# [CSE 340](/courses/cse340/22sp/schedule.html) Lab 1 Spring 2022 ## Week 1: Getting Started With Android Development .title-slide-logo[ 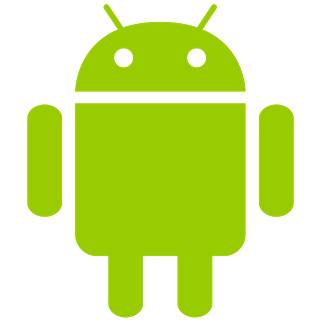 ] --- # Doodle Timeline - Doodle starter code out Wed 30-Mar (yesterday) - Doodle code, reflection, and video due Fri 8-Apr @ 10pm - Doodle peer evals will be distributed on Canvas, Fri 8-Apr - Peer evals due Sun 10-Apr @ 10pm --- ## Reminder!! - Optional Java Refresher Lab today at 4pm [Zoom link](https://washington.zoom.us/j/96930368150) --- ## Course Objectives - Practical applications for learnings in lecture - Understand design considerations when making mobile apps - Design and implement basic Android applications - Debugging in Java and for Android --- ## Lab 1 Objectives - Ice breaker activity - Introduce the building blocks of Android applications - Explore an Android project folder - Getting the first part of the Doodle started - Review section exercise - Show some basic Android debugging techniques --- ## Ice Breaker: 2 Truths and a Lie Answer these 3 questions (Make one of them a lie...) 1. What’s your most interesting injury? 2. When was the last time you traveled outside your immediate area, and where was it? 3. What’s your favorite game (video, board, party, outdoors, whatever), and why? --- ## Android Applications __Components__ - Activity - Service - Broadcast Receiver - Content Providers __Intents__ - Launch components (and provide them with data) - Not limited to components of the same app - Meaning: your components can be started by intents from other applications --- ## Activity [`android.app.Activity`](https://developer.android.com/reference/android/app/Activity) - Represents a single interface - Example: Activities for Gmail App - Inbox, Message Compose, Spam Folder, etc. - Activities can be started by other applications, if desired - E.g., sharing a photo by email starts Gmail's Message Compose activity --- ## Activity .center[ 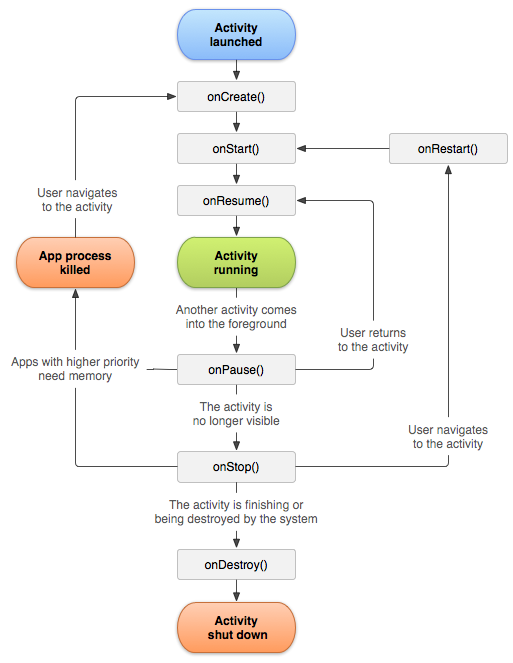 ] --- ## Service [`android.app.Service`](https://developer.android.com/reference/android/app/Service) - Entry point for background processes - Example: Spotify's player activity uses a service to play music - Could be done by activity alone - Use of a service allows music to continue when player activity is no longer in the foreground - Services may be killed and restarted as required by the OS - A persistent service such as music playback may use a persistent notification to prevent this --- ## Broadcast Receivers [`android.content.BroadcastReceiver`](https://developer.android.com/reference/android/content/BroadcastReceiver.html) - Entry point for events outside of regular userflow - Example: Alarms - Application schedules callback with OS in 10 minutes - In the meantime, the application can be killed - 10 minutes later, the OS broadcasts the callback event to the app - App begins playing sound; vibrating --- ## Content Providers [`android.content.ContentProvider`](https://developer.android.com/reference/android/content/ContentProvider) - Persistent storage of data - Supports multiple backends, depending on app permissions - Local filesystem, web, SQLite DB, etc. - Can permit access to other applications - Contacts can be read and written to by other apps, depending on permissions --- ## Android Project Structure .center[ 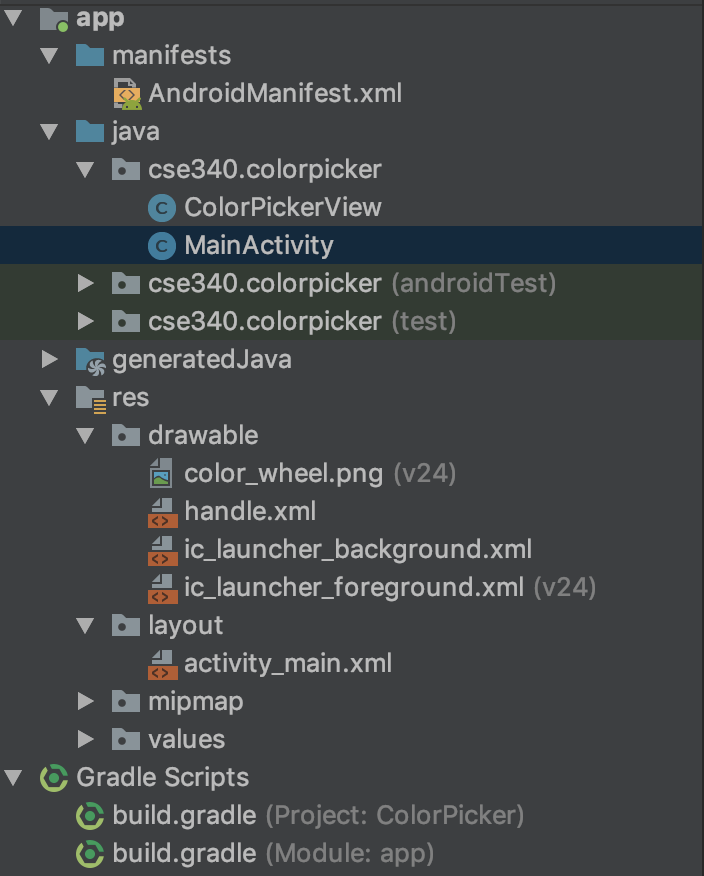 ] --- ## Android Project Structure `app/src/main/AndroidManifest.xml` - Describes application at a high level for both compilation and running - Includes app package, components, permissions, and hardware features `app/build.gradle` - Android build tool configuration file - Includes target and min SDK versions and dependencies `app/src/main/res/layout/activity_main.xml` - Declares the views and layout of the main activity --- ## Android Project Structure `app/src/main/java/com.example.myapp/MainActivity.java` - The main activity activated when application launches `layout/` - Layout XML description files for application activities `mipmap/` - Launcher icons for your application at various sizes and shapes --- ## Android Project Structure `drawable-
/` - Images at various pixel densities to accommodate various display resolutions - Keep filenames consistent between folders `values/` - A good place to store constants - Density-independent pixel values (sizes) - Color - Good for string localization as well - Storing strings here makes it much easier to add additional languages in the future! --- # Android Studio Tips - [Keyboard shortcuts](https://developer.android.com/studio/intro/keyboard-shortcuts) - Demo going over structure tab, layout inspector --- ## Resources Vogella Tutorials - [Android Animation](http://www.vogella.com/tutorials/AndroidAnimation/article.html) Android Developer Tutorials - [View Animation](https://developer.android.com/guide/topics/graphics/view-animation.html) - [Property Animation](https://developer.android.com/guide/topics/graphics/prop-animation.html) Relevant supplemental material is provided on the course website - Listed in the schedule - This week's supplemental material reviews Java, covers Android animation