# CSE 340 Lab 3 (Spring 2020) ## Week 3: Layout .title-slide-logo[ 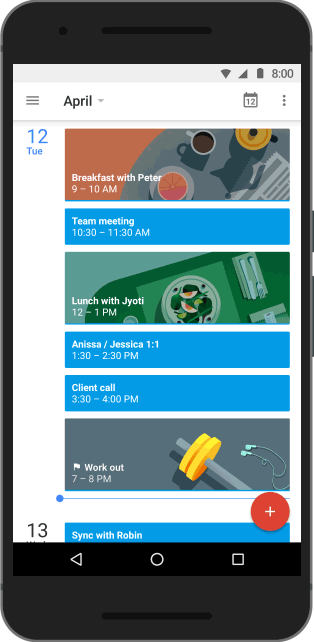 ] --- # Layout Timeline - Layout part 1-2 due: Tomorrow @ 10:00pm (No late days allowed) - Layout part 3-4 and Reflection due: Next Thursday, April 23 @ 10:00pm - Lock: Saturday, April 25 @ 10:00pm (If you are using late days) --- # Instruction for turning in Layout part 3-4 - **(Important!)** You will have to accept the Layout Part 3-4 at Gitgrade before you turn in - Track your acceptance/ submission of all assignments: https://gitgrade.cs.washington.edu/student/summary/8723 - Work in the same repo as Part 1-2 --- # Section 3 Objectives - User Interfaces on Android - ConstraintLayout - Interactor Hierarchy - `LayoutInflater` - XML vs. Programmatic (Java) - Previous exam problem of Layout - Worksheet: https://tinyurl.com/cse340lab3 --- # User Interfaces on Android .left-column-half[ - Views - Base class for __all__ UI elements - Interactors (e.g buttons, labels, image views, etc) - ViewGroups - Encapsulates one or more views (e.g. Android Components, **Layouts**) - Can define specific **layout** properties - Layout - Defines the structure for the user interface (UI) of your app - View and ViewGroup objects live within Layouts - We will use the word *Components* to include both layout components and interactors (Views) since you don't generally "interact" with layouts ] .right-column-half[
graph TD W(ViewGroup) --> V[ViewGroup] W --> V1[View] W --> V2[View] V --> V3[View] V --> V4[View] V --> V5[View] classDef blue font-size:14pt,text-align:center classDef darkblue font-size:14pt,text-align:center class W,V darkblue class V1,V2,V3,V4,V5 blue
] --- # Layout in Android Where we left off: "Many layout and other attributes for components. You should explore!" 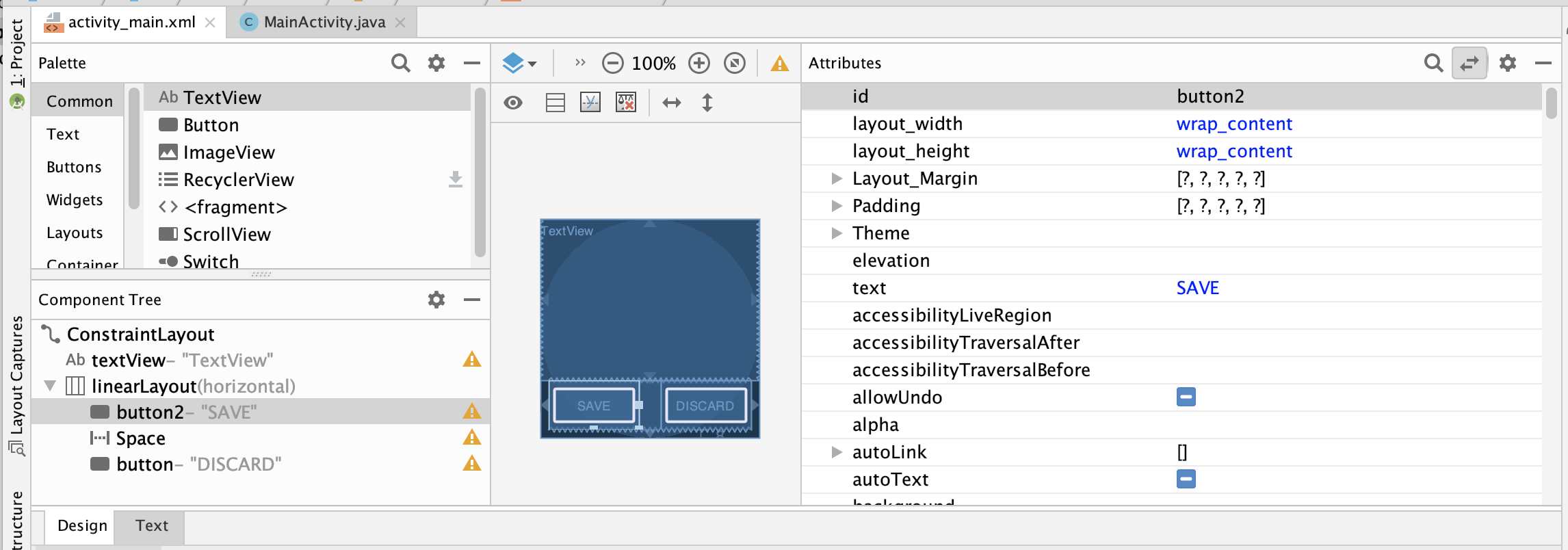 --- # Layout Types in Android - [FrameLayout](https://developer.android.com/reference/android/component/FrameLayout.html) - good for position views on top of each other, or encapsulating a bunch of views. Used in [Doodle](/assignments/doodle). - [__LinearLayout__](https://developer.android.com/reference/android/component/LinearLayout.html) - places views one after the other in order according to the orientation (Horizontal or Vertical). Used in [Layout](/assignments/layout). - [__RelativeLayout__](https://developer.android.com/reference/android/widget/RelativeLayout) - Positions of the children are desribed in relation to one another - [__TableLayout__](https://developer.android.com/reference/android/component/TableLayout.html) - Rows and columns style way of declaring a layout - [GridLayout](https://developer.android.com/reference/android/component/GridLayout.html) - Uses an [*adapter*](https://developer.android.com/reference/android/interactor/Adapter.html) that provides items to display in a grid - [ConstraintLayout](https://developer.android.com/reference/android/component/ConstraintLayout.html) Let's you use constraints to specify how things should lay out. Used in [Layout](/assignments/layout). - More on https://developer.android.com/guide/topics/ui/declaring-layout.html --- # Constraint Layout .left-column-half[ 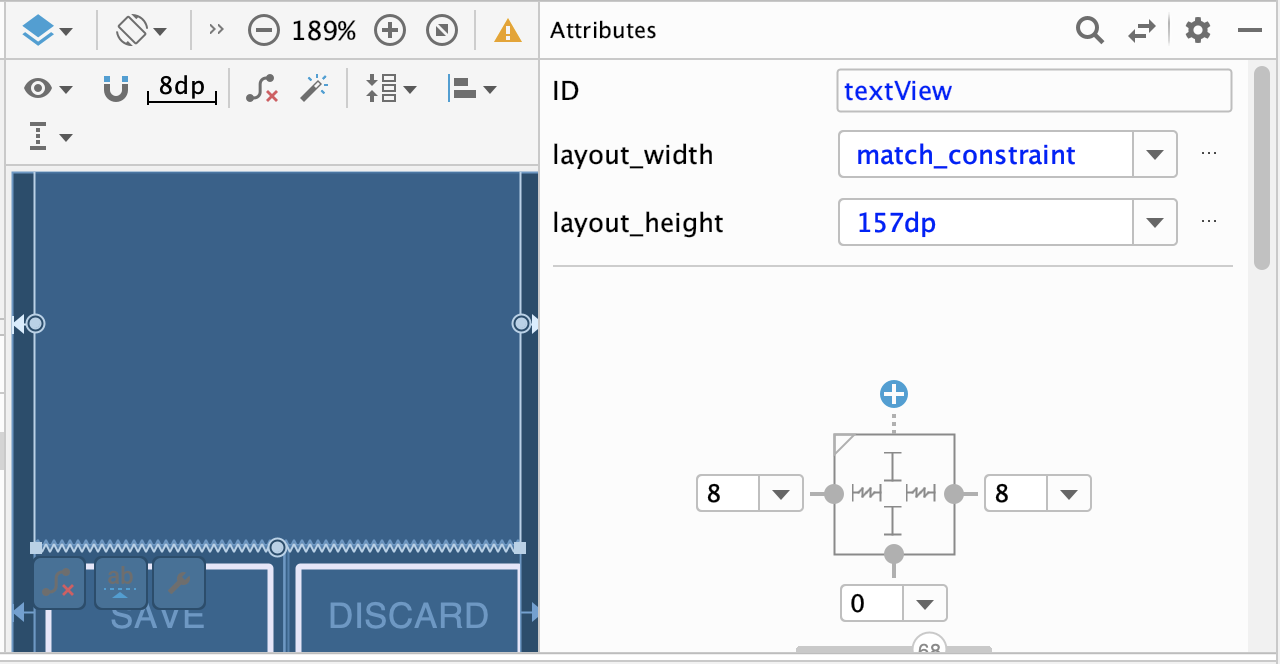 ] .right-column-half[ - ConstraintLayout is a ViewGroup that allows you to position widgets in a flexible way - Useful for building responsive interfaces in Android. - You can see little lines connecting the `textView` to its container and it's sibling (the `linearLayout`). - This specifies how it's attached (can change type by clicking on right) - If you were to change the interface (e.g. a different sized screen), it would stay attached and keep filling the space - All ends up in XML you can explore too ] --- # What are Constraints? .left-column[ 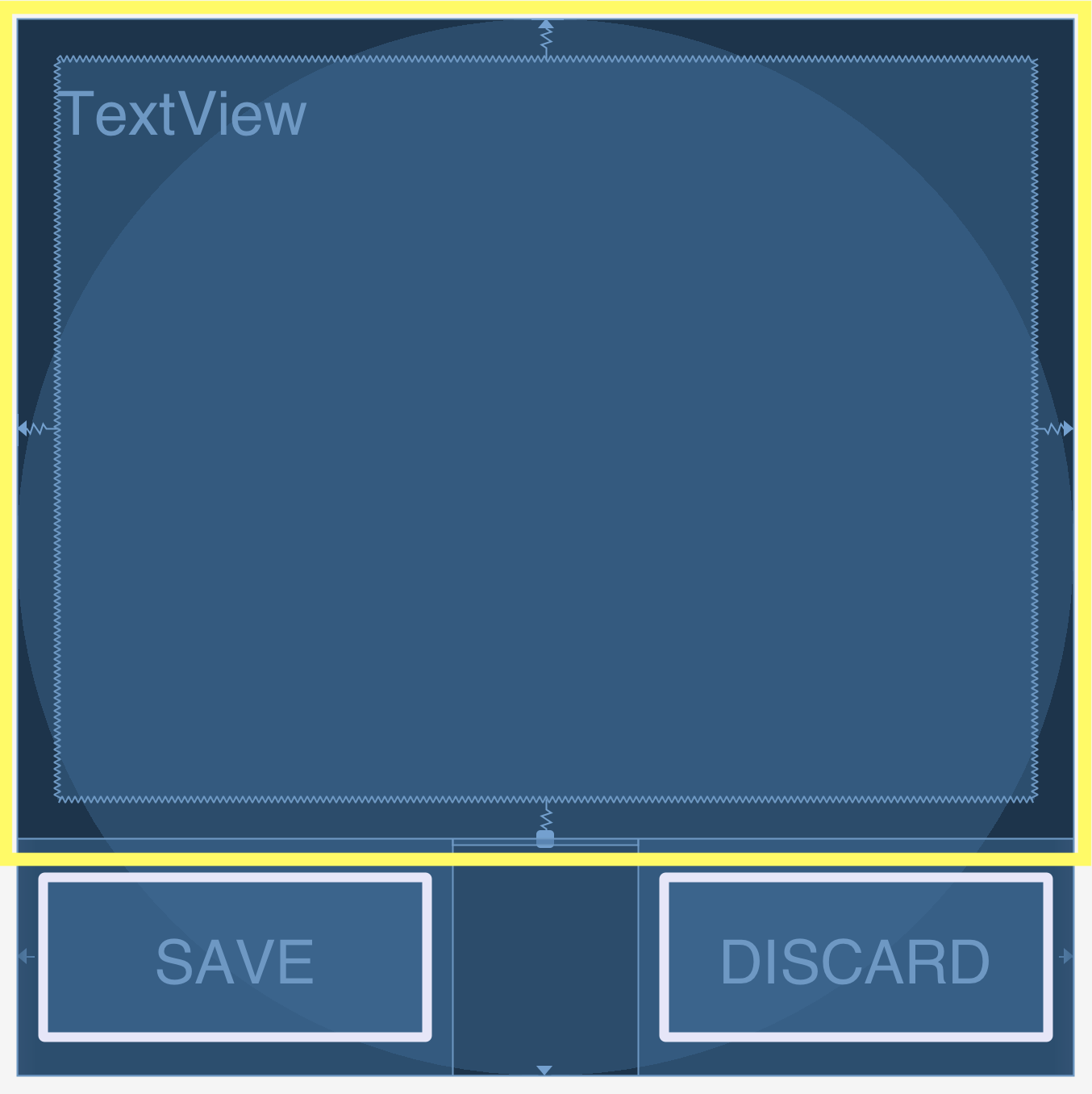] .right-column[ - Very general - Can reproduce most other things - Can operate on multiple axes - Can enhance other layout options ] --- # Worksheet: Interactor Hierarchy What would be the Component Tree for the Layout Part 1-2 program? .left-column-half[ 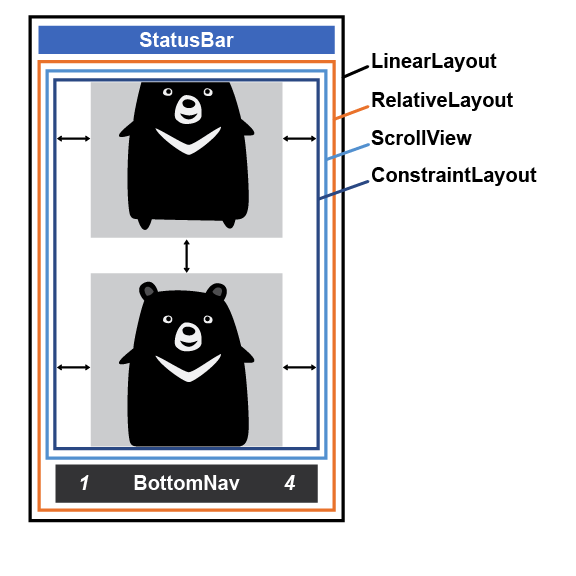 ] -- .right-column-half[
graph TD LL(LinearLayout) --> S[StatusBar] LL --> RL[RelativeLayout] RL --> SV[ScrollView] RL --> BN[BottomNav] SV --> CL[ConstraintLayout] CL --> V2[ImageView] CL --> V3[...] CL --> V4[ImageView] class LL,RL,SV,CL darkblue class S,SV,BN,V2,V3,V4 blue
] --- # LayoutInflater - Accepts a valid XML file and converts it into a `View` object or interactor hierarchy. - In Part 3 you will code part of it in an XML file and the rest programmatically (Java). - Why? (Discuss about pros and cons of constructing layout with XML and programmatically) --- # XML vs. Programmatic (Java) .left-column-half[ - XML is easier setting up the Layouts - XML shows the Layouts directly - XML can be inflated many times ] .right-column-half[ 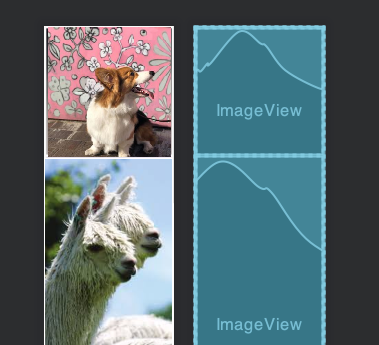 ] --- # XML vs. Programmatic (Java) - Programmatic is easier adding items to the Layouts - Programmatic can use loops - Sample solution of adding items into Layout in Part 1 (XML) has 55 lines - Sample solution of adding items into Layout in Part 2 (Java) has 17 lines --- # How do we use the LayoutInflater? 1. Setting up the LayoutInflater ```java // Obtains the LayoutInflater from the given context LayoutInflater.from(Context context) ``` 2. Inflate ```java // Inflate a new view hierarchy from the specified XML resource inflate(int resource, ViewGroup root) ``` --- # Worksheet: Quick Exercise Construct a LayoutInflater and pass in the `part1.xml` file. --- # Quick Exercise Solution ```java public Part1View(Context context, List
imageNames, int vMargin) { // Obtain the inflater from the context LayoutInflater inflater = LayoutInflater.from(context); // Inflate R.layout.part1 View newView = inflater.inflate(R.layout.part1, null); // newView is at the root of the inflated tree // Add it to this view this.addView(newView); } ``` --- # Previous Exam Question --- # Solution **What is the basic idea you have for how to fix it?** > Constrain the top of the scroll to the bottom of the text view. **Which view would you need to modify (provide the value you would set `android:id` to)?** > `@+id/scrollView` **What one line of XML would you add? Pseudocode ok here, you don't have to use exact names.** > `app:layout_constraintTop_toBottomOf="@id/textView"`