Now we want these events to actually cause the page to update, rather than just opening popup boxes. We can't just call the timeZone() function, because we don't know which radio button has been clicked and the timeZone() function requires that information to be included inside the parentheses.
So let's create another function that will figure out which radio button has been selected. Then we can use that information to call the timeZone() function.
We'll call this new function update().
1. Go to the input box and the drop-down list. You've put an alert statement inside an onkeyup attribute for the text box and inside an onchange attribute for the drop-down box. Replace these alert statements with this function call:
update();
Now you will actually create that function.
2. Go to the <head> section of your document and find the <script> tags. Your timeZone() function is inside of those script tags. Write the new function inside the <script> tags too. You can put it before or after the timeZone() function. Type this:
function update()
{
}
3. Put an alert statement in the function (have it say something like "the update function is working").
4. Save your file. Open it in a browser. Switch the am/pm value in the drop-down box. You should see the popup box you just created.
If you don't, try to figure out what happend. This is what should have happened:
When you changed the am/pm value, it triggered the "onchange" event for the drop-down list. The onchange event called the update() function. The update function carried out the instruction between the curly brackets which said to open a popup box.
Now we want to figure out which radio button has been selected.
We can find out if a given radio button is selected because each radio button has a "checked" status. We can retrieve that checked status with this statement: document.getElementById().checked.[ Note the .checked at the end.]
5. Remove the alert statement in the update() function and replace it with the following alert statement which checks whether the Hawaii radio box is checked:
alert(document.getElementById("zone0").checked);
6. Save your file and open it in a browser. Enter a number in the text box, or choose am/pm from the drop-down list. A popup box should appear saying "true" if the Hawaii radio box was checked. Or "false" if it wasn't.
We've checked to see if the Hawaii radio button is selected, but if it isn't, we don't know which was is. So we need to look at all 7 radio buttons until we find the one that was selected. Rather than writing the line 7 times, we can write a loop. Each time we run the loop, we'll check on a different radio button.
7. Write the code below inside of the update() function. Put the alert statement inside of this loop (between the curly brackets). Since there are 7 time zones, this statement is written to cause the loop to run 7 times:
for(i = 0; i <7; i++)
{
alert(document.getElementById("zone0").checked);
}
This code will check the Hawaii radio button 7 times because each time it runs the loop, it looks for the element with id="zone0" (which is the Hawaii radio button). The other radio buttons have these id values: zone1, zone2, zone3, zone4, zone5, zone6.
So how do we make the loop look at zone0 the first time, zone1 the second time, zone2 the third time etc...?
Notice that i changes with each loop. The first time, i=0, the second time i=1, the third time i=2. These line up nicely with the zone names. When i=0, we want to look at zone0. When i=1, we want to look at zone1 etc. So we can modify the alert statement by replacing "zone0" with "zone" + i.
8. Go ahead and make this modification to the alert statement. Now if you open this file in your browser and select a radio button, you'll see seven pop-up boxes (a new one will appear each time you close the old one). 6 of the popup boxes will say "false" and one will say "true" because 6 buttons are not selected and one is.
The selected one is the only one we care about. When we find it, we want to call the timeZone() function (which will then calculate the new time and modify the page).
We can use an if statement to tell us when we've found the selected radio button:
9. Change the code inside the loop by removing the alert statement and adding this if statement (which contains another alert statement):
if(document.getElementById("zone0").checked)
{
alert("this is the selected radio button");
}
This statement says "if the element with an id of "zone0" is checked, then open a popup button that says 'this is the selected radio button''
Now, if you save the file, open it in a browser and type in a new number, you should see just one popup box. The loop ran 7 times, but it only found 1 selected radio button so it only produced 1 popup box.
Ultimately, instead of creating a popup box, we want to run the timeZone() function. But to call that function, we need to know how many hours ahead, or behind the Pacific Time Zone we are.
To be able to do this, let's give each radio button a value attribute and set it equal to the time zone difference for that radio button (for example, the Hawaii radio button should get an attribute that looks like this: value="-2" since Hawaii time is two hours behind Pacific time.
9. Give each radio button a value attribute and set it equal to the the time difference for that zone.
Now that we've done this, we can use document.getElementById().value to retrieve the time zone difference for whichever time zone has been selected.
10. Remove the old alert statement and write an alert statement that creates a popup box with the time difference (i.e. it will display "1" if Mountain time was selected and "-1" if Alaska time was selected).
11. Save the file, open it in a browser and enter a time to see if this is working properly.
Now we're ready to call the timeZone() function:
12. To do this, just replace the word "alert" with "timeZone".
13. Save your file and open it in a browser....voila! You should now be able to just type in a number, or just choose a different am/pm choice and see the calculated value update.
If your adjusted time looks like this screenshot, JavaScript is concatenating strings instead of adding numbers.
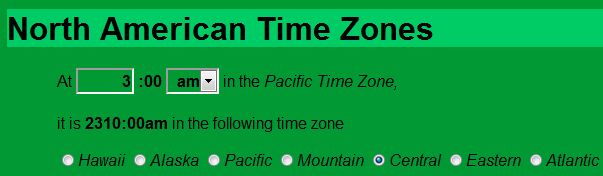
It's concatenating the difference in time zones (2), your new time (3), and the current time (10).
For it to concatenate instead of adding, one of your variables must be a string, even if it looks like a number!
Recall that all data from forms are strings until forced to be numbers.
Because you already used the Number() method to make hours into a number, zoneDiff must be the variable that is being treated as a string. Apply the Number() method to zoneDiff in this statement near the beginning of your header script:
var newTime = Number(zoneDiff) + hours;
Once all of your variables are numbers, your time-zone conversion will work again.
Review of what happens when you change a number:
1 The user types a number and the onkeyup attribute sends a message to run the update() function
2 The update function looks at all of the radio buttons. When it finds one that is checked, it sends a message to run the timeZone() function and includes the number of hours difference for that time zone
3 The timeZone() function checks to be sure the user entered a number between 1 & 12, then calculates the time in the selected time zone. If that time is less than 1:00, it adds 12 to the number and switches the am/pm value. If that time is greater than 12:00, it subtracts 12 from the number and switches the am/pm value.
4 Finally, the timeZone() function writes the new time on the page by replacing the contents of the <span> codes.
14. Upload your HTML file and the image to your network account on Dante so that you can view it at this URL:
http://students.washington.edu/yourUWnetID/fit100/Lab08&09/Lab08&09.html
15. Return to the Catalyst WebQ and answer the remaining questions.