Goals
- Practice controlling action on the screen and following the logic of the computation.
- Learn about events, which interrupt Processing when they occur (specifically
keyPressed()
andmousePressed()
).
Events
Step 1: Write the Starting Program
Write a Processing program that has an 800x100 drawing canvas and moves a red ball from left to right across the screen.
- The ball is 80 pixels in diameter and is centered vertically (i.e. same amount of space above and below the ball).
- The ball's horizontal position is an integer variable named
x_position
. - The ball's initial position is 100 pixels from the left.
- The ball moves one pixel each time the screen is redrawn.
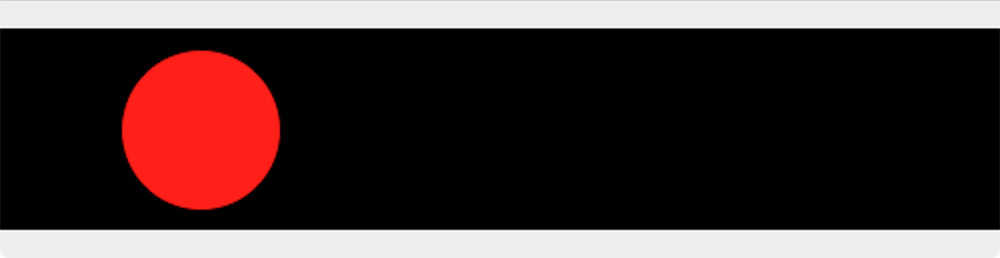
Step 2: Update Your Program with Variables
Declare four more integer variables at the top of your program:
Variable Name |
Initial Value |
Description |
move |
1 | The number of pixels the ball will move on each repetition of draw() (i.e. ball speed) |
rVal |
255 | The red ('R') value for the ball's fill |
gVal |
0 | The green ('G') value for the ball's fill |
bVal |
0 | The blue ('B') value for the ball's fill |
Now make the changes that are detailed below. Make sure to run your code after each change to ensure that it still works correctly!
- Change your program so the amount the ball moves each time
draw()
repeats ismove
instead of the value 1. - Change the RGB values in your
fill()
command, replacing them with the three variablesrVal
,gVal
, andbVal
. - Add a
keyPressed()
function at the bottom of your code to reverse the direction of the ball as shown below:void keyPressed() { move = -1 * move; }
- Add a
mousePressed()
function to the bottom of your code to change the ball's color everytime the mouse is pressed as shown below:void mousePressed() { int temp; temp = bVal; // save bVal (old) in a temporary variable bVal = gVal; // make new bVal the old gVal gVal = rVal; // make new gVal the old rVal rVal = temp; // make new rVal the old bVal }
Step 3: Understanding
Open a Google Sheet and set it up to have the form shown below.
Fill in the numbers for the first 10 clicks as they exist after the last assignment statement (rVal = temp;
).
Then explain in your own words (a short paragraph, in your spreadsheet) what is happening with these assignment statements in the mousePressed()
function.
Submission
- Make sure that your name is included in your file.
- Make sure that most of your lines of code have comments so that someone else can understand it.
- Make sure that the URL of your Google Sheet is included in the block comment at the top of your program.
- Submit your finished
.pde
file from Step 3 to the Canvas assignments page.