# CSE 340 Lab 9 (Winter 2021) ## Week 9: Getting started with Undo .title-slide-logo[ 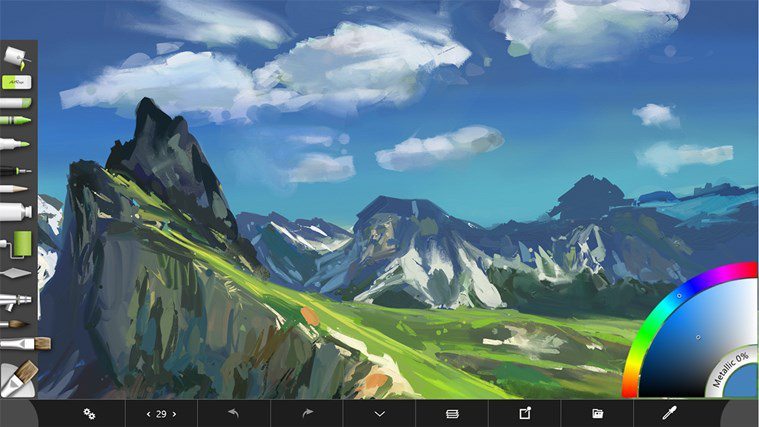 ] --- # HW Timeline - Menus part 5-6 due TONIGHT at 10pm - Locks Saturday March 6th - **AS6 Undo released!** We have pushed the dates back. - Programming: Due March 16th @ 10:00pm, locks March 18th @ 10:00pm - Reflection: Due March 18th (in 2 weeks), 10:00pm --- # Section 9 Objectives - Understanding Undo assignment - General concepts review - Practice Quiz 6 review - Work time/ Questions on Undo or Menus assignment --- # The Undo feature - Incredibly useful interaction technique - Reverts the application to an older state - Mistakes can easily be undone 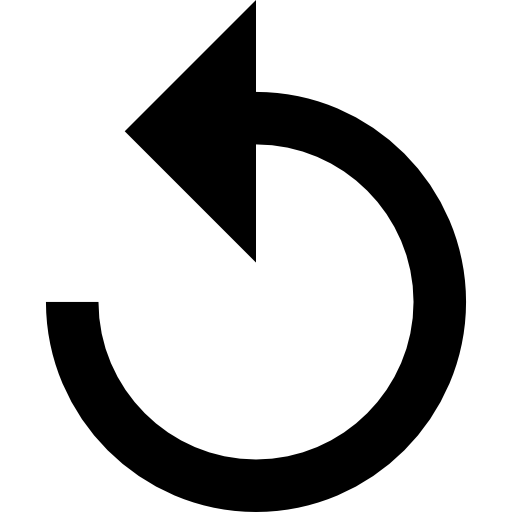 --- # The Redo feature - Inverse to the Undo feature - "Undoes" an undo - Work previously undone is reapplied - *"Huh, maybe I made the right decision after all..."* 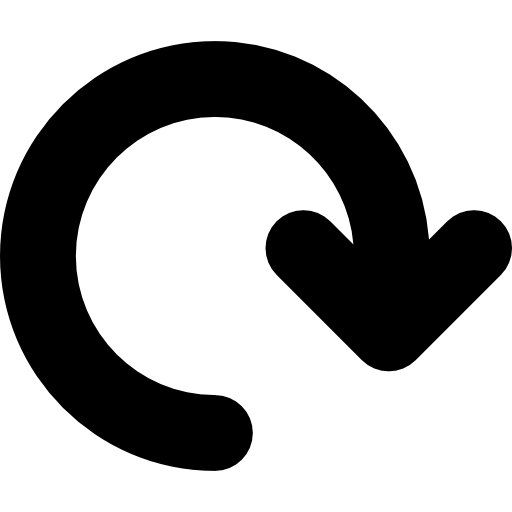 --- # Codebase: Application .left-column60[ - `ReversibleDrawingActivity`, class contains the entire interactor hierarchy - Floating Action Buttons (FAB), buttons that allow for actions supported by AbstractReversibleDrawingActivity - References DrawingView in AbstractDrawingActivity - `AbstractReversibleDrawingActivity`, abstract class that extends AbstractDrawingActivity - adds support for undo/redo - includes buttons for undo/redo - `doAction()`, `undo()`, `redo()`] .right-column30[
classDiagram AbstractDrawingActivity <|.. AbstractReversibleDrawingActivity AbstractReversibleDrawingActivity <|.. ReversibleDrawingActivity class AbstractDrawingActivity { DrawingView: "Canvas the user draws on" addMenu() addCollapsableMenu() doAction() } class AbstractReversibleDrawingActivity { +AbstractStackHistory +doAction() +undo() +redo() } class ReversibleDrawingActivity { +onAction() +onColorSelected() +onThicknessSelected() }
] --- # Codebase: Application .left-column60[ - `AbstractDrawingActivity` - abstract class for app that supports drawing without history - Wrapper around a `DrawingView` - `doAction()` - Contains methods for adding menus and changing visibility] .right-column30[
classDiagram AbstractDrawingActivity <|.. AbstractReversibleDrawingActivity AbstractReversibleDrawingActivity <|.. ReversibleDrawingActivity class AbstractDrawingActivity { DrawingView: "Canvas the user draws on" addMenu() addCollapsableMenu() doAction() } class AbstractReversibleDrawingActivity { +AbstractStackHistory +doAction() +undo() +redo() } class ReversibleDrawingActivity { +onAction() +onColorSelected() +onThicknessSelected() }
] --- # Codebase: DrawingView .left-column-half[- `AbstractDrawingActivity` is a wrapper around a `DrawingView` - Sets behavior for how strokes are drawn - DrawingView, a drawing canvas that handles strokes - Strokes are just `StrokeView`'s in the `DrawingView` - `onTouchEvent()` implements a PPS that describes lifetime of a stroke being created] .right-column40[
classDiagram class AbstractDrawingActivity { DrawingView: "Canvas the user draws on" addMenu() addCollapsableMenu() doAction() } class StrokeView { Path onDraw() }
] --- # Codebase: Actions .left-column-staff[ - `AbstractAction` abstract class defines basic behavior any Action should have - `AbstractReversibleAction` abstract class defines interface for actions that can be undone - `doAction()` chain: `AbstractReversibleDrawingActivity.doAction()` ➡`AbstractDrawingActivity.doAction()` ➡`AbstractAction.doAction()` - `AbstractReversibleDrawingActivity`'s `undo()` and `redo()` call methods on `AbstractReversibleAction` to undo/redo] .right-column55[
classDiagram AbstractAction <|.. AbstractReversibleAction AbstractReversibleAction <|.. ChangeColorAction AbstractReversibleAction <|.. ChangeThicknessAction AbstractReversibleAction <|.. AbstractReversibleViewAction AbstractReversibleViewAction <|.. StrokeAction class AbstractAction { doAction() } class AbstractReversibleAction { +boolean done +undoAction } class AbstractReversibleViewAction { +invalidate }
] --- # Codebase: Actions .left-column-staff[ - Actions: - `ChangeColorAction` - `ChangeThicknessAction`, implement for homework - `StrokeAction` - And more, create your own action!] .right-column55[
classDiagram AbstractAction <|.. AbstractReversibleAction AbstractReversibleAction <|.. ChangeColorAction AbstractReversibleAction <|.. ChangeThicknessAction AbstractReversibleAction <|.. AbstractReversibleViewAction AbstractReversibleViewAction <|.. StrokeAction class AbstractAction { doAction() } class AbstractReversibleAction { +boolean done +undoAction } class AbstractReversibleViewAction { +invalidate }
] --- # Codebase: History .left-column60[The `AbstractReversibleDrawingActivity` uses an StackHistory interface to manage the undo/redo history - You will implement the concrete `StackHistory` which implements `AbstractStackHistory` - Think: What data structures are used? Why does this make sense? - What happens to the redo history if you take a new action after undoing a couple times? - What happens to the undo history if you redo an action? - What happens to the redo history if you undo an action?] .right-column30[
classDiagram AbstractStackHistory <|.. StackHistory class AbstractStackHistory { addAction(AbstractReversibleAction action) undo() redo() canUndo() canRedo() } class StackHistory { +capacity: "Max stack size" }
] --- # Review of Mental Models What is a mental model? Choose one of the answers below: 1. When you see an image that has missing parts, your brain will fill in the blanks and make a complete image so you can still recognize the pattern 2. A model of the screen’s UI layout 3. A mental model is what the user believes about the system at hand 4. What you imagine when you concentrate really hard --- # Review of Mental Models What is a mental model? Choose one of the answers below: - **A mental model is what the user believes about the system at hand** 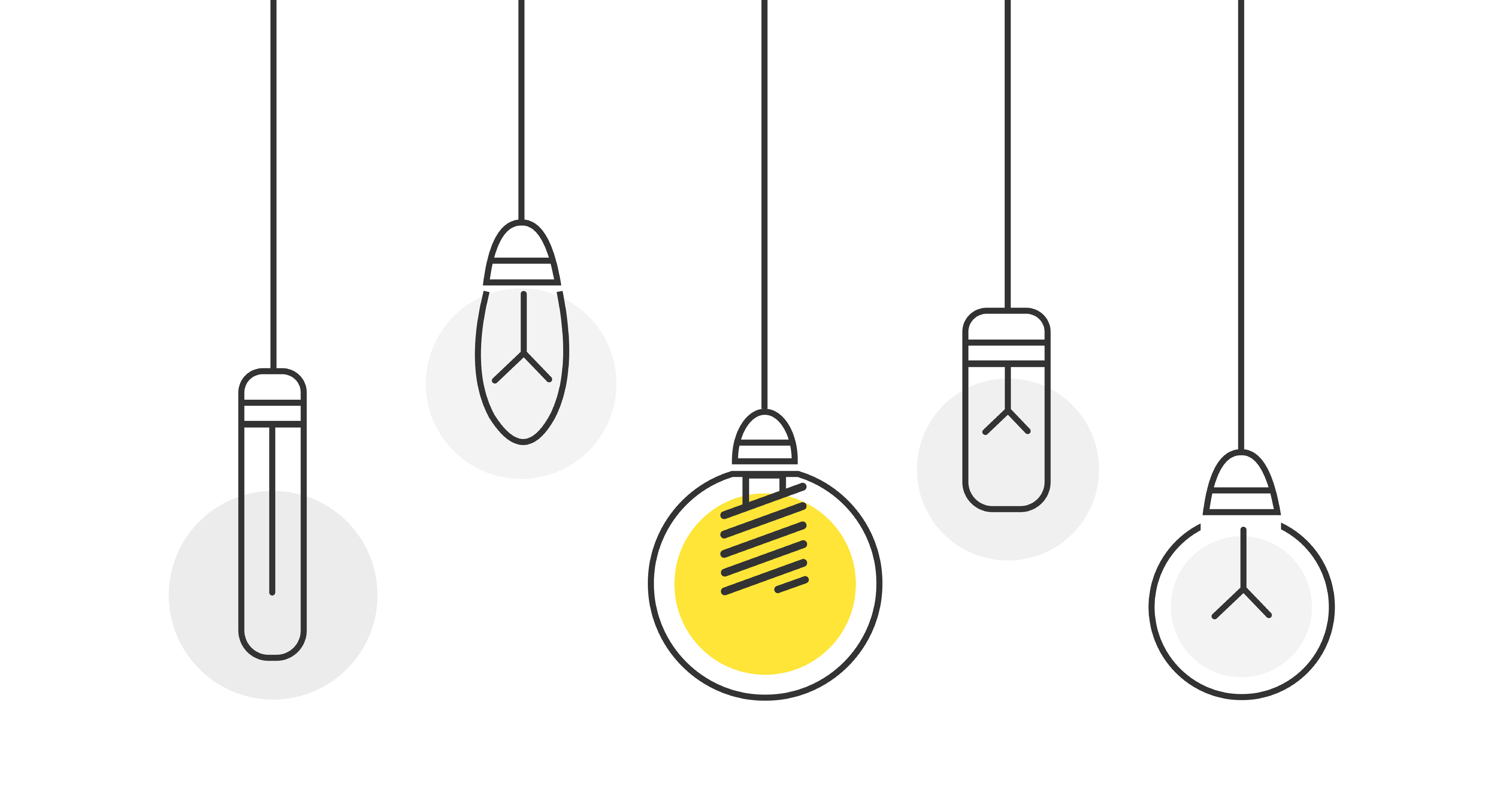 --- # Study Design Question, Part 1 In a research experiment you are attempting to determine if blocking notifications while the user is sleeping improves the overall quality of the user’s sleep. The first development task you’ve been given is to determine if the user is asleep and how you might block notifications. - List **3 sensors you might use on the phone** to determine this and why you chose those sensors. - For each sensor list what **type of callback** you would used with that sensor: snapshot, fences, or not applicable --- # Study Design Question, Part 2 The app has been built, yay! 😃 But now you need to test it to see if it actually helps people achieve better sleep. - How would you design a study to test this? - Describe the **hypothesis** for your user study. - Describe your study method. What **conditions would you study and what tasks** would you have participants complete? - What **ethical** implications might be raised in testing this application? --- # Using Google Awareness API in Android - Playground App by Joe Birch, Android Engineer @Buffer: https://github.com/hitherejoe/Aware - Reminder: refer to lecture [slides](https://courses.cs.washington.edu/courses/cse340/21wi/slides/wk08/ml.html#21) - Fence API = app reacts to current situation, **notifies user EVERY TIME a combination of CONDITIONS met** - Snapshot = "snapshot" of data at moment in time. Can check for data every X minutes/time **INTERVAL**. --- # Canvas Quiz Questions Question 1: 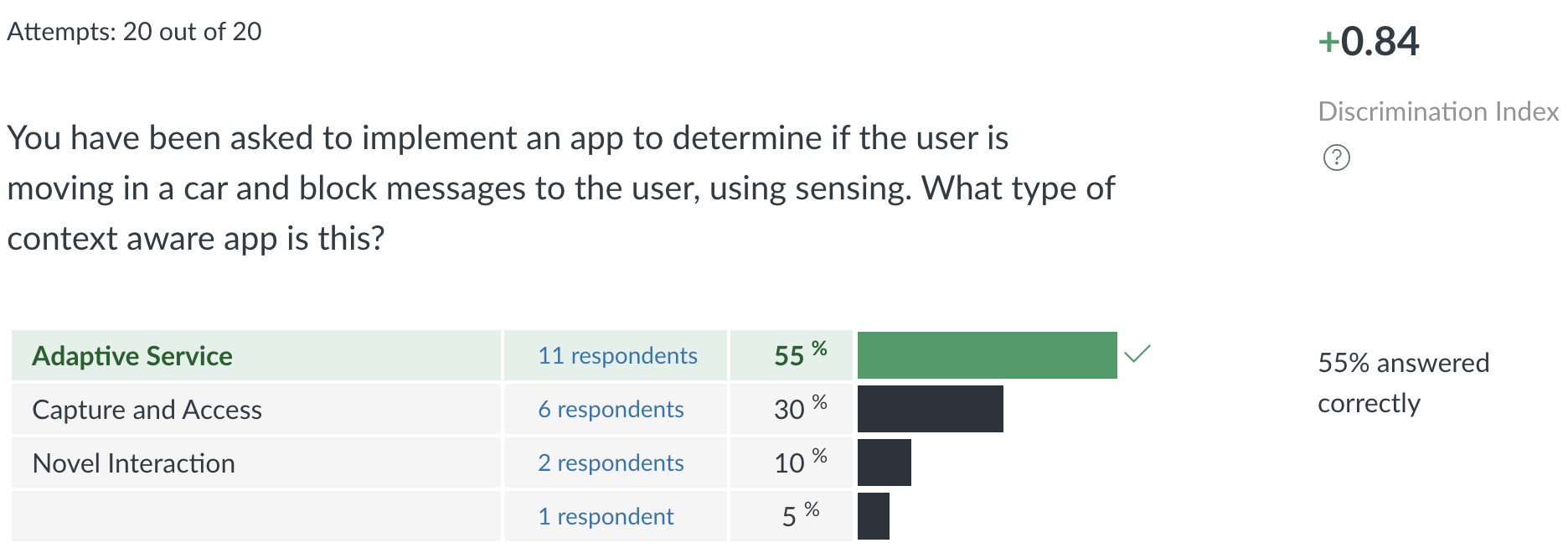 --- # Canvas Quiz Questions Question 1 continued: - Answer: **Adaptive Service: involves a *CHANGING* SERVICE or predictions based on user history or PAST data** - Focus on “adapting” - Capture and Access: focuses on CAPTURING/storing and analyzing immediate input data - Ex: Health analysis app from scanning pictures of receipts, Dream Diary app with user inputted data --- # Canvas Quiz Questions Question 1 continued: - Novel (new) interaction: Focus on creating NEW ways to physically INTERACT with app - Ex: VR headset sensing body movement, navigating smart watch with specific gestures - Behavioral Imaging: Focus on modeling HUMAN BEHAVIOR through observation - Detect behaviors that lead to negative impact and educate/inform user on how to change behavior - Ex: App that detects and models regular pick-up and drop-offs times from school for parents’ children --- # Canvas Quiz Questions Question 5: You have been asked to implement an app to determine if the user is moving in a car and block messages to the user. - List at least 2 sensors on the phone might you use to determine this. - Why did you pick those particular sensors? --- # Canvas Quiz Questions Question 6: See [these lecture slides on ethics](https://courses.cs.washington.edu/courses/cse340/21wi/slides/wk08/ml.html#68) 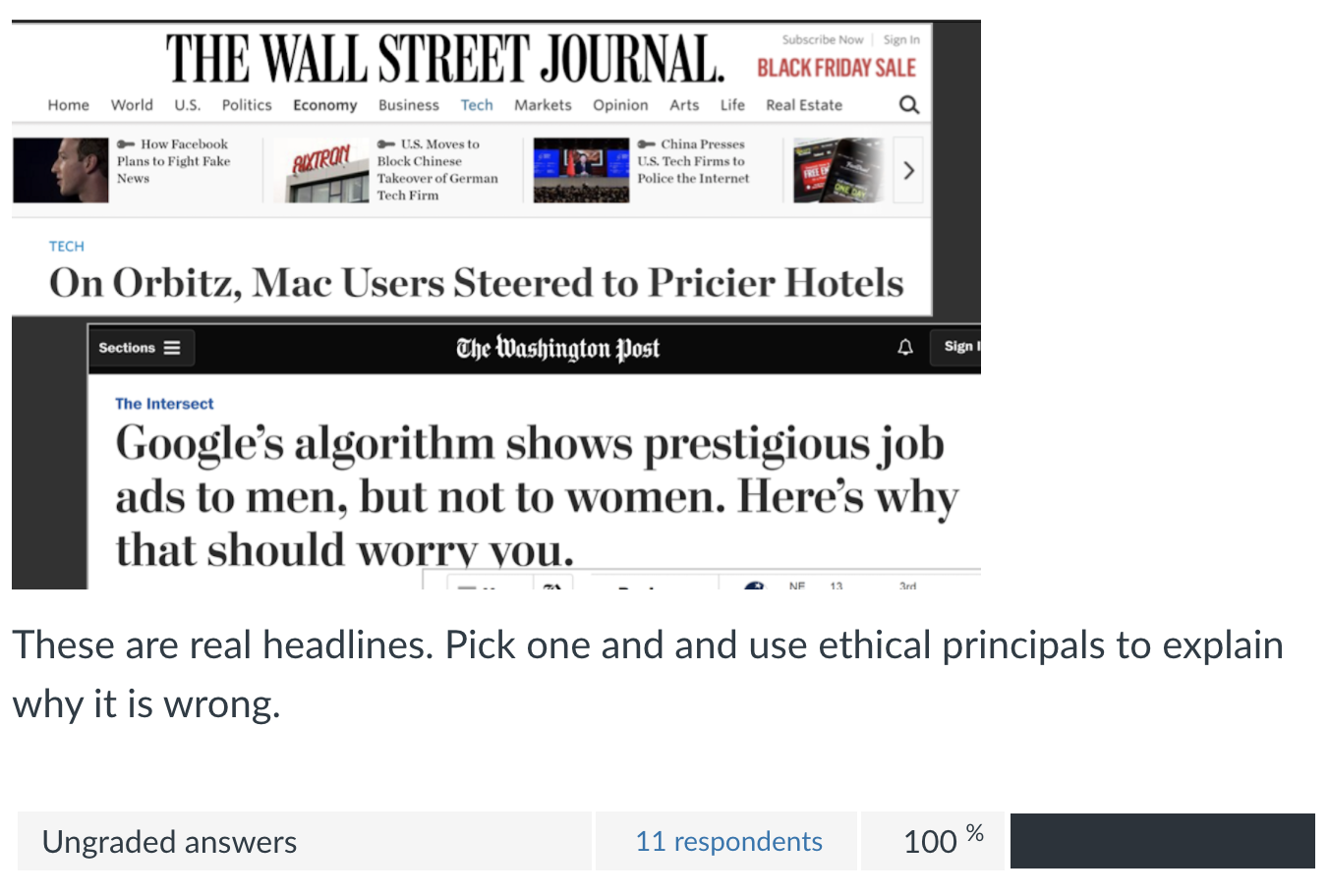