# CSE 340 Lab 5 Spring 2021 ## Week 6: Event Handling .title-slide-logo[ 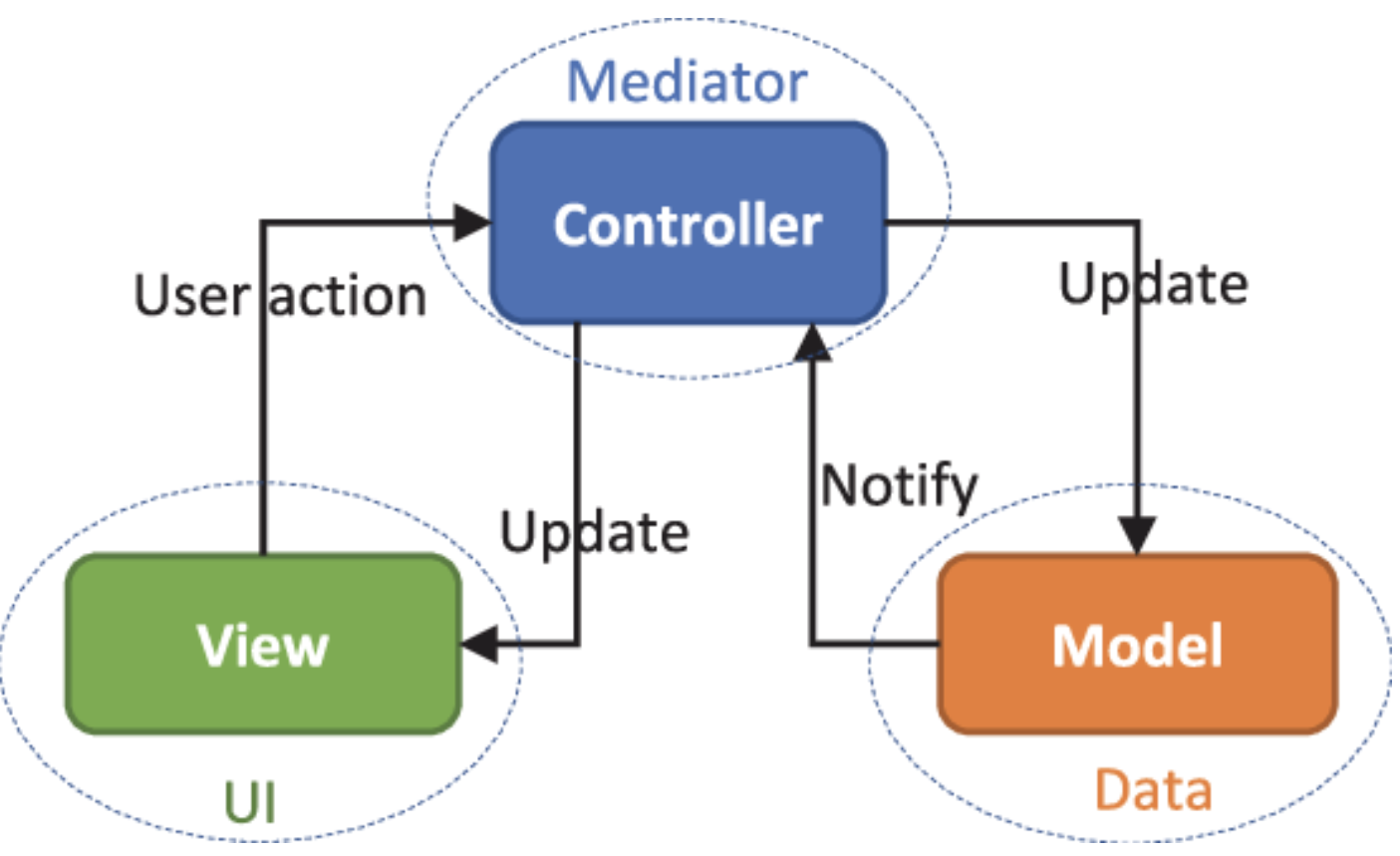 ] --- # Timeline 1. Accessibility assignment WITH REPORT due: Today, April 29th @ 10:00pm - Code Lock: Saturday, May 1st @ 10:00pm (if you are using late days) 2. Color picker due: in 2 weeks, May 13th @ 10pm - Code Lock/Reflection due Saturday, May 15th @ 10pm - Remember to turn in both your code, report, and video! --- # Section 5 Objectives - Model View Controller design - Example code, Simple Calculator App: https://tinyurl.com/y6cmkw7y - MVC in HW4? - Reviewing Event Record - Practice problems - Implementing callbacks and listeners - Homework 4 prep - Questions? HW help? --- # Model View Controller Review (1/3) - Architectural pattern used for developing user interfaces - App may be organized into the below 3 separate major logical components - **Model** = container of pure data - does NOT describe how to present the data - **View** = shows Model's data to user, the UI - **Controller** = mediates between View and Model - Handles event listening - Executes actions according to input - Manipulates data within the model, updates the View --- # Model View Controller Review (2/3) Example app: [Android Calculator App](https://gitlab.cs.washington.edu/cse340/exercises/mvc-calculator) - Note: the above app is written in Kotlin, but it is very similar to Java - Feel free to use this as a reference if you're curious about coding with Kotlin! - Briefly glance over the project or clone it. **What is the Model, View, and Controller in this case?** - Answers can be the general concept, don't have to specify X file --- # Model View Controller Review (3/3) Example answers: - **Model: CalculatorModel -> holds pure data the Calculator should store** - final calculation result - intermediate result (user has the option to edit their equation before submitting) - **View: activity_main.xml** - Calculator buttons... etc - **Controller: CalculatorActivity.java** - handles communication between View and Model --- # What would be the Model, View, Controller parts of HW4? (1/3) - Application Level MVC? - View/Component Level MVC? - Briefly glance at the spec and code base if haven't already --- # What would be the Model, View, Controller parts of HW4? (2/3) - Application Level - **Model**: ColorModel (located in AbstractMainActivity.java) - keeps track of color represented by int - can get/set the data (color) - this value is reflected across multiple views (the lower rectangle box, the picker... etc) - **View**: CircleColorPickerView, your custom color picker... etc - **Controller**: AbstractMainActivity and MainActivity.java - handle listeners - change the appearance of Views --> updateColor(int color), etc --- # What would be the Model, View, Controller parts of HW4? (3/3) - MVC WITHIN a View/Component (ex: CircleColorPickerView) - **Model:** mCurrentColor - **View:** the circles that are drawn out on the screen (center circle + thumb that you draw) + the white circle that is already defined in content_main.xml - **Controller:** the logical stuff inside CircleColorPickerView.java --- # Event Record Review (1/5) Event Record: your plan to capture information about a UI event - What (event type) - Where (input target) - When (time) - Value (XY location, which key, mouse coordinates...etc) - Context (Modifiers: CTRL, ALT... etc) --- # Event Record Review (2/5) **Example UI Event 1: User double taps to like a post on Instagram.** - If we were to represent this using an Event Record what would the record contain in terms of each category of recorded information? - Give hypothetical examples --- # Event Record Review (3/5) **UI Event 1: User double taps to like a post on Instagram, example answers** - What: Touch - Where: Image (probably a sub-component inside a “Post” object) - When: 2021-02-04-1234 (absolute time) - maybe even the time passed since the user opened the app or performed some action! - Value: XY location of tap - Context: Double-Tap --- # Event Record Review (4/5) **Example UI Event 2: On a smartphone, user presses the volume up button** - If we were to represent this using an Event Record what would the record contain in terms of each category of recorded information? --- # Event Record Review (5/5) **UI Event 2: Press volume up button** - What: Key down - Where: Physical volume button on phone - When: Absolute time (depending on what info you want to store) - Value: Volume level - Context: Depending on the phone, pressing the power button and volume up button at the same time (triggers a screenshot or soft resets phone) --- # Misinterpretations from Lecture: - Where: Some students thought this meant where in the code or what hardware was responsible. The 'where' is the part of the system that is going to receive the event. - From the 'Ok Google' example from this https://edstem.org/us/courses/4985/lessons/13332/slides/66843 ed lesson, it was the subsystem in the OS that takes in voice commands. - Value: This is different from the 'what'. Think of it as how you can measure the input. - From the 'Ok Google' example, this would be the type of command you are saying after prompting 'OK Google'. - Context: Last time we didn't ask about context. Context is the modifiers, or things that would change the nature of the event. For example, pressing the volume button is an event, but is this in the context of turning up the volume or in the context of taking a screenshot? Pressing the power button alongside the volume would modify the context of this event. --- # Section Exercise: - Given what you know now about Event Records, come up with your own event record and list its what, where, when, value, and context. - https://edstem.org/us/courses/4985/lessons/12619/slides/64006 --- # Callback and Listeners - **Callback**: method gets called by the Android framework when event happens and is delivered to a component that is registered to listen for the event - Ex: animal enclosure example in lecture --> makeNoise() - **Event Listeners**: type of event handling callback - Example Higher level events (already provided for you by Android View, based on Touch Events) - onClickListener, onFocusListener, onDragListener... - Override these to modify what happens in response to such events Can also define [custom listeners](https://guides.codepath.com/android/Creating-Custom-Listeners) that are triggered based on how you define it (ex: trigger upon HTTP GET request is successful and data loaded) --- # onClick vs onTouch - [onTouchListener](https://developer.android.com/reference/android/view/View.OnTouchListener?authuser=1) = allows you to control what happens in terms of different MotionEvents - Ex: ACTION_UP, ACTION_DOWN, ACTION_MOVE.. And many more! - [onClickListener](https://developer.android.com/reference/android/view/View.OnClickListener) = for when user clicks the view - used for a more basic purpose - cannot handle MotionEvents like onTouch - Android docs: Handling a click event of a Button [programmatically](https://developer.android.com/guide/topics/ui/controls/button#ClickListener) --- # HW4 Colorpicker (1/3) - Inside AbstractColorPickerView... - Has an interface (contract): what should happen when X event happens? ```java public interface ColorChangeListener { void onColorSelected(@ColorInt int color); } ``` - Ability to register a listener to self (and remove listener) ```java public final boolean addColorChangeListener(...) ``` --- # HW4 Colorpicker (2/3) - Inside AbstractColorPickerView... - Event handling - onTouchEvent itself is automatically triggered by the system - However we need to handle what happens depending on given MotionEvent - When to actually trigger the color change listener? Read the spec to find out! ```java @Override public boolean onTouchEvent(MotionEvent event) { ... ``` --- # HW4 Colorpicker (3/3) - Activity that implements the interface ```java public class MainActivity extends AbstractMainActivity implements AbstractColorPickerView.ColorChangeListener { ... @Override public void onColorSelected(int color) { // change how your views look with the given int color } ``` - Inside MainActivity, you should register all color pickers to "listen in" to ColorChangeListener - That way, when the onTouchEvent in AbstractColorPickerView is called and possibly triggers ColorChangeListener, the views will update accordingly. --- # In summary... for Colorpicker 1. Handle view layout, draw the circles...etc in **CircleColorPickerView.java** 2. Handle triggering the color change listener via onTouchEvent in **AbstractColorPickerView.java** 3. **MainActivity.java**: register listeners, define reaction behavior onColorSelected. Also save the state of the app in (we'll go over bundles in more detail next week) ```java @Override public void onSaveInstanceState(Bundle outState) { // save to your bundle --> [get your color from the Model] // See AbstractMainActivity for the Color Model } ``` 6. Make your own color picker in **MyColorPickerView.java** 7. **REFLECTION** (don't forget about this!!!)