# [CSE 340](/courses/cse340/23wi/schedule.html) Lab 1 Winter 23 ## Week 1: Getting Started With Android Development .title-slide-logo[ 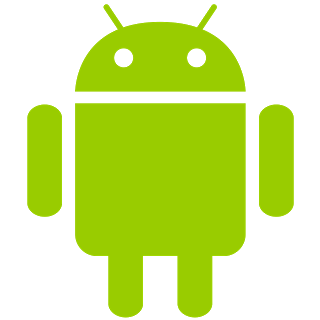 ] --- # Doodle Timeline - Doodle starter code out Wed 4-Jan - Doodle code, reflection, and video due Fri 13-Jan @ 10pm - Doodle peer evals will be distributed on Canvas, Sat 14-Jan - Peer evals due Mon 16-Jan @ 10pm --- ## Reminder!! - Optional Java Refresher Lab today at 4:30pm in LOW 105 ([Zoom link](https://washington.zoom.us/j/95335734045)) --- ## Course Objectives - Practical applications for learnings in lecture - Understand design considerations when making mobile apps - Design and implement basic Android applications - Debugging in Java and for Android --- ## Lab 1 Objectives - Introductions and Ice breaker activity - Course Administrivia - Expectations, Environment Setup, Assignment Submission - Explore Android Project Structure - Overview of Android Fundamentals - Android Debugging Techniques --- ## Ice Breaker: 2 Truths and a Lie Answer these 3 questions (Make one of them a lie...) 1. What’s your most interesting injury? 2. When was the last time you traveled outside your immediate area, and where was it? 3. What’s your favorite game (video, board, party, outdoors, whatever), and why? --- ## Section Exercises We will have section exercises for each section that will contribute towards your participation grade - Due on Fridays at 10pm - Graded based on completion and attempt, not accuracy - We will look through individual answers to make sure that the answers you submit make sense in the context of the problem --- ## Android Applications __Components__ - Activity - Service - Broadcast Receiver - Content Providers We will be mainly working with Activities and Services (towards the end of the quarter) __Intents__ - Launch components (and provide them with data) - Not limited to components of the same app - Meaning: your components can be started by intents from other applications --- ## Activity [`android.app.Activity`](https://developer.android.com/reference/android/app/Activity) - Represents a single interface - Example: Activities for Gmail App - Inbox, Message Compose, Spam Folder, etc. - Activities can be started by other applications, if desired - E.g., sharing a photo by email starts Gmail's Message Compose activity --- ## Activity .center[ 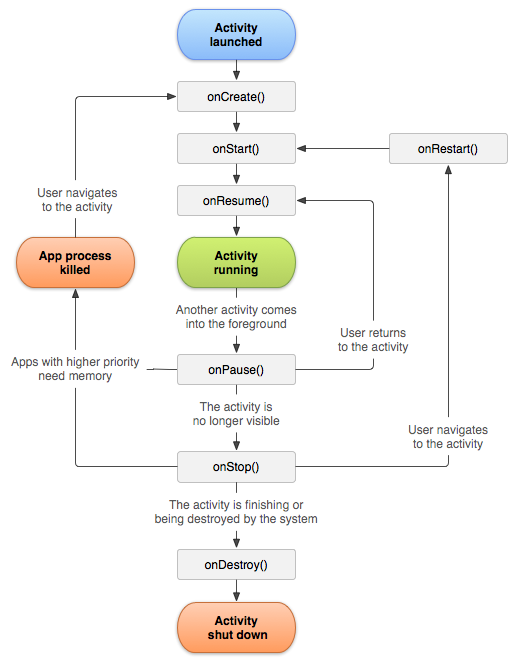 ] --- ## Service [`android.app.Service`](https://developer.android.com/reference/android/app/Service) - Entry point for background processes - Example: Spotify's player activity uses a service to play music - Could be done by activity alone - Use of a service allows music to continue when player activity is no longer in the foreground - Services may be killed and restarted as required by the OS - A persistent service such as music playback may use a persistent notification to prevent this --- ## InitFromParentCoords Attempt Questions 1 and 2 on the Ed Lesson for Section 1 __Question 1__ - Creating a CircleView is specified using its center in parent coordinates and a radius. What is the top left coordinate of the CircleView in child coordinates? __Question 2__ - How would you draw the circle given in the previous problem with center (px, py) in parent coordinates, radius r, paint object p, and a given canvas? --- layout:none # Cloning Assignments - The course staff will create a [Gitlab](https://gitlab.cs.washington.edu/cse340-23wi-students). - Clone and use the repository on your local machine in order to edit it with Android. - You **MUST** work within this assignment directory, as it will be turned in via an Gradescope when it is due. - Reach out to the course staff on our [Discussion board](https://edstem.org/us/courses/32031/discussion/) as soon as possible if you can not find your repository. - Please commit and push your work regularly. - Protects your code - Allow course staff to look at your code - Easily pull any changes we make to the assignment source - You can find instructions on setting up and maintaining a forked repository [here](https://help.github.com/en/articles/working-with-forks). --- .left-column[ ## Cloning Doodle You can find your unique repo by the notification email or by going to [GitLab](https://gitlab.cs.washington.edu/cse340-23wi-students) ] .right-column[ 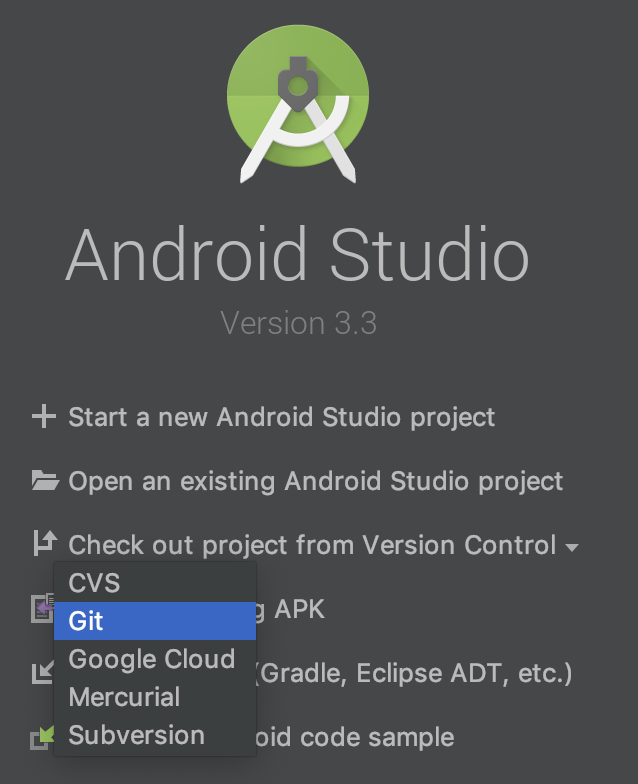 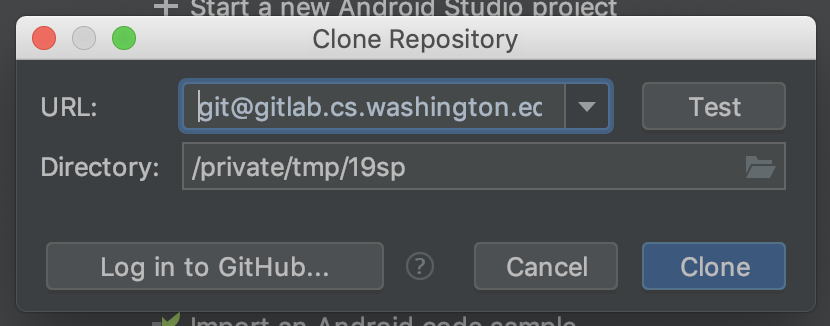 ] ??? Here, open up the [Doodle spec](https://courses.cs.washington.edu/courses/cse340/22wi/assignments/doodle.html) and skim through it. --- # Open project in Android Studio - Run configurations should be automatically imported from Gradle - If not, `build` should trigger an import - Run with ► - Connect an android device by USB or create a new virtual device - If by USB, debugging must be enabled on the device --- ## Cloned Doodle Upload a screenshot of your Android Studio opened with your cloned Doodle Repo - Question 3 of Ed Lesson --- ## Android Project Structure .center[ 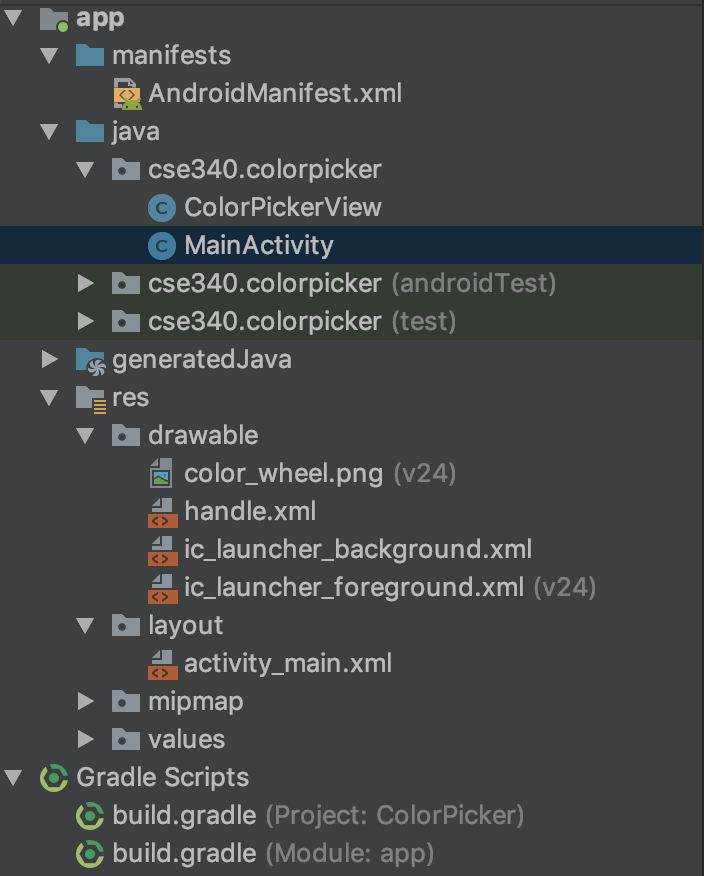 ] --- ## Android Project Structure `app/src/main/AndroidManifest.xml` - Describes application at a high level for both compilation and running - Includes app package, components, permissions, and hardware features `app/build.gradle` - Android build tool configuration file - Includes target and min SDK versions and dependencies `app/src/main/res/layout/activity_main.xml` - Declares the views and layout of the main activity --- ## Android Project Structure `app/src/main/java/com.example.myapp/MainActivity.java` - The main activity activated when application launches `layout/` - Layout XML description files for application activities `mipmap/` - Launcher icons for your application at various sizes and shapes --- ## Android Project Structure `drawable-
/` - Images at various pixel densities to accommodate various display resolutions - Keep filenames consistent between folders `values/` - A good place to store constants - Density-independent pixel values (sizes) - Color - Good for string localization as well - Storing strings here makes it much easier to add additional languages in the future! --- ## Android Structure Attempt Question 4 on the Ed Lesson for Section 1 __Question 4__ - In which folder would you store images needed for your application? What about icons? --- # Android Studio Tips - [Keyboard shortcuts](https://developer.android.com/studio/intro/keyboard-shortcuts) - We will demo going over structure tab, layout inspector --- ## Resources Vogella Tutorials - [Android Animation](http://www.vogella.com/tutorials/AndroidAnimation/article.html) Android Developer Tutorials - [View Animation](https://developer.android.com/guide/topics/graphics/view-animation.html) - [Property Animation](https://developer.android.com/guide/topics/graphics/prop-animation.html) Relevant supplemental material is provided on the course website - Listed in the schedule - This week's supplemental material reviews Java, covers Android animation