# [CSE 340](/courses/cse340/22wi/schedule.html) Lab 5 Winter 2022 ## Week 6: Event Handling .title-slide-logo[ 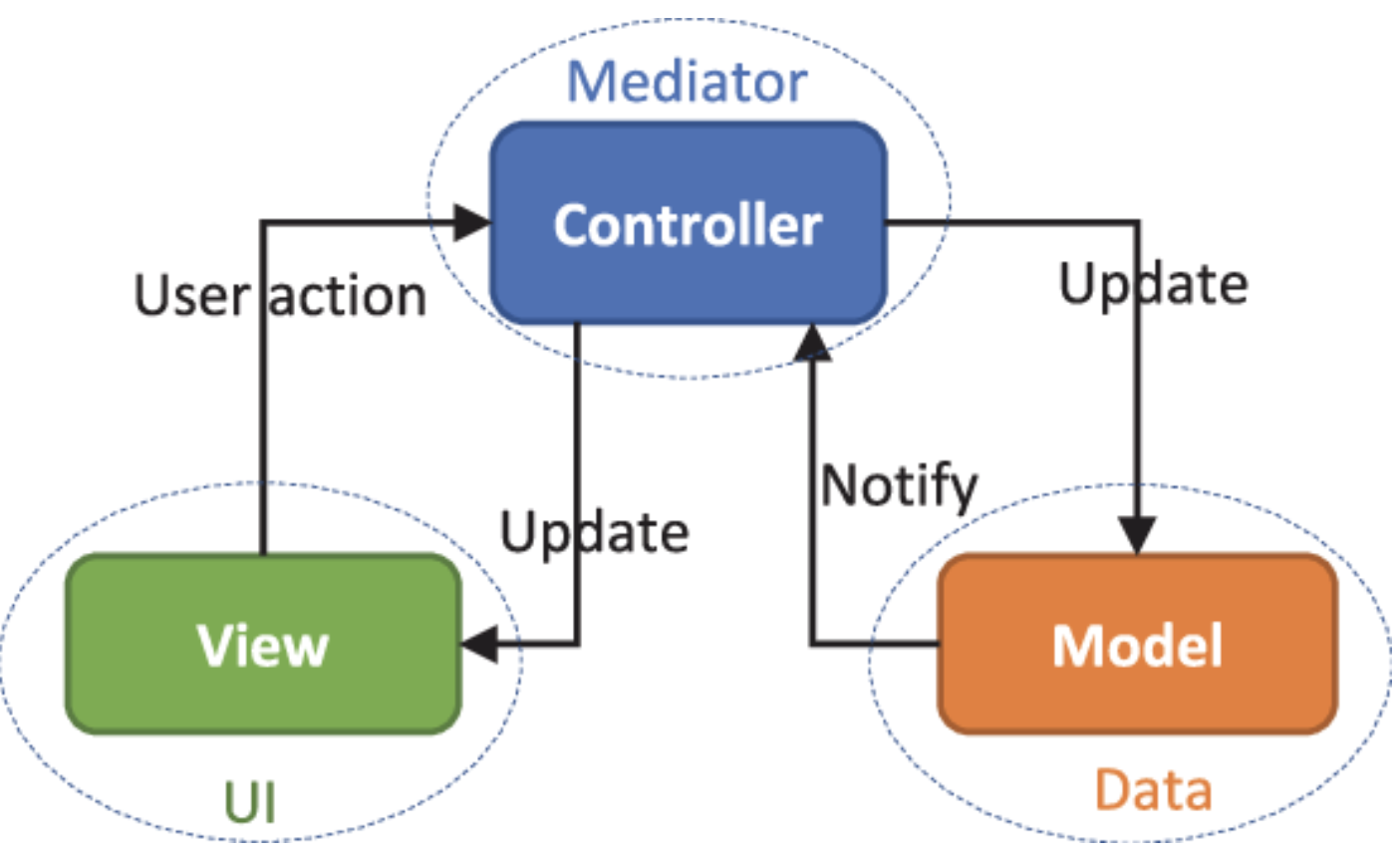 ] --- # Timeline - **Accessibility**: Out: W 26-Jan, Due: Th 3-Feb, Lock: Sa 5-Feb - **Menus**: Out: F 4-Feb, Code -> (Checkpoint: M 7-Feb, Due: Th 10-Feb, Lock: Sa 12-Feb). Testing/Report -> (Due: M 14-Feb, Lock: T 15-Feb). --- # Section 5 Objectives - [Model View Controller](#4) - [Reviewing Event Record](#8) - Section Exercise #1: [Event Record](#15) - [Callbacks and listeners](#16) - Preview of New Content: - [State Machine and PPS](#18) - Section Exercise #2: [PPS](#21) - Preview: [Menus User Study](#24) --- # Model View Controller Review - What: [Architectural pattern](https://en.wikipedia.org/wiki/Software_design_pattern) used for developing UI - App may be organized into 3 separate major logical components - Why: [Bridge the gap](https://folk.universitetetioslo.no/trygver/themes/mvc/mvc-index.html) between the user's mental model and the actual model in the program. - Way to organize code --- # MVC: 3 parts 1. **Model** = container of pure data - ex: List of Strings describing image alt text 2. **View** = displays the model's data to user, the UI - Layouts, representation of data - ex: XML layout resources 3. **Controller** = mediates between View and Model - Handles event listening/input - Manipulates data within the model, updates the View - ex: Android Java Activities --- # MVC Review [The Calculator App, again](https://gitlab.cs.washington.edu/cse340/exercises/mvc-calculator/-/tree/section5-mvc) - Can you identify which parts of the app count as the Model/View/Controller? --- # MVC Review Example answers: - **Model: CalculatorModel -> holds pure data the Calculator should store** - final calculation result - intermediate result (user has the option to edit their equation before submitting) - CalculatorModel extends Observable (some data to be watched), calls setChanged() and notifyObservers() - **View: activity_main.xml, etc** - Calculator buttons... etc - **Controller: Calculator.kt** - Handles communication between View (display) and Model (data) - Calculator extends Observer (listens for changes in the model), override update() --- # Event Record Review (1/5) Event Record: theoretical plan to capture information about a UI event - What (event type) - Where (input target) - When (time) - Value (XY location, which key, mouse coordinates...etc) - Context (Modifiers: CTRL, ALT... etc) --- # Event Record Review (2/5) **Example UI Event 1: User double taps to like a post on Instagram.** - If we were to represent this using an Event Record what would the record contain in terms of each category of recorded information? - Give hypothetical examples --- # Event Record Review (3/5) **UI Event 1: User double taps to like a post on Instagram, example answers** - What: Touch - Where: Image (probably a sub-component inside a “Post” object) - When: 2021-02-04-1234 (absolute time) - maybe even the time passed since the user opened the app or performed some action! - Value: XY location of tap - Context: Double-Tap --- # Event Record Review (4/5) **Example UI Event 2: On a smartphone, user presses the volume up button** - If we were to represent this using an Event Record what would the record contain in terms of each category of recorded information? --- # Event Record Review (5/5) **UI Event 2: Press volume up button** - What: Key down - Where: Physical volume button on phone - When: Absolute time (depending on what info you want to store) - Value: Volume level, duration of press... etc - Context: Depending on the phone, pressing the power button and volume up button at the same time may trigger a screenshot or soft reset --- # Key Points of Event Record (1/2) - Where: - **Part of the system that is going to receive the event.** - Ex: 'Hey Google' -> subsystem in the OS that takes in voice commands. - Value: - **How you can measure the input.** - Not the same as 'What' in the Event Record. - Ex: 'Hey Google' -> Command you are saying after prompting 'Hey Google', like "what's the weather in Seattle?" --- # Key Points of Event Record (2/2) - Context: Modifiers - **Things that change the meaning/nature of the event.** - Ex: Pressing the volume button is an event... context of turning up the volume or in the context of taking a screenshot? - Pressing the power button alongside the volume would modify the context of this event. --- # Section Exercise #1 ([Turn-in](https://edstem.org/us/courses/16206/lessons/26130/slides/152244)) - Come up with your own event record and list its what, where, when, value, and context. --- # Callback and Listeners - **Callback**: method gets called by the Android framework when event happens and is delivered to a component that is registered to listen for the event - Ex: animal enclosure example in lecture --> makeNoise() - **Event Listeners**: type of event handling callback - Example Higher level events (already provided for you by Android View, based on Touch Events) - onClickListener, onFocusListener, onDragListener... - Override these to modify what happens in response to such events Can also define [custom listeners](https://guides.codepath.com/android/Creating-Custom-Listeners) that are triggered based on how you define it (ex: trigger upon HTTP GET request is successful and data loaded) --- # onClick vs onTouch - [onTouchListener](https://developer.android.com/reference/android/view/View.OnTouchListener?authuser=1) = allows you to control what happens in terms of different MotionEvents - Ex: ACTION_UP, ACTION_DOWN, ACTION_MOVE.. And many more! - [onClickListener](https://developer.android.com/reference/android/view/View.OnClickListener) = for when user clicks the view - used for a more basic purpose - cannot handle MotionEvents like onTouch - Android docs: Handling a click event of a Button [programmatically](https://developer.android.com/guide/topics/ui/controls/button#ClickListener) --- # [State Machines](https://en.wikipedia.org/wiki/Finite-state_machine) 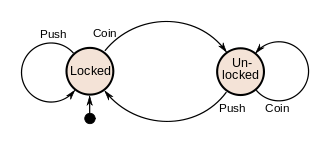 - Respond to incoming events and allows for us to store state between events. - Start state - indicated with incoming arrow - End state - indicated with double-layered shape - Transition States - indicated with single-layered shape - Event Arrows - indicated with an arrow between states, represent different actions taken up states. --- exclude: true # [Propositional Production System](https://tinyurl.com/14hzax1e) .left-column[
stateDiagram-v2 [*] --> S1: DOWN S1 --> S1: MOVE S1 --> [*]: UP
] --- # [Propositional Production System](https://tinyurl.com/14hzax1e) - **Formal Definition: consists of a "state space definition + set of rules"** - PPS = “superset of state machines” and is “formally equivalent to FSMs”. - PPS is specifically referring to user interface systems. - PPS = a state machine with: - ? (Boolean gates) - action calls - extra conditions required to fire - Ex: MOVE/DOWN ? display_pause() could mean: "If Move event was received and direction = Down, show a pause button" --- # State Machine Practice .left-column50[
stateDiagram-v2 [*] --> S1: PLACE_FINGER S1 --> S2: MOVE/DOWN?
display_pause() S1 --> S3: MOVE/UP?
display_play() S1 --> [*]: LIFT_FINGER/
nothing() S3 --> [*]: LIFT_FINGER/
play_music() S2 --> [*]: LIFT_FINGER/
pause_music()
] .right-column50[ Discussion: What is the behavior of this State Machine/PPS? ] --- # Section Exercise #2: PPS (No Turn-in) - Link: https://tinyurl.com/3m78mz8b --- # Worksheet Solutions Problem 1: 1. updateThumbPosition() 2. updateVolume() 3. INSIDE 4. EssentialGeometry.BAR 5. updateThumbPosition() 6. updateVolume() 7. State.START 8. updateThumbAlpha() [How to turn the PPS into code 1](/courses/cse340/22wi/docs/pps.html) and [How to turn the PPS into code 2](/courses/cse340/22wi/docs/ppsscroll.html) --- # Worksheet Solutions Problem 2: .left-column50[
stateDiagram-v2 [*] -->Pressed: DOWN/
InsideBar?A [*] --> Pressed: DOWN/
InsideButton?B Pressed --> [*]: UP/C Pressed --> Pressed: MOVE/D
] .right-column50[ Where - A is `updateButtonPosition(); updateButtonAlpha(); invokeScrollAction(); invalidate();` - B is `updateButtonAlpha(); invokeScrollAction(); invalidate();` - C is `updateButtonAlpha(); invalidate();` - D is `updateButtonPosition(); invokeScrollAction(); invalidate();` ] --- # Looking Ahead: Menus User Study You will be conducting a [user study](https://www.interaction-design.org/literature/topics/user-research). - Carefully review the implementation portion of the spec. - Test your app and make sure there are no unexpected/runtime errors. - Find 3 adult consenting participants (can be other students, TAs, family, etc) - If contacting a TA, please post on Ed and give us time to respond before the deadline.