name: inverse layout: true class: center, middle, inverse --- # Interaction Programming in Web Development Lauren Bricker CSE 340 Winter 23 --- layout: false [//]: # (Outline Slide) # Today's Agenda - Administrivia - Course evals released this weekend ([lecture](https://uw.iasystem.org/survey/258542), [section A](https://uw.iasystem.org/survey/269383), [section B](https://uw.iasystem.org/survey/269388)). Please fill these out. - Project Reminders - Final project code due Fri 11-Mar 10pm - SUS testing due Sun 12-Mar - NO Resubmissions on code/testing/video/report. - Section tomorrow! - Learnining Goals - Explore Interaction Programming in Web Development - Crash course on Web Programming - Create Spot The Heron: web version --- # Website SUS score SUS score from our website: 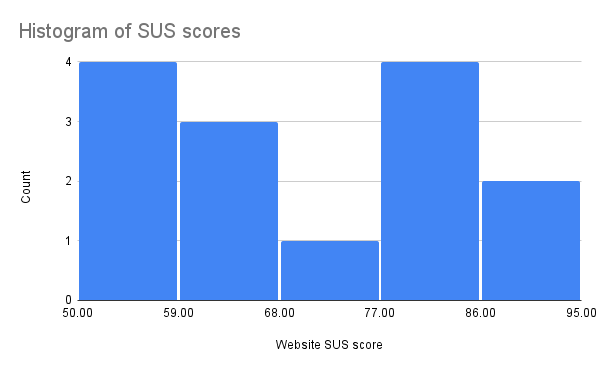 --- # Spot The Heron: Android version Code for the [Spot The Heron](https://gitlab.cs.washington.edu/cse340/exercises/cse340-spot-the-heron) case study. - Layout is achieved with high level tool which generated XML - Images are stored in the "drawable" directory - When app starts the `AppCompatActivity#onCreate(Bundle savedInstanceState)` is called. This method: - Sets the app to use the XML defined layout - Initializes some variables - Registers callbacks for the three buttons - previous, slideshow, and next - Uses the `loadImage(int image)` to load the first image (0th index) in the list of file names --- # Spot The Heron: Android version Code for the [Spot The Heron](https://gitlab.cs.washington.edu/cse340/exercises/cse340-spot-the-heron) case study. - The `loadImage(int image)` method - Gets a handle on the image view interactor using an id `findViewById(R.id.heronView)` - Sets the image view's source to be the new image. - Sets the content description of the image view to be in line with the new image. - If the previous or next button is pressed, the `switchImage(boolean forward)` method is called. - If the slideshow button is pressed, a timer would start that changes the image every 1500 ms --- # Spot The Heron Comparison |Android Version | Web Version | | :--: | :--: | |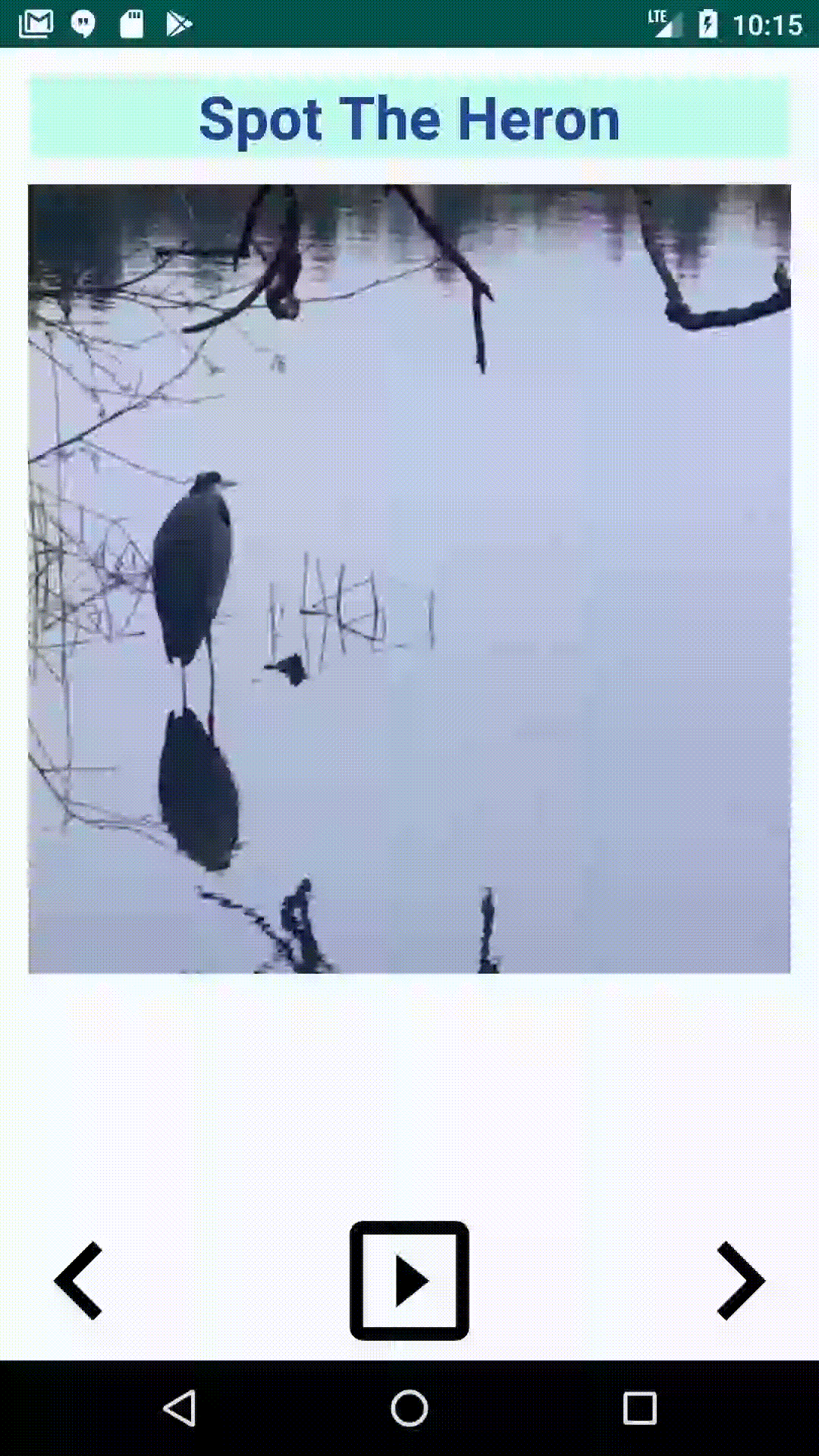|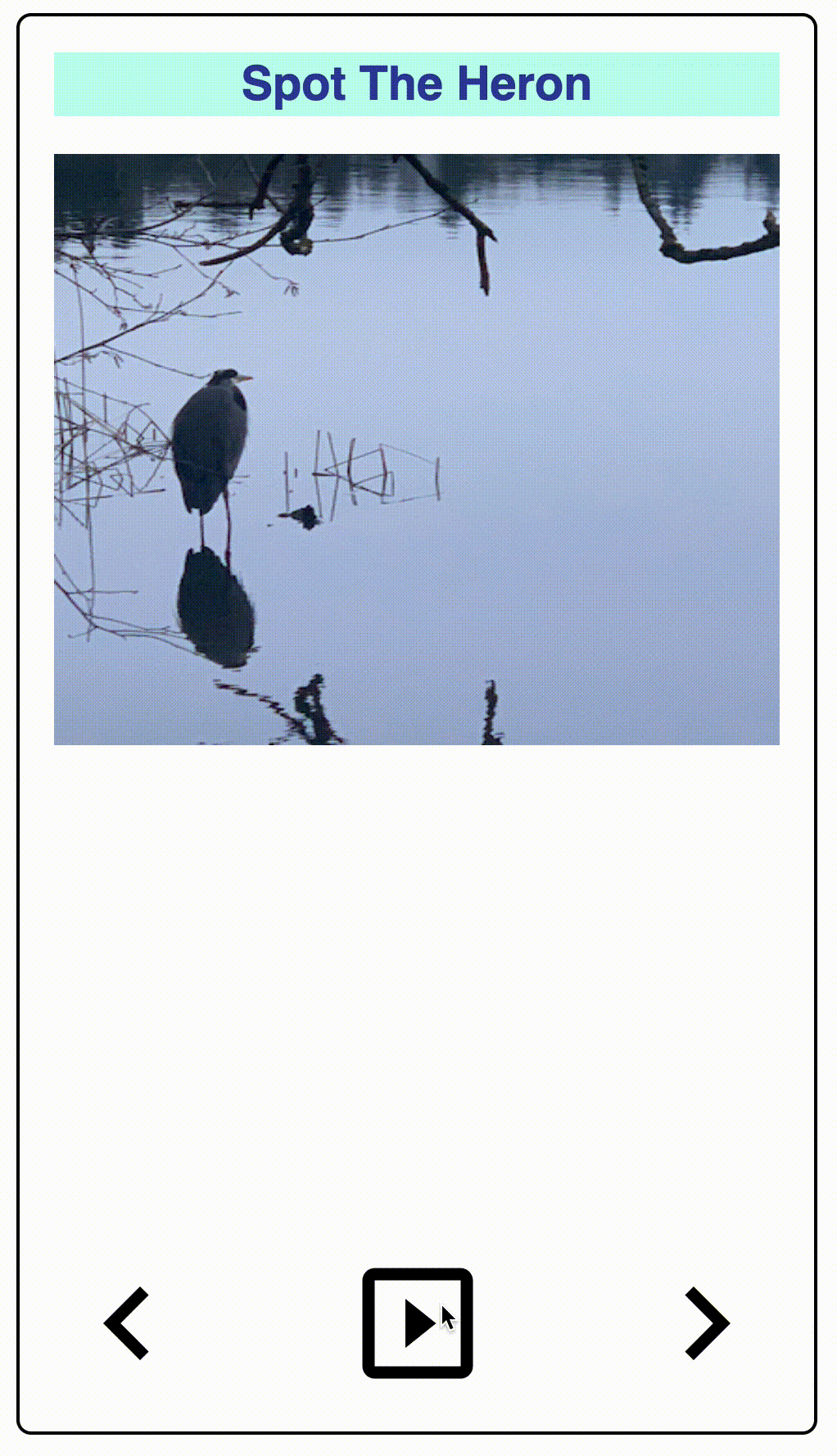| --- # Interlude: What is a web page really? | Content | Structure | Style | Behavior | | :--: | :--: | :--: | :--: | |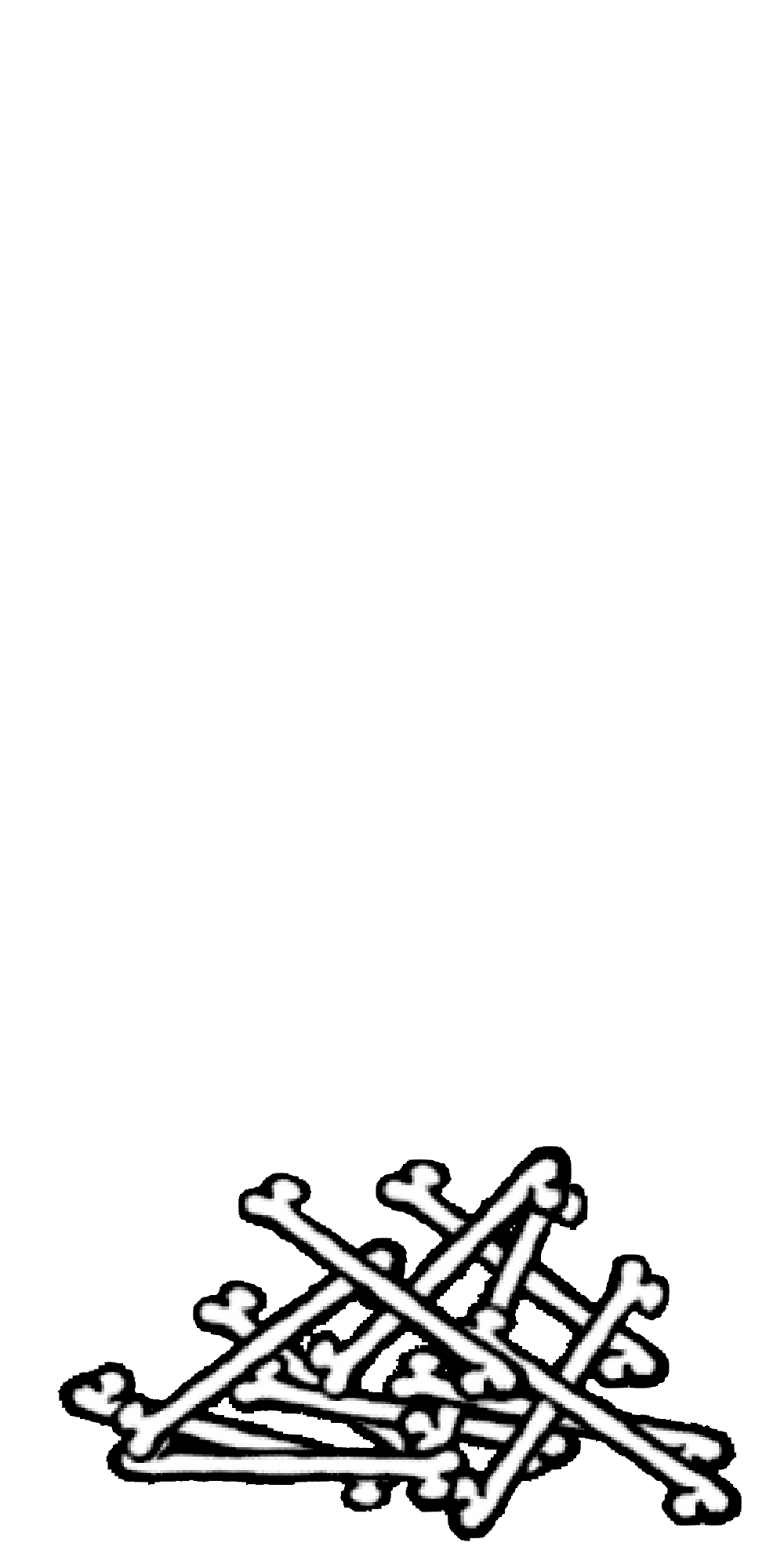| | Words and Images | HTML | CSS | JavaScript | --- # Interlude: What is a web page really? | Content | Structure | Style | Behavior | | :--: | :--: | :--: | :--: | |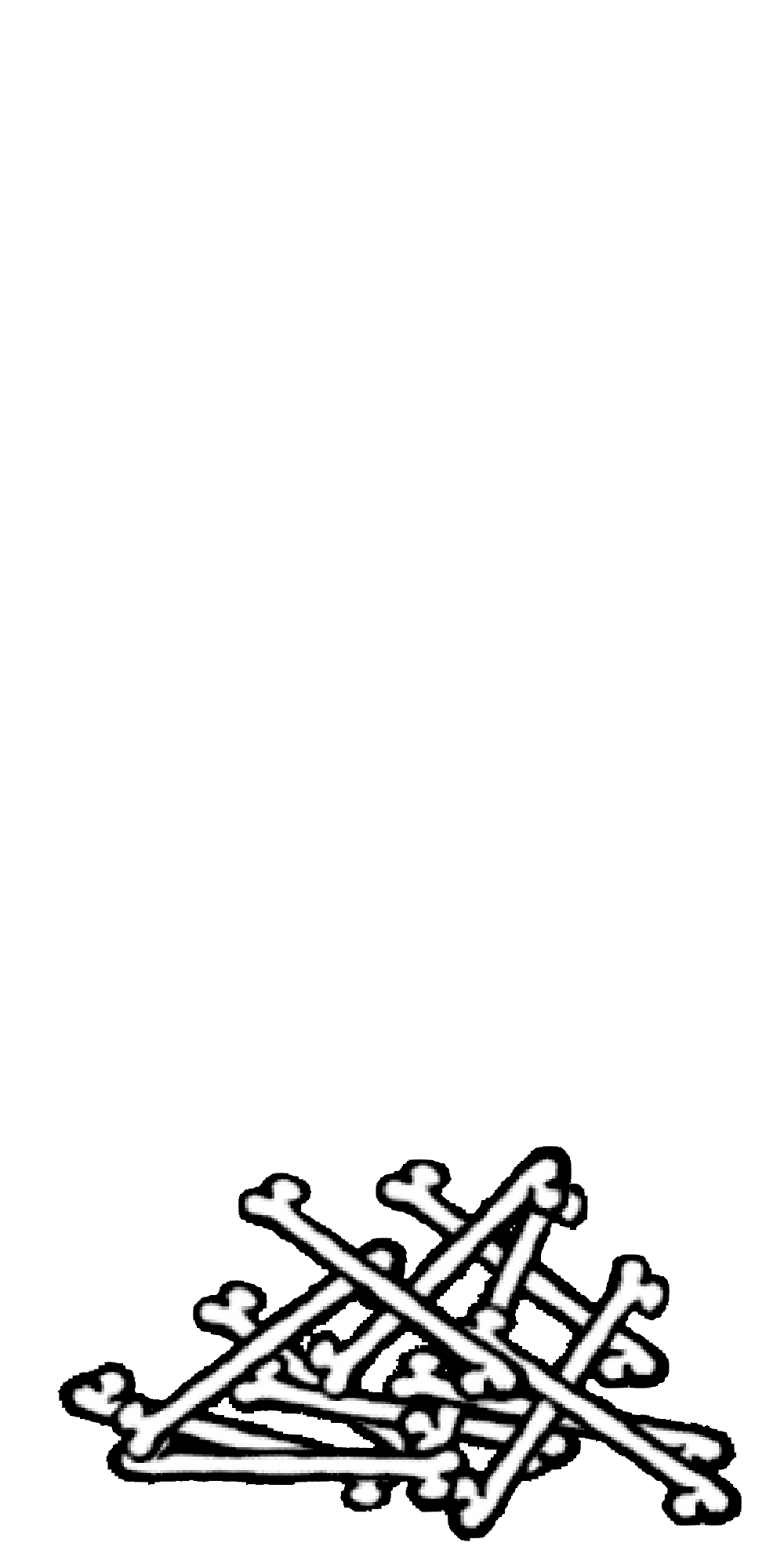|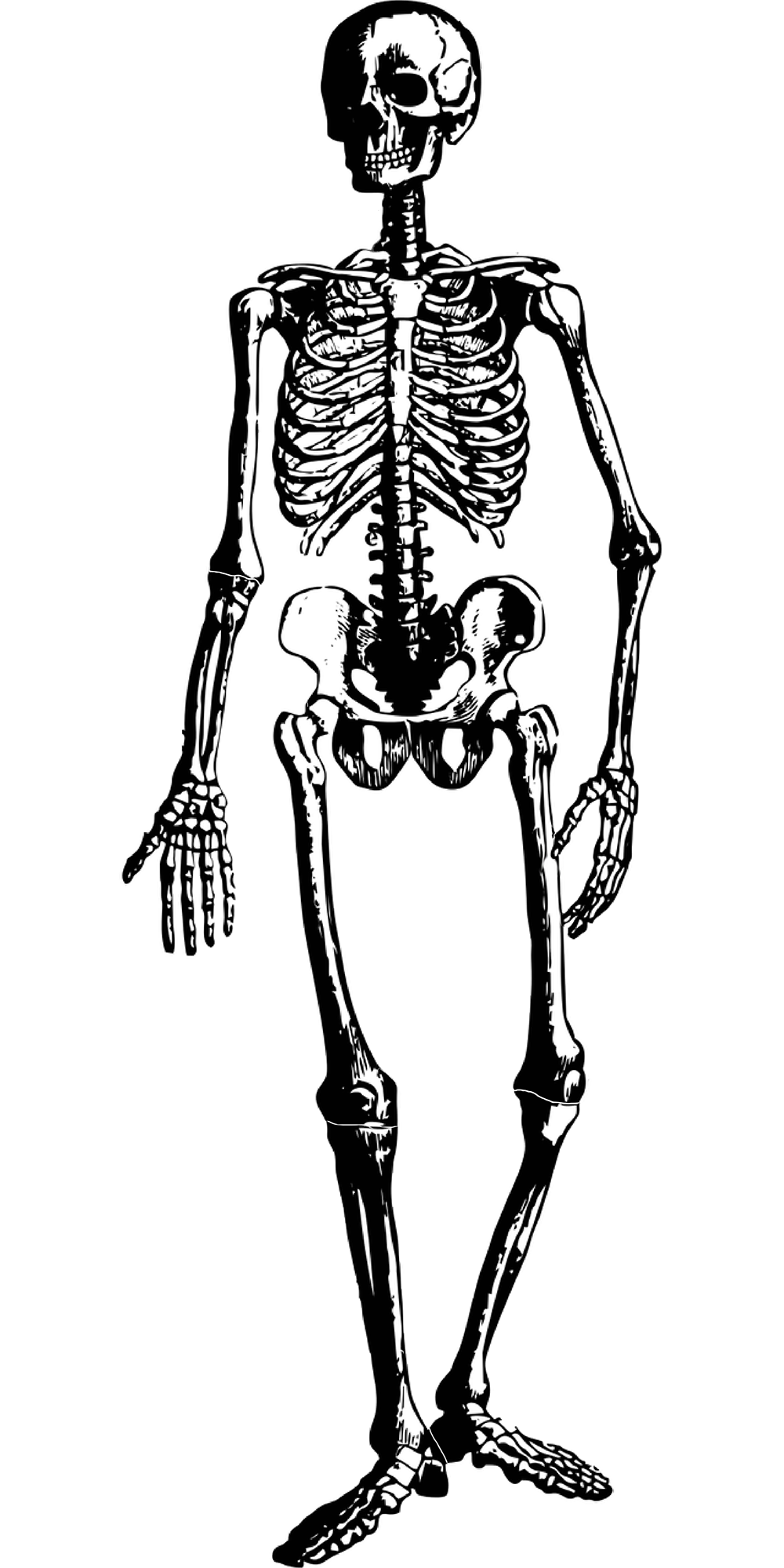| | Words and Images | HTML | CSS | JavaScript | --- # Interlude: What is a web page really? | Content | Structure | Style | Behavior | | :--: | :--: | :--: | :--: | |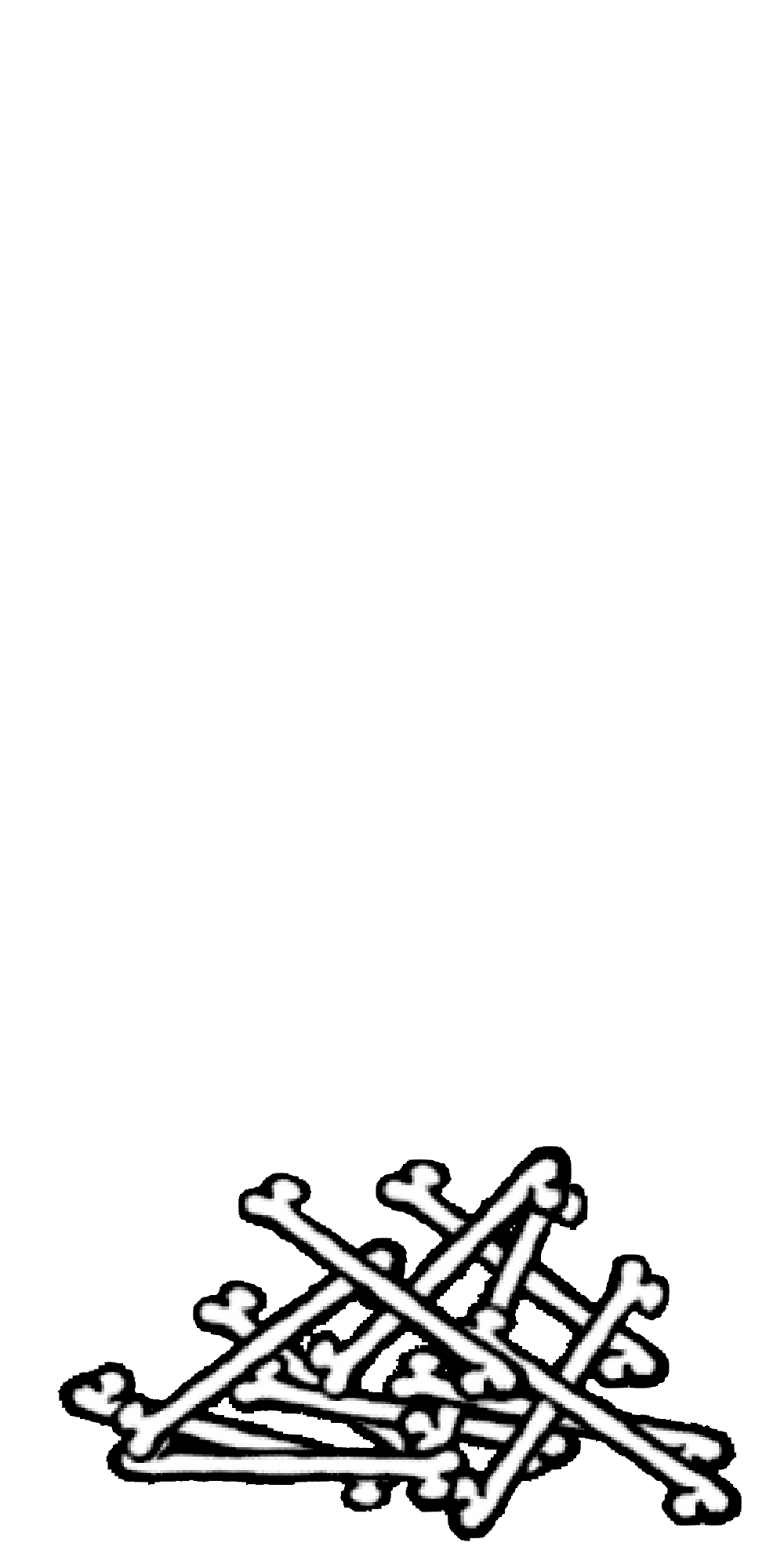|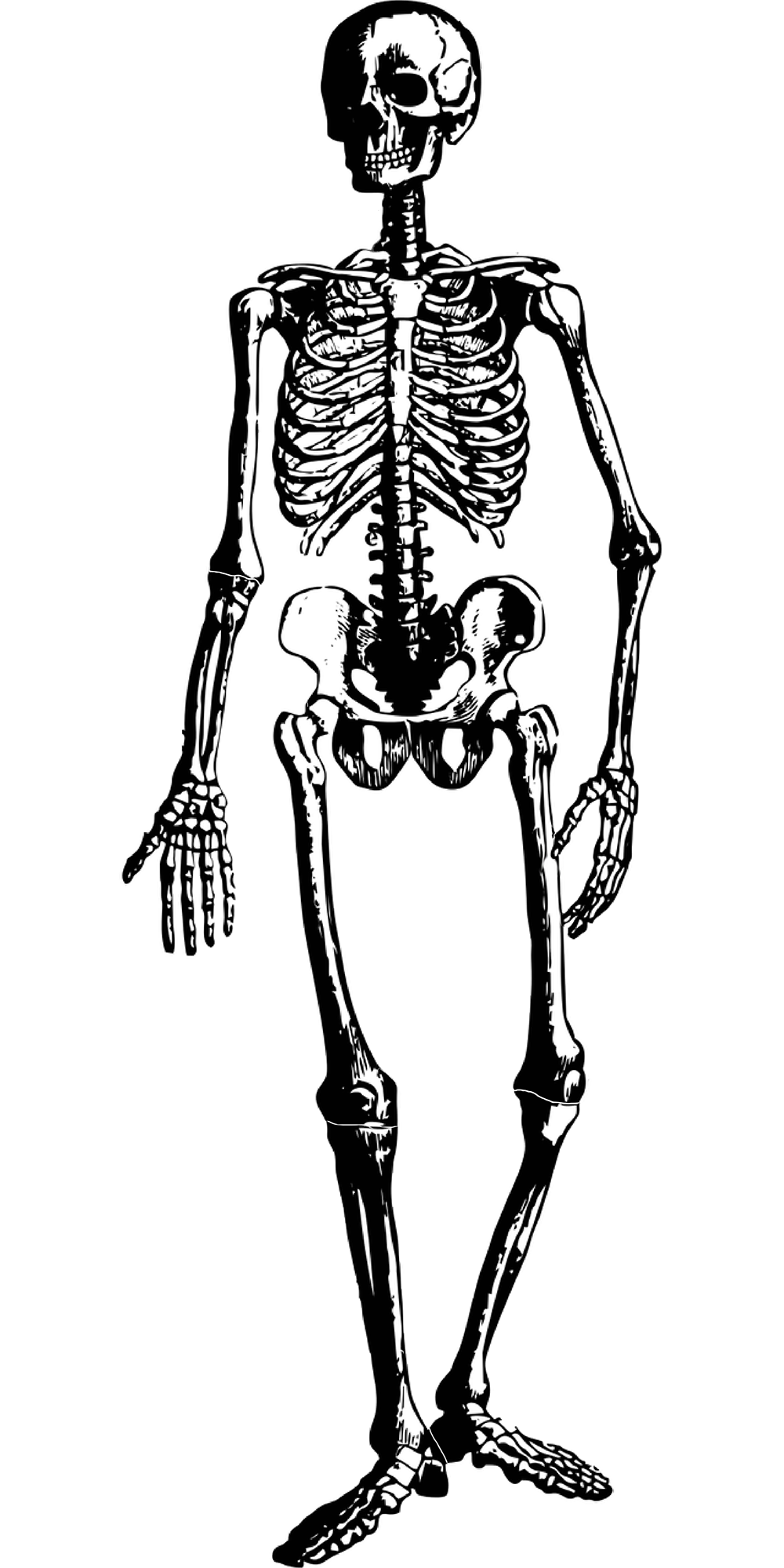|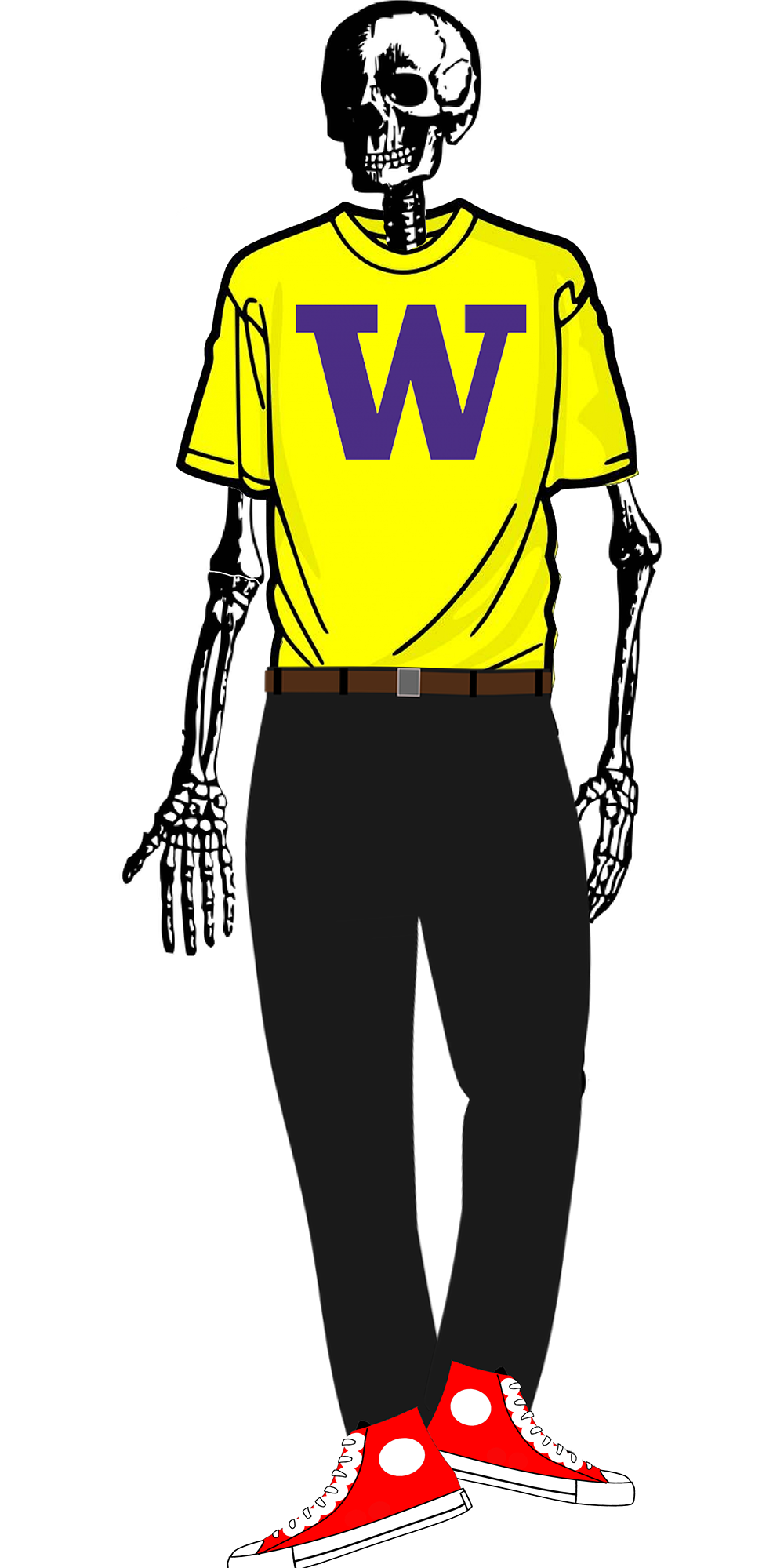| | Words and Images | HTML | CSS | JavaScript | --- # Interlude: What is a web page really? | Content | Structure | Style | Behavior | | :--: | :--: | :--: | :--: | |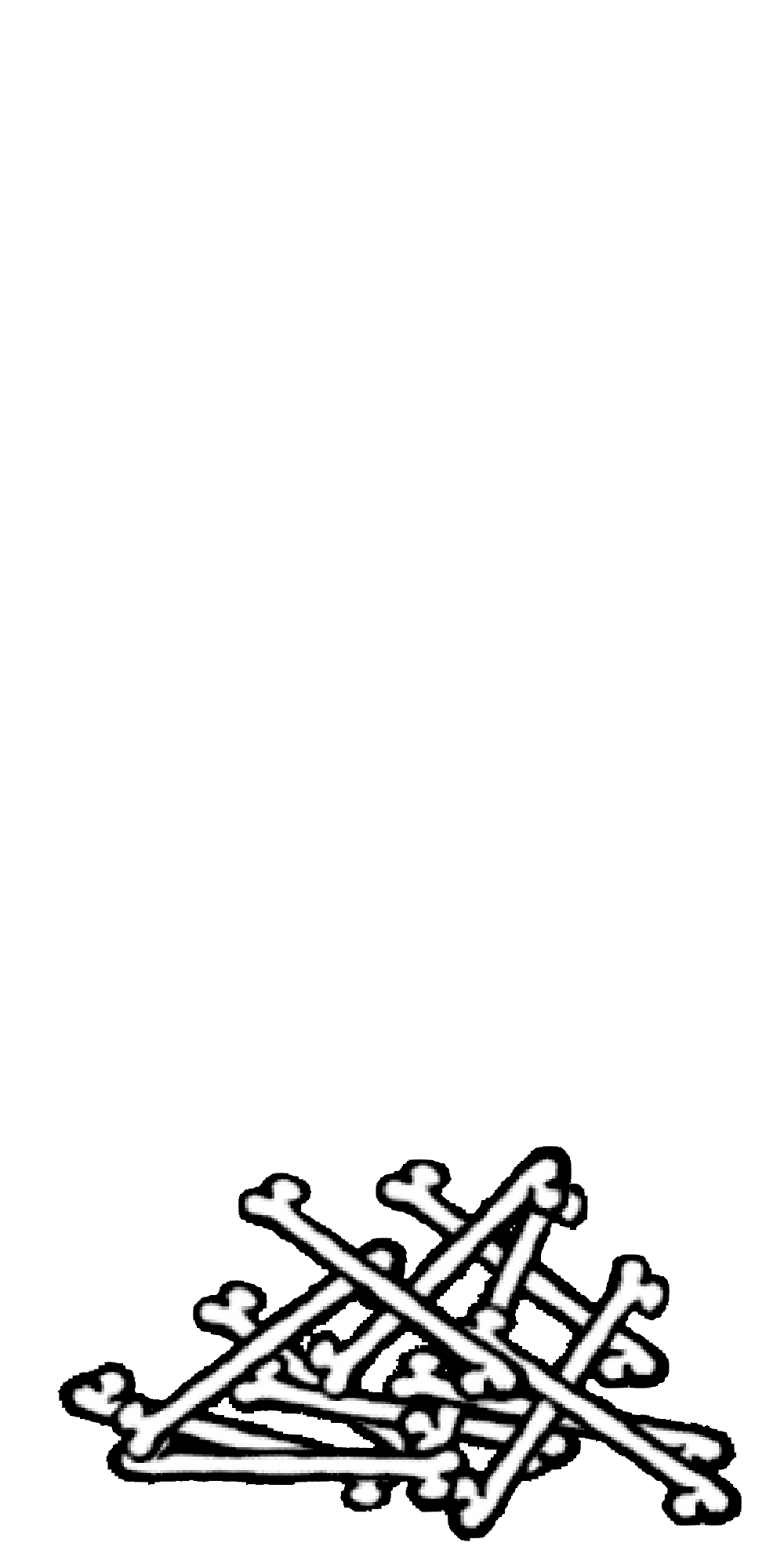|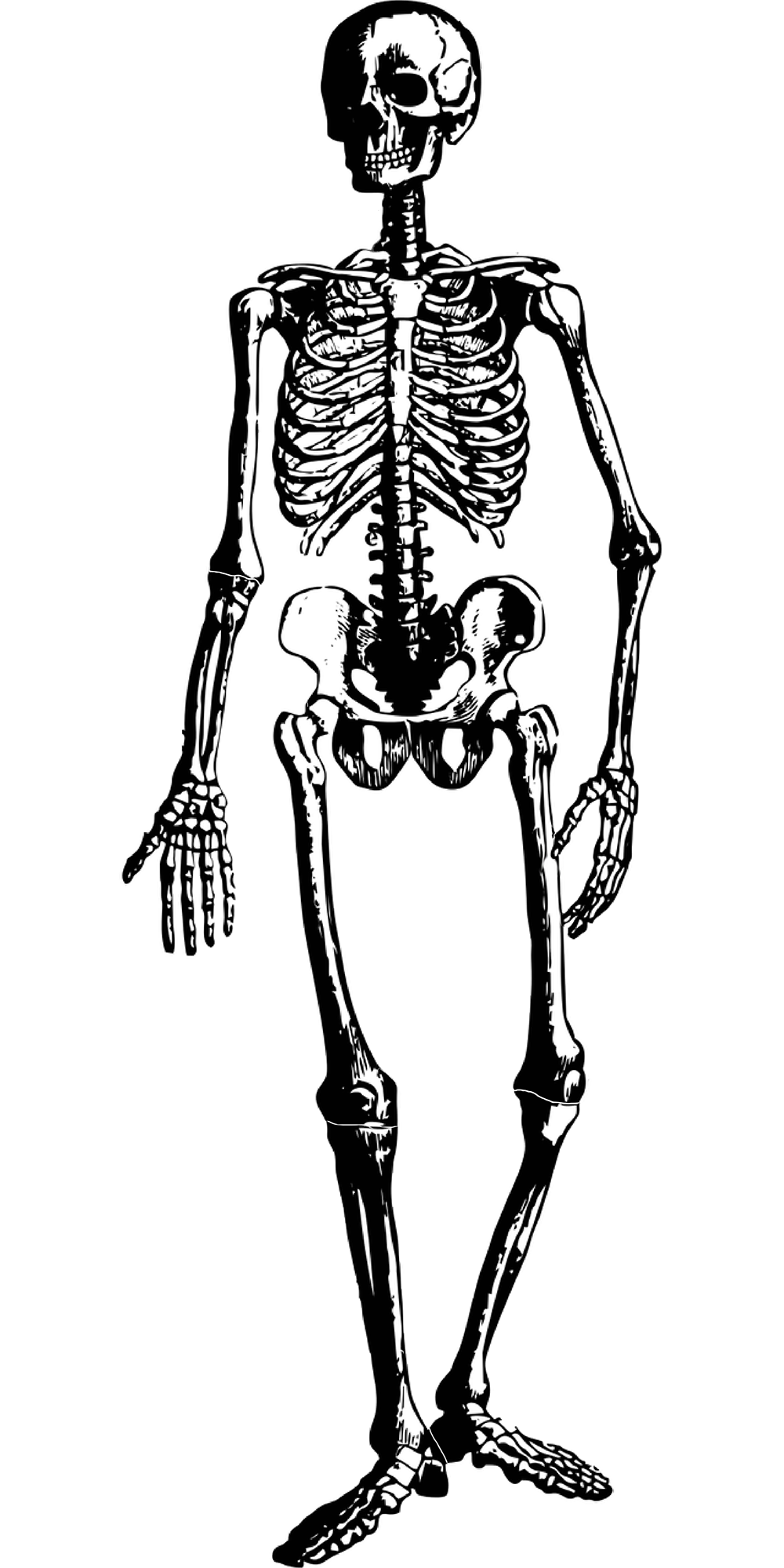|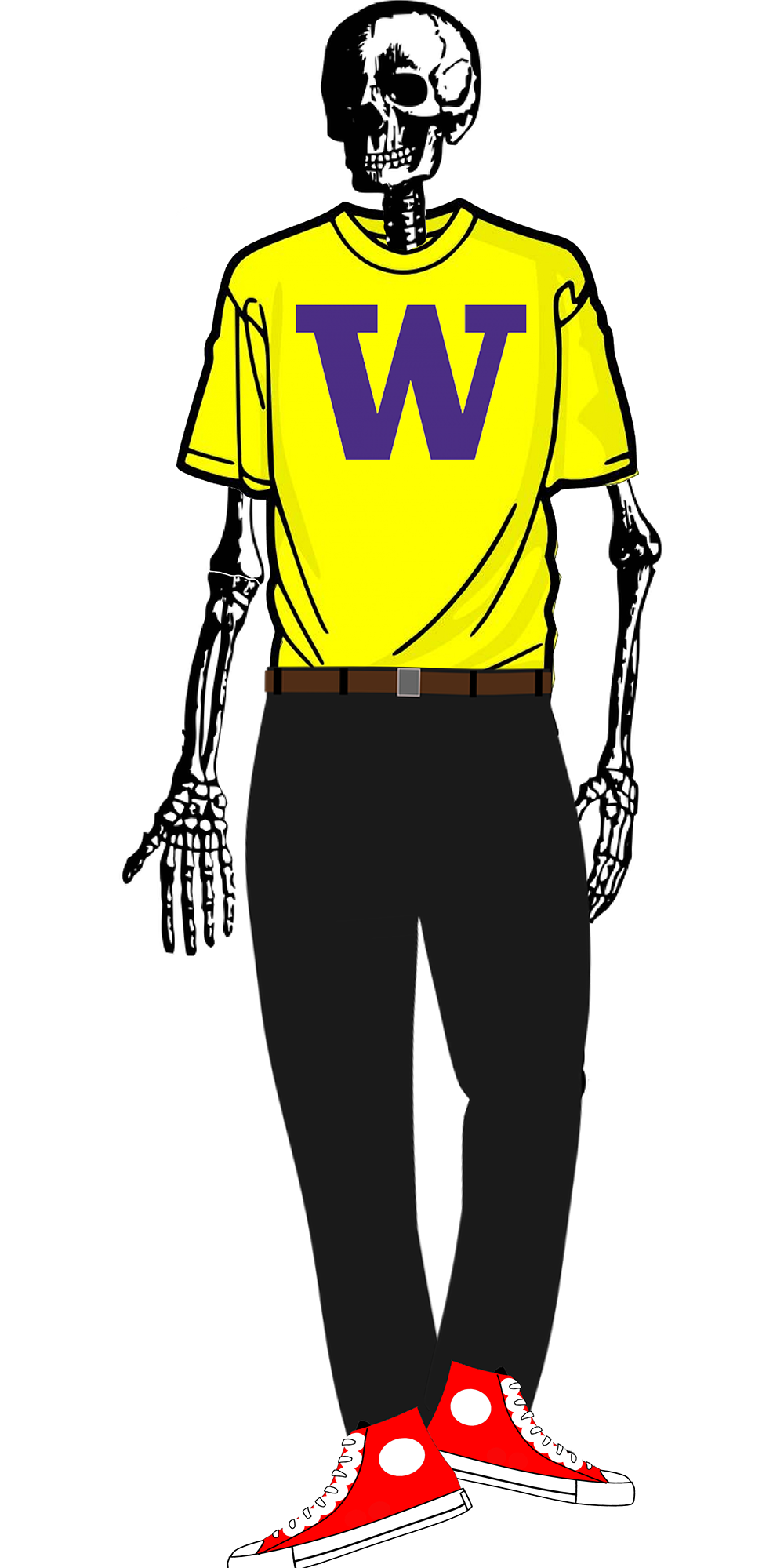|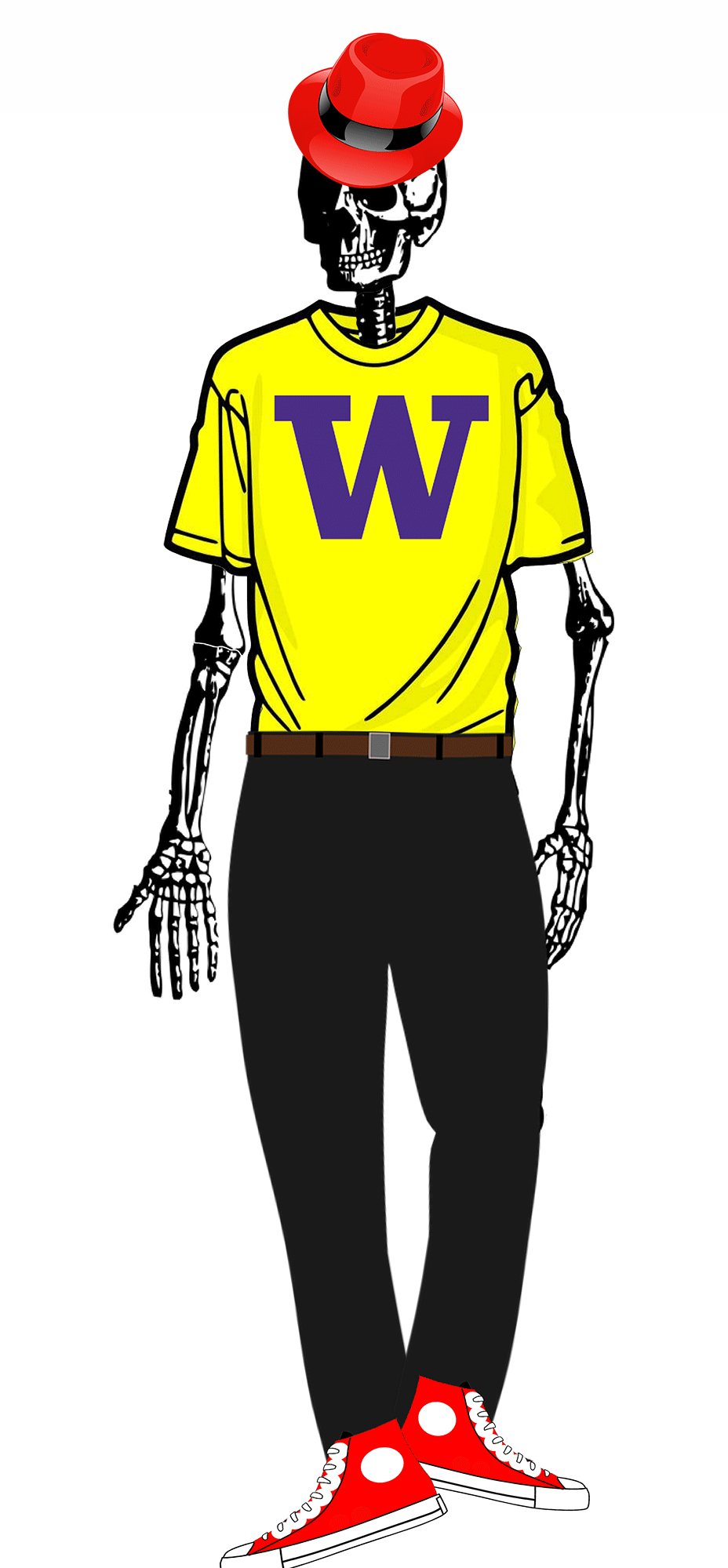| | Words and Images | HTML | CSS | JavaScript | --- # Vocabulary - **Internet** - Hardware infrastructure of connected computers and wires - **World Wide Web (WWW) (http)** - The information on the Internet made up of files and folders stored on computers - **Hyper Text Markup Language (HTML), Cascading Style Sheets (CSS), & JavaScript (JS)** - The languages that we use to program our webpages - **Web Browser** An application (Chrome, Netscape, Safari) that interprets our web languages and renders it visually --- # Languages Java != JavaScript | Java | JavaScript | HTML/CSS | | -- | -- | -- | | | Compiled | Interpreted | Rendered Data | | Type safe | Not type safe | N/A | --- # Lifecycle of a browser* loading a page .left-column40[ 1. Fetch the page 2. Parse the page 3. Build up an internal representation of the web page 4. Display the page ] .right-column60[ 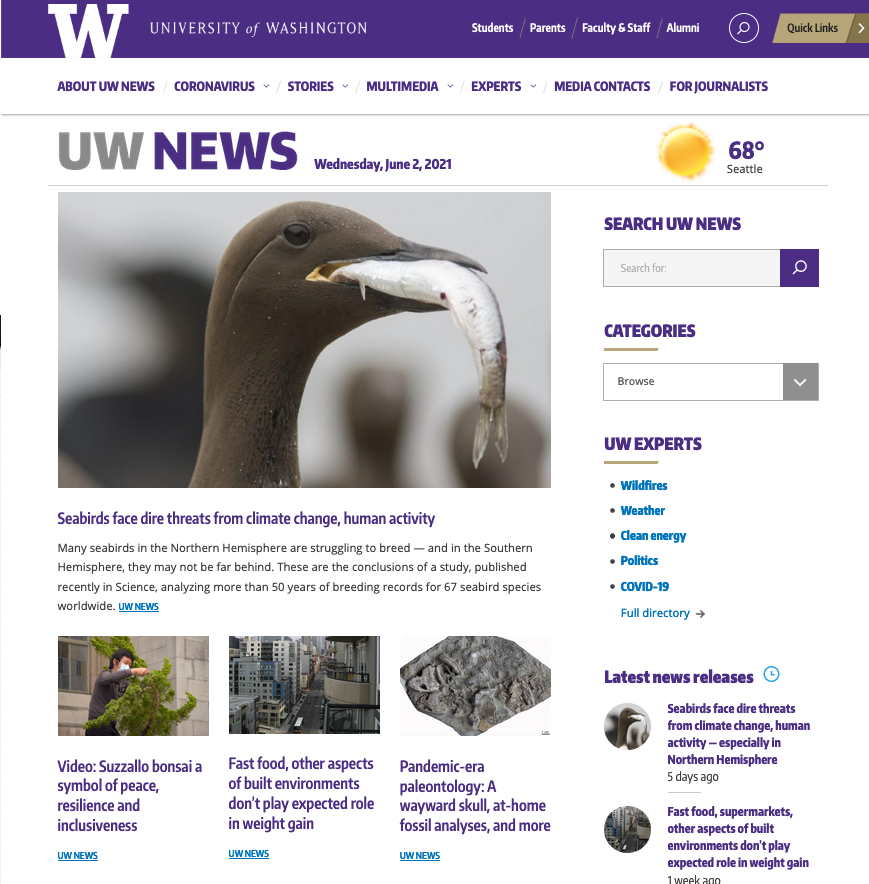 ] .footnote[*: As seen by Chrome] --- # Fetch the Page 1. Connect to the **Internet** and ask for the URL 2. As a **DNS** (Domain name service) to find the machine with the appropriate resources 3. Ask the machine with the resources for the web page with a **GET request** 4. Transfer file(s) the internet using the **TCP/IP** protocol back to your machine. --- .left-column[# View Page Source [Spot The Heron Solution](webpages/spottheheron-solution.html) ] .right-column[ 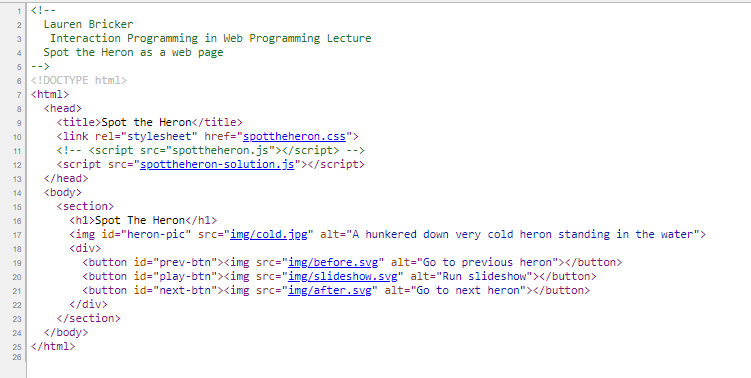 ] --- # Parse and Display the Page .left-column[ 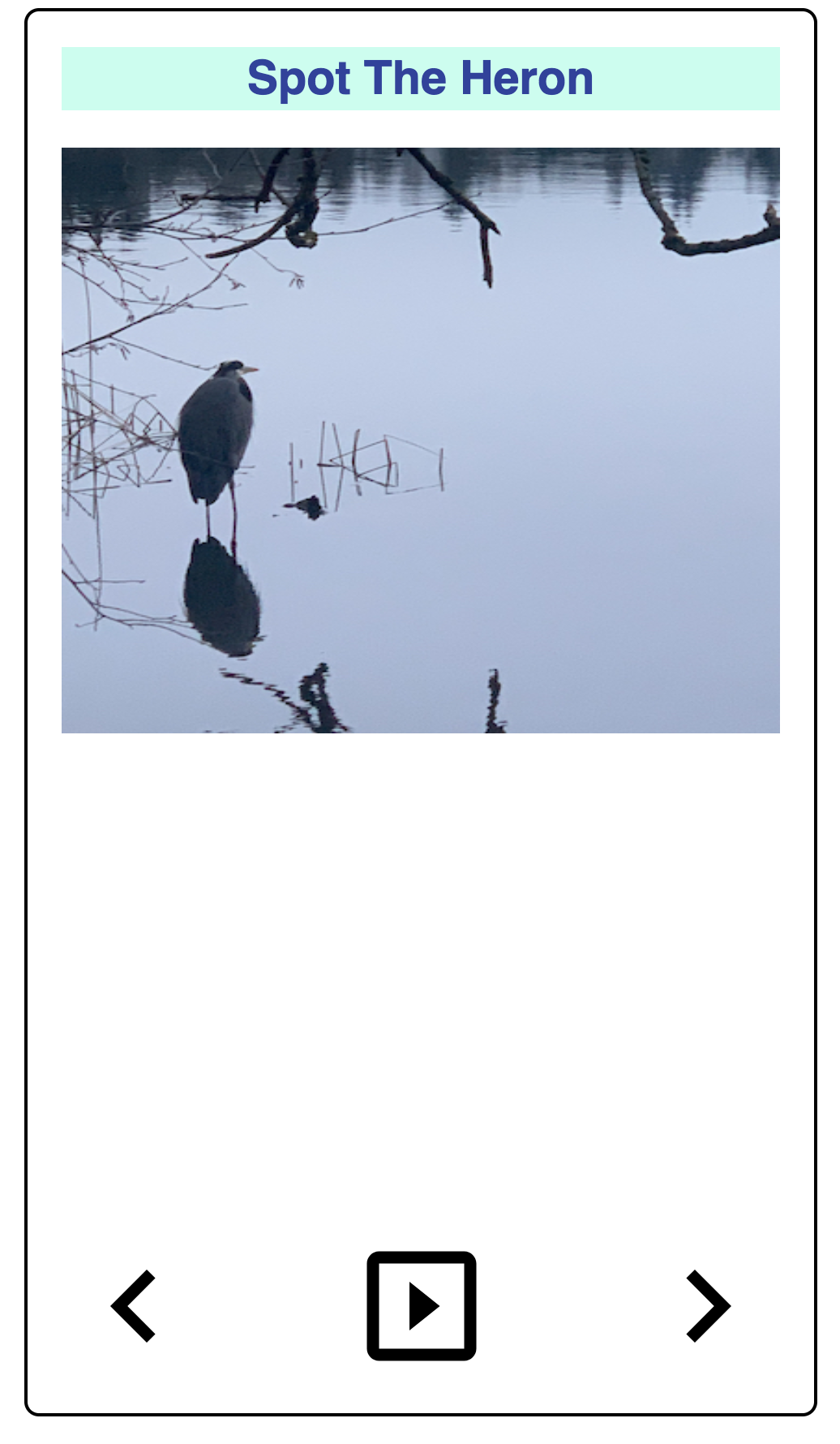 ] .right-column[ 1. First line: - Ok: need to build an internal representation of the page 2. Line-by-line, go through the HTML - If one of the tags links to a cascading style sheet (CSS) file, load and parse it - If one of the tags links to Javascript (JS) for behavior, load and parse it 3. FINALLY display the page… ] --- # Hypertext Markup Language (HTML) - A subset of XML - Keywords that are surrounded by the ‘<‘ and ‘>’ (“alligators”) are Tags - Tags label the structure of parts of your web page - You put the associated content within the tags - There are two types of tags - Open and closing pairs - Ex: This is some text for my body - Most content tags will be of this type - Self-closing tags - `
`, `
`, `
`, `
` - Every tag must be a pair or self-closing! - Tags can be nested! --- # Basic HTML Skeleton ```html
``` --- # Adding content - There are 100s of tags! See [Mozilla Developer Network](https://developer.mozilla.org/en-US/docs/Web/HTML/Element)! - Some simple tags - Title `
` (which nests inside your ``) - Headings `
` .. `
` - Paragraphs `
` - Ordered or unordered lists: `
`, `
`, with list elements `
` - Horizontal rules `
` - Strong `
` which defaults to a bold style and emphasis `
` which defaults to italicized in most browsers. --- # Adding content - There are 100s of tags! See [Mozilla Developer Network](https://developer.mozilla.org/en-US/docs/Web/HTML/Element)! - Some simple tags - Some tags add semantic context - `
`: The header or banner that displays the title of the page - `
`: The bulk of the content of the page - ``: The footer is optional but you can put contact info and copyright date in there. --- # Adding content - There are 100s of tags! See [Mozilla Developer Network](https://developer.mozilla.org/en-US/docs/Web/HTML/Element)! - Some simple tags - Some tags add semantic context - Some tags need additional information, added to a tag with attributes - Links to other pages `<a href="filename"></a>` - Links to images `
` --- # Adding content - There are 100s of tags! See [Mozilla Developer Network](https://developer.mozilla.org/en-US/docs/Web/HTML/Element)! - Some simple tags - Some tags add semantic context - Some tags need additional information, added to a tag with attributes - Some tags (comments) are important for documentation `` --- # Document Object Model (DOM) .left-column[ 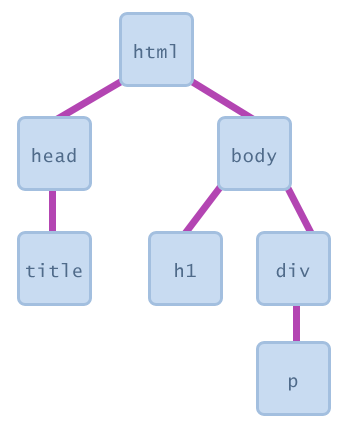 ] .right-column[ - This builds a hierarchy of document elements in what we call the **Document Object Model** - Looks very much like the Android Interactor Hierarchy ] --- # Cascading Style Sheets (CSS) - Allows us to change the look and feel of the content on the page - Style is separated into a .css file - Makes styling multiple pages easier - Allows changing multiple pages easier - Style sheets must be linked to an html page in the for the styles to work `<link href=“style.css” rel=“stylesheet” />` - Great example is [CSS Zen Garden](http://www.csszengarden.com/) - Exercise in [Ed](https://edstem.org/us/courses/32031/lessons/51137/slides/286518) --- # CSS .left-column50[ - Files consist of one or more rule sets - Each rule set has a selector which chooses which HTML elements you want to style - Style properties are set with rules which are property/value pairs - Syntax is important - More on [CSS](https://developer.mozilla.org/en-US/docs/Web/CSS) ] .right-column50[ 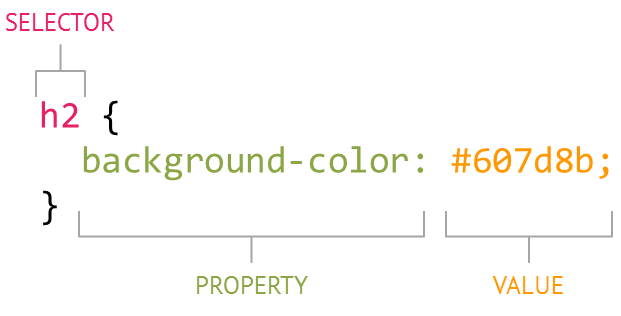 From [W3Schools](https://code.makery.ch/library/html-css/part3/) ] --- # Layout in CSS Layout can be [complicated](https://www.amazon.com/CSS-Awesome-Mug-Programmer-Developer/dp/B06Y13QC8N), fortunately there is CSS [Flexbox](https://courses.cs.washington.edu/courses/cse154/flexboxducky/) or [Grid](https://cssgridgarden.com/)!! 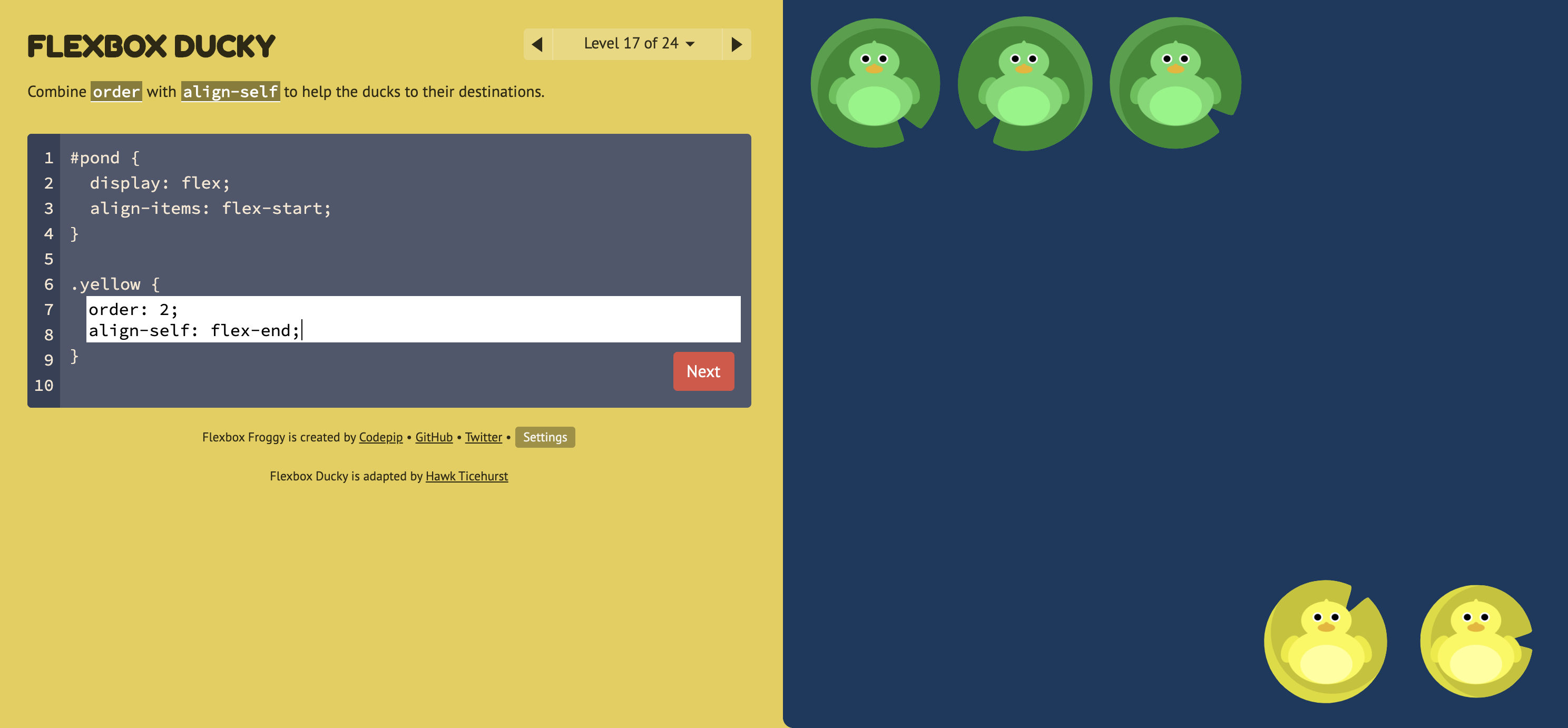 --- # Spot The Heron Conversion .left-column[ Mind blowing demo time! ] .right-column[ 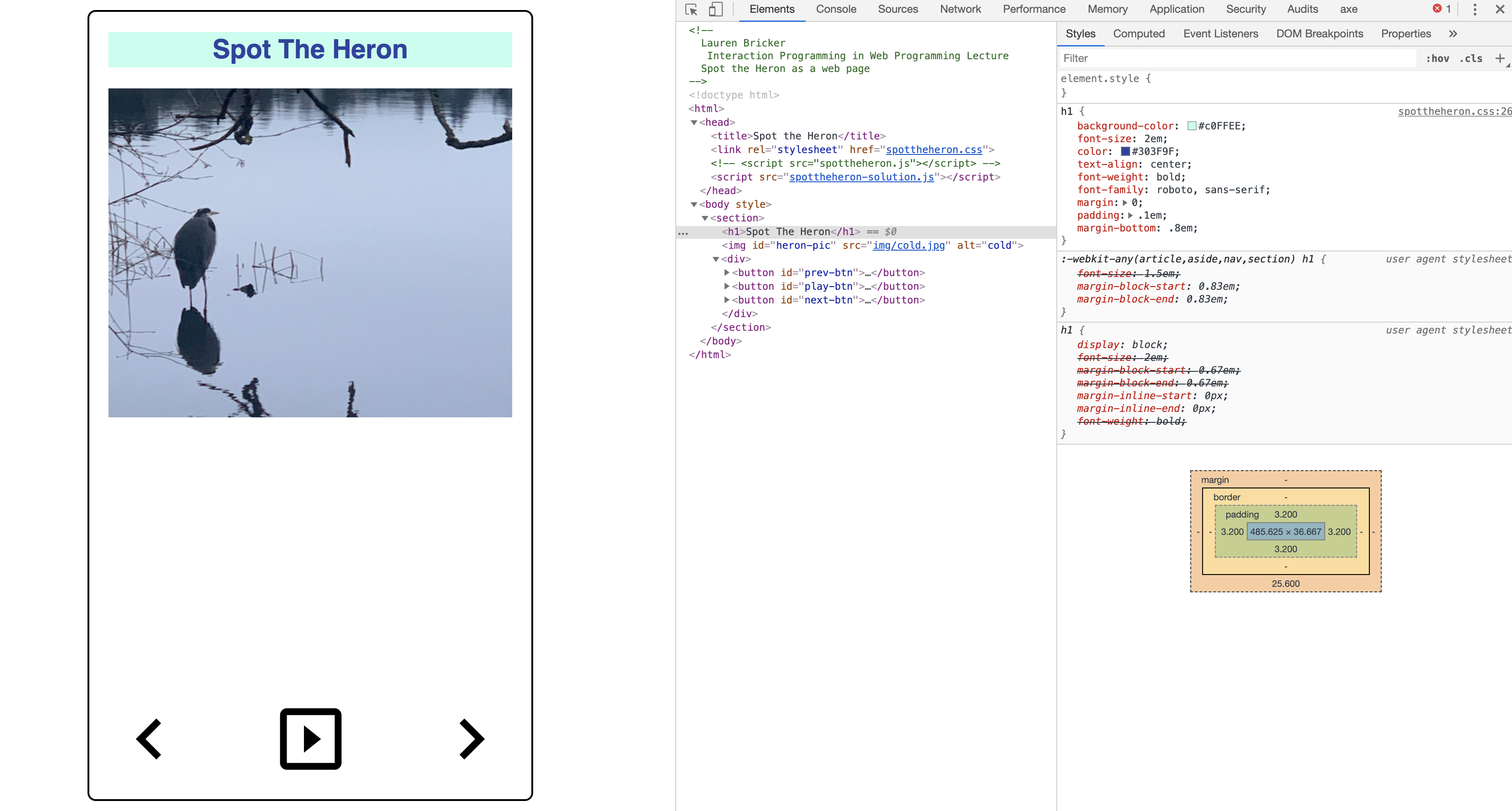 ] --- # Some Comparisons | Android | Web | | --- | --- | | Java | HTML/CSS/JS | | Layouts | CSS Flexbox or Grid | | Interactor Hierarchy | Document Object Model (DOM) | | Content Description | alt text | | Paint objects on a canvas | CSS | | `onCreate` | `window.addEventListener("load", init);` | | `View.OnClickListener#onClick` | `domElement.addEventListener("click", callback);` | --- # The Application Stack for Devices - Review .left-column[.font-smaller[
graph LR ap[Application Program] hlt[High Level Tools] t[Toolkit] w[Window System] o[OS] h[Hardware] class ap,w,o,h yellow class hlt,t green
]] .right-column[ - **Application Program** - An application designed for an end user to perform specific tasks. - **High Level Tools** - Graphical interfaces that that let you specify parts of your interface (such as layout). Subject to Worfian Effects - **Toolkit** - A set of libraries and tools you use to develop applications. - **Window System** - Manages window size and visibility across applications - **OS** - The operating system that is running on the device, providing system services such as acceess to displays, input devices, file I/O - **Hardware** - The device that is running software, such as an Android Phone High level tools and the the Toolkit are generally packaged as part of a toolkit but really should be thought of as separate things. (You can program the toolkit without the tools. ) ] --- # The Application Stack for Web Dev .left-column[.font-smaller[
graph LR ap[Application Program] hlt[High Level Tools] t[Toolkit] w[Window System] o[OS] h[Hardware] class ap,w,o,h yellow class hlt,t green
]] .right-column[ - **Application Program** - A web page - **High Level Tools** - Dream Weaver, Adobe XD, Google Web Designer, etc - **Toolkit** - HTML/CSS/JavaScript, jQuery, React, etc. Browser tools. - **Window System** - Browser running in a Windowing system. - **OS** - The web browser itself? Or the browser and the OS combined? - **Hardware** - The device that is running OS and browser, such as a Mac, PC or Phone. You can do web programming with a notepad editor and a browser, nothing more. ] --- # End of Deck