# [CSE 340](/courses/cse340/23wi/schedule.html) Lab 5 Winter 23 ## Week 5: Event Handling .title-slide-logo[ 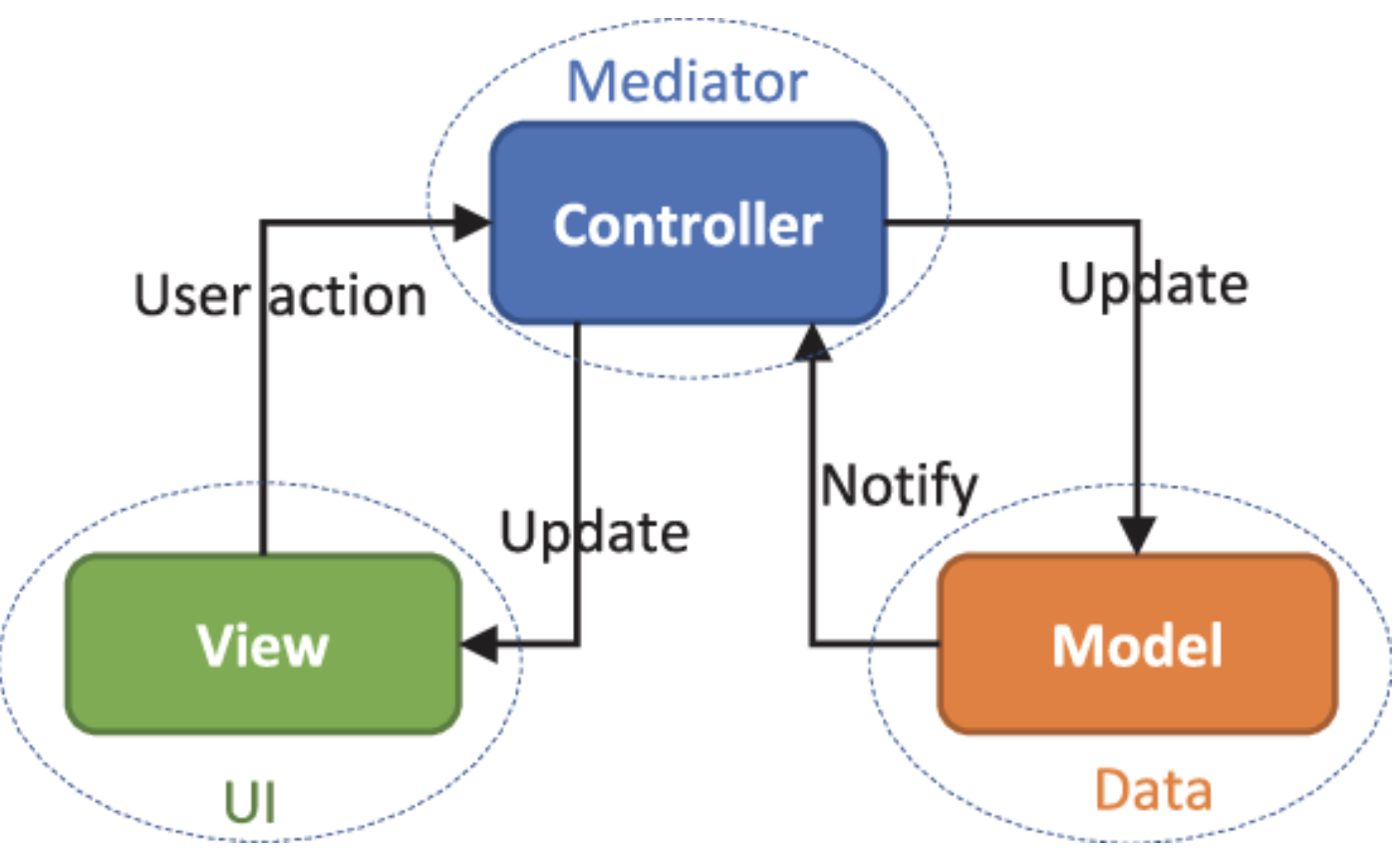 ] --- # Timeline - **Accessibility** Due: Thur 2-Feb - **Menus** - Checkpoint Due: Wed 8-Feb - Code Due: Sat 11-Feb - Report Due: Thur 16-Feb --- # Looking Ahead: Menus User Study You will be conducting a [user study](https://www.interaction-design.org/literature/topics/user-research). - Carefully review the implementation portion of the spec. - Test your app and make sure there are no unexpected/runtime errors. - Find 3 adult consenting participants (can be other students, TAs, family, etc) - If contacting a TA, please post on Ed and give us time to respond before the deadline. --- # Section 5 Objectives - [Review: Event Record](#5) - Section Exercise: Event Records - [Review: Callbacks and listeners](#33) - [Spot The Heron App](#36) - [Model View Controller](#39) --- # Event Record Review (1/5) Event Record: theoretical plan to capture information about a UI event - What (event type) - Where (input target) - When (time) - Value (XY location, which key, mouse coordinates...etc) - Context (Modifiers: CTRL, ALT... etc) --- # Event Record Review (2/5) **Example UI Event 1: User double taps to like a post on Instagram.** - If we were to represent this using an Event Record what would the record contain in terms of each category of recorded information? - Give hypothetical examples - Submit your response on Ed (Question 1) --- # Event Record Review (3/5) **UI Event 1: User double taps to like a post on Instagram, example answers** -- - What: -- Touch -- - Where: -- Image (probably a sub-component inside a “Post” object) -- - When: -- 2021-02-04-1234 (absolute time) maybe even the time passed since the user opened the app or performed some action! -- - Value: -- XY location of tap -- - Context: -- Double-Tap --- # Event Record Review (4/5) **Example UI Event 2: On a smartphone, user presses the volume up button** - If we were to represent this using an Event Record what would the record contain in terms of each category of recorded information? - Submit your response on Ed (Question 2) --- # Event Record Review (5/5) **UI Event 2: Press volume up button** -- - What: -- Key down -- - Where: -- Physical volume button on phone -- - When: -- Absolute time (depending on what info you want to store) -- - Value: -- Volume level, duration of press... etc -- - Context: -- Depending on the phone, pressing the power button and volume up button at the same time may trigger a screenshot or soft reset --- # Key Points of Event Record (1/2) - Where: - **Part of the system that is going to receive the event.** - Ex: 'Hey Google' -> subsystem in the OS that takes in voice commands. - Value: - **How you can measure the input.** - Not the same as 'What' in the Event Record. - Ex: 'Hey Google' -> Command you are saying after prompting 'Hey Google', like "what's the weather in Seattle?" --- # Key Points of Event Record (2/2) - Context: Modifiers - **Things that change the meaning/nature of the event.** - Ex: Pressing the volume button is an event... context of turning up the volume or in the context of taking a screenshot? - Pressing the power button alongside the volume would modify the context of this event. --- # Create your Own! - Come up with your own event record and list its what, where, when, value, and context. - Submit your response on Ed (Question 3) --- # Review: Callback and Listeners - **Callback**: method gets called when event happens, delivered to a component that is registered to listen for the event - **Event Listeners**: type of event handling callback - Example Higher level events (already provided for you by Android View, based on Touch Events) - onClickListener, onFocusListener, onDragListener... - Override these to modify what happens in response to such events Can also define [custom listeners](https://guides.codepath.com/android/Creating-Custom-Listeners) that are triggered based on how you define it (ex: trigger upon HTTP GET request is successful and data loaded) --- # onClick vs onTouch - [onTouchListener](https://developer.android.com/reference/android/view/View.OnTouchListener?authuser=1) = allows you to control what happens in terms of different MotionEvents - Ex: ACTION_UP, ACTION_DOWN, ACTION_MOVE.. And many more! - [onClickListener](https://developer.android.com/reference/android/view/View.OnClickListener) = for when user clicks the view - used for a more basic purpose - cannot handle MotionEvents like onTouch - Android docs: Handling a click event of a Button [programmatically](https://developer.android.com/guide/topics/ui/controls/button#ClickListener) --- # Reading the Documentation - Let's read through and understand the [Input Events Documentation](https://developer.android.com/develop/ui/views/touch-and-input/input-events) - Take a look at how we can set the onClickListener for [Buttons via XML and Programmatically](https://developer.android.com/develop/ui/views/components/button#ClickListener) --- # Spot the Heron Lab - We've worked with this application in lecture already 1. Clone [Spot the Heron App click branch](https://gitlab.cs.washington.edu/cse340/exercises/cse340-spot-the-heron/-/tree/click) 2. Find a partner or two in the classroom to work with 3. Run the code, then investigate the TODOs in MainActivity.java. - Get the "Next" and "Previous" button to go to the next/previous image in the slide - Get the "Slideshow" button to toggle Slideshow mode --- exclude: true # Spot the Heron App, Again! - [Solution code](https://gitlab.cs.washington.edu/cse340/exercises/cse340-spot-the-heron/-/tree/click-solution) on the same repo --- # Spot the Heron Lab - Push your solution to your cloned repo and submit the link to your repo on Ed! --- # Model View Controller Review - What: [Architectural pattern](https://en.wikipedia.org/wiki/Software_design_pattern) used for developing UI - App may be organized into 3 separate major logical components - Way to organize code --- # MVC: 3 parts 1. **Model** = container of pure data - ex: List of Strings describing image alt text 2. **View** = displays the model's data to user, the UI - Layouts, representation of data - ex: XML layout resources 3. **Controller** = mediates between View and Model - Handles event listening/input - Manipulates data within the model, updates the View - ex: Android Java Activities --- # MVC Review The [Section Calculator App, again!](https://gitlab.cs.washington.edu/cse340/exercises/mvc-calculator/-/tree/section5-mvc) - Work with a partner to identify which parts of the app count as the Model/View/Controller? --- # MVC Review: Example Answers - Model: -- [CalculatorModel](https://gitlab.cs.washington.edu/cse340/exercises/mvc-calculator/-/blob/section5-mvc/app/src/main/java/com/example/calculator/CalculatorModel.kt) -> holds pure data the Calculator should store** - Final calculation result - Intermediate result (user has the option to edit their equation before submitting) - CalculatorModel extends Observable (some data to be watched), calls setChanged() and notifyObservers() -- - View: -- [activity_main.xml](https://gitlab.cs.washington.edu/cse340/exercises/mvc-calculator/-/blob/section5-mvc/app/src/main/res/layout/activity_main.xml), etc -- - Controller: -- [Calculator.kt](https://gitlab.cs.washington.edu/cse340/exercises/mvc-calculator/-/blob/section5-mvc/app/src/main/java/com/example/calculator/Calculator.kt) - Handles communication between View (display) and Model (data) - Calculator extends Observer (listens for changes in the model), override update() --- # Question? - Any questions about - Accessibility Assignment - Event Records - Callbacks and EventListeners - MVC