name: inverse layout: true class: center, middle, inverse --- # Implementing Sensing Lauren Bricker CSE 340 Spring 2022 --- layout: false [//]: # (Outline Slide) # Today's Agenda - Administrivia - Undo due Fri 20-May - Finish [Sensing and Reacting to the User](/courses/cse340/22sp/slides/wk08/ml.html#45) - Sensing in practice - Review sensing and location - Implement sensing --- .left-column-half[ ## Types of Sensors - Review | | | | |--|--|--| | Clicks | Key presses | Touch | | Microphone | Camera | IOT devices | |Accelerometer | Rotation | Screen| |Applications | Location | Telephony| |Battery | Magnetometer | Temperature| |Bluetooth | Network Usage | Traffic| |Calls | Orientation | WiFi| |Messaging | Pressure | Processor| |Gravity | Proximity | Humidity | |Gyroscope | Light | Multi-touch | | ... | ... | ....| ] .right-column-half[
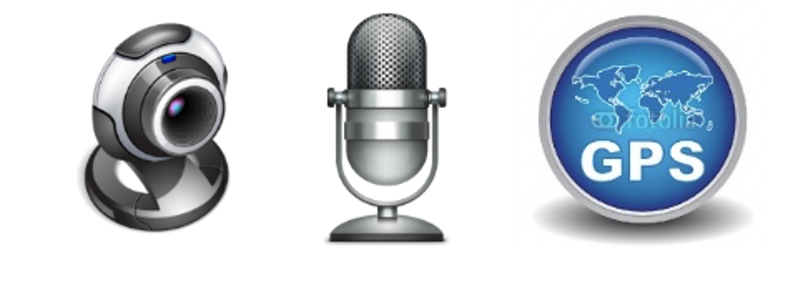 ] --- # Sensing: Categories of Sensors * Motion Sensors * Measure acceleration forces and rotational forces along three axes * Includes accelerometers, gravity sensors, gyroscopes, and rotational vector sensor * Accelerometers and gyroscope are generally HW based, Gravity, linear acceleration, rotation vector, significant motion, step counter, and step detector may be HW or SW based * Environmental Sensors * Measures relative ambient humidity, illuminance, ambient pressure, and ambient temperature * All four sensors are HW based * Position Sensors * Determine the physical position of the device. * Includes orientation, magnetometers, and proximity sensors * Geomagnetic field sensor and proximity sensor are HW based --- # Sensing: Android Sensor Framework `SensorManager` - Provides methods for accessing and listing sensors, registering and unregistering sensor event listeners. `Sensor` - A class to create an instance of a specific sensor. Can also be used to find a sensors abilities `SensorEvent` - Contains information about a specific event from a sensor. Includes the following information: the raw sensor data, the type of sensor that generated the event, the accuracy of the data, and the timestamp for the event. `SensorEventListener` - Used to create callback methods to receive notifications about sensor events. .footnote[[Sensor Framework](https://developer.android.com/guide/topics/sensors/sensors_overview#sensor-framework)] --- # Sensor Activity The following slides are related to the [Sensing and Location Activity](https://gitlab.cs.washington.edu/cse340/exercises/cse340-sensing-and-location) that was introduced in Week 8 Lab Clone this repo if you have not already. --- # Sensor Activity: SensorManager Getting the `SensorManager` ```java SensorManager mSensorManager = (SensorManager) getSystemService(Context.SENSOR_SERVICE); ``` Getting the list of all `Sensor`s from the manager ```java mSensorManager.getSensorList(Sensor.TYPE_ALL); ``` Getting a `Sensor` from the `SensorManager`. The `Sensor` type is a [class constant](https://developer.android.com/guide/topics/sensors/sensors_overview#sensors-intro) in `Sensor` ```java Sensor sensor = mSensorManager.getDefaultSensor(/* type of sensor */); ``` --- # Sensor Activity: Sensor listeners Create a `SensorEventListener` for use with the callback. For example: ```java public class SensorActivity extends AbstractMainActivity implements SensorEventListener { public void onSensorChanged(SensorEvent event) { ... switch (event.sensor.getType()) { case Sensor.TYPE_AMBIENT_TEMPERATURE: ... case Sensor.TYPE_LINEAR_ACCELERATION: ... } } public void onAccuracyChanged(Sensor sensor, int accuracy) { // if Used } } ``` You could use any of the ways to create a callback discussed earlier in this class. --- Registering the sensor. Done in `onResume` generally ```java Sensor sensor = mSensorManager.getDefaultSensor(/* type of sensor */); mSensorManager.registerListener(this, sensor, DELAY); ``` Make sure to unregister the listener in `onPause` so you're not working in the background (unless that's the expected behavior) ```java mSensorManager.unregisterListener(this); ``` --- # End of Deck