name: inverse layout: true class: center, middle, inverse --- # Introduction to Drawing Interfaces Jennifer Mankoff CSE 340 Winter 2020 --- layout: false # Let's make an interactor hierarchy .left-column-half[ 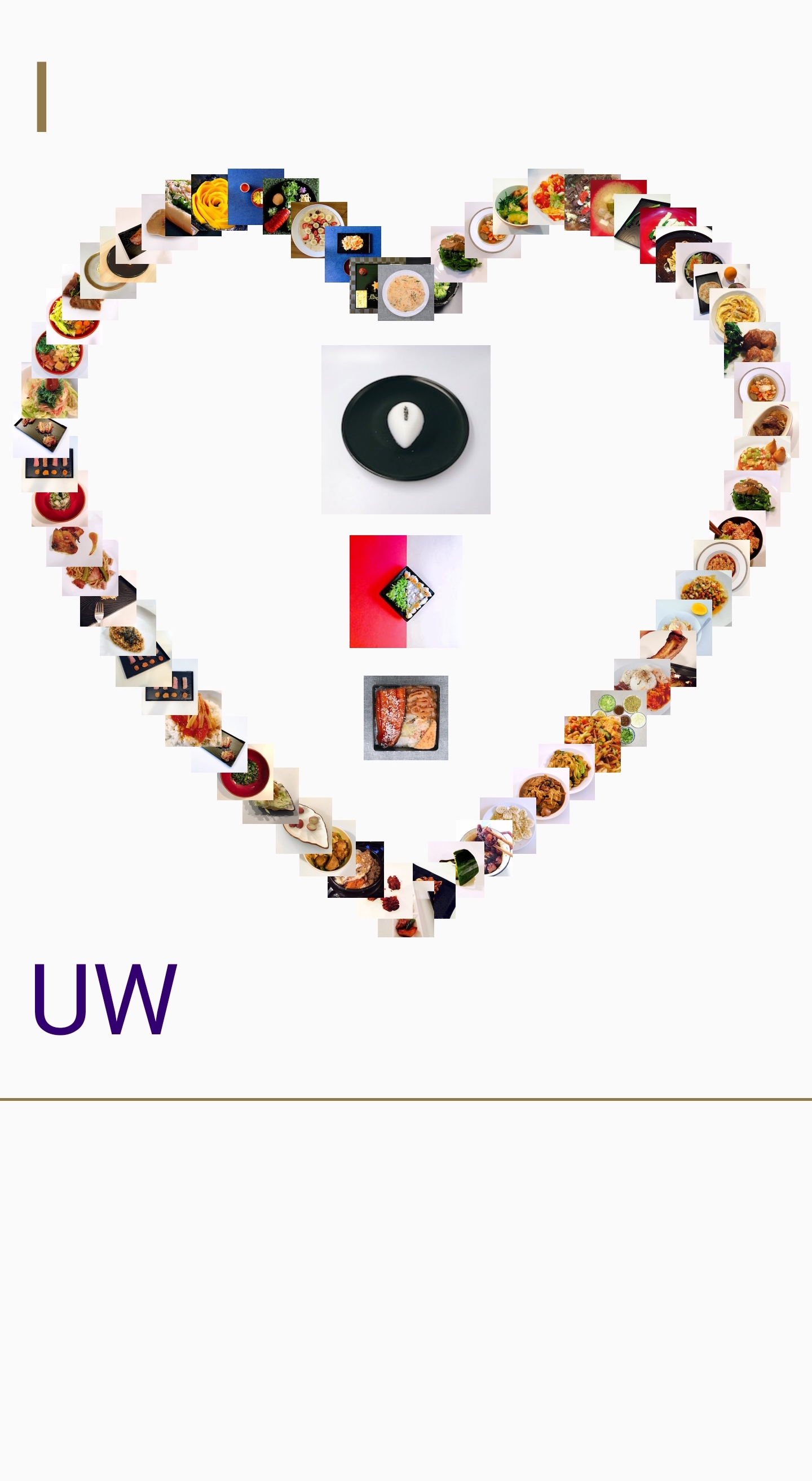 ] .right-column-half[ Note that we use a `View` for **each** thing on the screen ] --- # Why so many separate `Views`? Provides **separation of concerns** and good high level control - Can have a different paint object for each view - Can easily change z-order (what is on top of what) - Can store information used to draw that thing (whatever it is) Views can also be re-used easily ??? This could be a good place to ask them to think about what that means and demonstrating two things with different z order. --- [//]: # (Outline Slide) # Today's goals .left-column-half[
] .right-column-half[ Doodle Spec Update - Do change LineView - Progressive Handin: Code; Peer Eval; Reflection Outline - Role of Interactor Hierarchy in drawing the screen - How to use Animation to move Interactors - Summary of what we've learned so far ] --- # Simpler Example **Interface Programmer** .left-column[ 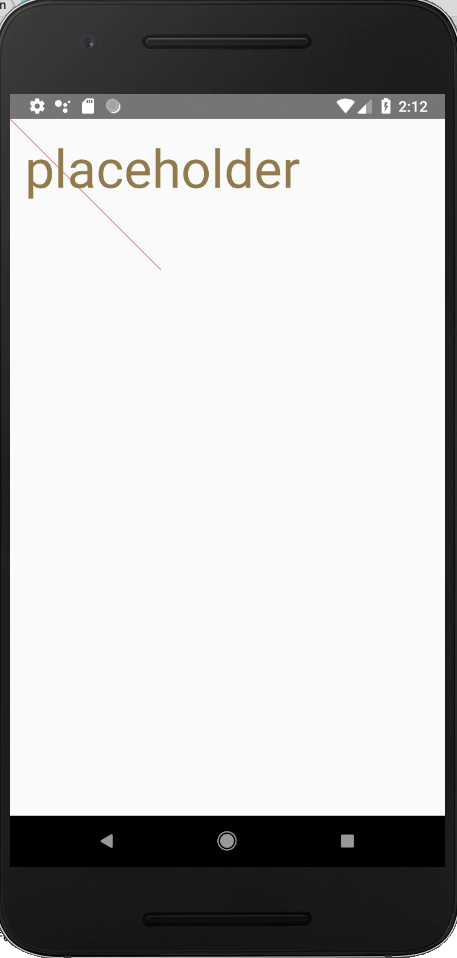 ] .right-column[ ```java LineView line = new LineView(this, startX, startY, endX, endY, width, color); canvas.addView(line); TextView text = new TextView(this); text.setX(x); text.setY(y); text.setText("placeholder"); canvas.addView(text); ``` ] --- # Review: Roles
graph LR ip[Interface Programmer] w[Component Developer] l[Library Extender] a[Architecture Extender] t[Toolkit Builder] class t green class w,l,a green class ip green
--- # What is a toolkit builder anyway? Example: Physical Interfaces Creates entirely new toolkits that changes what we can do ![:youtube Physical Interface made out of RFID tags,4k15uXpp7-g] ??? But there was once a time when ui toolkits didn't exist. How are THEY built? --- # What is a toolkit builder anyway? Or could be the person who made your user interface toolkit with its Library and Architecture --- # What is the toolkit library? ??? Example: All the different types of Views you can play with What could you add to a toolkit library? May create new forms of layout, types of input, etc. Supported by a few toolkits --- # What is the toolkit library? Example: All the different types of Views you can play with What could you add to a toolkit library? May create new forms of layout, types of input, etc. Supported by a few toolkits --- # Toolkit Support for Styling .left-column-half[ 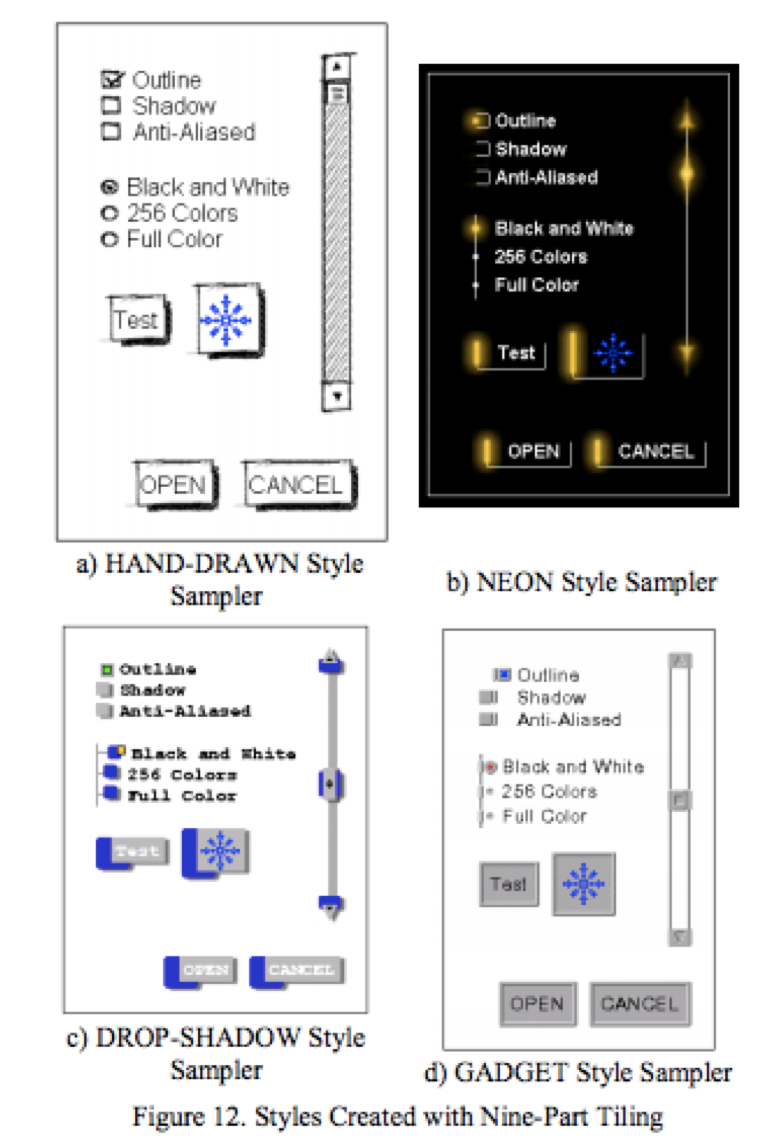 ] .right-column-half[ subArctic allowed visually rich, dynamically resizable, images to be provided using primarily conventional drawing tools (and with no programming or programming-like activities at all). Scott E. Hudson and Kenichiro Tanaka. (UIST '00) ] ??? This is in android now, views can be specified with 9 separate pieces for scalable styling --- # What is the toolkit architecture? ??? Applies to the flow of information within the toolkit Example: How the interface is drawn What could you add? Support for animation, machine learning, etc Supported by very few toolkits --- # What is the toolkit architecture? Applies to the flow of information within the toolkit Example: How the interface is drawn What could you add? Support for animation, machine learning, etc Supported by very few toolkits ... back to our regularly scheduled program --- # How is a View drawn on the screen? **Toolkit Architecture Builder** - Depth-first tree traversal of the interactor hierarchy - Each View draws itself in its location - Siblings drawn in order they appear Naive Pseudocode: ```java protected void drawAll() { foreach child c { if (child.isVisible()) { child.drawAll(); } onDraw(); } } ``` ??? What is missing here? How can children misbehave? What are they expecting that we don't do? Draw out what will happen on paper --- # Work arounds? ??? Have them brainstorm --- # Work arounds? Make the **Interface Programmer** fix this Could make every view the size of the whole screen; Then just draw in the right position Could tell every view where it is so it draws properly --- # For this, view needs a position, width and height .left-column-half[ 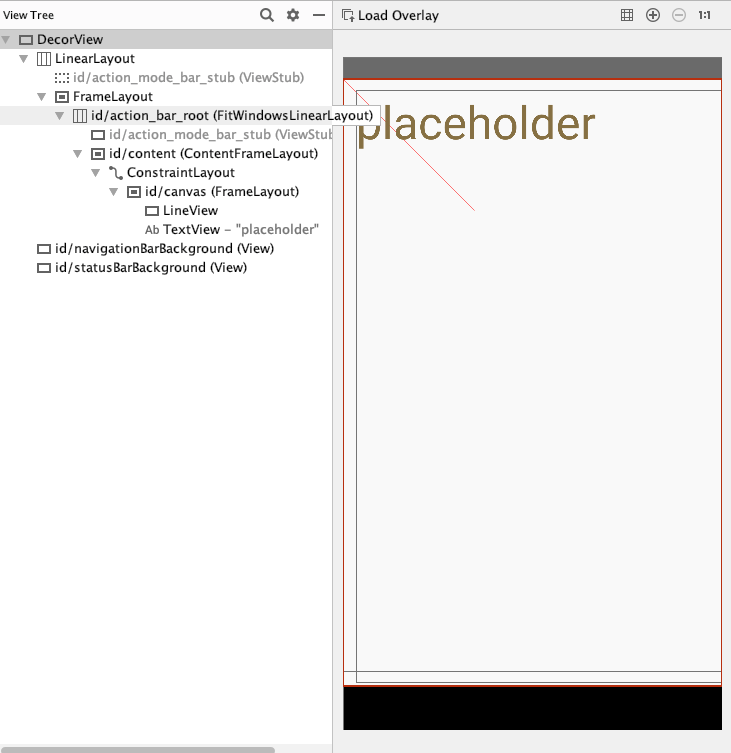 ] .right-column-half[ What could go wrong with this approach? ] ??? - What if we want to place a different view, automatically, to the right of the text? - How do we guarrantee that a view doesn't draw over it's neighbor? --- # For this, view needs a position, width and height .left-column-half[ 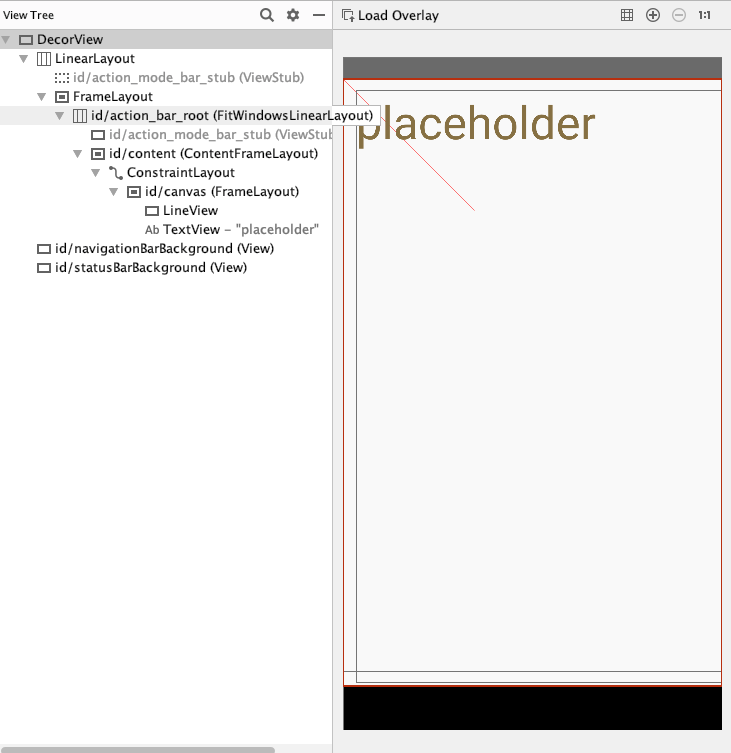 ] .right-column-half[ What could go wrong with this approach? - What if we want to place a different view, automatically, to the right of the text? - How do we guarrantee that a view doesn't draw over it's neighbor? ] --- # How do we specify View position, width and height? **Interface Programmer** can do this .left-column-half[ 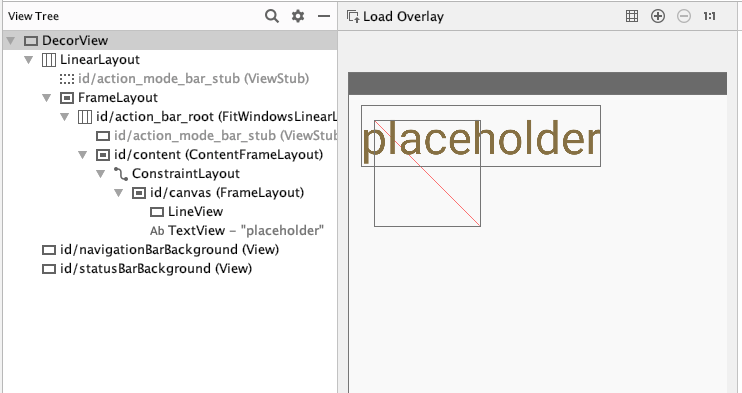 ] .right-column-half[ ```java View view = new View(); view.setX(); view.setY(); ViewGroup.LayoutParams params = view.getLayoutParams(); param.width = width; param.height = height; view.setLayoutParams(param); ``` ] ??? We'll go into much more depth on this in your next assignment --- # How does a toolkit use this? **Toolkit Architecture Builder** **Uses coordinate transformations to make each child the center of its universe!** - Parent (usually) specifies position of child when adding to the interactor hierarchy - Child counts on parent to set things up so child's internal coordinate system starts at (0,0) --- # How is a View drawn on the screen **Toolkit Architecture Builder** ```java protected void drawAll() { foreach child c { if (child.isVisible()) { child.drawAll(); } onDraw(); } } ``` Is this part of the _Toolkit Architecture_ or _Toolkit Library_? --- # How is a View drawn on the screen **Toolkit Architecture Builder** ```java protected void drawAll() { foreach child c { if (child.isVisible()) { * Rectangle r = child.getLayoutParams(); // Find child coordinates * canvas.save(); // Capture the current state of canvas * canvas.translate(r.x, r.y); // Move origin to child's top-left corner * canvas.clip(0,0,r.width,r.height); // Clip to child child.drawAll(); // Draw child (and all it's kids) * canvas.restore(); // Restore } onDraw(); } } ``` Is this part of the _Toolkit Architecture_ or _Toolkit Library_? ??? Draw out on paper again Note that this is has absolute layout! --- [//]: # (Outline Slide) .left-column[# Today's goals] .right-column[ - Role of Interactor Hierarchy in drawing the screen - How to use Animation to move Interactors - Summary of what we've learned so far ] --- # [ObjectAnimator](https://developer.android.com/reference/android/animation/ObjectAnimator) .left-column[ 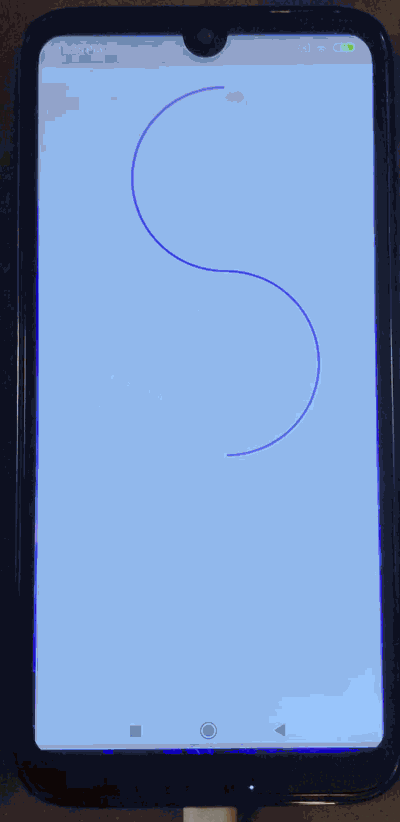 ] .right-column[ Example animation of position (using a [Path](https://developer.android.com/reference/android/graphics/Path)) ```java // takes a target view, a property name, and values ObjectAnimator anim = ObjectAnimator.ofFloat(foo, View.X, View.Y, path); anim.setDuration(1000); ImageView mouse = addImage(mainCanvas, "mouse", 500f, 50f, size); Path path = new Path(); path.moveTo(400f, 50f); path.arcTo(200f, 50f, 600f, 450, 270, -180, true); path.arcTo(200f, 450f, 600f, 850, 270f, 180f, true); ObjectAnimator anim = ObjectAnimator.ofFloat(mouse, View.X, View.Y, path); anim.setDuration(10000); anim.start(); ``` ] --- # Why is the bounding box important here? 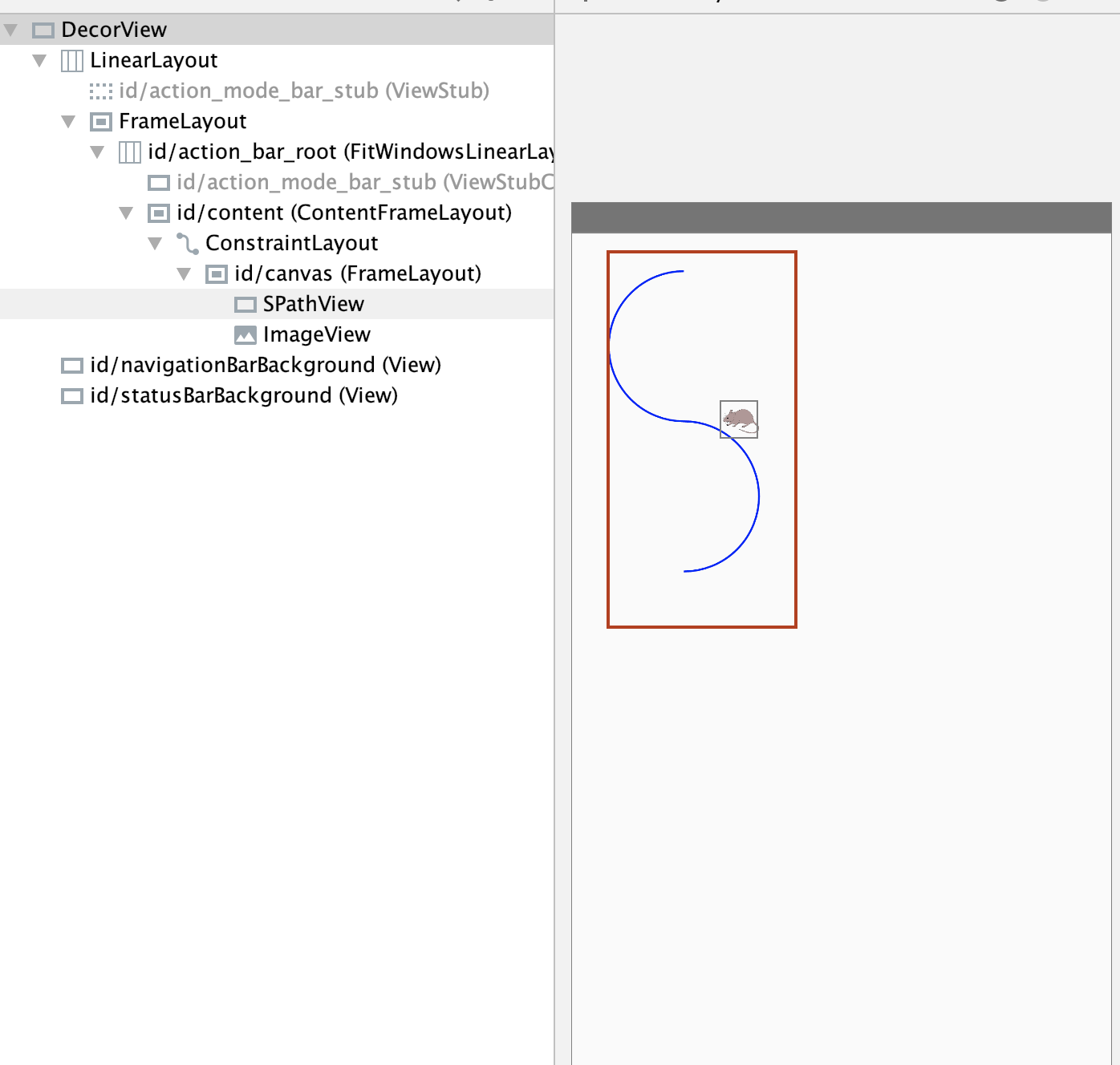 --- # [ObjectAnimator](https://developer.android.com/reference/android/animation/ObjectAnimator) Can also directly animate a properties on an object **However**: the property that you are animating must have a setter function (in camel case) in the form of set
() for this to work Can use a string (like `"alpha"`) or a property (like `View.X` in [View](https://developer.android.com/reference/android/view/View)) --- # Useful properties for animation - `translationX` /`translationY` - view location as a delta from its top/left coordinates relative to the parent - `rotation` / `rotationX`/`rotationY` - control 2D rotation and 3D rotation around a pivot point - `scaleX` / `scaleY` - 2D scaliong of a `View` around a pivot point - `pivotX` / `pivotY` - changes location of thej pivot point (default is object's center) - `x` / `y` - utility property to describe the final location of a `View` in its container as a sum of (left, top) + `translationX`, `translationY`) - `alpha` .footnote[All found in the [View](https://developer.android.com/reference/android/view/View) class] --- # [ObjectAnimator](https://developer.android.com/reference/android/animation/ObjectAnimator) .left-column[
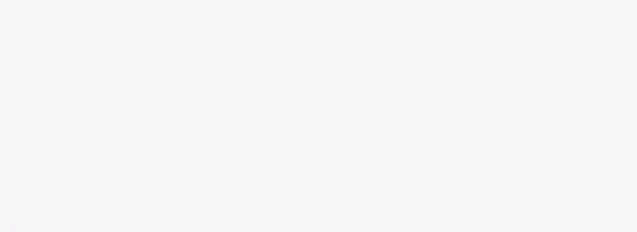 ] .right-column[ Example for color ```java // takes a target view, a property name, and values ObjectAnimator anim = ObjectAnimator.ofFloat(foo, "alpha", 0f, 1f); anim.setDuration(1000); anim.start(); ``` ] --- # Taking Animation to the next level .quote[ Despite the differences between user interfaces and cartoons -- cartoons are frivolous, passive entertainment and user interfaces are serious, interactive tools -- cartoon animation has much to lend user interfaces to realize both affective and cognitive benefits] .small[Principals of animation borrowed from Johnston & Thomas ‘81, Lasseter ‘87] --- # Chang & Ungar, UIST '93 ![:youtube Opening cartoon from who framed roger rabbit showing him trying to keep a baby safe,oQe0OWZvwWo] What strategies do you see here? ??? - Squash-and-Stretch - Staging, Overlapping Action - Anticipation - Follow-Through - Slow In Slow Out --- ## Example pacing functions -- derived from Disney style animation! .left-column[ 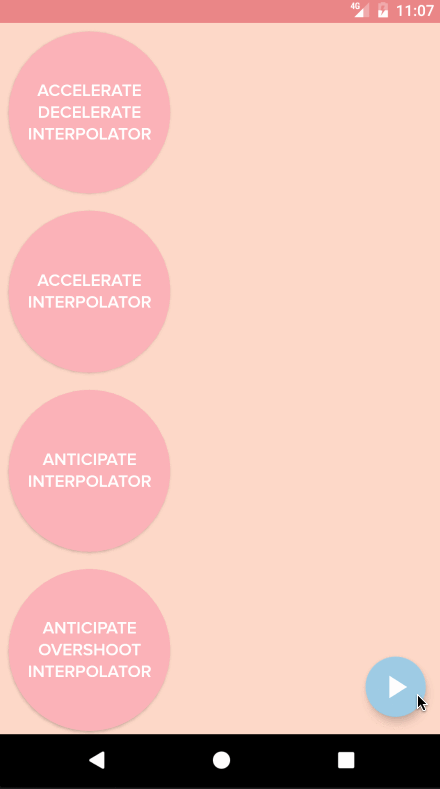 ] .right-column[ Slow in slow out (Accelerate/decelerate)
Slow in (Accelerate)
Anticipate (back up slightly, then accelerate)
Anticipate Overshoot (same, then go too far and slow down) ] --- # Do you see them here? ![:youtube Opening cartoon from who framed roger rabbit showing him trying to keep a baby safe,oQe0OWZvwWo] What strategies do you see here? ??? - Squash-and-Stretch - Staging, Overlapping Action - Anticipation - Follow-Through - Slow In Slow Out --- # Why is pacing so important? Need to mimic real world - Observing motion tells us about size, weight, rigidity - No abrupt changes in velocity! Gives a feeling of reality and liveness - “animation” = “bring to life” - make inanimate object animate With this can come appeal and desirability --- # How do we use these for animation? How would `t` and `x` change for slow in slow out? 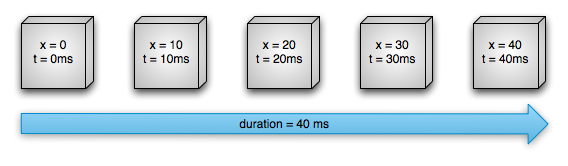 .footnote[[image source: Android animation documentation](https://developer.android.com/guide/topics/graphics/prop-animation)] --- # We implement these with `Interpolators` in Android .left-column-half[ 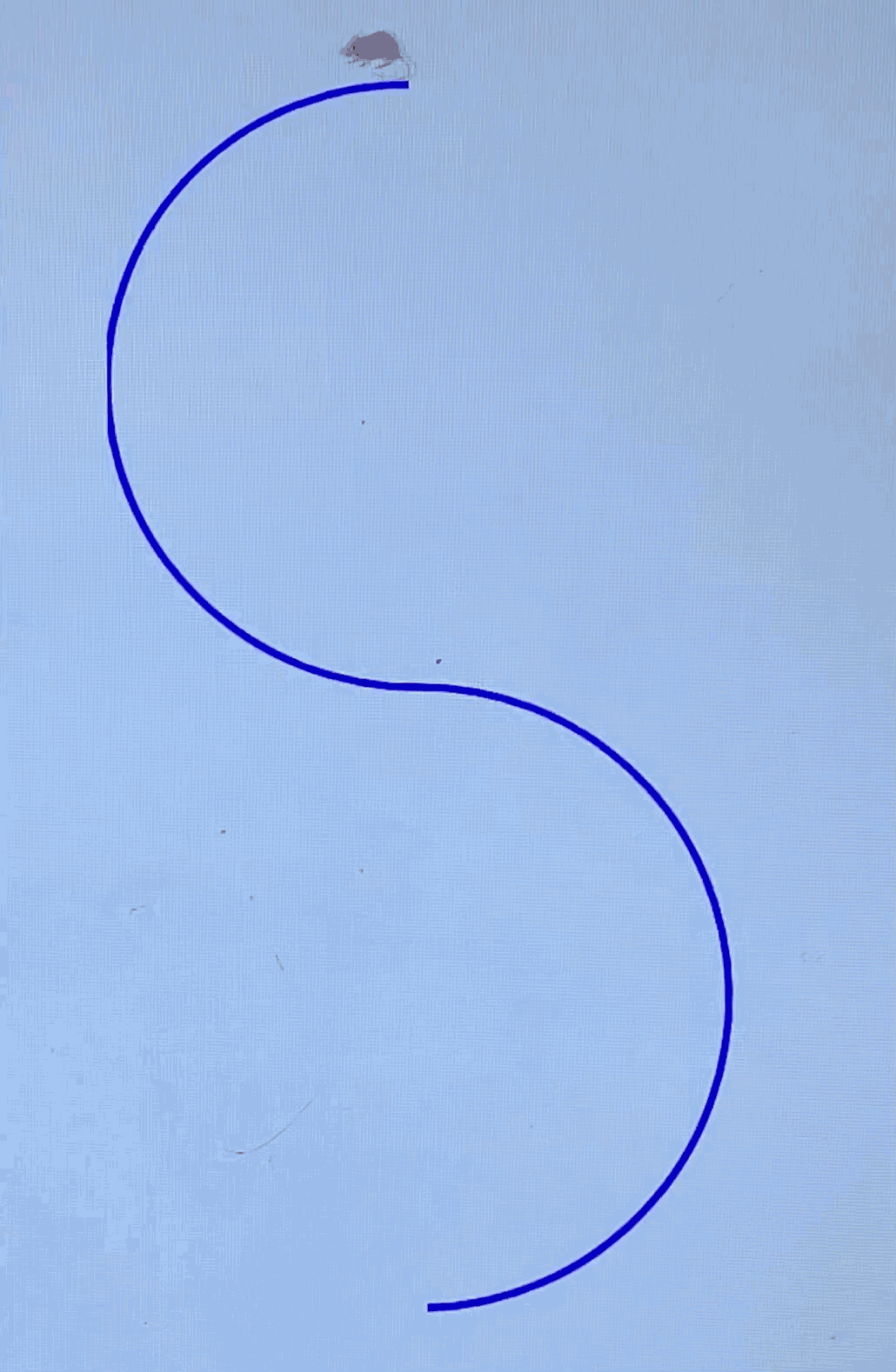 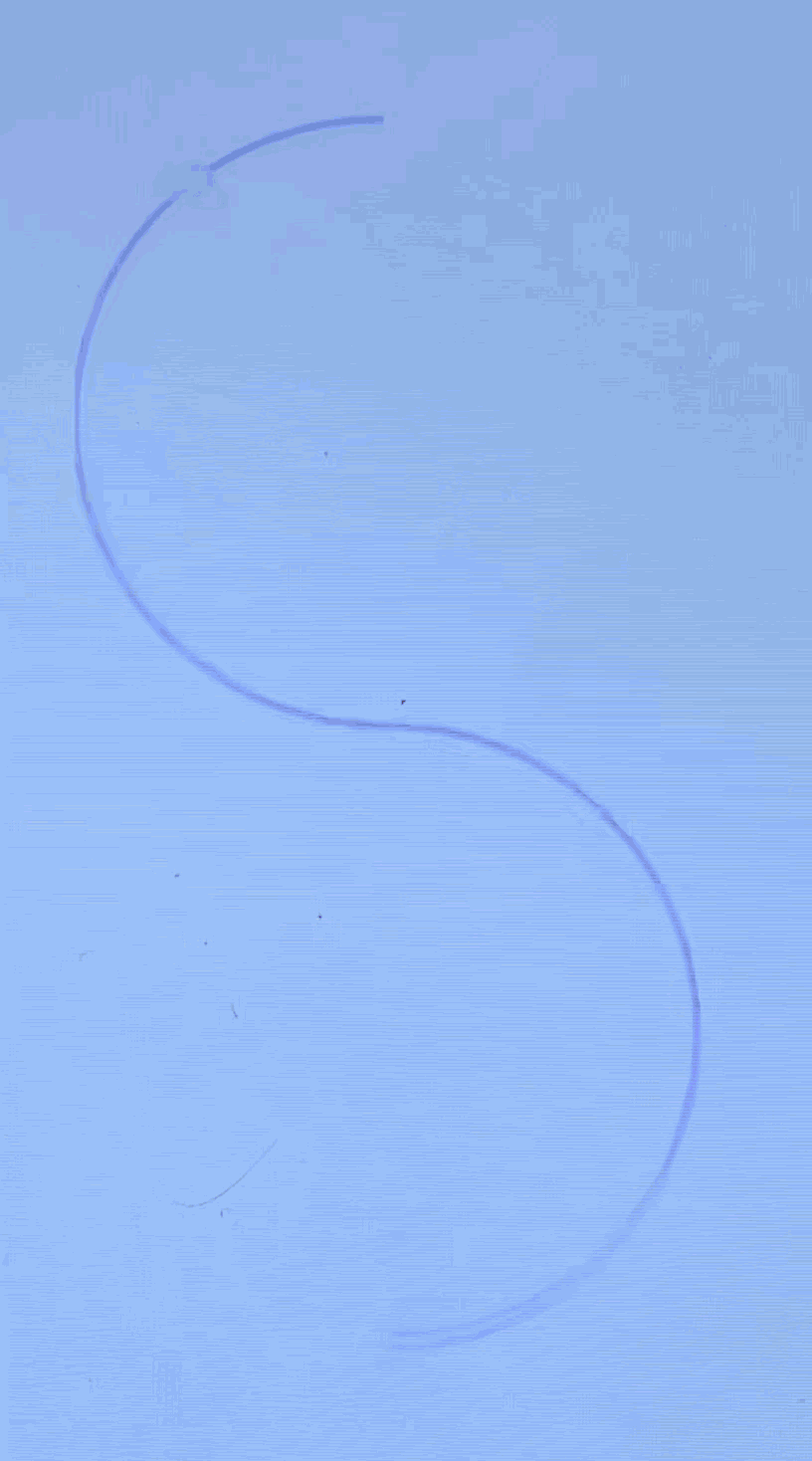 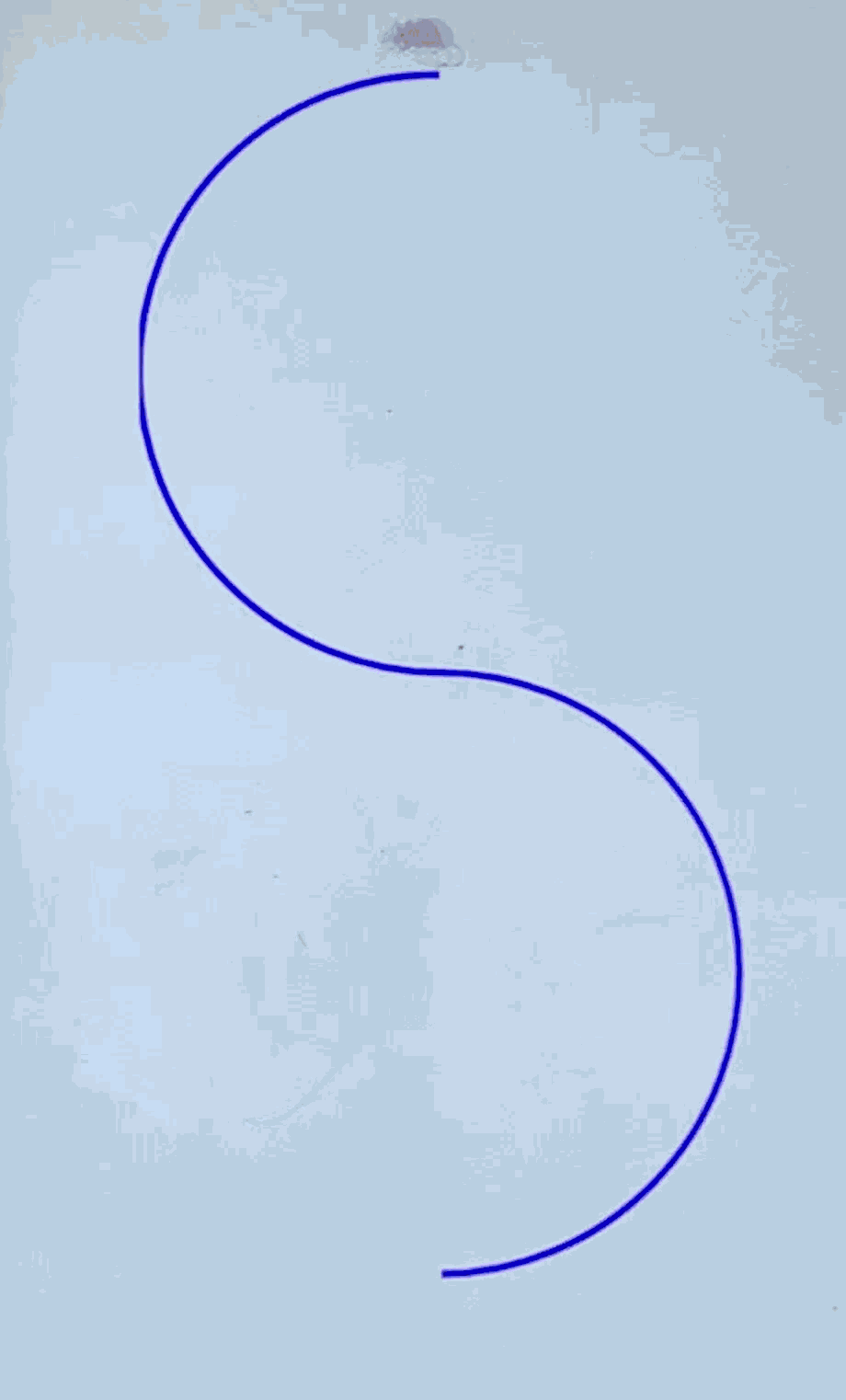 ] .right-column-half[ Left side is _Linear_ Middle is ?? Right side is ?? Durations are the same ```java ObjectAnimator anim = ObjectAnimator.ofFloat(mouse, View.X, View.Y, path); * OvershootInterpolator interpolator = * new OvershootInterpolator(); * anim.setInterpolator(interpolator); anim.setDuration(3000); anim.start(); ``` ] ??? overshoot --- # Summary & revisiting learning goals for this week and last
??? --- # Summary & revisiting learning goals for this week and last .left-column-half[ - What is HCI? - What is a toolkit? How do an architecture and library differ? - How do we draw on the screen? - What is a pixel? - What is a raster vs a vector model? - What abstractions help with drawing? - How do we use affine transformations? - What is the interactor hierarchy? - How is it used for drawing? - What role do affine transformations play in this? - What are the key abstractions for animation? ] .right-column-half[ - Android basics - What is a `View`? - `onCreate()` and `onDraw()` - How does `Canvas` work? - How do we construct the interactor hierarchy? - Implementing Animation ]