name: inverse layout: true class: center, middle, inverse --- layout: false # Lab 2: Layout in Android
--- layout: false # Goals for today - Peer grade Doodle exercise - Layouts in Android - Examples - How do you create layouts? - ConstraintLayout in Android - Code along a simple ConstraintLayout --- layout: false # Peer Grading - Why? - Chance to externalize your work - What to expect - Your grade won't be determined by peer grading alone - Questions about spec requirements -- no room for interpretation - Questions that prompt about subjective feedback - Provide constructive feedback - Anonymous to your peers (but not to the teaching staff) - Your feedback on the exercise! (be honest) --- layout: false # Peer Grading Instructions - You should have been assigned to peer grade 4 assignments - If you have an Android phone - Click on the download link to install the app on your phone - If you don't have a phone - Download the APK files - Open the APK file in Android Studio and run the app in the emulator - Fill out the google form for each APK file
--- layout: false # User Interfaces on Android - Views - Base class for __all__ UI elements - Controls (switches, sliders, etc) - UI Components - typical display elements mentioned in SSUI lecture (e.g buttons, labels, image views, etc) - ViewGroups - Encapsulates one or more views (e.g. Android Components, Layouts) - Can define specific **layout** properties - Layout - Defines the structure for the user interface (UI) of your app - View and ViewGroup objects live within Layouts
--- layout: false # Layout Types in Android - [FrameLayout](https://developer.android.com/reference/android/component/FrameLayout.html) - good for position views on top of each other, or encapsulating a bunch of views - [__LinearLayout__](https://developer.android.com/reference/android/component/LinearLayout.html) - places views one after the other in order according to the orientation (Horizontal or Vertical) - [__TableLayout__](https://developer.android.com/reference/android/component/TableLayout.html) - Rows and columns style way of declaring a layout - [GridLayout](https://developer.android.com/reference/android/component/GridLayout.html) - Uses an [*adapter*](https://developer.android.com/reference/android/interactor/Adapter.html) that provides items to display in a grid - [ListView](https://developer.android.com/guide/topics/ui/layout/listview.html) - Uses an [*adapter*](https://developer.android.com/reference/android/component/Adapter.html) class that provides items to display in a list - [__RelativeLayout__](https://developer.android.com/reference/android/component/RelativeLayout.html) - positions views with respect to on another - [WebView](https://developer.android.com/reference/android/webkit/WebView.html) - uses html/css to layout a page, but requires `INTERNET` permission in your manifest - More on https://developer.android.com/guide/topics/ui/declaring-layout.html --- layout: false # Layouts in Play
FrameLayout
TableLayout
LinearLayout
GridLayout
ListView
RelativeLayout
--- layout: false # Declaring Layouts - Declare UI elements in XML: Android provides XML vocabulary that corresponds to specific views / layouts - Alternatively, you could also use Android's Layout Editor tool - Instantiate layout elements at run time - Programmatic v. XML - Depends on the developer - Starting with XML is considered to be better style --- layout: false ## ConstraintLayout in Android - ConstraintLayout is a ViewGroup that allows you to position widgets in a flexible way - Useful for building **responsive** interfaces in Android. - Will be using ConstraintLayout for the Layout exercise - Short exercise using ConstraintLayout for the remaining time. - https://developer.android.com/reference/android/support/constraint/ConstraintLayout --- template: inverse .center[ [Code Along!](https://github.com/harshitha-akkaraju/layoutlab) ] --- .left-column[ # How Android Draws Views (1/2) ] .right-column[ When an `Activity` receives focus, Android requests the activity draw its layout ] -- .right-column[ An activity __must__ provide a root node of the layout hierarchy ] -- .right-column[ The root node will measure and draw the layout tree ] --- .left-column[ # How Android Draws Views (2/2) ] .right-column[ __In-order tree traversal__ is done on the layout hierarchy.. ] -- .right-column[ Each view is responsible for drawing itself before (i.e. __behind__) their children ] -- .right-column[ Siblings are drawn in the order that they appear .center.half-width-img[ 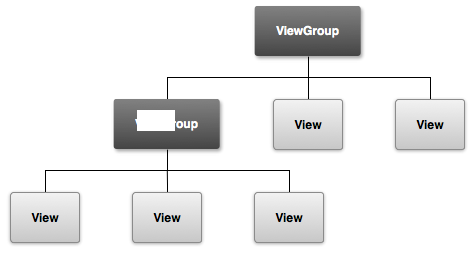 ] ] --- .left-column[ # When to (re)Draw? ] .right-column[ Drawing is costly- (e.g. frame rates dropping for scrolling interactions) ] -- .right-column[ Often the screen is static ] -- .right-column[ We should *draw when we need to* ] -- .right-column[ Re-drawing "damaged" areas: call `invalidate()` ] --- .left-column[ # The Drawing Process ] .right-column[ 1) Android calls measure on root node (view) ```java // View specifies size of self protected void onMeasure(int widthMeasureSpec, int heightMeasureSpec); ``` ] -- .right-column[ 2) Android calls layout on view ```java // View specifies size and position of children protected void onLayout(boolean changed, int left, int top, int right, int bottom); ``` ] -- .right-column[ 3) Android calls draw on view ```java // Add the drawing happens protected void onDraw(Canvas canvas); ``` ] --- .left-column[ # Measuring ] .right-column[ `onMeasure()` is a callback invoked by `View.measure()` ] -- .right-column[ Guidance about the size is given from parent view in the form of `MeasureSpec` parameters ] -- .right-column[ Ensures every view has a width and height value set prior to a layout pass ] -- .footnote[ Note: You must manually take padding into account (subtract padding from the width/height dimensions) ] --- .left-column[ # Layout ] .right-column[ `onLayout()` is a callback triggered when a view's position is changed ] -- .right-column[ Gives the new position __relative__ to the parent ] -- .right-column[ Causes a layout call on all children (!) ]
layout: true