# CSE 340 Lab 6 (Winter 2021) ## Week 6: State Machines! Bundles! .title-slide-logo[ 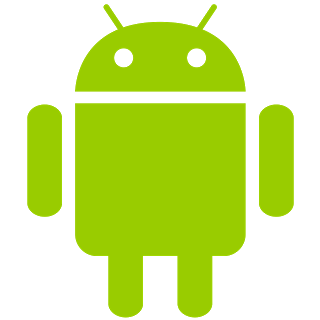 ] --- # Timeline - Color Picker and Reflection due: Thursday, February 18th @ 10:00pm - Code Lock/Reflection Due: Saturday, February 20th @ 10:00pm (if you are using late days) - Reflection Lock: Sunday, February 21st @ 10:00pm (10% deduction) - Examlet 2 is tomorrow! --- # Section 6 Objectives - Solicit some anonymous feedback and questions - AS5 Menus survey - State Machines - Worksheet: https://tinyurl.com/340section5 - Bundles - What're they? - Why they're important - How we use them (for the assignment and otherwise) - Examlet 2 Practice, Format below: - Layout, Redraw process - Accessibility, Alt text, Inclusive Design - Events: MVC, Event Record, Dispatch order - Questions on Color Picker? Examlet? --- # Feedback Feedback Form link: https://forms.gle/8LmGqU1ZhCFxmJpx5 - Any questions you have about assignment/ examlet - Any feedback you have on section - What can we do better? What is going well? - We want this to be a good use of time for you. --- # AS5 Menus Survey - Please fill out this [form](https://forms.gle/1D3wPyCRqDHnHC7o8) by this Saturday 02/13, so we can plan for the next assignment - AS5 Menus. --- # State Machines
stateDiagram-v2 [*] --> S1: DOWN S1 --> S1: MOVE S1 --> [*]: UP
- State Machines are used to respond to incoming events and allows for us to store state between events. - Start state - indicated with incoming arrow - End state - indicated with double-layered shape - Transition States - indicated with single-layered shape - Event Arrows - indicated with an arrow between states, represent different actions taken up states. --- # [Propositional Production System](https://tinyurl.com/14hzax1e) .left-column[
stateDiagram-v2 [*] --> S1: DOWN S1 --> S1: MOVE S1 --> [*]: UP
] .right-column[ - **Formal Definition: consists of a "state space definition + set of rules"** - PPS = state machine with: - ? (Boolean gates) - action calls - extra conditions required to fire ] --- # State Machine Practice .left-column50[
stateDiagram-v2 [*] --> S1: PLACE_FINGER S1 --> S2: MOVE/DOWN?
display_pause() S1 --> S3: MOVE/UP?
display_play() S1 --> [*]: LIFT_FINGER/
nothing() S3 --> [*]: LIFT_FINGER/
play_music() S2 --> [*]: LIFT_FINGER/
pause_music()
] .right-column50[ Discussion: What is the behavior of this State Machine/PPS? ] --- # Worksheet: State Machine Practice - Link: https://tinyurl.com/340section5 --- # Worksheet Solutions Problem 1: 1. updateThumbPosition() 2. updateVolume() 3. INSIDE 4. EssentialGeometry.BAR 5. updateThumbPosition() 6. updateVolume() 7. State.START 8. updateThumbAlpha() Read more: [How to turn the PPS into code 1](/courses/cse340/21wi/docs/pps.html) and [How to turn the PPS into code 2](/courses/cse340/21wi/docs/ppsscroll.html) and --- # Worksheet Solutions Problem 2: .left-column50[
stateDiagram-v2 [*] -->Pressed: DOWN/
InsideBar?A [*] --> Pressed: DOWN/
InsideButton?B Pressed --> [*]: UP/C Pressed --> Pressed: MOVE/D
] .right-column50[ Where - A is `updateButtonPosition(); updateButtonAlpha(); invokeScrollAction(); invalidate();` - B is `updateButtonAlpha(); invokeScrollAction(); invalidate();` - C is `updateButtonAlpha(); invalidate();` - D is `updateButtonPosition(); invokeScrollAction(); invalidate();` ] --- # Bundles: So you got lots of apps.. And they all want to use _tons_ of memory, but they don't **need** that memory all the time. - Android: You get no/minimal memory when you're not actively being used. - Apps: But then how do we remember stuff when we are being used if we can't save it in memory - Android: Use this `bundle` _(In truth the app could write/read its state to disk whenever it is being closed/opened but that is time consuming and would delay the OS launching new things)_ --- # What is a Bundle? - First what happens when the user closes the application? Does it die? - **No!** --- # What is a Bundle? - First what happens when the user closes the application? Does it die? **No** - Where does it go then? - Think about it as **hibernation** - All of it's memory is cleared, so all of your variables are _GONE_ 😲. - **But** Android lets you save some variables to a `bundle` right before your memory is cleared, and gives you your `bundle` back when you get the memory space back. --- # What is a Bundle? - First what happens when the user closes the application? Does it die? **No** - Where does it go then? **Stored by Android OS** - What's in the bundle? - You decide, entirely up to the application developer (you). - Values are mapped to unique KEYS (for set/retrieve) - Bundle is destroyed if the user **force quits** the app/phone is turned off. --- # Bundles & Code - Bundle management is occuring at the `activity` layer, not to be confused with any individual `view`. - When the user closes the app, right before it closes and its memory is cleared, Android invokes `onSaveInstanceState(Bundle outState)` on the activity, providing a reference to the activity for the app to save values into. - Then when the user opens the app again, Android invokes `onRestoreInstanceState(Bundle savedInstanceState)` returning the same bundle from earlier. - You can think of the `bundle` as a `Map
` that only supports certain types of objects (they must be `Serializable` which includes all primitives and `String`) _(Read more [here](https://developer.android.com/topic/libraries/architecture/saving-states))_ --- # Bundles: Code Example ```java public abstract class MainActivity extends AppCompatActivity { @Override public void onSaveInstanceState(Bundle outState) { super.onSaveInstanceState(outState); outState.putString("OUR_KEY", "bundles? bundles!!!"); } @Override protected void onRestoreInstanceState(Bundle savedInstanceState) { super.onRestoreInstanceState(savedInstanceState); String whatWeSaved = savedInstanceState.getString("OUR_KEY"); // whatWeSaved would contain "bundles? bundles!!!" } } ``` --- # Discussion: Using Bundles in Color Picker Not in this assignment but you could store the state of the Color Picker view directly into the bundle instead of storing the state of the application. - Think about the model of the application - Think about the model of the color picker view/interactor - Is it better to store the state of the application or the view? --- # Examlet 2 Practice Question 1A: Layout - Assume there is (A) MyView, (B) MyView's parent, and (C) other, in a larger view hierarchy. - Which is responsible for drawing MyView (implements onDraw)? - For deciding final size of MyView? - For deciding preferred dimensions of MyView? --- # Examlet 2 Practice Question 1A: Layout - Assume there is (A) MyView, (B) MyView's parent, (C) other, in a larger view hierarchy. - A is responsible for drawing MyView (implements onDraw) - B decides final size of MyView - A decides preferred dimensions of MyView - Refer to Android docs for review on [How Android Draws Views](https://developer.android.com/guide/topics/ui/how-android-draws) --- # Examlet 2 Practice Question 1B: Layout - Why would you ever manually call invalidate() in Android? --- # Examlet 2 Practice Question 1B: Layout - Why would you ever manually call invalidate() in Android? - Usually, Android system handles resizing/drawing automatically for given components - Ex: TextView.setText() -> already calls invalidate() - Use invalidate() to force redraw on custom component that rely on external resources/data set - Ex: Image resource on a View changes --- # Examlet 2 Practice Question 2: Accessibility - In what circumstances would you want to set the **focusable** attribute of a UI element to TRUE? --- # Examlet 2 Practice Question 2: Accessibility - In what circumstances would you want to set the **focusable** attribute of a UI element to TRUE? - Interactive UI Elements (accepts input in some form) - NOT for decorative elements like Spacers... etc - See WebAIM guidelines for decorative image [examples](https://webaim.org/techniques/alttext/#decorative) --- # Examlet 2 Practice Question 3A: Events - In the Event Record, what information about the UI event should be stored? (Hint: there are 5 categories) --- # Examlet 2 Practice Question 3A: - What: Event Type (mouse moved, key down, etc) - Where: Event Target (the input component, ex: Button), - When: Timestamp (when did event occur) - Value: Mouse coordinates; which key; etc. - Context: Modifiers (Ctrl, Shift, Alt, etc); Number of clicks at once; etc. --- # Examlet 2 Practice Question 3B: - Regarding Event Dispatch (how events are handed out to Views), what type of traversal does "picking" do? --- # Examlet 2 Practice Question 3B: - Regarding Event Dispatch (how events are handed out to Views), what type of traversal does "picking" do? - "Picking": process of choosing + filtering Views that can receive a particular Event - Answer: **REVERSE pre-order traversal** of the View tree (RIGHT LEFT SELF), not the same as post-order - Save all references to Views in an array - Filter out Views that don't listen for the event --> Get final list of picked Views! --- # Work time on Color Picker - Color-Angle conversion [hints](/courses/cse340/21wi/docs/angles.html) - We were supposed to have you implement Color -> Angle but we accidentally switched it around for this quarter's stub code. - You'll implement Angle -> Color. - Spec has been updated to reflect the above