# CSE 340 Lab 9 (Spring 2020) ## Week 9: Getting started with Undo .title-slide-logo[ 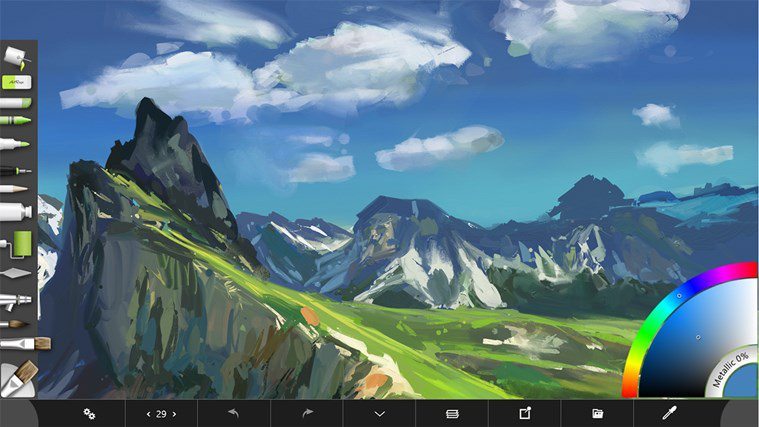 ] --- # Undo Timeline - Programming part due: Next Monday, June 1 @ 10:00pm - Lock: Wednesday, June 3 @ 10:00pm (if you are using late days) - Heuristic Evaluation due: Friday, June 5 @ 10:00pm - Reflection due: Monday, June 8 @ 10:00pm - **Examlet 4 is tomorrow!** Friday, May 29 --- # Section 9 Objectives - Understanding Undo assignment - Examlet review with Kahoot - Work time/ Questions on Undo assignment --- # The Undo feature - Incredibly useful interaction technique - Reverts the application to an older state - Mistakes can easily be undone - *"Ugh...that was definitely not right, but manually erasing all this work is going to take forever"* --- # The Redo feature - Inverse to the Undo feature - "Undoes" an undo - Work previously undone is reapplied - *"Huh, maybe I made the right decision after all..."* --- # Codebase: Application .left-column60[ - `ReversibleDrawingActivity`, class contains the entire interactor hierarchy - Floating Action Buttons (FAB), buttons that allow for actions supported by AbstractReversibleDrawingActivity - References DrawingView in AbstractDrawingActivity - `AbstractReversibleDrawingActivity`, abstract class that extends AbstractDrawingActivity - adds support for undo/redo - includes buttons for undo/redo - `doAction()`, `undo()`, `redo()`] .right-column30[
classDiagram AbstractDrawingActivity <|.. AbstractReversibleDrawingActivity AbstractReversibleDrawingActivity <|.. ReversibleDrawingActivity class AbstractDrawingActivity { DrawingView: "Canvas the user draws on" addMenu() addCollapsableMenu() doAction() } class AbstractReversibleDrawingActivity { +AbstractStackHistory +doAction() +undo() +redo() } class ReversibleDrawingActivity { +onAction() +onColorSelected() +onThicknessSelected() }
] --- # Codebase: Application .left-column60[ - `AbstractDrawingActivity` - abstract class for app that supports drawing without history - Wrapper around a `DrawingView` - `doAction()` - Contains methods for adding menus and changing visibility] .right-column30[
classDiagram AbstractDrawingActivity <|.. AbstractReversibleDrawingActivity AbstractReversibleDrawingActivity <|.. ReversibleDrawingActivity class AbstractDrawingActivity { DrawingView: "Canvas the user draws on" addMenu() addCollapsableMenu() doAction() } class AbstractReversibleDrawingActivity { +AbstractStackHistory +doAction() +undo() +redo() } class ReversibleDrawingActivity { +onAction() +onColorSelected() +onThicknessSelected() }
] --- # Codebase: DrawingView .left-column-half[- `AbstractDrawingActivity` is a wrapper around a `DrawingView` - Sets behavior for how strokes are drawn - DrawingView, a drawing canvas that handles strokes - Strokes are just `StrokeView`'s in the `DrawingView` - `onTouchEvent()` implements a PPS that describes lifetime of a stroke being created] .right-column40[
classDiagram class AbstractDrawingActivity { DrawingView: "Canvas the user draws on" addMenu() addCollapsableMenu() doAction() } class StrokeView { Path onDraw() }
] --- # Codebase: Actions .left-column-staff[ - `AbstractAction` abstract class defines basic behavior any Action should have - `AbstractReversibleAction` abstract class defines interface for actions that can be undone - `doAction()` chain: `AbstractReversibleDrawingActivity.doAction()` ➡`AbstractDrawingActivity.doAction()` ➡`AbstractAction.doAction()` - `AbstractReversibleDrawingActivity`'s `undo()` and `redo()` call methods on `AbstractReversibleAction` to undo/redo] .right-column55[
classDiagram AbstractAction <|.. AbstractReversibleAction AbstractReversibleAction <|.. ChangeColorAction AbstractReversibleAction <|.. ChangeThicknessAction AbstractReversibleAction <|.. AbstractReversibleViewAction AbstractReversibleViewAction <|.. StrokeAction class AbstractAction { doAction() } class AbstractReversibleAction { +boolean done +undoAction } class AbstractReversibleViewAction { +invalidate }
] --- # Codebase: Actions .left-column-staff[ - Actions: - `ChangeColorAction` - `ChangeThicknessAction`, implement for homework - `StrokeAction` - And more, create your own action!] .right-column55[
classDiagram AbstractAction <|.. AbstractReversibleAction AbstractReversibleAction <|.. ChangeColorAction AbstractReversibleAction <|.. ChangeThicknessAction AbstractReversibleAction <|.. AbstractReversibleViewAction AbstractReversibleViewAction <|.. StrokeAction class AbstractAction { doAction() } class AbstractReversibleAction { +boolean done +undoAction } class AbstractReversibleViewAction { +invalidate }
] --- # Codebase: History .left-column60[The `AbstractReversibleDrawingActivity` uses an StackHistory interface to manage the undo/redo history - You will implement the concrete `StackHistory` which implements `AbstractStackHistory` - Think: What data structures are used? Why does this make sense? - What happens to the redo history if you take a new action after undoing a couple times? - What happens to the undo history if you redo an action? - What happens to the redo history if you undo an action?] .right-column30[
classDiagram AbstractStackHistory <|.. StackHistory class AbstractStackHistory { addAction(AbstractReversibleAction action) undo() redo() canUndo() canRedo() } class StackHistory { +capacity: "Max stack size" }
]