# CSE 340 Lab 2 (Spring 2020) ## Week 2: Get Help with Doodle .title-slide-logo[ 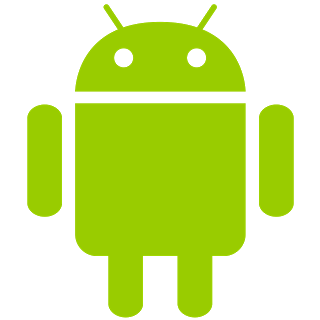 ] --- # Doodle Timeline - Doodle Due: Thursday, April 9 @ 10:00pm (**Today!**) - Lock: Saturday, April 11 @ 10:00pm (If you are using late days) - Peer Eval: Sunday, April 12 - Tuesday, April 14 @ 10:00pm - Reflection: Wednesday, April 15 @ 10:00pm --- # Lab 2 Objectives - Go through peer evaluation and reflection - How to debug in Android - Android Animation --- # Lab 2 After Implementation - Peer evaluation - fill the survey that we send out - The best ones will show off in class - Part 3: Turn in reflection on Gradescope after peer evaluation --- # Peer Evaluation - Why? - Chance to externalize your work - Get user feedback (the user experience is after all the point of HCI) - What to expect - Your grade won't be determined by peer evaluating alone - You get credit for **doing** the peer evaluation - Questions about spec requirements -- no room for interpretation - Questions that prompt about subjective feedback - Provide constructive feedback - Anonymous to your peers (but not to the teaching staff) - Your feedback on the exercise! (be honest) --- # Peer Evaluation Practice - Download the apk file: https://tinyurl.com/wdgjkcu - Google form: https://tinyurl.com/DoodleTestEval (Please use your UW Net ID) --- # Peer Evaluation Instructions .right-column-staff[ - You will be assigned to peer grade 3 assignments via email - If you have an Android phone - Click on the download link to install the app on your phone - If you don't have a phone - Download the APK files - Open the APK file in Android Studio and run the app in the emulator - Fill out the google form for each APK file ] .left-column-staff[
] --- # Android Debugging - Select a device to debug your app on - Set breakpoints in your Java, Kotlin, and C/C++ code - Examine variables and evaluate expressions at runtime - How to use Debugger: https://developer.android.com/studio/debug#java - Helpful tool (Logcat): https://developer.android.com/studio/debug/am-logcat#java --- # Animation on Android - _Property Animation_ - preferred method; more flexible - _View Animation_ - simple setup; the old way (but you have to deal with xml...) - _Drawable Animation_ - load `Drawable` resources and display them one frame after the other (it is like a gif) --- # Property Animation - Define an animate that changes on object's _property_ (a field in an object) over _a length of time_ ## `ValueAnimator` - Keeps track of the animation's timing (how long its been running and current value of property) ```java ValueAnimator animation = ValueAnimator.ofFloat(0f, 1f); animation.setDuration(1000); // Starts the animation animation.start(); // TODO: need to listen for updates to get the returned value ``` --- # `ObjectAnimator` (the one you need in this assignment) - Rather than listening for a value, we can simply directly animate a property on an object - **However**: the property that you are animating must have a setter function (in camel case) in the form of set
() for this to work (_setDuration()_) ```java ObjectAnimator anim = ObjectAnimator.ofFloat(textView, "alpha", 0f, 1f); anim.setDuration(1000); anim.start(); ``` --- # Resources - Vogella Tutorials - http://www.vogella.com/tutorials/AndroidAnimation/article.html - Android Developer - View Animation - https://developer.android.com/guide/topics/graphics/view-animation.html - Property Animation - https://developer.android.com/guide/topics/graphics/prop-animation.html - Relevant supplemental material is provided on the course website - Listed in the Doodle assignment - Don't stress out :) this isn't required