name: inverse layout: true class: center, middle, inverse --- # Building your first Android App CSE 340 Winter 2021 Instructor: Jennifer Mankoff
--- layout: false | Hall of Shame(?) | Hall of Fame | |---|---| |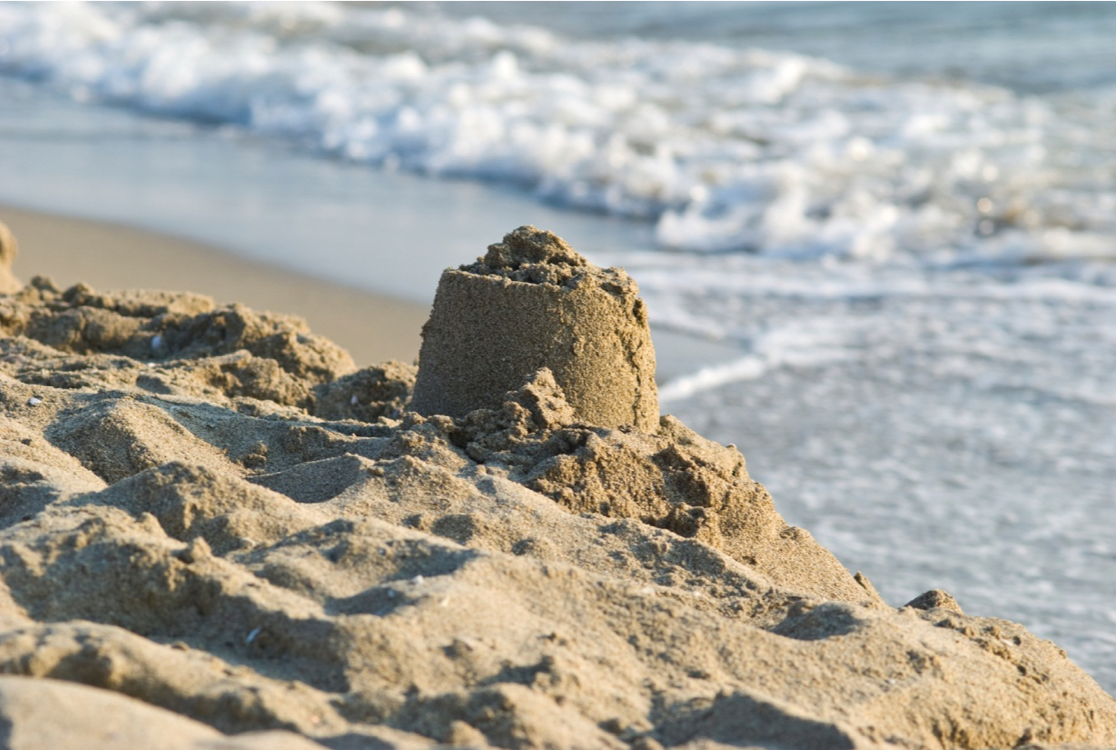 | 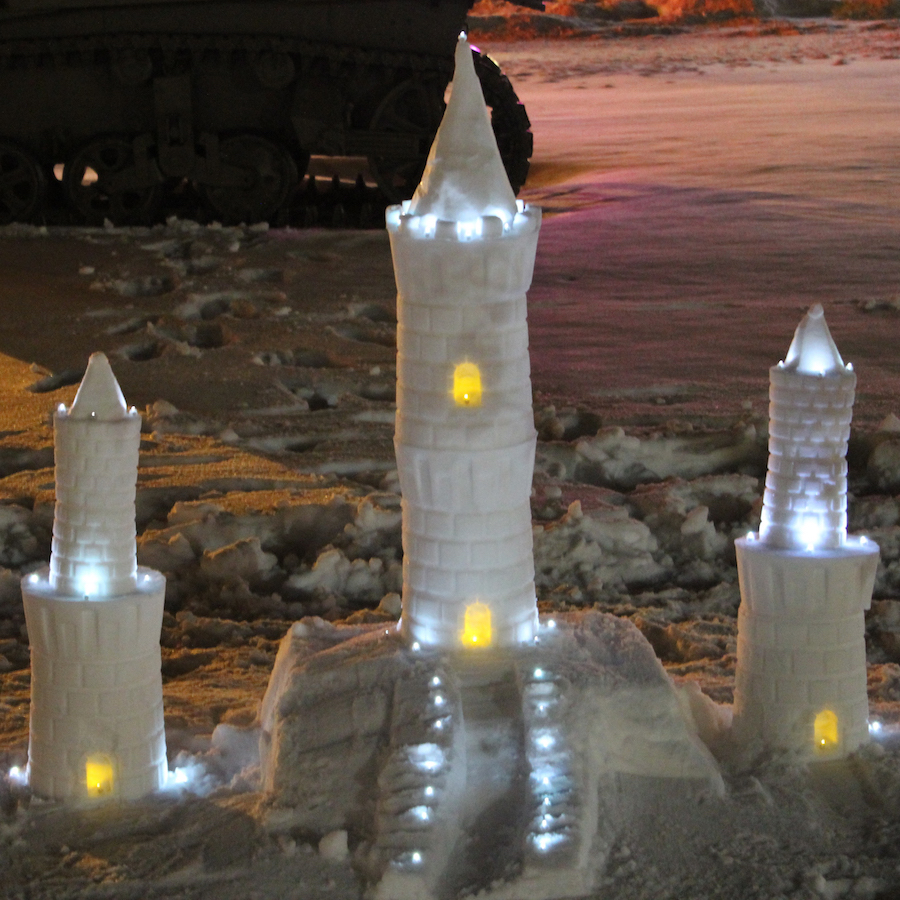 --- 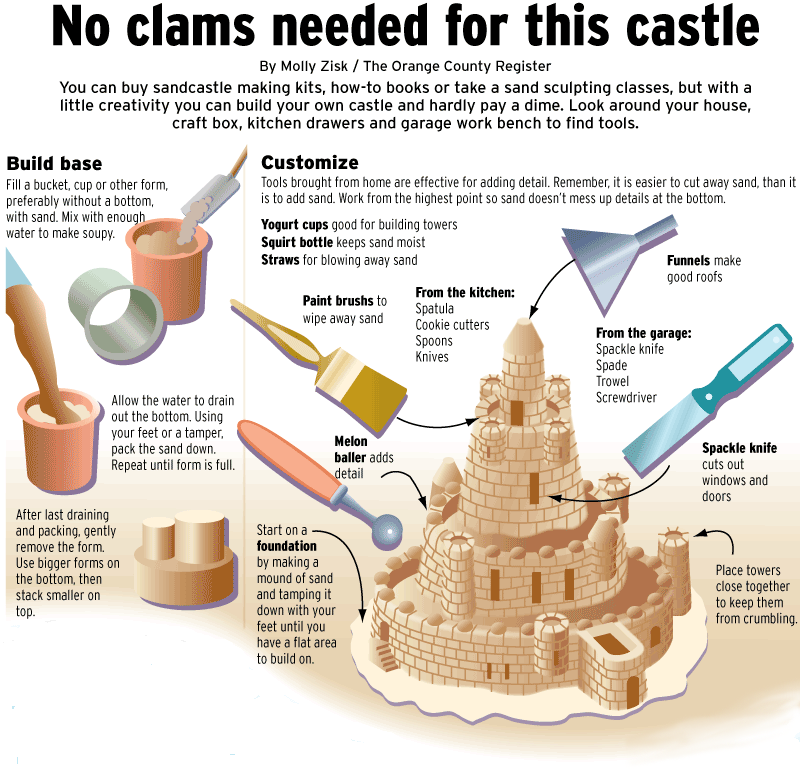 --- # Goals for this week - Build an android app from scratch - Show some text on the screen - Add a button --- # Creating a new app .left-column[ 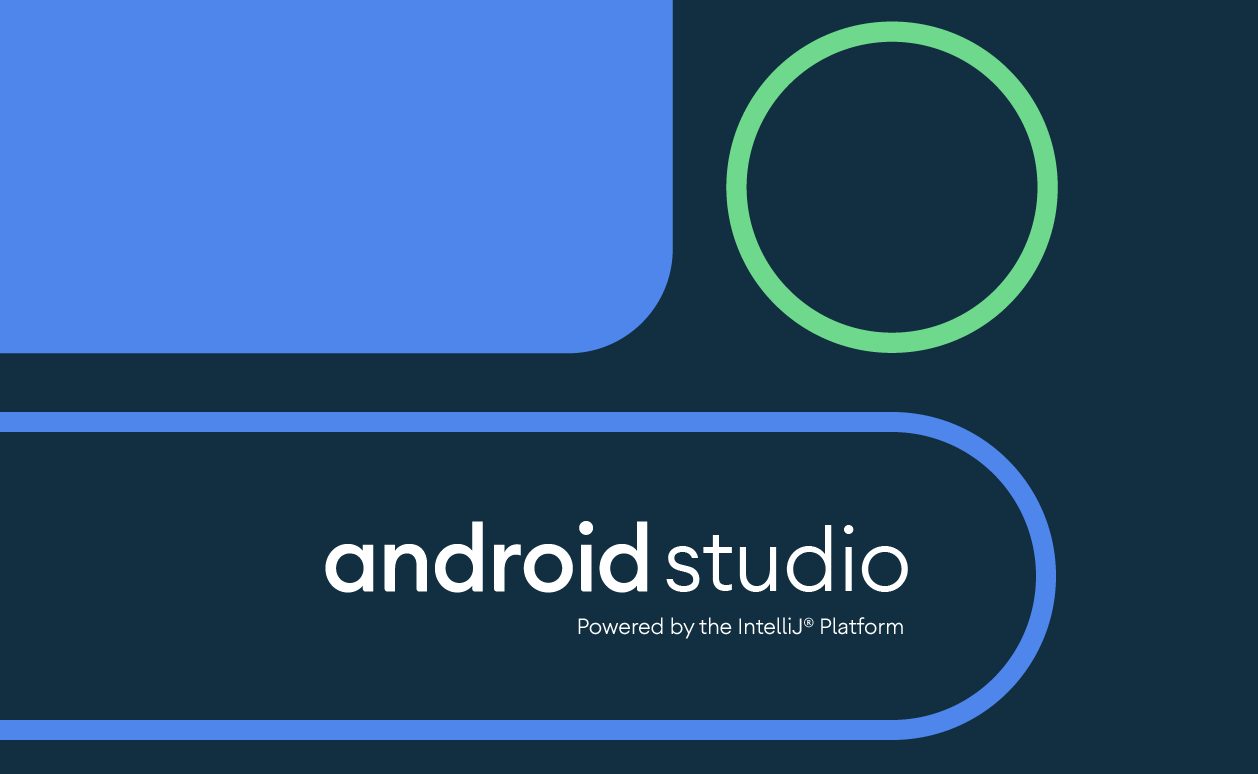 ] .right-column[ Open Android Studio [should be Version 4.0] Select "Start a new Android Studio project" ] --- # What is Android? Most commonly used interface development platform for Java -- - Open source - Around 75% market share - Thousands of supported devices -- - Exposes Android SDK - Framework for building apps on mobile devices -- - Written in __Java__ and E__X__tensible __M__arkup __L__anguage (__XML__) --- # Needed level of Java experience? **Background**: Lots of Java programming expected. Need 143 or equivalent - Comfortable with Java; basic software engineering; some Data Structures - Fast-paced introduction to git & Android Studio IDE - Advanced Java use (e.g. anonymous inner classes) - Must be comfortable with reading documentation (not just Stack Overflow) - We will use trees, state machines, etc. - Math computations (trig) for later assignments --- # Java refresher course The TAs will be hosting a Java refresher that covers most facets of Java we will be using in this class: - Inheritance - Generics - Anonymous Inner Classes - Lambdas (and "::" notation) If these are not topics you're comfortable with (some are not directly covered in any CS class), come join us! Time: 6:30-7:30pm 01/07/21 (upcoming Thursday PST) at [Jay/Zeynep's OH](https://washington.zoom.us/j/97484373235). --- # Start Android Studio! Choose your project type You'll see a number of choices that mention the word "Activity" 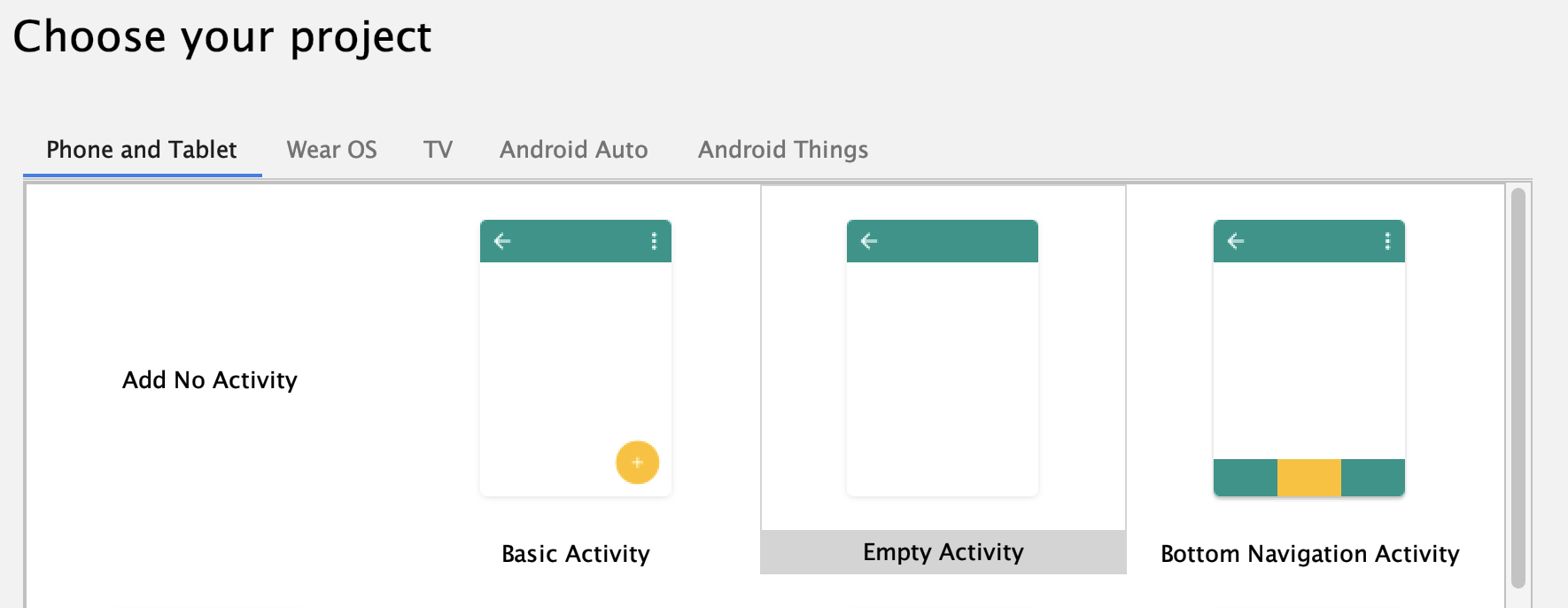 If you need it, Android studio is installed on he VDI remote Windows ugrad PCs at [vdi.cs.washington.edu](vdi.cs.washington.edu) --- ## Activity [`android.app.Activity`](https://developer.android.com/reference/android/app/Activity) - Represents a single interface - Example: Activities for Gmail App - Inbox, Message Compose, Spam Folder, etc. - Activities can be started by other applications, if desired - E.g., sharing a photo by email starts Gmail's Message Compose activity --- ## Activity Lifecycle 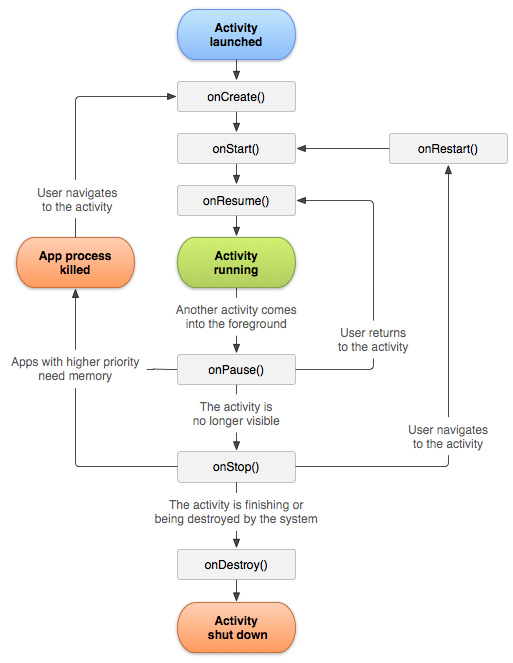 ???
graph TD AL(Activity Launched) --> C["onCreate()"] C --> S["onStart()"] S --> R["onResume()"] R --> RUNNING(Activity Running) RUNNING --> |Another activity foregrounded| P["onPause()"] P --> STOP["onStop()"] STOP --> |Activity finishing/destroyed| D["onDestroy()"] D --> SHUT(Activity shut down) STOP --> |High Priority needs memory| KILL(App process killed) KILL --> |User navigates to activity| C STOP --> |User navigates to activity| RESTART["onRestart()"] RESTART --> S class AL darkblue class RUNNING blue class KILL,SHUT yellow
--- # Let's choose "Empty Activity" - Give it a name (```cse340-aCount```) - A location - Minimum API Level (```Pie```) --- ## What is an API? Application Programming Interface Usually refers to the methods used to access someone else's backend service --- .left-column50[ ## What is a Minimum API Level? 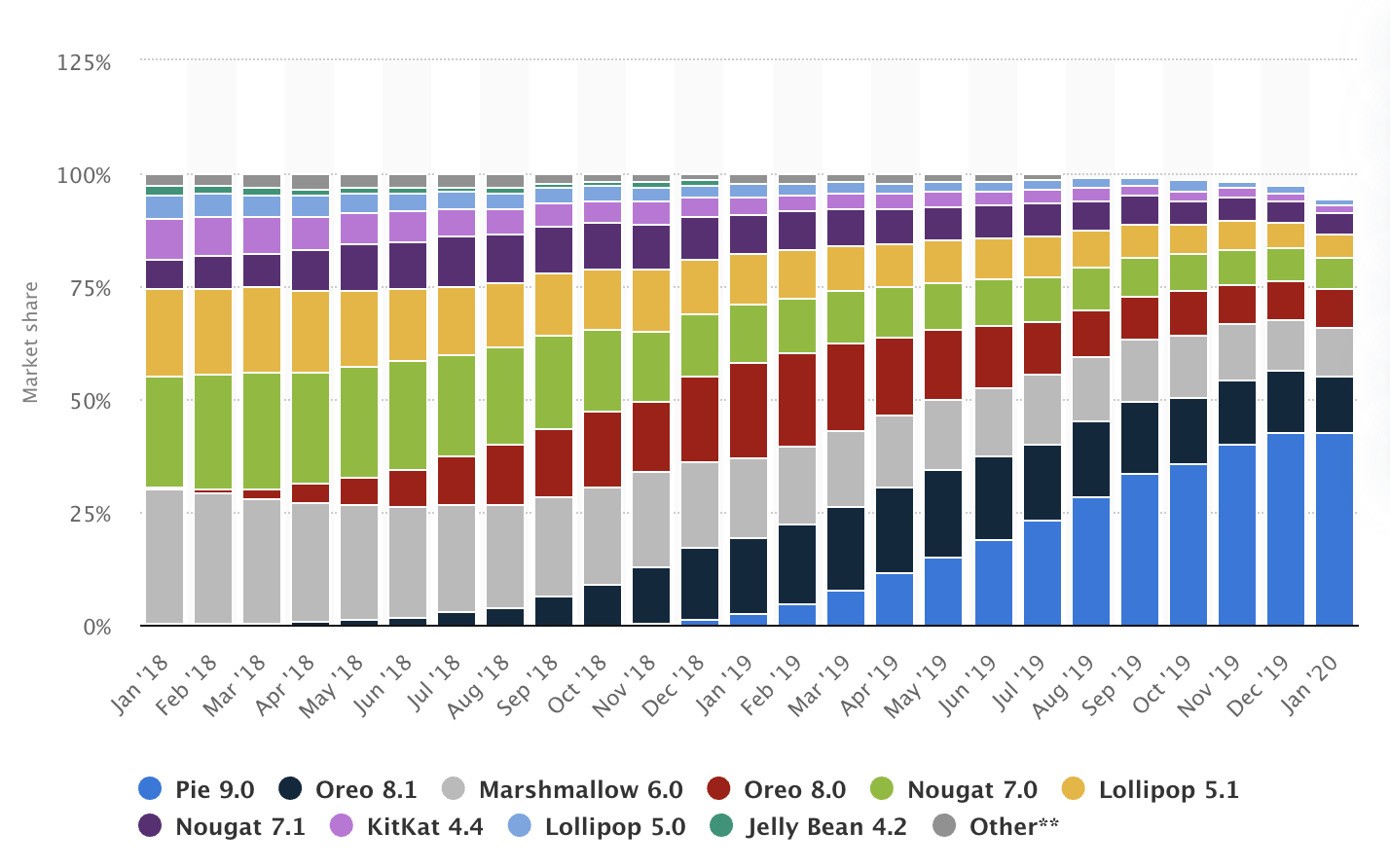 ] .right-column50[ ## We're going to target .red[Pie] ([live stats visualization](https://www.statista.com/statistics/921152/mobile-android-version-share-worldwide/)) ] --- ## What is an API? Application Programming Interface Usually refers to the methods used to access someone else's backend service Android is an API, but it's also a toolkit --- # What is a toolkit? .left-column[
graph LR ap[Application Program] hlt[High Level Tools] t[Toolkit] w[Window System] o[OS] h[Hardware] classDef yellow font-size:14pt,text-align:center classDef green font-size:14pt,text-align:center classDef darkblue font-size:14pt,text-align:center class ap,w,h,o yellow class hlt,t green class t darkblue
] .right-column[ Services a toolkit supports (in the order we will learn them) - Graphics - Animation - Layout - Accessibility - Event Handling - Interactive Components - Undo - Sensing (not covered) - Data (not covered) - databases; apis ] ??? What are some things you expect every interface to have that I didn't mention here? Example: Undo (we will learn about this) Do all user interface toolkits have all of these? --- # What is an Interface Toolkit (Main subject of this course) - Difference between toolkit and IDE (Integrated Development Environment)? - Difference between toolkit and API (Application Programming Interface)? ??? Connect what they learned in 143 (i.e that they used the SDK for Java which had a bunch of libraries that had pre-defined code). The IDE most people used was JGrasp. --- # What is an Interface Toolkit (Main subject of this course) - Difference between toolkit and IDE (Integrated Development Environment)? - IDE helps you at the time you are programming; toolkit is used *by* your program - Difference between toolkit and API (Application Programming Interface)? - API is used *by* your program to access services on demand; toolkit has a runtime architecture that structures what your program does ??? Connect what they learned in 143 (i.e that they used the SDK for Java which had a bunch of libraries that had pre-defined code). The IDE most people used was JGrasp. --- # Your toolkits
--- # What is an Interface Toolkit (Main subject of this course) - IDE helps you at the time you are programming; toolkit is used *by* your program - API is used *by* your program to access services on demand; toolkit has a runtime architecture that structures what your program does Structure of a Toolkit - Library of components - Architecture ??? --- # Toolkit parts .left-column[
graph LR ip[Interface Programmer] w[Components] l[Library] a[Architecture] class ip yellow class w,l,a green
] .right-column[ Let's say you want to build an app - What do we use to build the app? ] --- **Interface Programmers** combine library elements according to toolkit rules & following constraints of architecture; common to all UI toolkits How does the toolkit know what to draw? 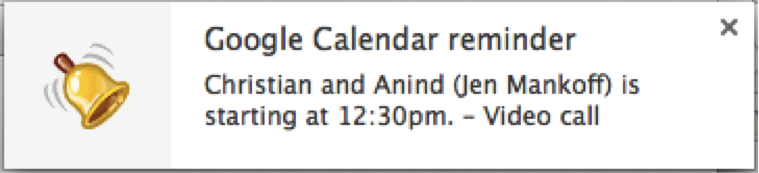 - Deconstruct: Let's list all the components... What do we need to know to draw them? ??? discuss with your neighbor - what to draw; where to draw it --- # Let's run our Android interface  --- # First you need to select a deployment target .left-column50[  Click on "Create new Virtual Device" (or plug your phone in to your computer and select that) ] -- count: false .right-column50[ Pick an existing one, or set up an emulator. - Pixel 2 is a good choice. - Use API level 28 - Make sure it has the play store ] --- # A note: Technology Requirements for the course - Phone will be sent by mail to those who wish (**loan only**) - Fill out the survey tonight if you need - We'll have to figure out what to do if we get too many requests - You need a laptop sufficient memory/disk space to run
Android Studio with emulators - Ask questions if you need more guidance --- # Run your app .left-column[ What does it do? 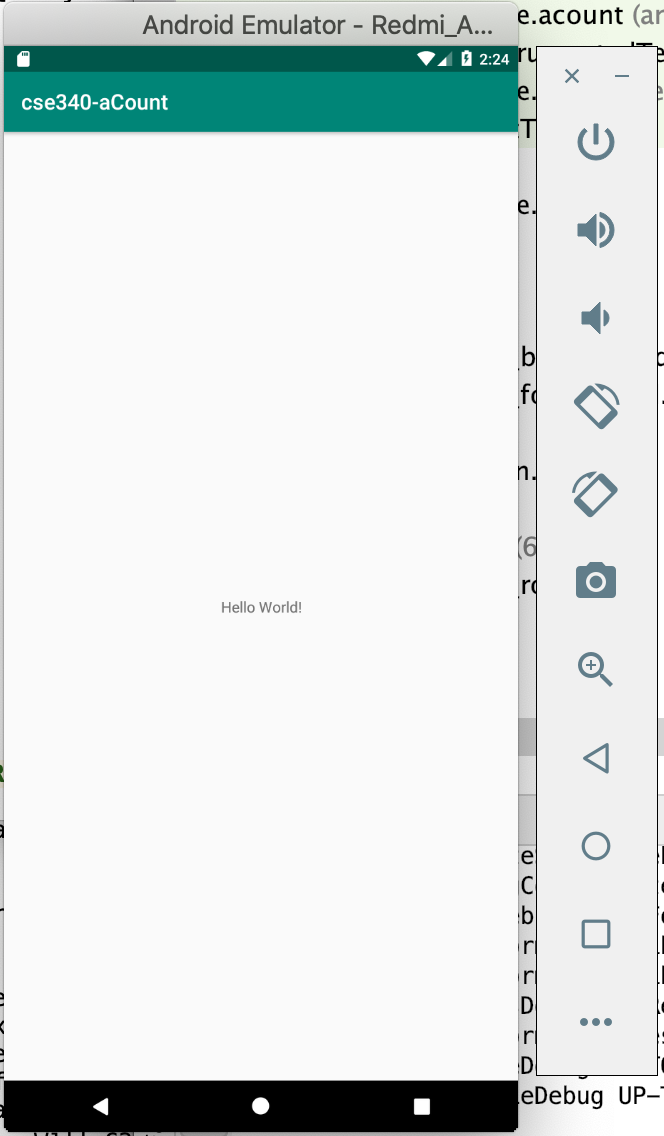 ] .right-column[ Complete the setup of your emulator - Select the deployment target - Select "Next" - Select a System Image ("Pie") - Select "Finish" Run it - Select the new virtual device - Click "Ok" ] --- # Let's peek inside Open `java/cse340.example.acount` --- # These are "static" resources Good programming practice to remove "static" resources from your code - Often used in internationalization or localization - Often used in A/B testing in software development --- # These are "static" resources Good programming practice to remove "static" resources from your code
1
Android resources are stored in `.xml` files in subdirectories of the `app/res` directory may include - `drawable` - images you might draw on the screen in various formats - `layout` - layouts of various screens - `menu` - the menus for your application - `values` - might contain `colors.xml` and `strings.xml` .footnote[
1
Think of this like the "No Magic Numbers" principle you learned early in 142)] --- # Let's explore layout Open `res/layout/activity_main.xml` - Look at the "Design" view - Look at the "Text" view --- # How do we make it more interactive? .left-column[
graph LR ip[Interface Programmer] w[Components] l[Library] a[Architecture] class ip yellow class w,l,a green class l darkblue
] .right-column[ How about we add a button? What is a button? ] --- # How do we make it more interactive? .left-column[
graph LR ip[Interface Programmer] w[Components] l[Library] a[Architecture] class ip yellow class w,l,a green class l darkblue
] .right-column[
] --- # What do we tell the toolkit about the button? .left-column[
graph LR ip[Interface Programmer] w[Components] l[Library] a[Architecture] class ip yellow class w,l,a green class a darkblue
] .right-column[ Where should it go? What should it do? ] --- # First: Where should it go? .left-column50[ Have to do this first so we can even see it This is a "Layout" problem -- something all user interface toolkits have to support. Layout is complicated because we want layout to handle things like resizing or changing from portrait to landscope mode well. ] -- .right-column50[ Specified using a component hierarchy
(a tree)
graph TD W(ConstraintLayout) --> V[Ab TextView--Hello World!] W --> V1[fa:fa-square Button--Next ] class W darkblue class V1,V blue
Android's rendering of the same: 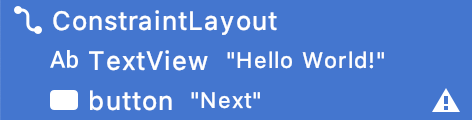 ] --- # Placing the button Set width to ```match_parent``` Set height to ```wrap_content``` Set button text to "Next" ??? live demo if time --- # Look and feel
You might think this looks ugly. - If so, you have some good designerly instincts! - Design is *not* a focus of this class. - However we will discus some design theory --- count: false # Look and feel .red[**Take-home challenge: Can you change the font or font size for the text or the button to be more appealing/better?**] You might think this looks ugly. - If so, you have some good designerly instincts! - Design is *not* a focus of this class. - However we will discus some design theory --- # Second: What should it do? We need to know when the user *interacts* with it - This is called *Event Handling* - To do this we need a *reference* to the button - We will use it's ```android:id``` property (see the XML version of activity_main.xml) -- count: false It is ```@+id/button``` --- # Set up a listener We can do this in ```onCreate``` ```java Button button = (Button) findViewById(R.id.button); button.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { Toast.makeText(getApplicationContext(), "Button Pressed", Toast.LENGTH_LONG).show();//display the text of button } }); ``` --- # Toolkit parts .left-column[
graph LR ip[Interface Programmer] w[Components] l[Library] a[Architecture] class ip yellow class w,l,a green
] .right-column[ So even to build this simple interface, we used Components (a button), other library elements (layout, events, etc), and interacted with the toolkit architecture Building an interface well also requires considering things like accessibility and security. We'll learn how to do this properly in the *Accessibility/Security* assignment. ] --- # Toolkit parts .left-column[
graph LR ip[Interface Programmer] w[Components] l[Library] a[Architecture] class ip yellow class w,l,a green
] .right-column[ But what if something we need is missing? ("Whorfian effects") Our tools influence how we think and thus what we do. Origins of the term "whorfian effects" are linguistic (e.g. the way in which [language about color affects what we perceive](http://www.radiolab.org/story/91730-new-words-new-world/))] --- # Developer Roles .left-column[
graph LR ip[Interface Programmer] w[Component Developer] l[Library Extender] a[Architecture Extender] t[Toolkit Builder] class ip yellow class t,l,a green class w darkblue
] .right-column[ - **Component development** is supported by many toolkits - **Components** are just small, reusable, well defined pieces of code that allow programmers to design and develop our UIs in a consistent way ([source](https://blog.bitsrc.io/building-a-consistent-ui-design-system-4481fb37470f)). - A UI component can create both functional and visual consistency in an application or set of applications. - Often components support new forms of input/direct manipulation. ] --- # Component Developers .left-column50[ - Develop novel interaction techniques (for carrying out a specific interactive task) - Enter a number in a range - Swiping to invoke an action - Gesture based text entry 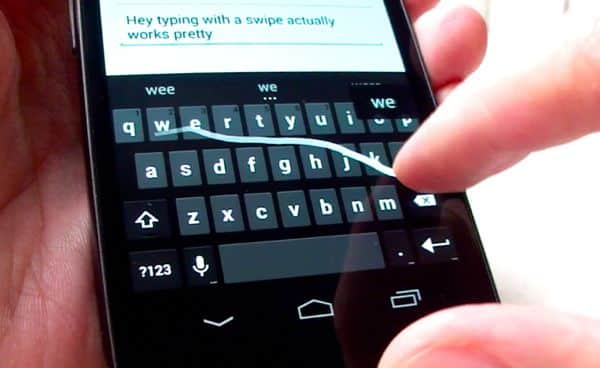 ] .right-column50[ 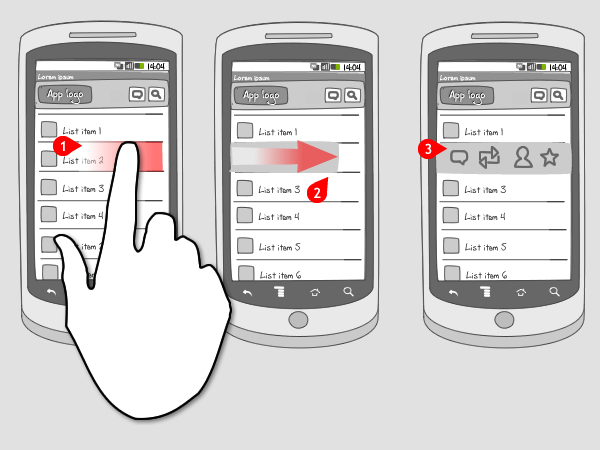 ] --- # Doodle assignment: What are the components? .left-column-half[Inspired by Google's Doodles, and will include drawing animation (to be discussed): [Example from last year's class](img/drawing/doodlevid.mp4)] .right-column-half[ ![:youtube Animation showing images of food moving in a line down the page around a U finally forming a W, Sx8oiJGjaIM] ] --- # Component Development in 340 In the Doodle assignment you will be developing three components -- `LineView`, `CircleView` and `TextView`. They're not interactive, but they can each draw things on the screen. - The *Doodle* assignment helps you learn - What abstractions we use to draw and manipulate graphics on the screen - How to properly specify the bounding boxes for such graphics - How to create active graphics (animations) - How to load image and drawable resources in Android --- # More Component Development in 340 But how do I design my *own* fancy button? Does it have to be a rectangle? What makes for a good interactor?. .left-column50[ The *Colorpicker* assignment - Android event handling - Handling touch input - How and when to save application state - How to create non-rectangular interactors ] .right-column50[ The *Menus* assignment - How to analyze experimental results - How to properly design an interactor for picking from a list - More about callbacks ] --- # Developer Roles .left-column[
graph LR ip[Interface Programmer] w[Component Developer] l[Library Extender] a[Architecture Extender] t[Toolkit Builder] class ip,t yellow class w,a green class l darkblue
] .right-column[ - **Library Extenders** create new forms of layout, types of input, etc. - Supported by a few toolkits - Examples: - The Java JDK can be extended by developers who create a set of components in a `.jar` file that is included with a software project (example [JFreeChart](http://jfree.org/jfreechart/)) - JavaScript has been extended by [many libraries](https://en.wikipedia.org/wiki/List_of_JavaScript_libraries), such as Bootstrap or jQuery. ] --- # Library Extender: Support for Styling .left-column30[ 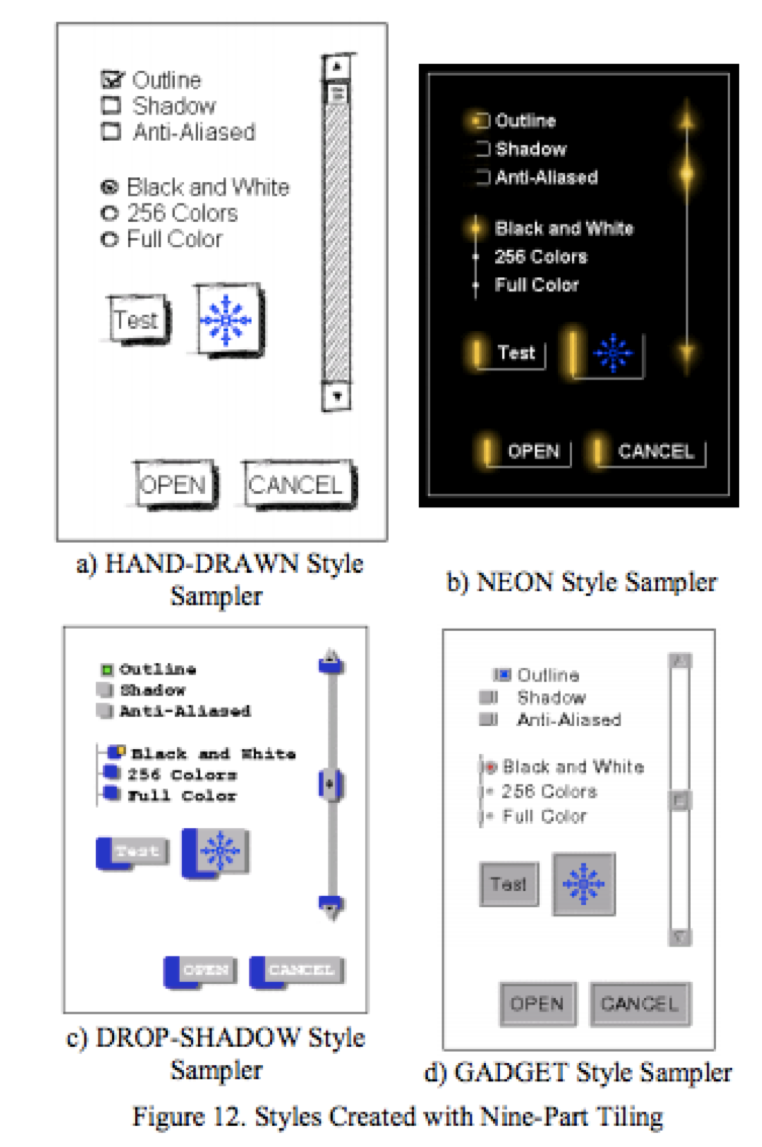 ] .right-column60[ subArctic allowed visually rich, dynamically resizable, images to be provided using primarily conventional drawing tools (and with no programming or programming-like activities at all). Scott E. Hudson and Kenichiro Tanaka. (UIST '00) ] --- # Library Extension in 340 In the *Layout* assignment you will learn - How to use the interactor hierarchy and how to understand it - How to use Android constraints to control layout and properly respond to changes such as switching from portrait to landscape mode - How to create a *layout container* that can properly position its contents --- # Developer Roles .left-column[
graph LR ip[Interface Programmer] w[Component Developer] l[Library Extender] a[Architecture Extender] t[Toolkit Builder] class ip,t yellow class w,l,a green class a darkblue
] .right-column[ **Architecture Extenders** modifies the flow of information within a toolkit to create entirely new effects (e.g. adding support for command objects) Supported by very few toolkits ] --- # Architecture Extender: Animation .right-column50[ 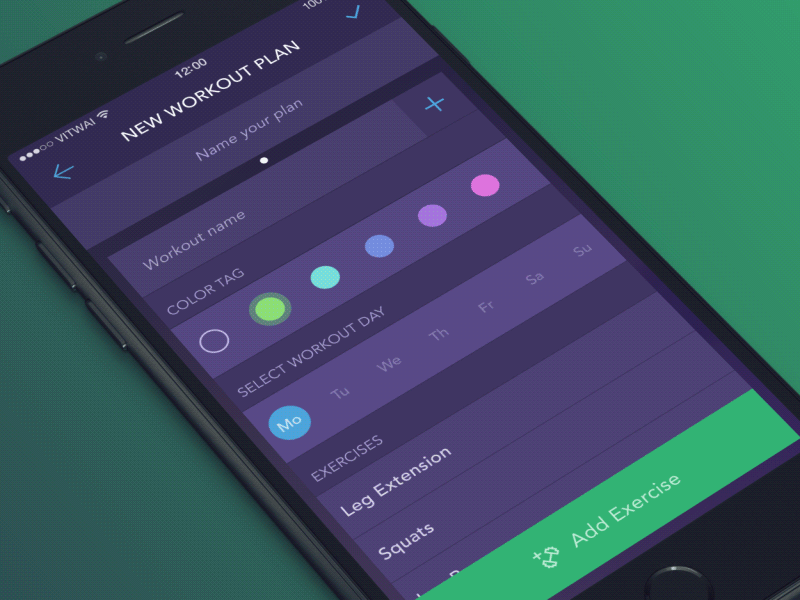 ] Integrated into existing GUI toolkit Primary abstraction: transition - models movement over time - through arbitrary space of values (e.g., color) - screen space is most common .footnote[Great post about [types of animation on mobile phones](https://yalantis.com/blog/-seven-types-of-animations-for-mobile-apps/), also source of the image] --- # Architecture Extension in 340 We don't fully extend the architecture, but we do add new infrastructure in the *Undo* assignment: We add support for command objects and stacks for keeping track of what can be undone and redone. --- # Developer Roles .left-column[
graph LR ip[Interface Programmer] w[Component Developer] l[Library Extender] a[Architecture Extender] t[Toolkit Builder] class ip,t yellow class w,l,a green class t darkblue
] .right-column[ **Toolkit builders** create entirely new toolkits that enable radical new forms of interaction e.g. RapID https://make4all.org/portfolio/rapid/ ] --- # Toolkit Builder: Physical Interfaces Creates entirely new toolkits that changes what we can do ![:youtube Physical Interface made out of RFID tags,4k15uXpp7-g] --- # Toolkit for Overloading Existing Interfaces .left-column60[ Prefab supports pixel based enhancements: ![:youtube Prefab Demo,lju6IIteg9Q] ] .right-column30[ Can be composed, reused, shared Robustly annotate interface elements with metadata Enables runtime enhancements.]