name: inverse layout: true class: center, middle, inverse --- # Practice Quiz 1 Review & Questions Lauren Bricker CSE 340 Spring 2021 --- name: normal layout: true class: --- layout: false [//]: # (Outline Slide) # Agenda - Reminders: - Doodle is due at 10pm on Thursday (Lock is saturday) - Includes video turned into canvas BY THAT TIME! - Review Quiz & Questions - [Transformations and Animation](https://courses.cs.washington.edu/courses/cse340/21sp/slides/wk01/animation.html#1) --- # Poll
??? --- # Developer Roles .left-column[
graph LR ip[Interface Programmer] w[Component Developer] l[Library Extender] a[Architecture Extender] t[Toolkit Builder] class ip,t,l,a,w yellow
] .right-column[ You are creating Views that will be used in our Doodle application. Which developer role best describes what you will be doing? ] --- # Developer Roles .left-column[
graph LR ip[Interface Programmer] w[Component Developer] l[Library Extender] a[Architecture Extender] t[Toolkit Builder] class ip,t,l,a yellow class w darkblue
] .right-column[ You are creating Views that will be used in our Doodle application. Which developer role best describes what you will be doing? You're a component developer! Recall: - **Components** are just small, reusable, well defined pieces of code that allow programmers to design and develop our UIs in a consistent way ([source](https://blog.bitsrc.io/building-a-consistent-ui-design-system-4481fb37470f)). - A UI component can create both functional and visual consistency in an application or set of applications. ] --- # Views in Doodle In `PartXActivity#doodle` (which is called from `AbstractMainActivity#onCreate`) you are creating views that are being added to the parent a coordinate locations (in device independent pixels). These are added to an interactor hierarchy. ```java abstract class Doodler extends AppCompatActivity { // ... @Override protected void onCreate(@Nullable Bundle savedInstanceState) { super.onCreate(savedInstanceState); // ... doodle(...) } abstract protected void doodle(FrameLayout doodleView); ``` --- # How is the tree used for drawing? Toolkit Architecture: - Draw an interface == walk the tree and tell interactors to draw - Update an interface == walk the tree, only redraw things that are marked as dirty - Deliver input == walk the tree and deliver events (after midterm) Our components only draw when the *system* calls `onDraw` for us. onDraw renders objects on the canvas based on pixel coordinates --- .left-column[ 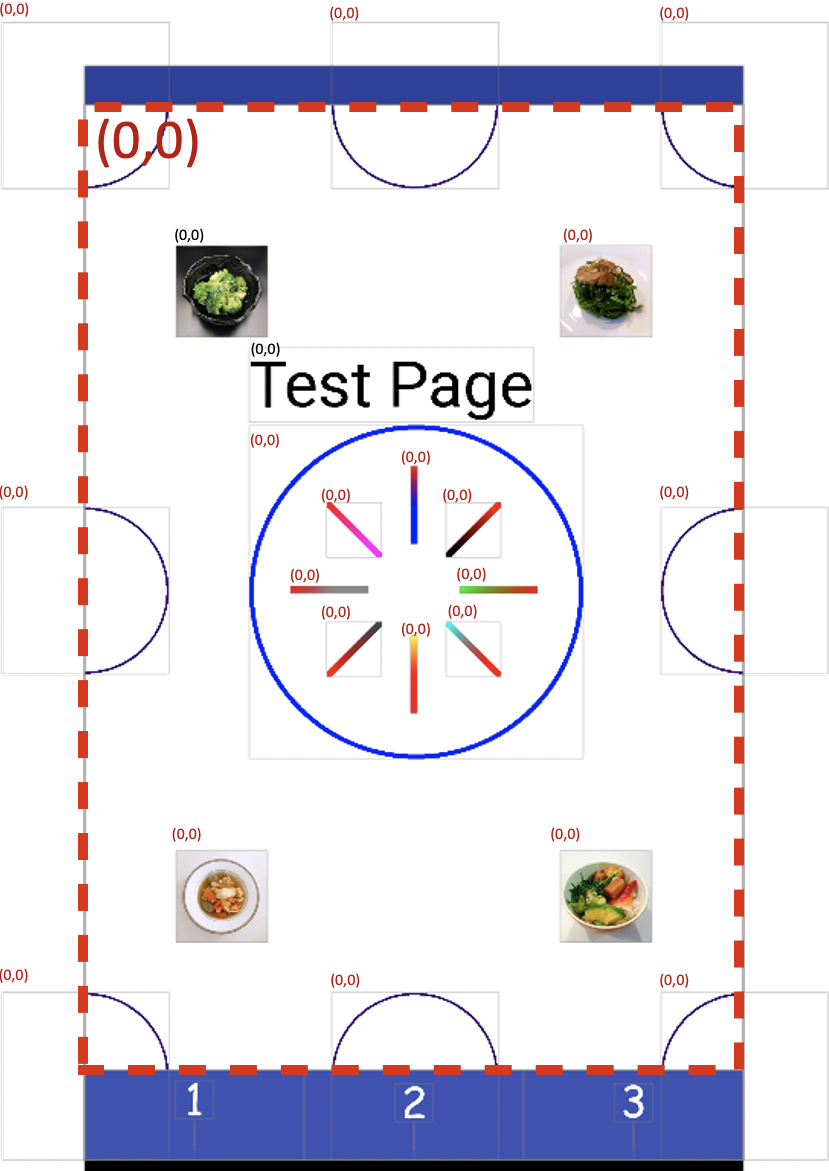 ] .right-column[ The parent in this image is shown with a dotted red line. Suppose I want to add a new `LineView` going from (450,250) to (300,450) (in parent coordinates). What will the position of the top left corner of that `LineView`'s bounding box be in parent coordinates? ] ??? The position will be (300, 250). The line goes from the top right corner to the bottom left corner of the bounding box, but it's leftmost coordinate is 300 and it's topmost coordinate is 250 --- .left-column[ 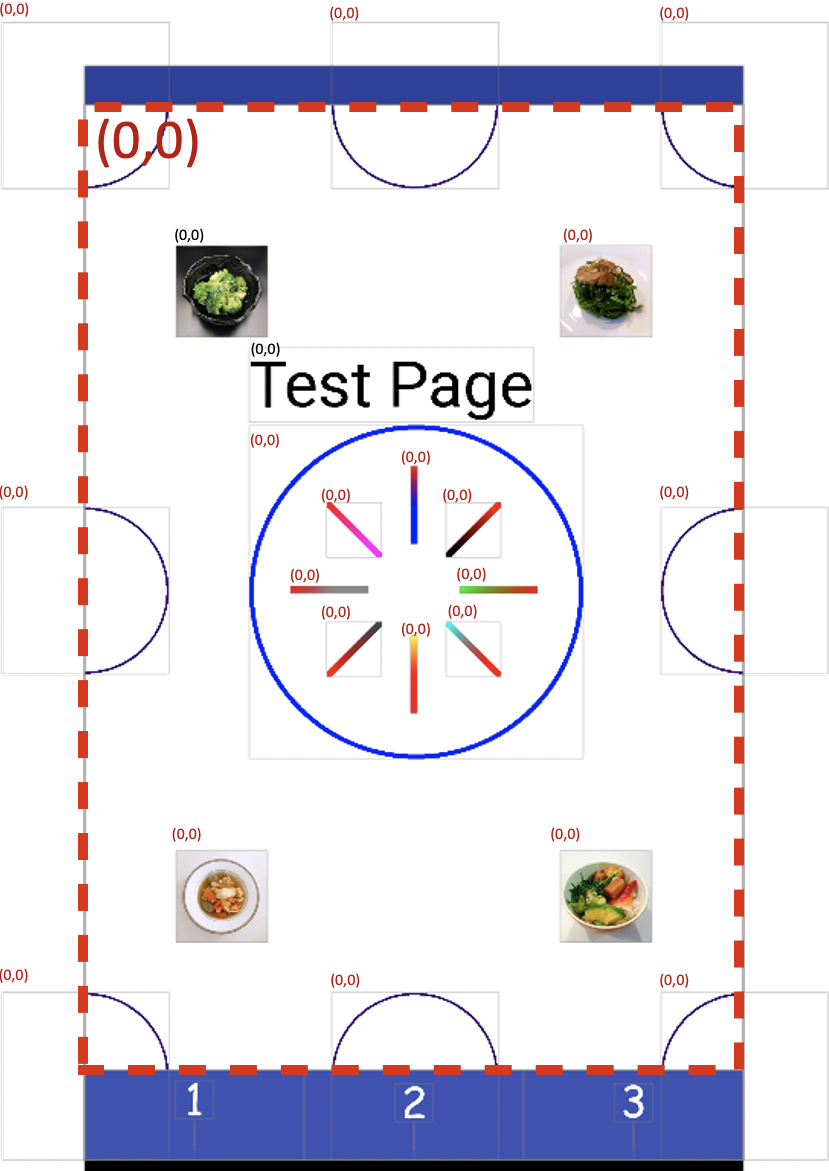 ] .right-column[ Suppose I add a `LineView` instead going from (15, 5) to (5, 15). What will the start and end coordinates of the line be in child coordinates (*i.e.* within `onDraw()` in the `LineView` object itself?). ] ??? The position will be (5, 0) to (0, 20). This is because position should be relative to a bounding box that starts at the leftmost and topmost position of the child --- # More practice with position Suppose the user wants to add a circle so that the center point is at (100,100) on the drawingView and has a radius of (10). In `CircleView#onDraw(Canvas)` you will need to call `canvas.drawCircle(cx, cy, radius, paint)` (note that drawCircle takes the (x, y) location of the center of the circle as input). What values should you use for `cx` and `cy` in this your `onDraw` code? --- # More practice with position Consider the same CircleView object that contains a circle of radius 10 that we want to display in the drawingView so the center appears at `100, 100` (pixels) in the parent view's coordinate system. How should we set up the bounding box for the `CircleView` so that its canvas will be correctly clipped? What are `x`, `y`, `width` and `height` for the bounding box (in parent coordinates?) --- .left-column[ 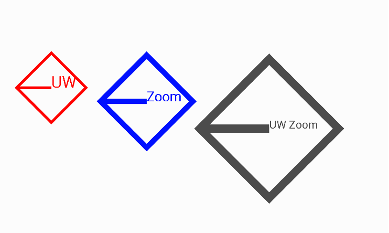 ] .right-column[ The Zoom marketing team decides they want to be able to display their new logo in any size, location, and color in the app. As a Zoom Software Engineer, your team assigns you to write a `LogoView` class (a subclass of `DrawView`) that can be used for this purpose. The `LogoView` object will contain the following features drawn on its `Canvas` in the same color. - A rotated square that is 60% the size of the `LogoView`’s bounding box. Text in the default font and in a specified font size that is drawn starting at the mid point of the `Canvas`. - A line halfway down the `LogoView`’s `Canvas` that starts 10% over from the left side of the canvas and goes to the midpoint of the Canvas mark. ] ??? --- .left-column[ 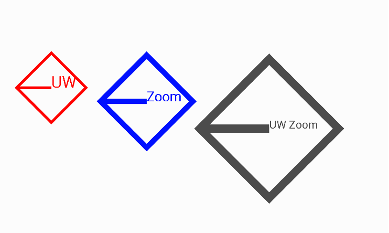 ] .right-column[ Other than the context that is passed into any subclass of an `DrawView`, what other parameters might you need to construct the `LogoView`? Justify each parameter by explaining how it would be used either in `onDraw()`, to modify the `brush`, or in `initFromParentCoords()`. A lot of people were confused by this... ] ??? Possibilities include: - `brush`: containing the stroke width of the line that the rotated rectangle and line are drawn with, and the color and the Font information. - `text`:the text to show in the logo - The parentX and parentY coordinates - The width and the height of the logo --- .left-column30[ "I'm still a little confused about the difference between the 'vector model' and the 'raster model'" ] .right-column70[ A raster model is a 2D array of pixel color values which are light up on the screen. A vector model allows us to specify objects, like the start and end point of a line relative to the coordinate system. So if the coordinate system gets "bigger" (i.e. more pixels per "unit" in the coordinate system) we can scale it up appropriately. Example: Let's say I'm using Adobe Illustrator. I have a circle on the screen (which is 72 DPI - dots per inch) relative to a "Frame" which represents a piece of paper. When I print (on a printer with 600 dpi resolution) the circle will stay at the same location relative on the page. If we did the same thing with a raster drawing of a circle, each pixel would be enlarged by 600/72 per pixel which would look blocky. ]