Goals
- Practice using functions, variables, declarations, and assignment statements.
- Make computations dynamic in interesting ways.
- Create an abstracted image of a recognizable family using simple shapes.
Setup
You may choose to start from scratch or use this Simpsons family code as a starting point.
Lego Family
You will program your very own lego family. You can base it off of a TV show (Simpsons is shown below, so you may not choose the Simpsons), a movie, a comic, or even your own family if you want to. You may look here for more inspiration, but your creation should be your own and not copied from somewhere else.
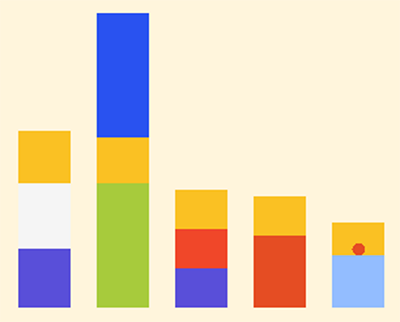
Specifications
- Background color is not white.
- The drawing canvas should be around 900x600, but adjust as needed for your project.
- Each character should be made out of rectangles, but feel free to add in more details with other shapes (like Maggie's pacifier -- an ellipse).
- At least three different fill colors should be used for your family.
- Your family must consist of a minimum of three characters.
Step 1: Sketch
Decide on the inspiration for your family. Sketch out your family on paper, color it in, and label all the important points so it is easier to program.
Note: You will be submitting your sketches, so keep them with you.
Step 2: Program Your Family
Program your family in the center of the screen, making sure each character lines up on the same baseline (see the Simpson family image).
Helpful Reminders:
- Positions on the screen are numbered from (0,0) in the upper-left corner and increase to the right and down. On a 900x600 drawing canvas, the middle position in the top row is (450,0).
- Sizes are always given width before height.
- Colors are given by three numbers between 0-255 for 'red', 'green', and 'blue' (RGB).
- An object's color is set with the
fill(r,g,b)
function, where r, g, and b are the three color values. Once set, all following shapes are filled with that color until a newfill()
command changes the color. noStroke()
will remove the outlines around your shapes.- Use [Help -> Reference] if you want to look up a function.
Step 3: Add Variables
At the top of your code, add an x_position
and y_position
variable for each family member, then add these variables into your code as shown in class.
Examples for the Simpsons variables are shown below.
Run your code, and make sure each character is now starting in a corner.
Character | Variable Names | ![]() |
|
Homer | homer_x, homer_y | ||
Marge | marge_x, marge_y | ||
Bart | bart_x, bart_y | ||
Lisa | lisa_x, lisa_y | ||
Maggie | maggie_x, maggie_y |
Declaring Variables:
To understand our programs Processing needs to know what variables we will use in our program, so we declare them.
Declarations come at the start of our program (before setup()
) by writing code such as int position = 0;
.
This line of code tells Processing several things:
position
is a variable that will be used in the program.position
will have integer values (whole numbers) because we used the abbreviationint
for integer.position
starts with the value 0.
Using Variables:
A variable holds a value that can change.
For example, if the variable position
holds the value 21 and then later in the program we write position = 23;
, then the value of position
has changed from what it was (21) to 23.
Variables are important to dynamic programs, because values change all the time.
Step 4: Move Your Family
Program your family to start in the corners and move to the center of the screen. If you have 3 characters, pick 3 different corners; if you have 4 characters, use all 4 corners; if you have more than 4 characters, you can have multiple characters start in the same corner.
You will be adding statements like homer_x = homer_x + 1;
or homer_x = homer_x - 1;
into your code to make this movement happen.
Here we are using the fact that the draw()
routine refreshes the image several times per second.
Each time it runs draw()
it repeats the "change" operation (such as homer_x = homer_x + 1;
).
This alters the variable's value.
By using that variable to set the position of shapes, we can make your characters move across the screen!
![]() |
==> | ![]() |
Step 5: Stop Your Family
Finally, you will want to program your family to stop once it has reached the center.
To do this you will want to use the min()
and max()
functions as shown in class.
For the Simpsons, we might use something like homer_x = min(0, homer_x);
OR homer_x = max(0, homer_x);
Submission
- Make sure that most of your lines of code have comments so that someone else can understand it.
- Submit your sketch from Step 1 (
.jpg
,.png
, or.pdf
) along with your finished.pde
file from Step 5 to the Canvas assignments page. - You should add this program to your portfolio.