Useful CSE 163 Resources¶
Tip
See the introductory video for this assignment here.
Welcome to your first take-home assessment! In every assessment, we will define a set of problems we would like you to solve. Even if the assessment is solving some larger problem, we usually break it up into small sub-problems for you to help manage the work. For this assessment, we have already implemented some buggy solutions to these problems. Your job is to identify and fix the bugs.
-
hw0.py
is the (potentially buggy) code for the problems described below.hw0.py
is not a runnable program, so we don’t use the main-method pattern. -
hw0_test.py
is the file for you to put your own tests. The Run button executes this program. -
cse163_utils.py
is a helper file that has code to help you test your code. You do not need to look at or modify this file ever.
Useful Buttons
Run executes python hw0_test.py
to run your tests.
Check executes flake8
on all Python files.
Mark submits your work and runs our automated tests.
funky_sum
¶
Write a function funky_sum
that does a special sum to combine two numbers. An initial (but incomplete) implementation has already been done for you! We will revisit funky_sum
later in the spec to fix a bug in this initial code.
The function should take three parameters, the first two are numbers a
and b
to combine and a third mix
is a number to determine the ratio to use from each. mix
acts as a “slider” to control how much to use of each number. If mix
is 0 or less, the result should be the same as a
. On the other hand, if mix
is 1 or more, the result should be the same as b
. For any value of mix
between 0 and 1, it should add 1-mix
times a
and mix
times b
. The technical name for this operation is “linear interpolation”.
-
funky_sum(1, 3, 0.5)
returns2.0
. -
funky_sum(1, 3, 0)
returns1
. -
funky_sum(1, 3, 0.25)
returns1.5
. -
funky_sum(1, 3, 0.6)
returns2.2
. -
funky_sum(1, 3, 1)
returns3
.
total
¶
Write a function total
that takes a number n
and returns the sum of the integers from 0 (inclusive) to n
(inclusive). If n
is negative, the function should return the value None
instead. This has already been done for you, so you will not need to modify any code for this function!
Documenting code¶
Task: Document the code in hw0.py
and hw0_test.py
.
There is no documentation in the provided code! We recommend writing your documentation while you code to build up the habit. Writing documentation for your function is a great way to start to get you thinking about what your method needs to do. You should go through and add the following documentation:
-
Each function in
hw0.py
andhw0_test.py
should have a doc-string comment describing their parameters, returns and behavior. -
Each of
hw0.py
andhw0_test.py
should have a doc-string comment as the first lines of that file. This comment should describe what the whole file is for. A doc-string for a file looks the same as a doc-string for a function, but goes as the first lines of the file. Each doc-string for the file should have your name and your section in it as well. Since this assignment doesn’t really have a context, the description forhw0.py
can just say something like “Implements the functions for HW0”.
Importantly, it helps to think about who the audience of your comments will be. They are interested in knowing WHAT your function does and how to call it, they are not looking for you to simply rewrite the code in English. You commonly want to avoid phrases that are telling the reader how you accomplish something (e.g. “uses a for loop”) since that is a little too detailed into the specific implementation. Instead, describe your function at a high-level and make sure to describe any import edge-cases that the reader might want to know about. For example, does your function ever return None
?
flake8
¶
Task: Fix all of the flake8
errors reported by the Check button.
The provided code has some errors according to flake8
. Fix them so that flake8
reports no errors. See the Flake8 Rules website for details on how to diagnose and fix these errors.
Testing¶
Task: The provided test function for total
doesn’t test a really important case described in the spec for that problem. Write a test-case in that function to test that special case is handled correctly.
We use testing to help us feel confident that the programs we write are correct. Testing involves calling the functions you wrote with some input and comparing the output with some expected value.
We can’t just call functions defined in hw0.py
from hw0_test.py
since they come from a different file. To fix this, we have to import hw0
in hw0_test.py
and any time we call a function from hw0.py
we have to prefix it with hw0.
(e.g. hw0.total(10)
). You do not need to understand how imports work yet, just know that you will need to use this syntax to call the functions you define in your hw0.py
.
We have provided a function called assert_equals
that takes an expected value and the value returned by your function, and compares them: if they don’t match, the function will crash the program and tell you what was wrong. The starter file hw0_test.py
has some test-cases already included to show you an example of how to write tests. Note that assert_equals
is defined in cse163_utils.py
, but imported in a special way so you don’t have prefix all the function calls with cse163_utils
.
More testing¶
Task: Write a function test_funky_sum
in hw0_test.py
that makes at least 4 calls to assert_equals
. At least two calls should be examples shown in the specification and at least two should be new examples you make up. Make sure this function is actually called by calling it from main!
You might have noticed that we only provided one test function even though there were three functions that were supposed to be “implemented” in this assignment. In general, you should have a separate test function for each function that you were supposed to write in the assignment to make sure you’re confident that it works.
This will be very helpful because there is some sort of bug in our starter code! When you press Mark, the provided code should fail some tests. To get you in the practice of writing your own tests and debugging your solution, our test cases from the Mark interface are intentionally vague. This will not be purposefully done in future assignments, but as we mentioned in section, it is a common fact that it is difficult to use the Mark interface to debug your code. The next problem asks you to fix the bug.
Fix the bugs¶
Task: Fix the bug(s) in funky_sum
. You should pass all of your own tests and all of ours. Your function can return any numeric type (e.g. int
, float
, etc…) and you will not need to perform any casting.
swip_swap
¶
Task: Write a function swip_swap
that takes a string source
and characters c1
and c2
and returns a copy of source
with all occurrences of c1
and c2
swapped. You may assume that the c1
and c2
are single characters. Use str
concatenation with the +
operator to solve this problem.
-
swip_swap('foobar', 'f', 'o')
returns'offbar'
. -
swip_swap('foobar', 'b', 'c')
returns'foocar'
. -
swip_swap('foobar', 'z', 'c')
returns'foobar'
.
In order to demonstrate the learning objectives for this problem, you should not use the string replace
function or use any data structures like list
to solve this problem.
Task: Write a function test_swip_swap
in hw0_test.py
that makes at least 4 calls to assert_equals
. At least two calls should be examples shown in the specification and at least two should be new examples you make up. Make sure this function is actually called by calling it from main!
Quality¶
Assessment submissions should pass two checks: flake8
and our code quality guidelines. The code quality guidelines are very thorough. For this assessment, the most relevant rules can be found in these sections:
-
-
Factoring
-
Loop Zen
-
Unnecessary Cases
-
For Java Programmers
Note that Python naming convention is snake_case
, not camelCase
!
Submission¶
Submit your work by pressing the Mark button. Submit as often as you want until the deadline for the initial submission. Note that we will only grade your most recent submission. You can view your past submissions using the “Submissions” button.
Please make sure you are familiar with the resources and policies outlined in the syllabus and the take-home assessments page.
A0 - Startup
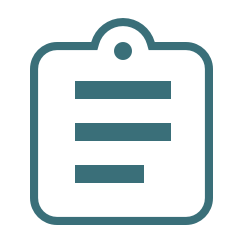
Initial Submission by Thursday 04/07 at 11:59 pm.
Submit on Ed