# CSE 340 Lab 3 (Winter 2020) ## Week 3: Layout .title-slide-logo[ 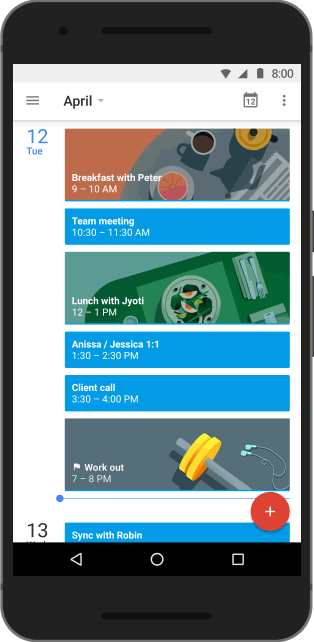 ] --- # Timeline - Layout part1-2 due: Yesterday (But you can still use the late days) - Layout part3-4 due: Jan 31 (Next Friday) - Reflection due: Feb 2 --- # Reflection? - What did you learn from doing Part4? - Was there anything that was surprisingly easy or hard? - Include your diagram of the interactor hierarchy and the corresponding interface. --- # Interactor Hierarchy What would be the Interactor Tree for the Layout Part1/2 program? .left-column-half[ 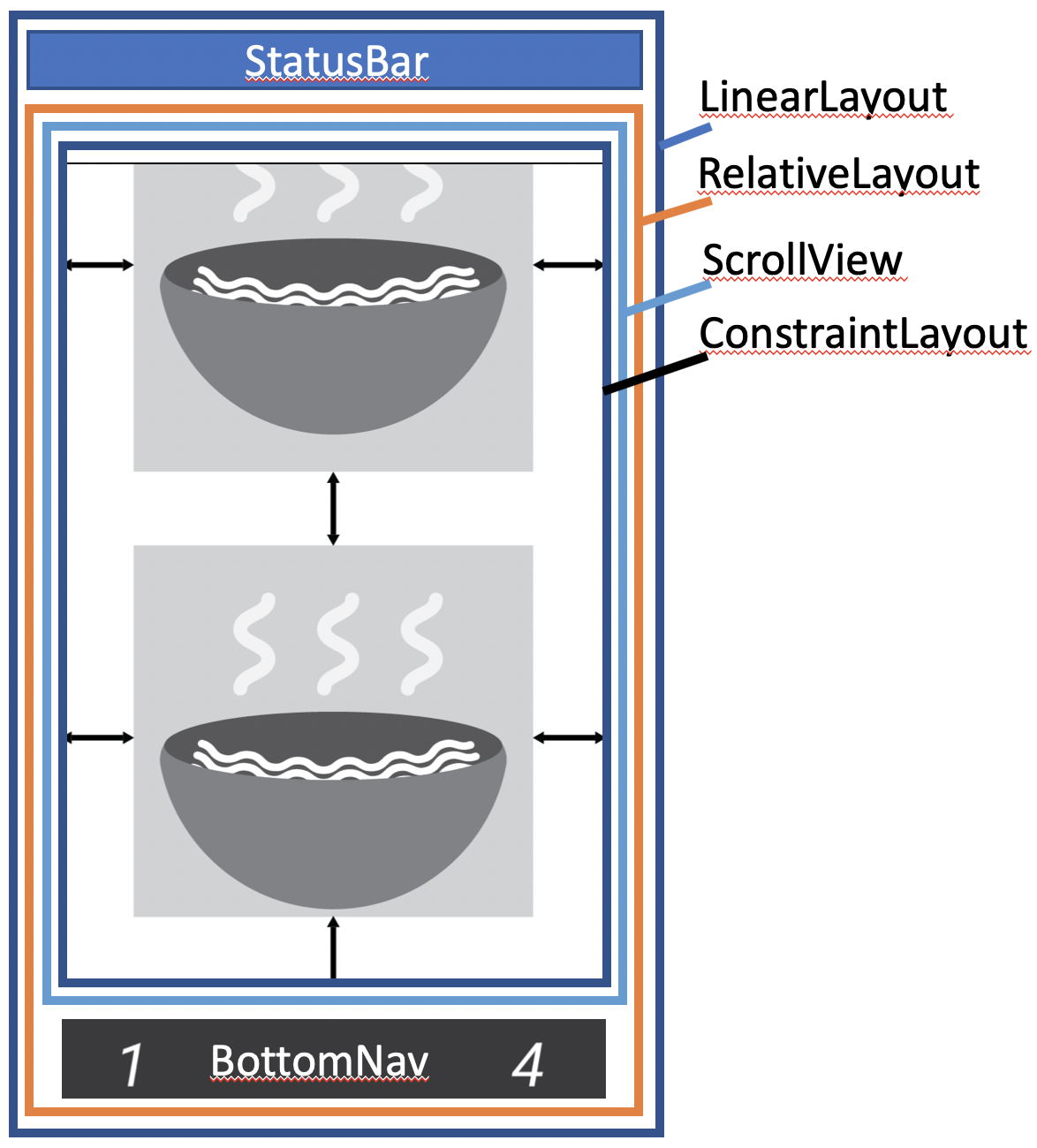 ] -- .right-column-half[
graph TD LL(LinearLayout) --> S[StatusBar] LL --> RL[RelativeLayout] RL --> SV[ScrollView] RL --> BN[BottomNav] RL --> CL[ConstraintLayout] CL --> V2[ImageView] CL --> V3[...] CL --> V4[ImageView] class LL,RL,SV,CL darkblue class S,SV,BN,V2,V3,V4 blue
] --- # Instruction for turning in Layout part 3-4 - **(Important!)** You will have to accept the Layout part3-4 at gitgrade - Before you turn in: https://gitgrade.cs.washington.edu/student/summary/8086 - Work in the same repo as part1-2 - More information on piazza post: https://piazza.com/class/k2verexa56l5y3?cid=92 --- # Section 3 Overview - `LayoutInflater` - Previous exam problem of Layout --- # What is LayoutInflater? --- # LayoutInflater Accepts a valid XML file and converts it into a `View` object or interactor hierarchy. In part3 you will code half of it in an xml file and half of it in java. Why? (Think about pros and cons of constructing with xml and java) --- # Xml vs. Java .left-column-half[ - Xml is easier setting up the Layouts - Xml shows the Layouts directly - Xml can be inflated many times ] .right-column-half[ 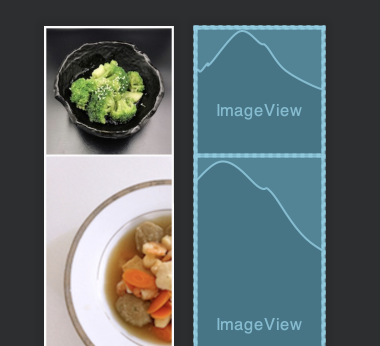 ] --- # Xml vs. Java - Java is easier adding items to the Layouts - Java can use loops - Sample solution of adding items into Layouts in part1(xml) has 55 lines - Sample solution of adding items into Layouts in part2(java) has 17 lines --- # How do we use the LayoutInflater? 1. Setting up the LayoutInflater ```java LayoutInflater.from(Context context) // Obtains the LayoutInflater from the given context ``` 2. Inflate ```java inflate(int resource, ViewGroup root) // Inflate a new view hierarchy from the specified xml resource ``` --- # Quick Exercise Construct a LayoutInflater and pass in the `part1.xml` file. --- # Quick Exercise Solution ```java // Obtain the inflater from the context LayoutInflater inflater = LayoutInflater.from(context); // Inflate R.layout.part1 and add to this vie inflater.inflate(R.layout.part1, this); ``` --- # Previous Exam Question --- # Solution **What is the basic idea you have for how to fix it?** > Constrain the top of the scroll to the bottom of the text view. **Which view would you need to modify (provide the value you would set `android:id` to)?** > `@+id/scrollView` **What one line of XML would you add? Pseudocode ok here, you don't have to use exact names.** > `app:layout_constraintTop_toBottomOf="@id/textView"`