# Warmup: .left-column-half[ Recall the ["Door Study"](../wk03/people-vision.html#59) from our Properties of People 1: Vision class. Think, then discuss with your neighbor: do you think this follows the basic ethics of the [Belmont Report](../wk06/menus-experiment.html#53) (Beneficence, Respect for Persons, Justice)? We'll share out in a moment ] .right-column-half[ ![:youtube Video of someone asking for directions while another person walks by with a door,FWSxSQsspiQ] ] --- name: inverse layout: true class: center, middle, inverse --- # Undo reasoning and implementation Lauren Bricker CSE 340 Winter 23 --- layout: false [//]: # (Outline Slide) # Today's Agenda - Administrivia - Menus part 5-6, due Thur 16-Feb @10pm - Menus code will be graded over the weekend - Undo - parts 1-7 due Fri 24-Feb - part 8 (reflection) due Sun 26-Feb - A note on `View` visibility - Learning goals - Introduce need for Undo by reviewing mental models - Introduce Undo conceptually - Describe Implementation details for assignment --- ## A Note from Menus A TODO item in `MenuExperimentView#startSelection` prompted you to make the menu visible. How did you accomplish that? -- count: false ```Java setVisibility(VISIBLE); ``` .footnote[(see the Android documentation for [View#setVisibility(int)](https://developer.android.com/reference/android/view/View#setVisibility(int))) ] -- count: false A TODO item in `MenuExperimentView#endSelection` prompted you think about what to reset other than the state machine? What did you do there? -- count: false We saw two different solutions that mean different things: ```Java setVisibility(INVISIBLE); ``` ```Java setVisibility(GONE); ``` --- # `INVISIBLE` vs `GONE` `INVISIBLE` Means the view is invisible, but it still takes up space for layout purposes ([doc](https://developer.android.com/reference/android/view/View#INVISIBLE)) `GONE` Means the view is invisible, but it **does not** take up space for layout purposes ([doc](https://developer.android.com/reference/android/view/View#GONE)) .footnote[(see the Android documentation for [View#setVisibility(int)](https://developer.android.com/reference/android/view/View#setVisibility(int))) ] -- count: false (Hint: something to write down...) You will need to know this difference for Undo.... --- .left-column40[ ## Reminder: Every system has at least 3 different models ] .right-column60[
graph TD S[System Image:
Your Implementation ] --> |System Feedback | U[User Model: How the user thinks
the system works] D[Design Model: How you
intend the system to work ]-->S U -->|User Feedback | S
] --- # Relating the Human and the Interaction .column[ 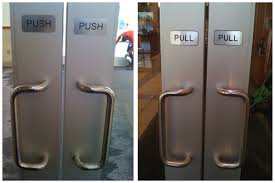 ] .column[ 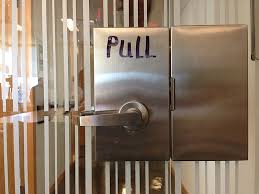 ] -- .column[ 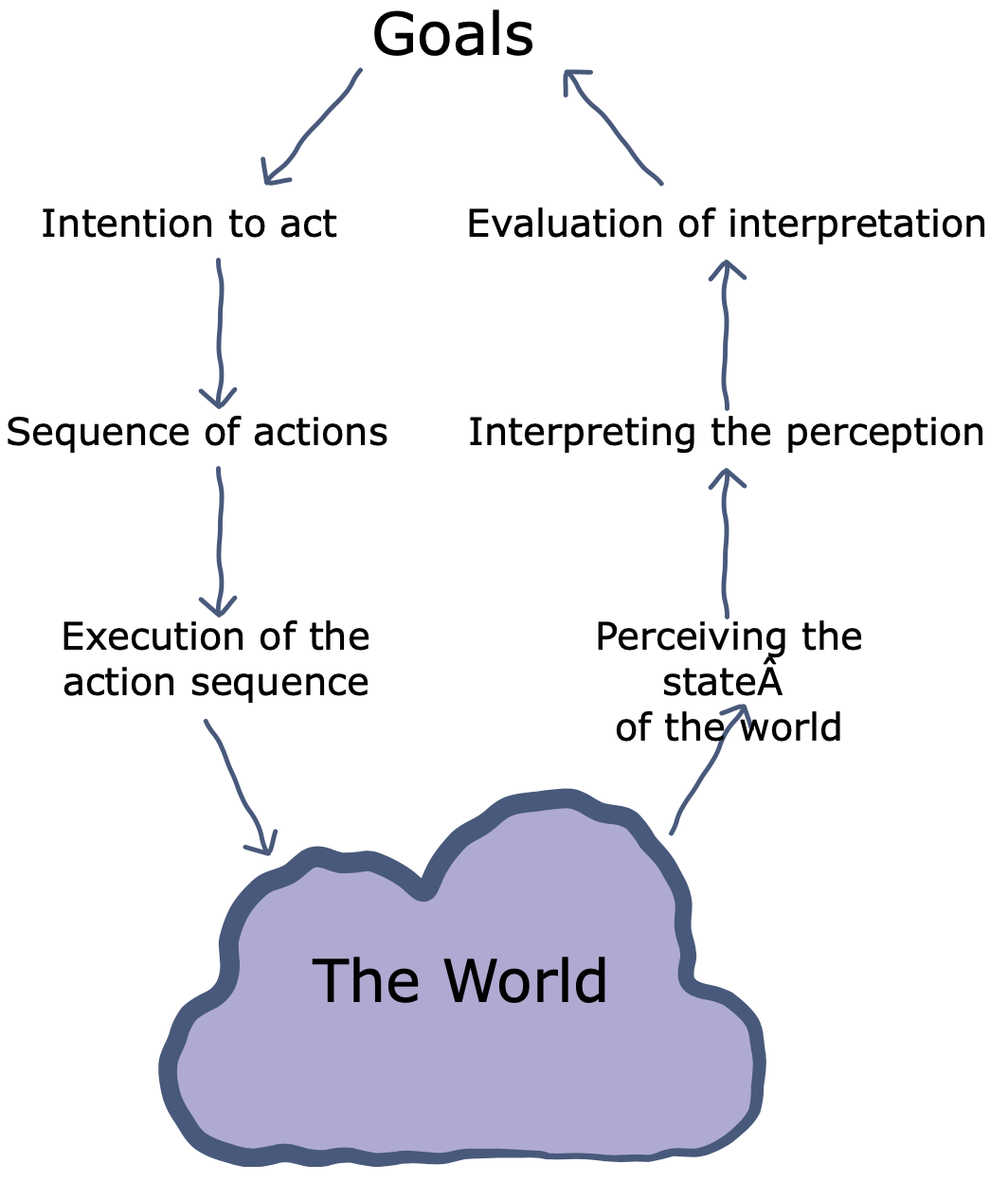 ] .footnote[[Don Norman, When Three World Collide: A model of the Tangible Interaction Process, 2009](https://www.researchgate.net/publication/221332102_When_three_worlds_collide_A_model_of_the_tangible_interaction_process)] ??? Note to instructors: Need to change image to mermaid --- # Relating the Human and the Interaction .right-column70[ **Gulf of Execution** is the user's belief in the functions the system _doesn't have_ **Gulf of Evaluation** is where the user _doesn't realize the system HAS a functionality_. ] .left-column30[ 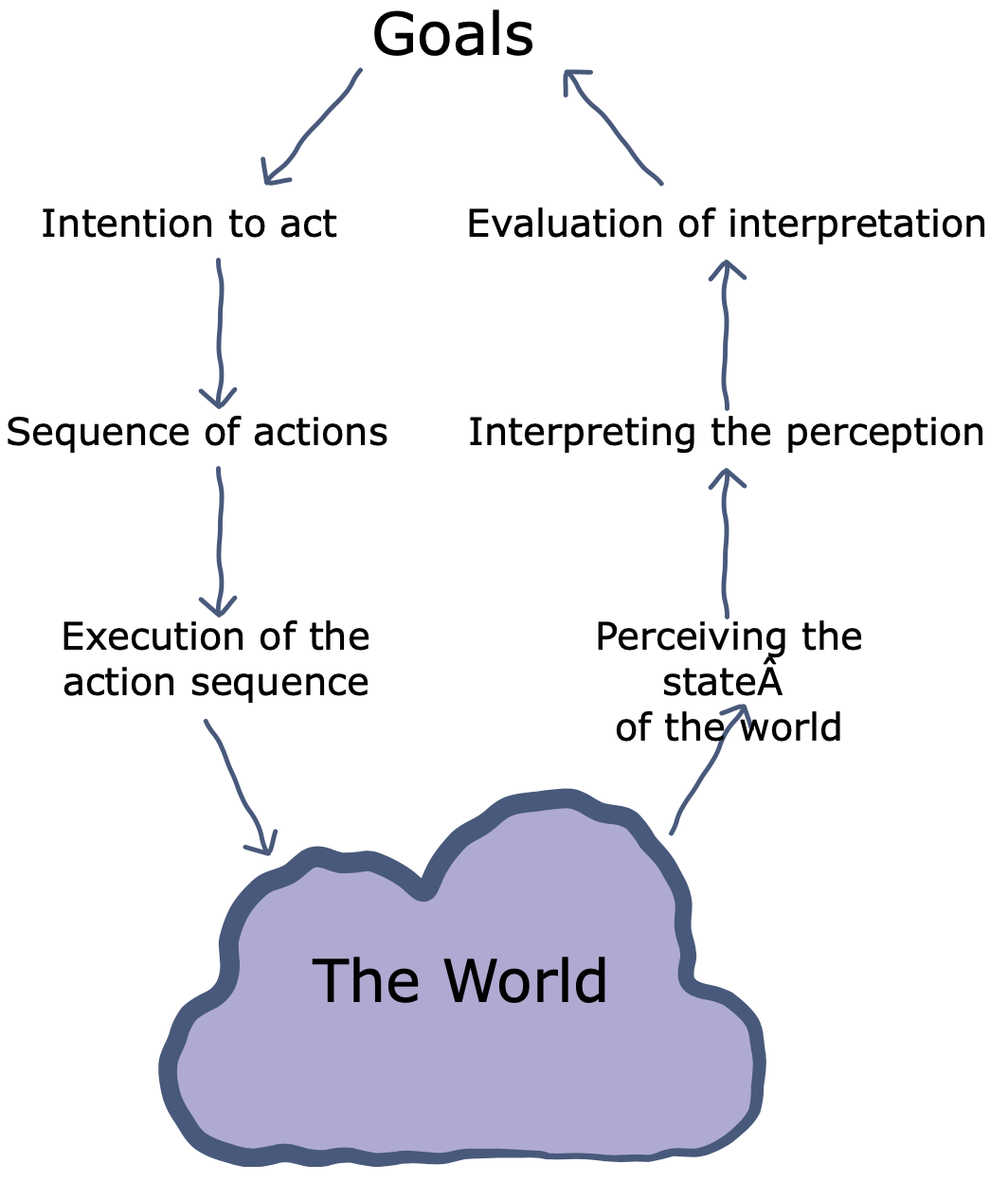 ] --- # Better definitions .right-column70[ **Gulf of Execution** is "the gap between a user's goal for action and the means to execute that goal."
1
**Gulf of Evaluation** is the difficulty interpreting the state of a system and how well the interface supports the discovery and interpretation of that state.
2
- "The gulf is small when the system provides information about its state in a form that is easy to get, is easy to interpret, and matches the way the person thinks of the system" - Don Norman ] .left-column30[ 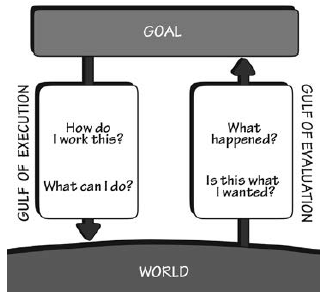 ] .footnote[
1
[Gulf of Execution](https://en.wikipedia.org/wiki/Gulf_of_execution#cite_note-3)
2
[Gulf of Evaluation](https://en.wikipedia.org/wiki/Gulf_of_evaluation) ] --- # Relating Gulfs and Mental Models .left-column40[ 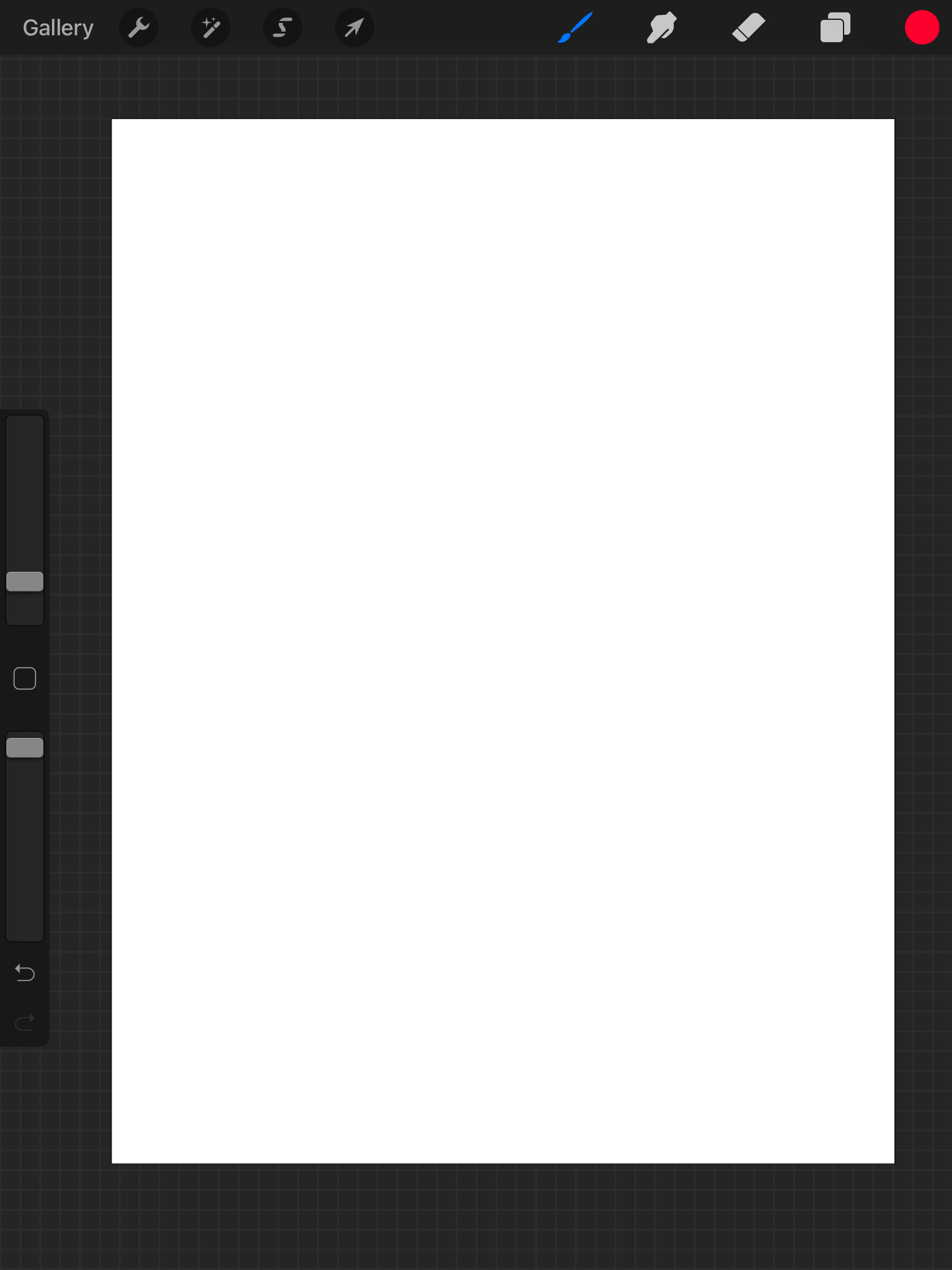 ] .right-column60[ How do you use this program? - What "affordances" do you see? - What features do you think exist? - How would you first approach this interface? - What would you try? ] --- # Relating Gulfs and Mental Models .left-column40[ 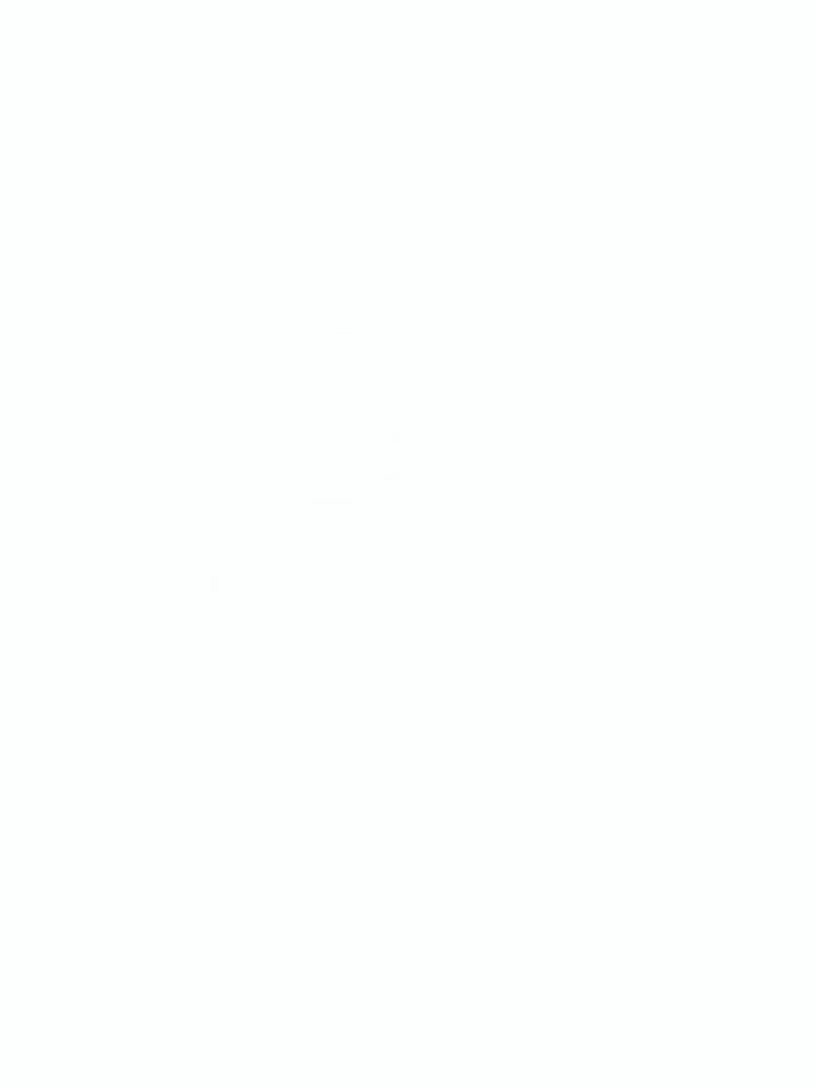 ] .right-column60[ What are you noticing here? ] --- # Relating Gulfs and Mental Models .left-column40[ 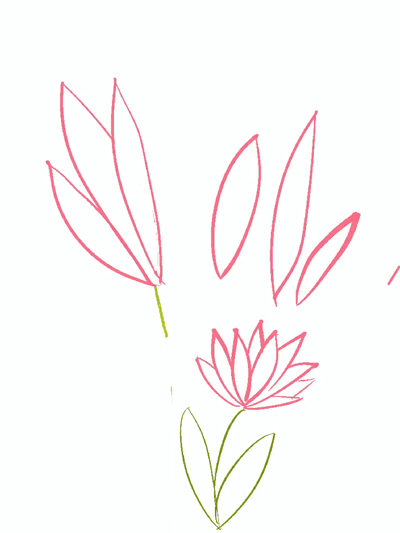 ] .right-column60[ How does a user explore the system? What happens when the user does something they think is core but is really not supported? ] -- count: false .right-column60[ - Need to undo! ] --- class: center, middle, inverse # How do we support Undo? --- layout:false .left-column-half[ ## Remember this? 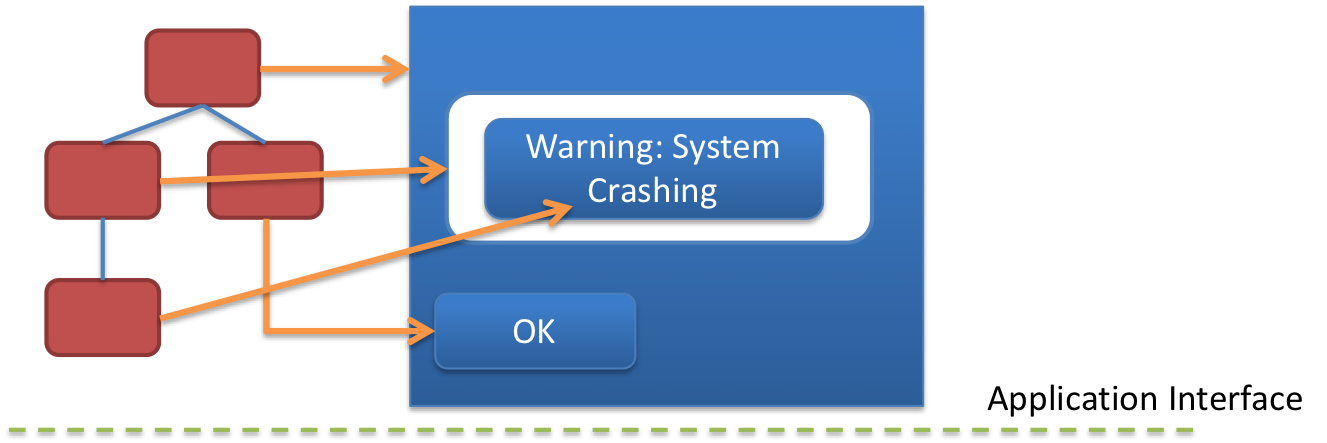] .right-column-half[ Dispatch Strategies - Bottom-first and top-down positional - Focus-based State Machine describes *within-view* response to events ] --- .left-column-half[ ## Event Dispatch 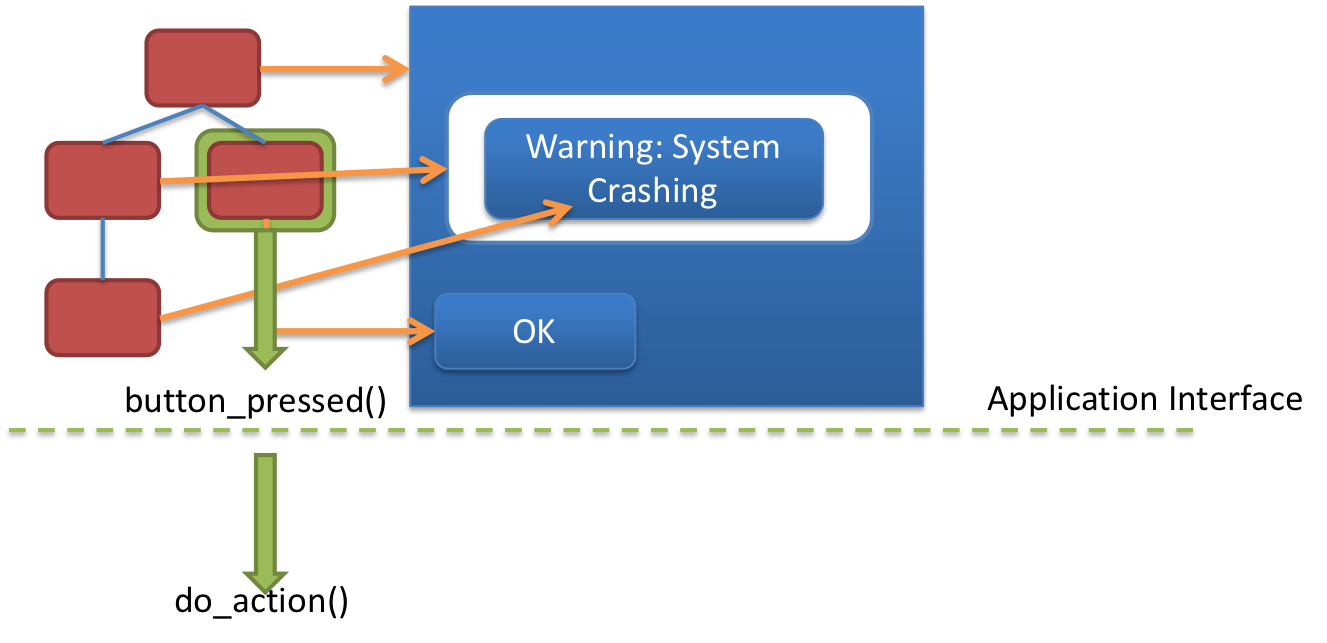] .right-column-half[ Callbacks handle *application* response to events - Update Application Model ] --- .left-column-half[ ## Event Dispatch 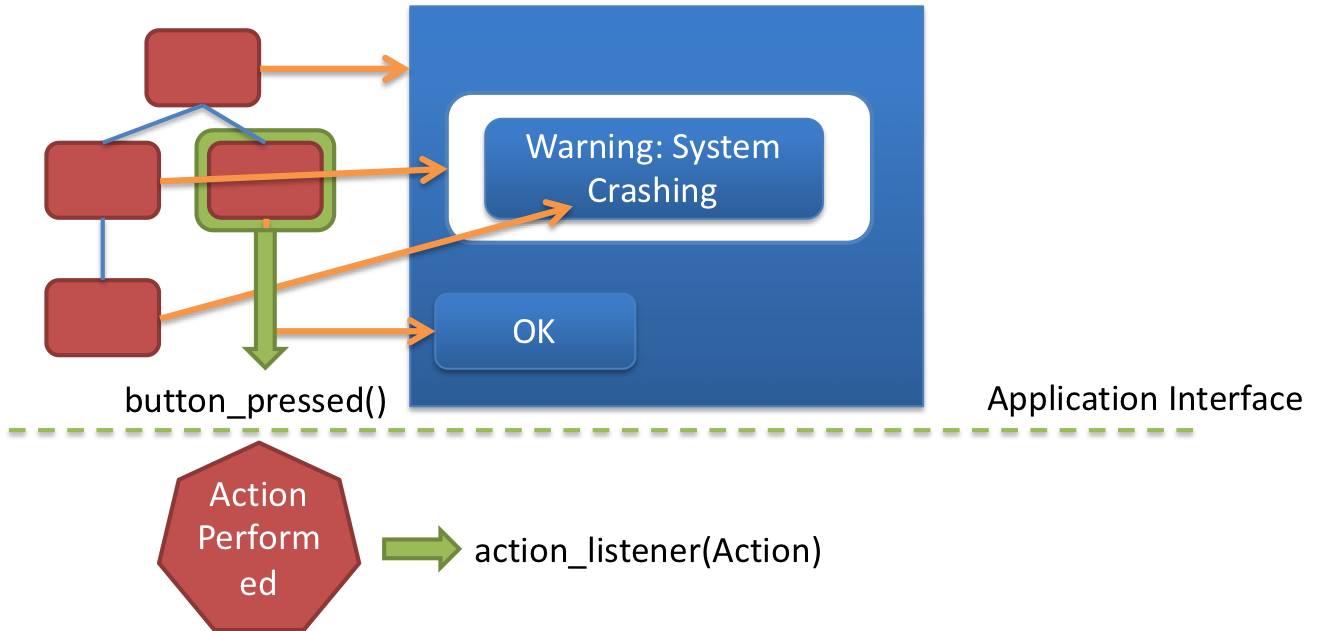] .right-column-half[ Callbacks handle *application* response to events - Update Application Model - Best implemented using custom listeners ] --- # What is `ActionPerformed`? `Higher level` input event (`Command` or `Action` object) - Puts some separation between UI and translation objects - Application (or UI) can ‘listen’ for these events: Key advantage: interactors don’t need to know who/what got notification Same basic flow as simple callbacks --- # What should an Action object do? `doAction()` ??? seems like a lot of work when we could just directly do the action. Major reason for action objects -- `undoAction()` ??? What additional information do we need to undo an action? --- # Advantages of an action object .left-column[ - can be stored on an undo stack - can create a consistent abstraction for reversing an action ] .right-column[
classDiagram AbstractAction <|.. AbstractReversibleAction AbstractReversibleAction <|.. ChangeColorAction AbstractReversibleAction <|.. ChangeThicknessAction AbstractReversibleAction <|.. AbstractReversibleViewAction AbstractReversibleViewAction <|.. StrokeAction class AbstractAction { doAction() } class AbstractReversibleAction { +boolean done +undoAction() } class AbstractReversibleViewAction { +invalidate }
] --- # Where do we store actions? A stack .left-column50[
classDiagram AbstractHistory <|.. StackHistory class AbstractHistory { addAction(AbstractReversibleAction action) undo() redo() canUndo() canRedo() } class StackHistory { +capacity: "Max stack size" }
] .right-column50[ Why a stack? ] ??? Consider having some volunteers be actions and have them act it out? --- # Undo and Redo 1. new action object created and `doAction()` called 2. Undo stack updated 3. new action object created and `doAction()` called 4. Undo stack updated 5. `undo()` invoked 6. Undo stack reduced and Redo stack increased 7. `undo()` invoked 8. Undo stack reduced and Redo stack increased 9. `redo()` invoked 10. Redo stack decreased and Undo stack increased 11. new action object created and `doAction()` called 12. Redo stack cleared and Undo stack stack increased ??? draw sequence --- # What if an action can't be undone? Actions that put system into a totally different context (like saving a file) Clear both `undo` *and* `redo` stacks! -- Users may get frustrated with this design --- # Implementing undo() System pops action off undo stack Calls `undoAction()` method on it Pushes it on redo stack --- # Why is undoAction() hard? Two ways to implement `undoAction()`: - *Direct Code* (each action object has custom code) - Need parameters of original action - Better store in `doAction()` for later - This is what we will implement --- # Why is undoAction() hard? Two ways to implement `undoAction()`: - *Direct Code* (each action object has custom code) - *Change Records* (Keep a record of the “old value” for everything changed by the application, then put all those values back to undo) - Like some version control systems - More general - Takes more space - `Action` object records `ChangeRecord` (changes which are abstracted into a common data format) - Application has to provide code to restore from change records --- # Implementing redo() System pops action off redo stack Calls `doAction()` method Pushes it on undo stack --- # More sophisticated forms of Undo .left-column60[ Explicit visualization of steps Manipulation of action list Delete actions from the middle, reorder, etc. by undoing back to point of change then redoing forward But note: `doAction()` must be able to work in new context ] .right-column40[ 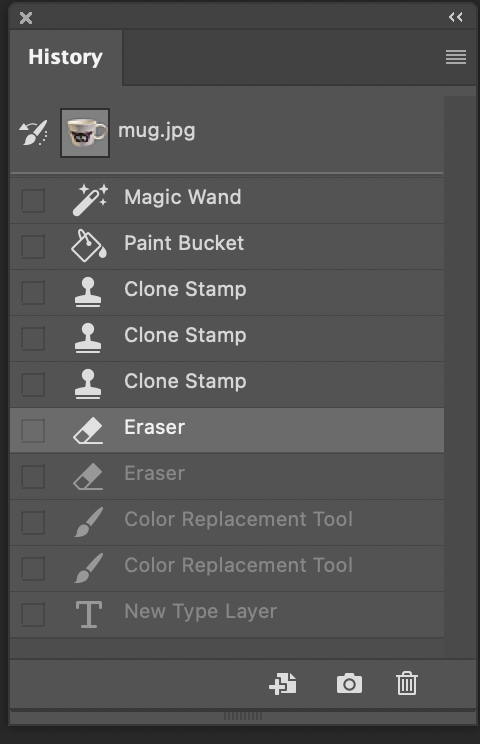 ] --- # Flatland: Semantic Undo .left-column30[ 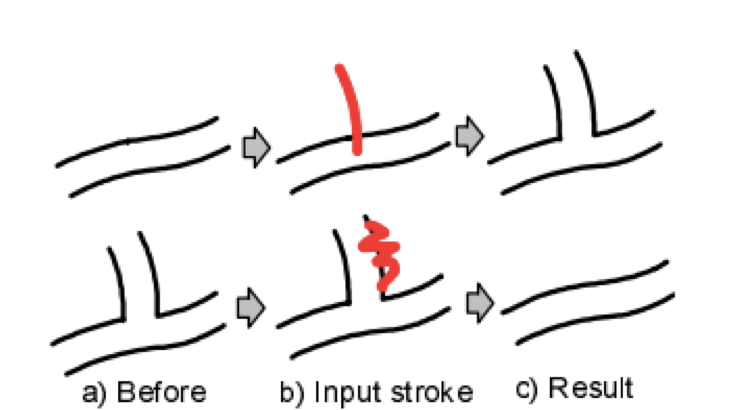 ] .right-column60[ 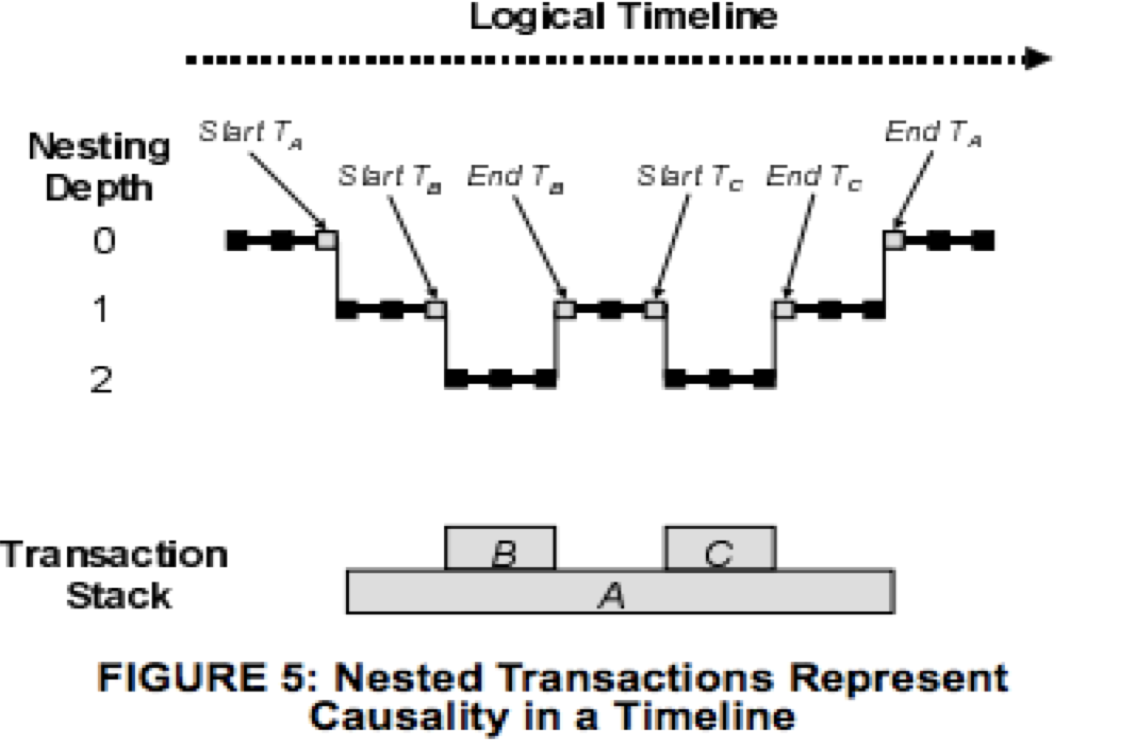 ] .footnote[ Edwards, W. K. ; Igarashi, T. ; LaMarca, A .; Mynatt, E. D. A temporal model for multi-level undo and redo. UIST 2000, Proceedings of 13th Annual ACM Symposium on User Interface Software and Technology; 2000 November 5-8; San Diego, CA. NY: ACM; 2000; 31-40. ] --- # Discussion of assignment HCI Goals - Modify and existing app in a consistent fashion - Make your modifications accessible - Make your modifications usable Android Goals: - Be able to understand and modify an existing user interface - Learn about floating action buttons - Integrate a custom ColorPicker view - Implement core data structure for Undo Open ended portion: Implement something new --- # Discussion of assignment .left-column50[ ![:youtube Video of assignment, V9_LEi6wLsw] ] .right-column50[ ![:youtube Video of assignment, KKJTSkVnBLc] ] Other example videos on this [playlist](https://www.youtube.com/playlist?list=PLdU5lz7sdq7k5GL82NgOP5lCZzyoZh9wK) --- # Discussion of assignment .left-column[ Like accessibility, you are modifying a fully working program Lots to explore and understand ] -- .right-column[
graph TD M[ReversibleDrawingActivity] --> D[DrawingView] M --> FUndo[FAB:Undo] M --> FRedo[FAB:Redo] M --> FColor[FAB:Color] M --> FThick[FAB:Thickness] FColor --> Red[Red] FColor --> Green[Green] FColor --> Blue[Blue] FThick --> Thin[Thin] FThick --> Med[Med] FThick --> Thick[Thick] classDef normal fill:#e6f3ff,stroke:#333,stroke-width:2px; classDef start fill:#d1e0e0,stroke:#333,stroke-width:4px; class M,D,FColor,FThick,Vis start class Red,Green,Blue,Thin,Med,Thick,Hid normal
] --- # Discussion of assignment .left-column[ DrawingView: Holds strokes FABs: - Green ones are always visible - Purple ones are only visible when active ] .right-column[
graph TD M[ReversibleDrawingActivity] --> D[DrawingView] M --> FUndo[FAB:Undo] M --> FRedo[FAB:Redo] M --> FColor[FAB:Color] M --> FThick[FAB:Thickness] FColor --> Red[Red] FColor --> Green[Green] FColor --> Blue[Blue] FThick --> Thin[Thin] FThick --> Med[Med] FThick --> Thick[Thick] classDef normal fill:#e6f3ff,stroke:#333,stroke-width:2px; classDef start fill:#d1e0e0,stroke:#333,stroke-width:4px; class M,D,FColor,FThick,Vis start class Red,Green,Blue,Thin,Med,Thick,Hid normal
] You should read through `AbstractDrawingActivity#addCollapsibleMenu` and `ReversibleDrawingActivity#toggleMenu` to see how Undo "opens up" a FAB menu --- # Code deliverables There are five parts for the coding part of this assignment: - Part 1: Implement `ChangeThicknessAction` - Part 2: Implement history - Part 3: Add a thickness 0 FAB to the thickness menu - Part 4: Integrate colorpicker - Part 5: Your own Undo-able thing --- # End of Deck