name: inverse layout: true class: center, middle, inverse --- # Quiz Review & Questions Jennifer Mankoff CSE 340 Winter 2021 --- name: normal layout: true class: --- # On Tap for today Announcements Review on anything up to 2/9 --- # Meta notes - Remember: this class is about Interaction Programming. Programming Android is the substrate for the discussion. - We try to explicitly state when are discussing theory vs practice. - Subgoal of this class: a transition or bridge to 300 level courses. - Important that you learn how to read less structured specifications - Important that you learn how to search for __usable__ information - Is StackOverflow friend or foe? --- # Second reminder: "effort, participation and altruism" grade about? - What you put into the class - How you help others - Whether you show up If you are skipping the quizzes and lecture, not participating/answering questions in lecture, not answering questions on Ed, and so on you will not do well on this grade. You don't have to do ALL of these but you have to do most of them. Initial grades on this released on canvas this week. If you are below average on something, comment has a note about what you can do more of. --- class: center, middle, inverse # Finishing Monday's content --- layout:false # The Whole Toolkit Architecture Input - Input models (events) - Event dispatch - Event handling (state machine) - Callbacks to application Output - Interactor Hierarchy design & use *(examlet1)* - Drawing models (`onDraw()`) *(examlet1)* - Layout (`onLayout()` or `XML`) *(examlet1)* - **Damage and redraw process** --- .left-column-half[ # Core Toolkit Architecture If damage do - *layout* (may change) - position - size - *redraw* ] -- .right-column-half[ # Component Developer - May need to implement `onMeasure()` and `onLayout()` (if a container) - Will always implement `onDraw()` but *never call it* (call `invalidate()` instead) ] --- # View Update: .red[Damage/Redraw] How does the toolkit know what to redraw? What causes damage? ??? concrete example on next slide --- # View Update: .red[Damage/Redraw] What should be redrawn? 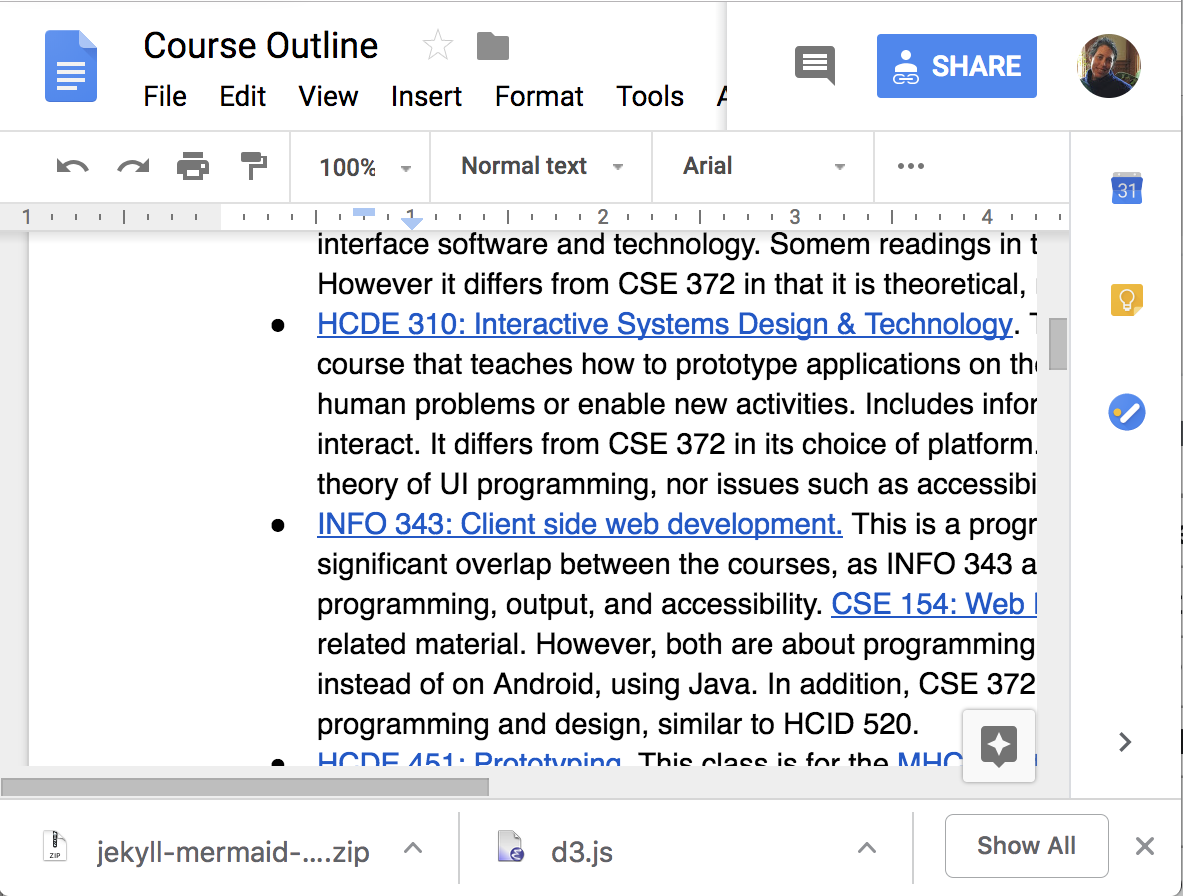 --- # View Update: .red[Damage/Redraw] What should be redrawn? 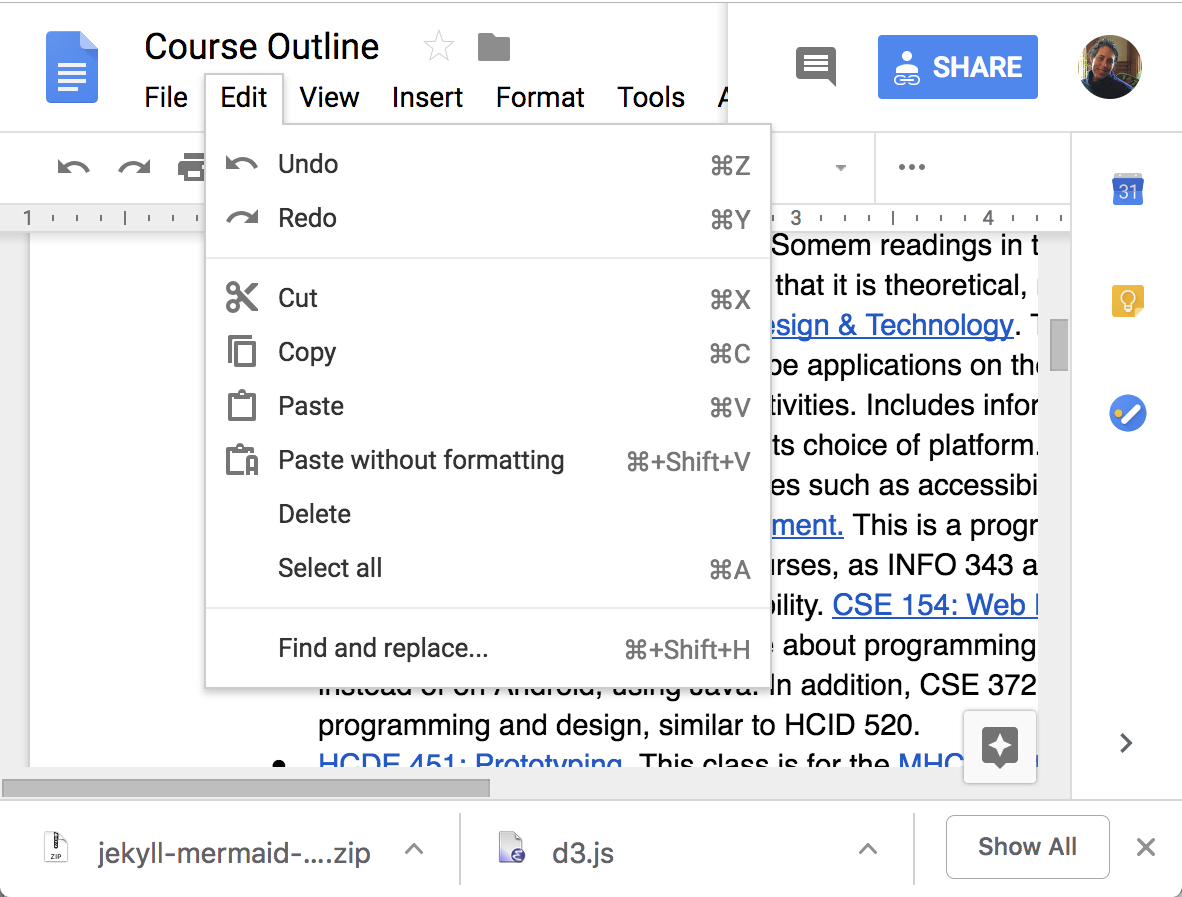 --- # View Update: .red[Damage/Redraw] What should be redrawn? 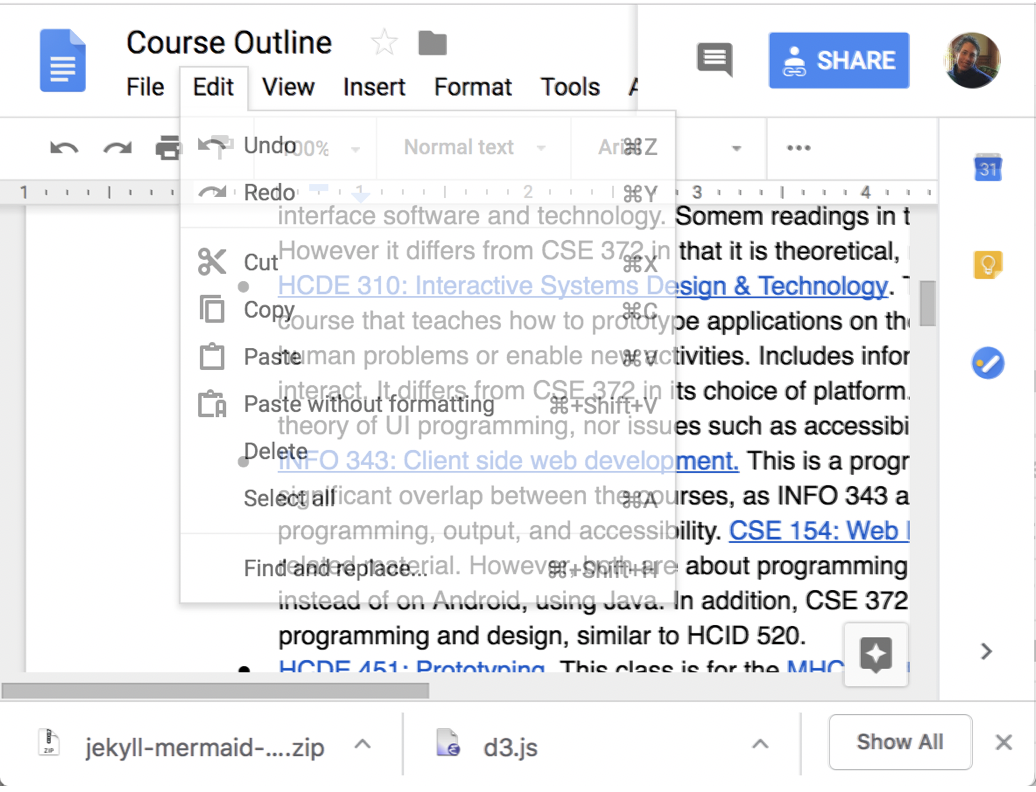 --- # View Update: .red[Damage/Redraw] How does the toolkit know what to redraw? What causes damage? ??? Can you think about other things? - Window hidden and re-exposed - Resizing - redrawing --
Naive approach to redraw --- ## View Update: .red[Damage/Redraw]  ??? XXX TODO ADD pic like this using divs? - Can be slow (redrawing everything unecessary) - Can cause flickering - double buffering is better, - hence the 'Canvas' abstraction or equivalent - can then switch which FB is displayed (very fast) --- ## View Update: .red[Damage/Redraw]   --- ## View Update: .red[Damage/Redraw]   **Never** just draw: Why not? ??? - Update *state* - Report *damage* (by calling 'repaint()) - Wait for *toolkit to request redraw* (also works if damage comes from elsewhere) - How does it generalize to any cause of damage (always need state!!) --- ## View Update: .red[Damage/Redraw] How does the toolkit know what to redraw? - Let the component report: Damage/Redraw invoked by `invalidate()` or equivalent --- ## View Update: .red[Damage/Redraw] How does the toolkit know what to redraw? - Let the component report (`invalidate()`) **NOTE** we are *not* calling *onDraw()* directly (important for your assignment) - Aggregate - Usually calculate bounding box --- ## View Update: .red[Draw/Redraw] Virtual device abstraction provided by windowing system Component abstraction provided by toolkit - Enforced using clipping - Supported using coordinate system transformations Drawing is recursive - Makes it possible for parent to *decorate* kids - Parent responsible for making kids think they are the center of the universe (translate) - Clipping: intersect parent and child, also handled by parent ??? Allows each program to (mostly) pretend that it has the screen (frame buffer) to itself Allows each component to (mostly) pretend that it has the screen to itself --- # Core Toolkit Architecture If damage do - *layout* (may change) - position - size - do *redraw* - Union of damage (any of those can cause it) used to trigger redraw of anything inside that union - Drawing + clipping – standard drawing order, but only for things damaged; clipped to damage region - Clear damage --- layout:false # The Whole Toolkit Architecture Input - Input models (events) - Event dispatch - Event handling (state machine) - **Callbacks to application** Output - Interactor Hierarchy design & use - Drawing models (`onDraw()`) - Layout (`onLayout()` or `XML`) - Damage and redraw process --- # Callbacks You have created a new driving app. You have registered your app to listen for `OffRoute` callbacks (when the driver’s vehicle deviates from the route, `onOffRoute()` will be called). Correctly label each method call, based on what object (`Application` or `View`) you would use to *call it*, and where you would put the code to *implement it*. Please answer the following questions using **Application**, **View**, or **Neither** as your answers. `onOffRoute()` is called by `[appview1]` and implemented in `[appview2]` `addOnOffRouteListener()` is called by `[appview3]` and implemented in `[appview4]` --- # Callbacks - onOffRoute() is called by [appview1] and implemented in [appview2] - addOnOffRouteListener() is called by [appview3] and implemented in [appview4] .left-column50[
sequenceDiagram participant Application participant View participant Driver Application->>View: addOnOffRouteListener(this) Driver->>View: Deviated from road View-->>Application: onOffRoute()
] .right-column50[
classDiagram class OnOffRouteListener { } class View{ #onOffRouteListenerList +addOnOffRouteListener() } class Application { onOffRoute() } OnOffRouteListener <|-- Application : Implements View --> "many" OnOffRouteListener : Contains
] --- https://PollEv.com/jmankoff; text JMANKOFF to 22333 to join the session, then text a response.
--- # What to expect Friday Another round of feedback on class The examlet will open at 12:40 - The expectation is that everyone that is in our timezone (PDT) or close to PDT would take this before the end of class. You should be in communication with me if this is an issue. - You should start right away as you would in a traditional classroom and not wait around. - We will be checking to see that everyone has taken it by 1:20 - You will not need to stay on Zoom to take the assessment. However we will stay in the classroom session in case you have questions about the format etc. - This should be about 30 minutes, but you will have a buffer to ensure that everyone can submit on time. - If you have problems with any of the technology you MUST get in touch with the course staff immediately. - You may use your notes, the website, take a look at your code, etc, but *you may not collaborate with others on the exam*.