# [CSE 340](/courses/cse340/23wi/schedule.html) Lab 1: Doodle Winter 23 ## Introduction to Doodle .title-slide-logo[ 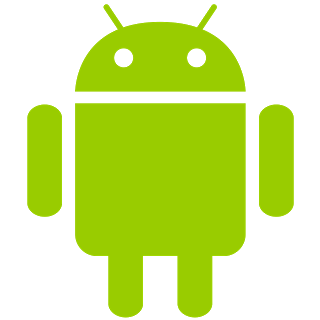 ] --- # Doodle Assignment .left-column50[
classDiagram Activity <|-- AbstractMainActivity AbstractMainActivity <|-- Part1Activity AbstractMainActivity <|-- Part2Activity AbstractMainActivity <|-- Part3Activity ImageView <|-- DrawView DrawView <|-- CircleView DrawView <|-- TextView DrawView <|-- LineView class DrawView { +getBrush() #initFromParentCoords() #onDraw() } class AbstractMainActivity { #PHONE_DIMS +onCreate() *doodle() }
] .right-column50[ - `DrawView` can draw on screen (and be moved around). You will implement part of it. It inherits from [View](https://developer.android.com/reference/android/view/View). - You will implement subclasses `CircleView`, `TextView` and `LineView`. - AbstractMainActivity (which you don't edit) *inherits from* [Activity](https://developer.android.com/reference/android/app/Activity). ] ??? Green: Android toolkit Blue: Classes we provide you don't have to modify Yellow: Classes you implement Remind students that they did do inheritance in 142/143 like with Critters. --- # Implementing `initFromParentCoords` ```java initFromParentCoords(float parentX, float parentY) ``` ### Params: - `parentX`, `parentY`: This is the position your view should be drawn at *in parent coordinates* - What is this in child coordinates? When you're drawing in onDraw()... you'll need to draw from **child coordinates**. ??? answer is (0,0) help them see why! --- # Implementing `CircleView` Circle is specified using its center (provided in *parent coordinates*) and radius. How do you translate this to child coordinates? How do you actually draw the circle? It is drawn in *child coordinates* --- # Implementing `LineView` .left-column[ 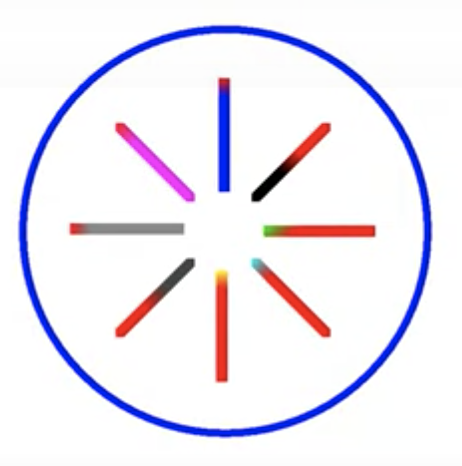 ] .right-column[Lines are specified using a start and end point. They can be drawn in any direction. But we need to position the child's *top left* corner. No matter which line. It is ok if the diagonal lines' ends look "cut off", as seen in the spec and the picture on the left. ] --- # Implementing `LineView` - Examine the Direction.java file - Calculate which Direction each endpoint is in. This uses the Direction enums we gave you. - If a line is going from parent coordinates (0, 0) to (15, 100)... then where are the start and end points according to Direction? --- # Android Debugging Tips Resource: [https://developer.android.com/studio/debug](https://developer.android.com/studio/debug) --- layout:none # Px vs. dp - Always use dp (density independent pixels) when specifying the physical size of UI elements - Why not use pixels? - Different screen sizes have different number of pixels and therefore different pixel densities - So if you use pixels to define UI element sizes, they will be inconsistent between screens with different pixel densities .grid[ 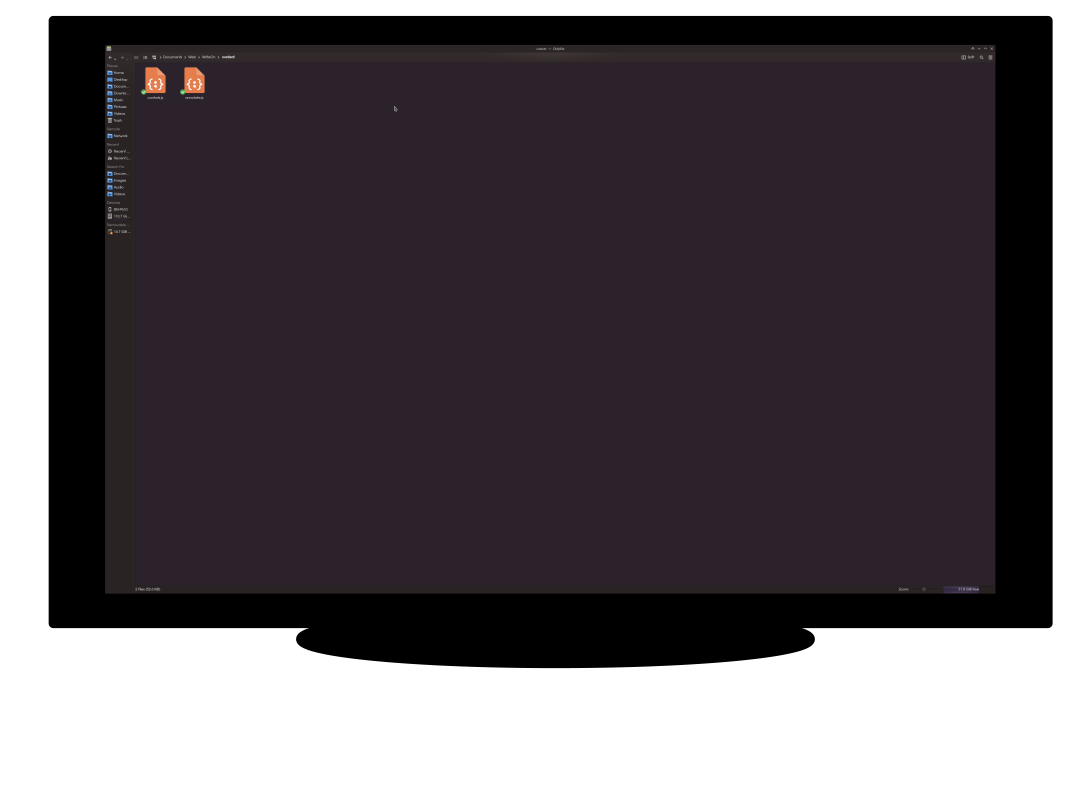 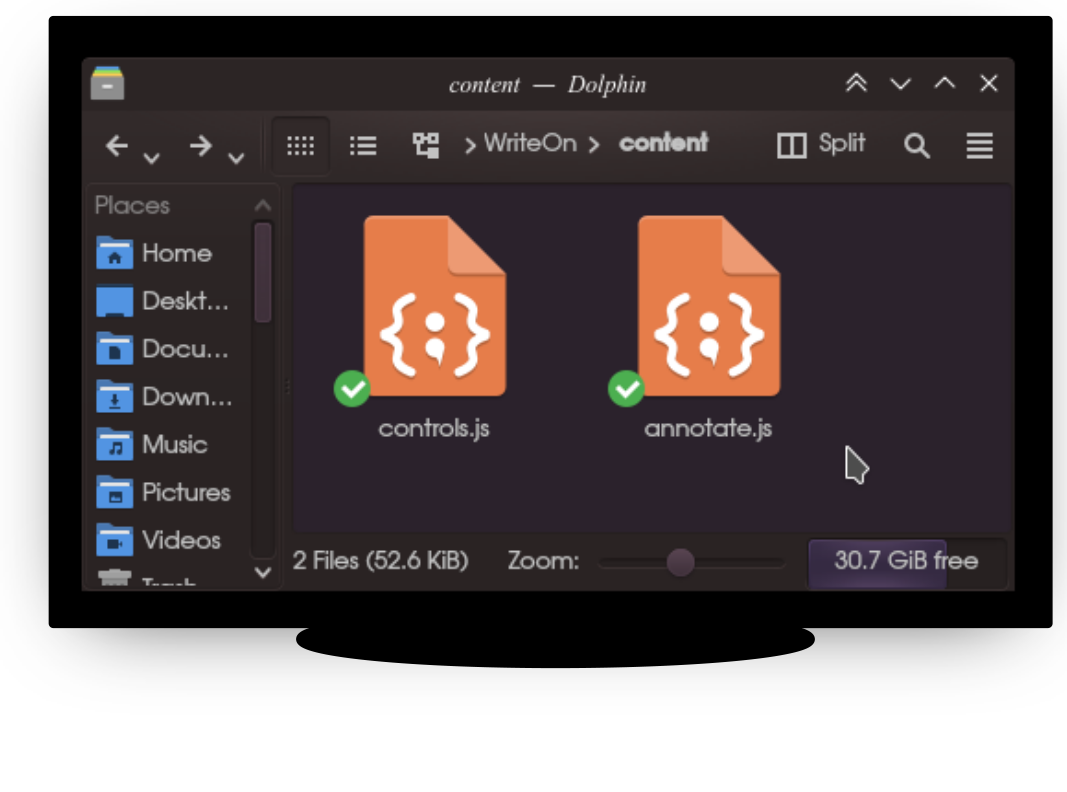 ] --- layout:none # Px vs. dp - Dp are flexible units that scale to have uniform dimensions on any screen. - They provide a flexible way to accommodate a design across platforms. - A `dp` is equal to one physical pixel on a screen with a density of 160 pixels per inch - We provide a class that helps you with translation in Doodle: `DimHelp` - For more info see [Support different pixel densities](https://developer.android.com/training/multiscreen/screendensities).