerometer, potentiometer, and button on
the ATMega16 breadboard to control the TriColor LED on the SoundBoard_1 similar
to the light control in Lab 3. The interaction should be the same as in Lab 3,
which means the LED's color should only change when the button is pressed.
-
Create a new folder in your apps directory for your solution to part 2. In this
folder you will want to create your application files and a dimmer module (HPLTriLEDM.nc)
to control the PWM. Your application should consist of a configuration file (LightNode.nc)
and a module (LightNodeM.nc)
that receives radio packets, parses the light value from the radio packet, and
sends the light value to
HPLTriLEDM.nc to
output the correct brightness. You will also need to create an interface(TriColorLED.nc)
to issue commands to your dimmer module. NOTE: Leave all files in your
application directory as the compiler will look in the local directory first
before moving on to tos/system, tos/interfaces, tos/platform, and tos/libs.
This will avoid problems with files being deleted when you log off.
-
Implement your LED dimmer module (HPLTriLEDM.nc)
in TinyOS using Timer 1 of the ATmega128. Refer to the
ATmega128 microcontroller datasheet and the
SoundBoard_1
datasheet to determine how to control the tri-color LED. Your LightNode
application should update the tri-color LED based on the last RGB value
received from the radio (use AM message type #33). You should send update
packets approximately every 250-500ms. NOTE: We suggest using TOSH_INTERRUPT
instead of TOSH_SIGNAL to make sure your program doesn't interfere with
anything important in TinyOS.
-
Modify your SPI/USB code to use the MoteConn class to transmit the desired
light level in a TinyOS radio packet with AM message type #33. The light level
being sent over the radio should be controlled by your accelerometer. MoteConn
is a simple class that opens up a connection to the TinyOS SerialForwarder and
allows you to send packets. MoteConn needs to link to ws2_32.lib. Instructions
on how to link MoteConn to ws2_32.lib in MSVC are provided in MoteConn.h.
Here are the MoteConn Files
SOMETHING TO CONSIDER: We could have easily created a small
handheld
USB
device using the same
USB/
SPI
chip, ATmega16, and accelerometer. These chips come in smaller packages that
could be soldered to a small printed circuit board to create a small device.
This device could be put into some sort of packaging and receive all necessary
power from the USB port creating a nicely packaged controller.
Deliverables
Turn in your code from the exercises in answers to the two questions
above. All your files should contain comments that include: both
partners' full name, login, student number, the lab number (e.g. “Lab 6, Lesson
1”), as well as important words about how your code works.
-
Turn in your solutions for exercises in Lessons 1, 3, and 4.
-
Turn in your diagrams for
questions 1 and 2.
-
Demonstrate Part 2 working to a TA and
hand-in all the modified TinyOS files. (i.e. LightNode.nc,
LightNodeM.nc, HPLTriLEDM.nc, TriColorLED.nc)