# CSE 340 Lab 1: Doodle (Spring 2020) ## Introduction to Doodle .title-slide-logo[ 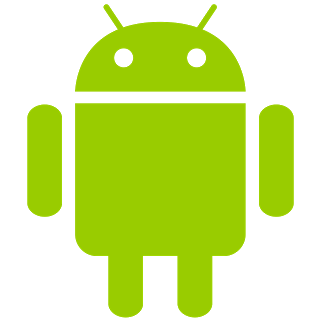 ] --- .left-column[ ## **Library** (and Inheritance Hierarchy)
graph LR Activity[Activity] Activity -->|...| Doodler[Doodler] Doodler --> Part1[Part1] Part1 --> Part1Activity[Part1Activity] Part1 --> Part2Activity[Part2Activity] classDef yellow font-size:19px,text-align:center classDef green font-size:19px,text-align:center class Part1,Part1Activity,Part2Activity yellow class Activity,Doodler green
] .right-column[ ## Android Classes we are using in Doodle and how they relate - Doodler (which you don't edit) *extends* [Activity](https://developer.android.com/reference/android/app/Activity) - Part1 *extends* Doodler. It implements *helper methods* for Part1Activity and Part2Activity. - Part1Activity and Part2Activity both extend Part1 ] --- layout:none .title[Running Sample Code] .body[ When you accept the assignment on GitGrade, a repository containing the starter code is generated for you on CSE GitLab. You **MUST** work within this assignment, as it will be turned in via GitGrade when it is due. Please clone and use the repository and commit and push your work regularly. Not only will doing so protect your code, but it will also allow course staff to look at your code and it will allow you to easily pull any changes we make to the assignment source.You can find instructions on setting up and maintaining a forked repository [here](https://help.github.com/en/articles/working-with-forks). ] --- ## Cloning Doodle .left-column[ You can find your unique repo by the notification email or by going to [GitLab](https://gitlab.cs.washington.edu) or via the [GitGrade](https://gitgrade.cs.washington.edu) interface. ] .right-column[ 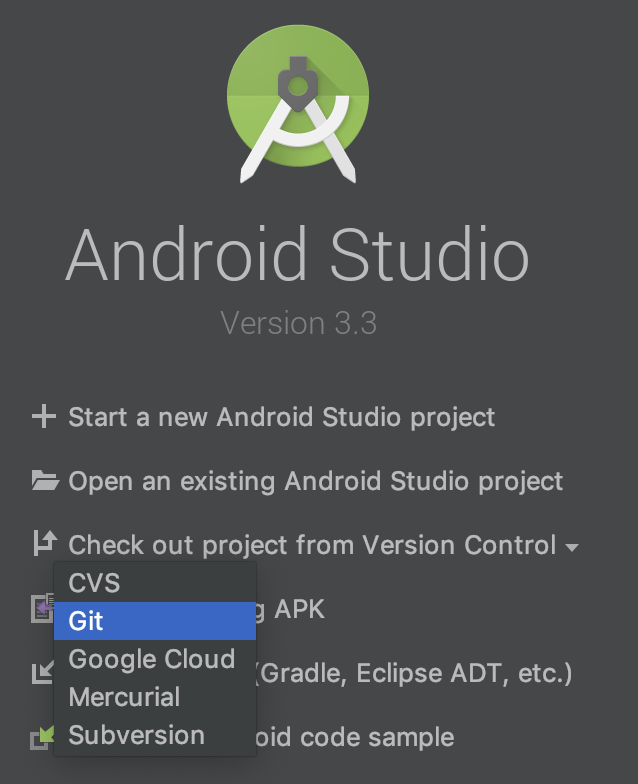 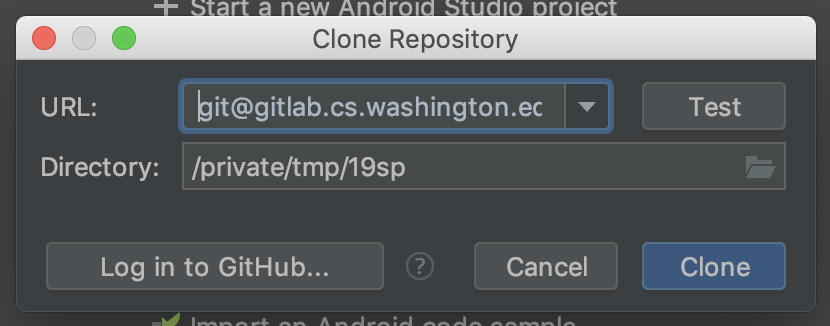 ] --- .title[Open project in Android Studio] .body[ - Run configurations should be automatically imported from Gradle - If not, `build` should trigger an import - Run with ► - Connect an android device by USB or create a new virtual device - If by USB, debugging must be enabled on the device ] --- .title[Implementing `addImage`] .body[ ```java private ImageView addImage(FrameLayout doodleView, String imageName, Float x, Float y, int size); ``` ### Params: - `doodleView`: Canvas in which to render the image. - `imageName`: Filename of image to draw in `res/drawable`. ] -- .body[ - `x`: Horizontal distance from top-left corner of canvas to top-left of image. - `y`: Veritcal distance from top-left corner of canvas to top-left of image. - `size`: Width and height of rendered image, in pixels. ] --- .title[Implementing `addImage`] .body[ ### Returns: - An `ImageView` which has been added to the canvas. ] --- .title[Implementing `addImage`] -- .body[ Break down into component steps, look up documentation, and implement 1) Create `ImageView` ] -- .body[ 2) Add new view to canvas ] -- .body[ 3) Position and set view size ] -- .body[ 4) Set view contents ] --- .title[Implementing `addImage`] .body[ ```java private ImageView addImage(FrameLayout doodleView, String imageName, Float x, Float y, int size) { // Create ImageView and add it to doodleView. ImageView image = new ImageView(this); // Add image to doodleView // Set image size and position // Set image contents using filename return image; } ``` ] --- .title[Implementing `addImage`] .body[ ```java private ImageView addImage(FrameLayout doodleView, String imageName, Float x, Float y, int size) { // Create ImageView and add it to doodleView. ImageView image = new ImageView(this); // Add image to doodleView doodleView.addView(image); // Set image size and position // Set image contents using filename return image; } ``` ] --- .title[Implementing `addImage`] .body[ ```java private ImageView addImage(FrameLayout doodleView, String imageName, Float x, Float y, int size) { // Create ImageView and add it to doodleView. ImageView image = new ImageView(this); // Add image to doodleView doodleView.addView(image); // Set image size image.getLayoutParams().height = size; image.getLayoutParams().width = size; // Set image position ? // Set image contents using filename return image; } ``` ] --- .title[Breakout Room Discussion] .body[
] --- .title[Implementing `addImage`] .body[ ```java private ImageView addImage(FrameLayout doodleView, String imageName, Float x, Float y, int size) { // Create ImageView and add it to doodleView. ImageView image = new ImageView(this); // Add image to doodleView doodleView.addView(image); // Set image size image.getLayoutParams().height = size; image.getLayoutParams().width = size; // Set image position ? // Set image contents using filename int resID = getResources().getIdentifier(imageName, "drawable", getPackage()); image.setImageResource(resID); return image; } ``` ] --- .title[Android Debugging Tips] .body[ Resource: [https://developer.android.com/studio/debug](https://developer.android.com/studio/debug) ]