# CSE 340 Winter 2021 .title-slide-logo[ 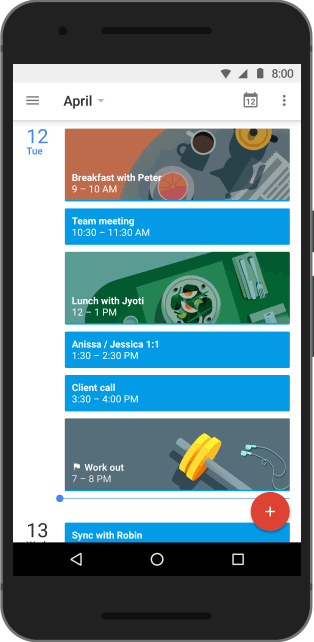 ] --- # Layout Timeline - Layout part 1-2 due: **TODAY @ 10:00pm (No late days allowed)** - Layout part 3-4 and part 5 (reflection) due: Next Thursday, January 28 @ 10:00pm - Lock: Saturday, January 30 @ 10:00pm (If you are using late days) --- # Instruction for turning in Layout part 3-4 - **(Important!)** You will have to ACCEPT the Layout Part 3-4 at Gitgrade before you turn in part 3-4 - Track your acceptance/ submission of all assignments: https://gitgrade.cs.washington.edu/student/summary/8723 - Work in the same repo as Part 1-2 --- # Section 3 Objectives - Prep for Layout Part 3 and 4 - XML vs. Programmatic (Java) - Concept of "inflate" in Android - `LayoutInflater` - Sample [Layout Code](https://gitlab.cs.washington.edu/cse340/exercises/layoutlab/) - Examlet 1 Practice - Help with Layout --- # XML vs. Programmatic (Java) .left-column50[ - XML files -> good for describing **static finite** information, a data format - XML is easier setting up the Layouts - XML shows the Layouts directly - XML can be inflated many times, like a blueprint ] .right-column50[ 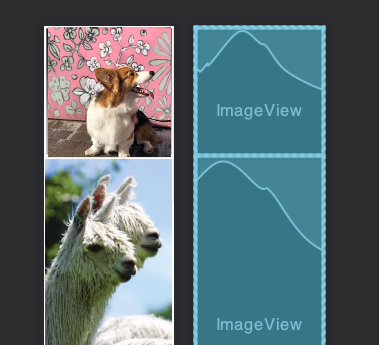 ] --- # XML vs. Programmatic (Java) - Programmatic method: easier adding items to the Layouts - Can use loops! - Sample solution of adding items into Layout in Part 1 (XML) has 55 lines - Sample solution of adding items into Layout in Part 2 (Java) has ONLY 17 lines! --- # LayoutInflater - **"Inflate":** Accepts a valid XML file and converts it into a `View` object or interactor hierarchy. - stuff declared in XML -> be able to manipulate them in Java - done by the Android OS; render the thing by creating view objects in memory - In Part 3 you will code part of it in an XML file and the rest programmatically (using Java). - Why? (Discuss about pros and cons of constructing layout with XML and programmatically) --- # How do we use the [LayoutInflater](https://developer.android.com/reference/android/view/LayoutInflater#inflate(int,%20android.view.ViewGroup)? 1. Setting up the LayoutInflater ```java // Obtains the LayoutInflater from the given context LayoutInflater.from(Context context) ``` 2. Inflate ```java // Inflate a new view hierarchy from the specified XML resource inflate(int resource, ViewGroup root) ``` - resource = int ID for an XML layout resource to load (e.g., R.layout.main_page) - root = optional view to be the **parent** of the generated hierarchy. - May be null. --- # Quick Exercise Construct a LayoutInflater and pass in the `part1.xml` file. --- # Quick Exercise Solution Construct a LayoutInflater and pass in the `part1.xml` file. ```java public Part1View(Context context, List
imageNames, int vMargin) { // Obtain the inflater from the context LayoutInflater inflater = LayoutInflater.from(context); // Inflate R.layout.part1 View newView = inflater.inflate(R.layout.part1, null); // newView is at the root of the inflated tree // Add it to this view this.addView(newView); } ``` --- # From Last Week: [Layout Lab](https://github.com/harshitha-akkaraju/layoutlab) - When doing Layout Part3/4: Reference the solution the for the "programmatic" method - GitHub Link to [Solution](https://gitlab.cs.washington.edu/cse340/exercises/layoutlab/-/blob/master/app/src/main/java/cse340/exercises/layoutlab/ProgrammaticConstraints.java) --- # Examlet 1: TOMORROW Friday 1/22 during class! - Drawing - Toolkit architecture vs Toolkit library - Interactor Hierarchy labeling and drawing trees - Layout (what parameters to assign to something to look a certain way, how final sizes are determined...etc) --- # Quick Examlet 1 Review (1/5) Bounding Box question 1: - You are creating a new CircleView that can draw a circle in the Doodle app. - The user wants to add a circle so that the center point is at **(150, 50)** (in parent coordinates). - The circle should have a **radius of 5.** - In **CircleView#onDraw** you will need to call canvas.drawCircle(cx, cy, r, p). - **What values should you use for cx and cy in this your onDraw code?** --- # Quick Examlet 1 Review (1/5) Bounding Box question 1: - You are creating a new CircleView that can draw a circle in the Doodle app. - The user wants to add a circle so that the center point is at **(150, 50)** (in parent coordinates). - The circle should have a **radius of 5.** - In **CircleView#onDraw** you will need to call canvas.drawCircle(cx, cy, r, p). - **What values should you use for cx and cy in this your onDraw code?** - (5, 5) --- # Quick Examlet 1 Review (2/5) Bounding Box question 2: - Which are valid corners for a bounding box for a circle created using the android Canvas to drawCircle(60, 45, 5, p)? - For the below prompt, assume we are drawing from respect to **parent coordinates** (not inside child class). - Assume to not account for line thickness. Choices: - (0,0) - (60, 45) - (55, 50) - (55, 40) - (5, 5) --- # Quick Examlet 1 Review (2/5) Bounding Box question 2: - Which are valid corners for a bounding box for a circle created using the android Canvas to drawCircle(60, 45, 5, p)? - For the below prompt, assume we are drawing from respect to **parent coordinates** (not inside child class). - Assume to not account for line thickness. Answers: - **(55, 40)** - **(55, 50)** --- # Quick Examlet 1 Review (3/5) .left-column50[ View Hierarchy question: - Draw a View Hierarchy based on the screen capture. - Only include the contents below the top green bar. ] .right-column50[ 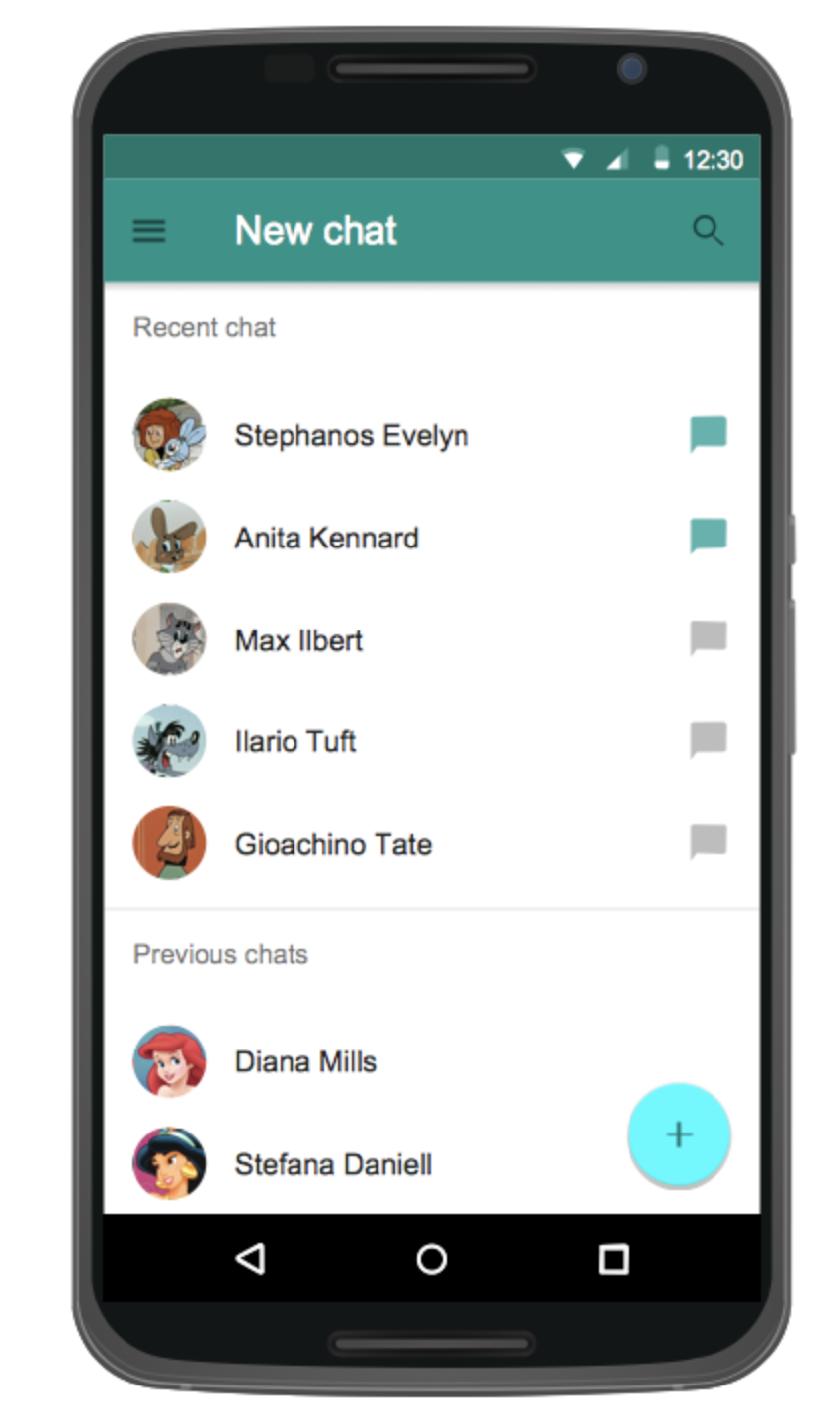 ] --- # Quick Examlet 1 Review (3/5) .left-column50[ Possible answer: 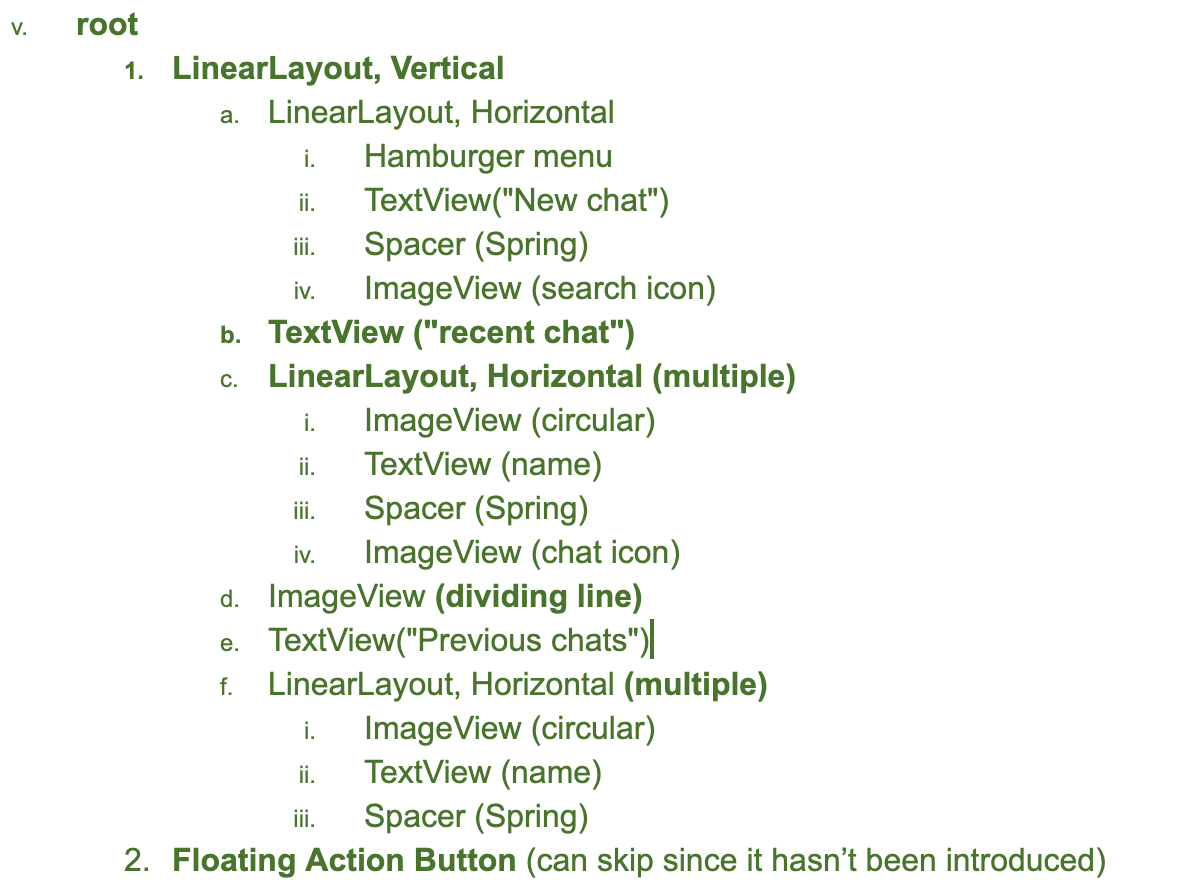 ] .right-column50[ 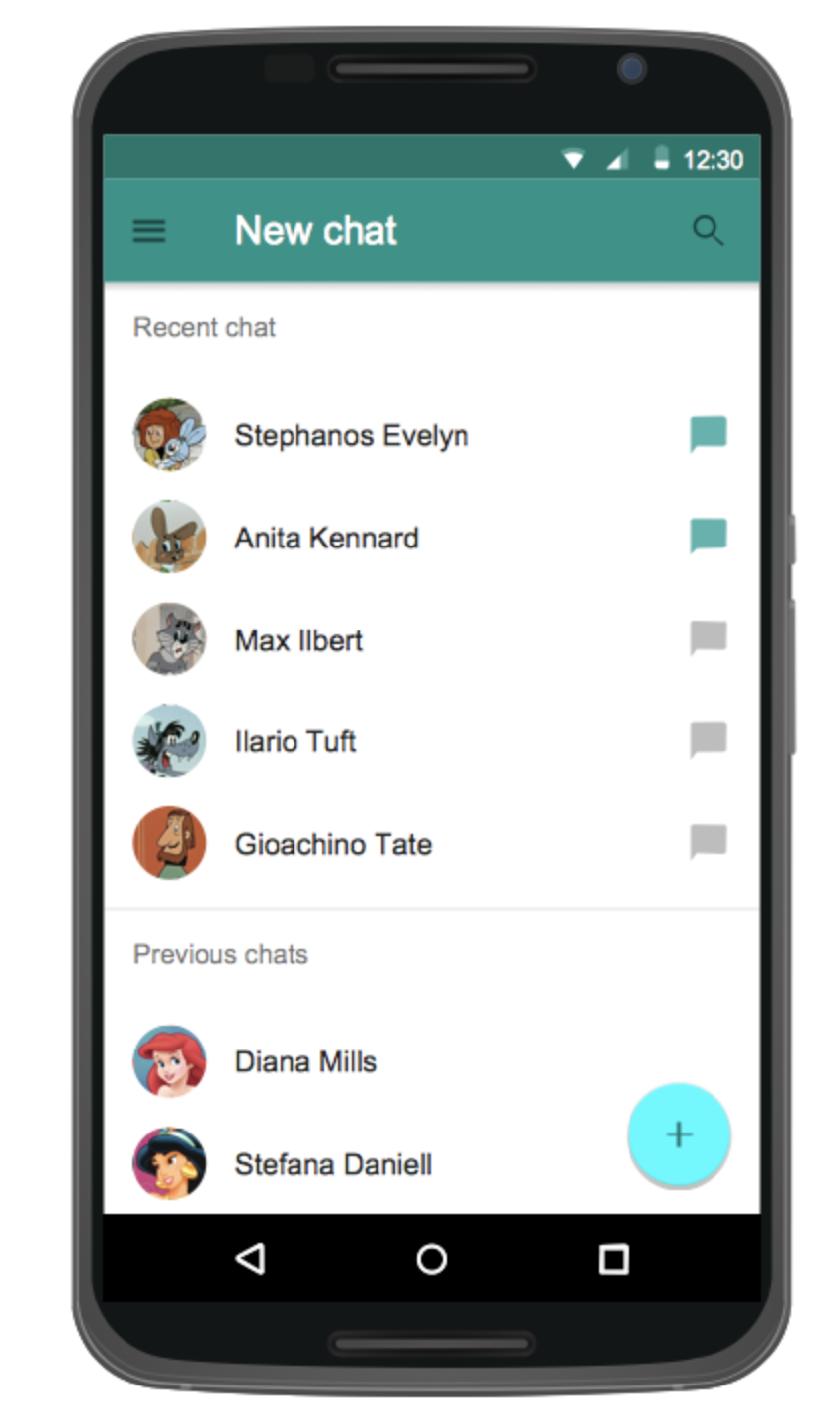 ] --- # Quick Examlet 1 Review (4/5) Interactor Hierarchy: Parent -> Children - In regards to a tree consisting of 1 parent and 3 children, list the z-order (from bottom to top) they will be drawn in. - ViewGroup (parent) -> [children: Foo Bar Baz] --- # Quick Examlet 1 Review (4/5) Interactor Hierarchy: Parent -> Children - In regards to a tree consisting of 1 parent and 3 children, list the z-order (from bottom to top) they will be drawn in. - ViewGroup (parent) -> [children: Foo Bar Baz] --- # Quick Examlet 1 Review (4/5) Interactor Hierarchy: Parent -> Children - In regards to a tree consisting of 1 parent and 3 children, list the z-order (from bottom to top) they will be drawn in. - ViewGroup (parent) -> [children: Foo Bar Baz] - **1. parent 2. foo 3. bar 4. baz** --- # Quick Examlet 1 Review (5/5) - Consider the onMeasure() that is overridden in Layout Part 3. - Is this method part of the **toolkit architecture or the toolkit library?** --- # Quick Examlet 1 Review (5/5) - **Answer: Toolkit Architecture** - [Interface Toolkit](https://courses.cs.washington.edu/courses/cse340/21wi/slides/wk01/first-app.html#19): provides services that help you create things - used by your program - contains library of components, architecture (see below) - Architecture: the fundamental running code (queue) that builds your app (hierarchy...structure) - Includes Interactor Hierarchy - Decides structure, redraw, layout, MEASURING stuff! - Manage your components/app lifecycle - Library: optional COLLECTION of functions, classes, COMPONENTS... that you can include in your app - Includes ex: ImageView class, ____View class ...etc - many more libraries out there that can be used w/Android: Expression Parsing Libraries, [ExoPlayer](https://github.com/google/ExoPlayer)...etc - Don’t need to reinvent the wheel. --- # Need help with Layout?